一、编辑单元格
Ext 中通过配置表格的属性 plugins 来设置表格是否可编辑,
表格的配置具体如下:
var gridTable = Ext.create('Ext.grid.Panel', {
id: 'gridTable',
region: 'center',
layout: 'fit',
columns: cols,
store: gridStore,
autoScroll: true,
selModel: { // 光标显示的是单元格模式
selType: 'cellmodel'
},
plugins: [
Ext.create('Ext.grid.plugin.CellEditing', {
clicksToEdit: 1 //设置单击单元格编辑
})
],
});
配置完表格还需要对表格列的 editor 进行配置,
才能实现单元格编辑,例如:
{
text: '姓名',
dataIndex: 'Name',
minWidth: 85,
maxWidth: 85,
sortable: false,
menuDisabled: true,
editor: {
xtype: 'textfield',
enableKeyEvents: false,
}
},
{
text: '列',
dataIndex: 'col',
120,
sortable: false,
menuDisabled: true,
renderer: function (a, b, c, rowIdx, colIdx) {
if ("object" == typeof (a)) {
var r = Ext.util.Format.date(a, 'H:i');
return r;
}
return "";
},
editor: {
xtype: 'timefield',
format: 'H:i',
enableKeyEvents: false,
}
}
效果如下:
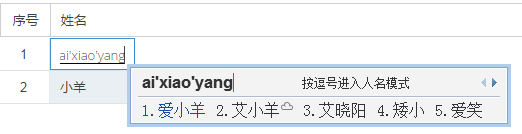
修改完按回车键,发现修改的单元格左上角有一个红色三角形,
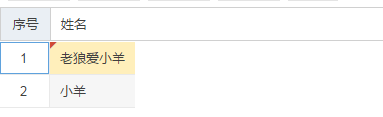
这是 Ext 用来标记修改过的单元格,
如果想要修改完后不显示红色图标,
可以调用 commit()。
例如可以在 gridTable 中绑定一个 'edit' 事件,
当检测到单元格数据发生改变的时候触发,
代码如下:
listeners: {
'edit': function (editor, e) {
e.record.commit();
},
}
二、右击单元格弹窗
首先先定义一个右键弹窗的面板,如下:
var contextmenu = new Ext.menu.Menu({
id: 'context-menu',
items: [{
id: 'context-menu-first',
rowIdx: 0,
colIdx: 0,
handler: function (m) {
if ("设置" == m.text) {
Ext.MessageBox.alert("提示", "设置成功");
}
}
}]
});
然后在 gridTable 中定义一个鼠标右击事件:
'itemcontextmenu': function (view, record, item, index, e) {
// 禁用系统默认右键菜单
e.preventDefault();
contextmenu.items.items[0].setText("设置");
contextmenu.showAt(e.getXY());
}
效果如下:
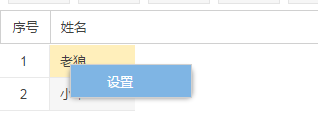
完整的代码:
1 <!-- 数据定义 -->
2 <script type="text/javascript">
3 var data; // 表格数据
4 var cols; // 表格列
5
6 var gridStore = Ext.create('Ext.data.Store', {
7 fields: ['Name']
8 });
9
10 </script>
11
12 <!-- 事件定义 -->
13 <script type="text/javascript">
14 // 初始化整个页面
15 function onInit() {
16 onLoadData();
17
18 onInitVar();
19 onInitColumn();
20 };
21 // 请求加载表格数据
22 function onLoadData() {
23 data = [{ 'Name': '老狼' }, { 'Name': '小羊' }];
24 gridStore.loadData(data);
25 };
26
27 // 初始化页面的变量参数
28 function onInitVar() {
29 cols = [
30 {
31 xtype: 'rownumberer',
32 text: '序号',
33 align: 'center',
34 minWidth: 50,
35 maxWidth: 50,
36 },
37 {
38 text: '姓名',
39 dataIndex: 'Name',
40 minWidth: 85,
41 maxWidth: 85,
42 sortable: false,
43 menuDisabled: true,
44 editor: {
45 xtype: 'textfield',
46 enableKeyEvents: false,
47 }
48 }
49 ];
50 };
51 // 初始化列
52 function onInitColumn() {
53 gridTable.reconfigure(gridStore, cols);
54 };
55
56 // 添加行
57 function onAddRow() {
58 gridStore.add({});
59 };
60 // 删除行
61 function onDelRow() {
62 gridStore.removeAt(gridStore.count() - 1);
63 };
64 // 添加列
65 function onAddColumn() {
66 cols.push({
67 text: '列',
68 dataIndex: 'col',
69 120,
70 sortable: false,
71 menuDisabled: true,
72 editor: {
73 xtype: 'textfield',
74 enableKeyEvents: false,
75 }
76 });
77
78 gridTable.reconfigure(gridStore, cols);
79 };
80 // 删除列
81 function onDelColumn() {
82 cols.pop()
83 gridTable.reconfigure(gridStore, cols);
84 };
85
86 // 保存
87 function onSave() {
88
89 console.log(gridTable);
90 console.log(gridStore.data);
91 };
92
93 </script>
94
95 <!-- 面板定义 -->
96 <script type="text/javascript">
97 var toolbar = Ext.create('Ext.form.Panel', {
98 id: 'tool-bar',
99 region: 'north',
100 bbar: [
101 {
102 xtype: 'button',
103 text: '添加行',
104 handler: onAddRow
105 },
106 {
107 xtype: 'button',
108 text: '删除行',
109 handler: onDelRow
110 },
111 {
112 xtype: 'button',
113 text: '添加列',
114 handler: onAddColumn
115 },
116 {
117 xtype: 'button',
118 text: '删除列',
119 handler: onDelColumn
120 },
121 {
122 xtype: 'button',
123 text: '保存',
124 handler: onSave
125 }
126 ]
127 });
128
129 //表格右键菜单
130 var contextmenu = new Ext.menu.Menu({
131 id: 'context-menu',
132 items: [{
133 id: 'context-menu-first',
134 rowIdx: 0,
135 colIdx: 0,
136 handler: function (m) {
137 if ("设置" == m.text) {
138 Ext.MessageBox.alert("提示", "设置成功");
139 }
140 }
141 }]
142 });
143 var gridTable = Ext.create('Ext.grid.Panel', {
144 id: 'gridTable',
145 region: 'center',
146 layout: 'fit',
147 columns: cols,
148 store: gridStore,
149 autoScroll: true,
150 selModel: { // 光标显示的是单元格模式
151 selType: 'cellmodel'
152 },
153 plugins: [
154 Ext.create('Ext.grid.plugin.CellEditing', {
155 clicksToEdit: 1 //设置单击单元格编辑
156 })
157 ],
158 listeners: {
159 'edit': function (editor, e) {
160 e.record.commit();
161 },
162 'itemcontextmenu': function (view, record, item, index, e) {
163 // 禁用系统默认右键菜单
164 e.preventDefault();
165
166 contextmenu.items.items[0].setText("设置");
167
168 contextmenu.showAt(e.getXY());
169 }
170 },
171
172 });
173 </script>
174
175 <!-- 脚本入口 -->
176 <script type="text/javascript">
177 Ext.onReady(function () {
178 Ext.create('Ext.Viewport', {
179 id: 'iframe',
180 layout: 'border',
181 items: [
182 toolbar,
183 gridTable,
184 ]
185 });
186
187 onInit();
188 });
189 </script>