1、概述:
- 设所排序序列的记录个数为n。i取1,2,…,n-1,从所有n-i+1个记录(Ri,Ri+1,…,Rn)中找出排序码最小的记录,
- 与第i个记录交换。执行n-1趟 后就完成了记录序列的排序。
- 在简单选择排序过程中,所需移动记录的次数比较少。最好情况下,
- 即待排序记录初始状态就已经是正序排列了,则不需要移动记录。
- 最坏情况下,即待排序记录初始状态是按逆序排列的,则需要移动记录的次数最多为3(n-1)。
- 简单选择排序过程中需要进行的比较次数与初始状态下待排序的记录序列的排列情况无关。
- 当i=1时,需进行n-1次比较;当i=2时,需进行n-2次比较;依次类推,
- 共需要进行的比较次数是(n-1)+(n-2)+…+2+1=n(n-1)/2,即进行比较操作的时间复杂度为O(n^2),
- 进行移动操作的时间复杂度为O(n)。
2、图解:
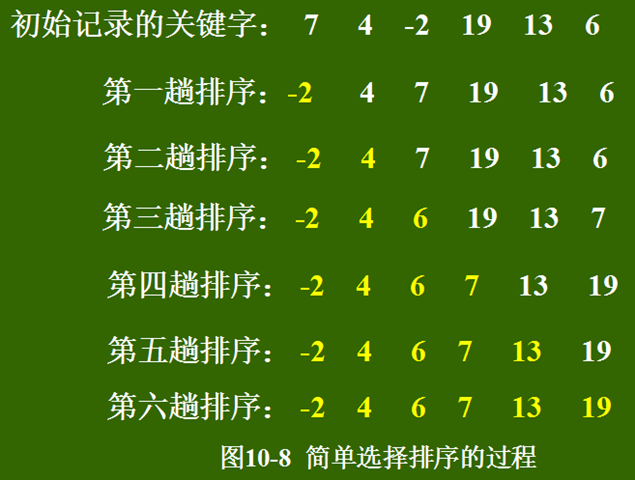
3、代码:
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5 using System.Threading.Tasks;
6
7 namespace 简单选择
8 {
9 class Program
10 {
11 static void SimpleSelectSort(int[] arry)
12 {
13 int tmp = 0;
14 int t = 0;//最小数标记
15 for (int i = 0; i<arry.Length; i++)
16 {
17 t = i;
18 for (int j = i + 1; j<arry.Length; j++)
19 {
20 if (arry[t] > arry[j])
21 {
22 t = j;
23 }
24 }
25 tmp = arry[i];
26 arry[i] = arry[t];
27 arry[t] = tmp;
28 }
29 }
30 static void Main(string[] args)
31 {
32 int[] data = new int[] { 42, 20, 17, 27, 13, 8, 17, 48 };
33 SimpleSelectSort(data);
34 foreach (var temp in data)
35 {
36 Console.Write(temp + " ");
37 }
38 Console.ReadKey();
39 }
40 }
41 }