一.邮件收发
1.什么是电子邮件
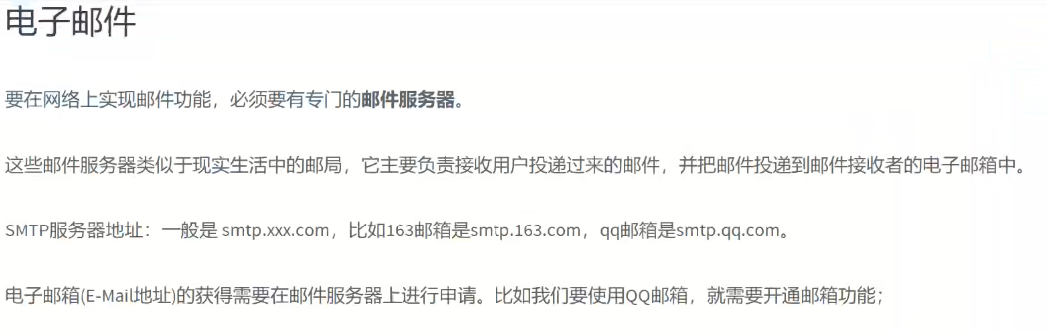
2.传输协议
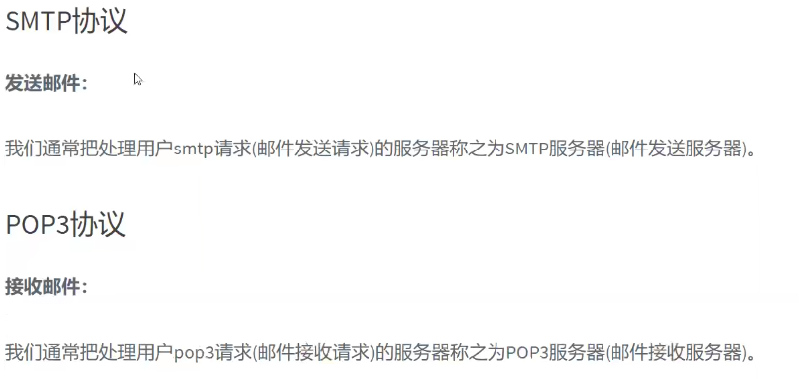
3.邮件收发原理
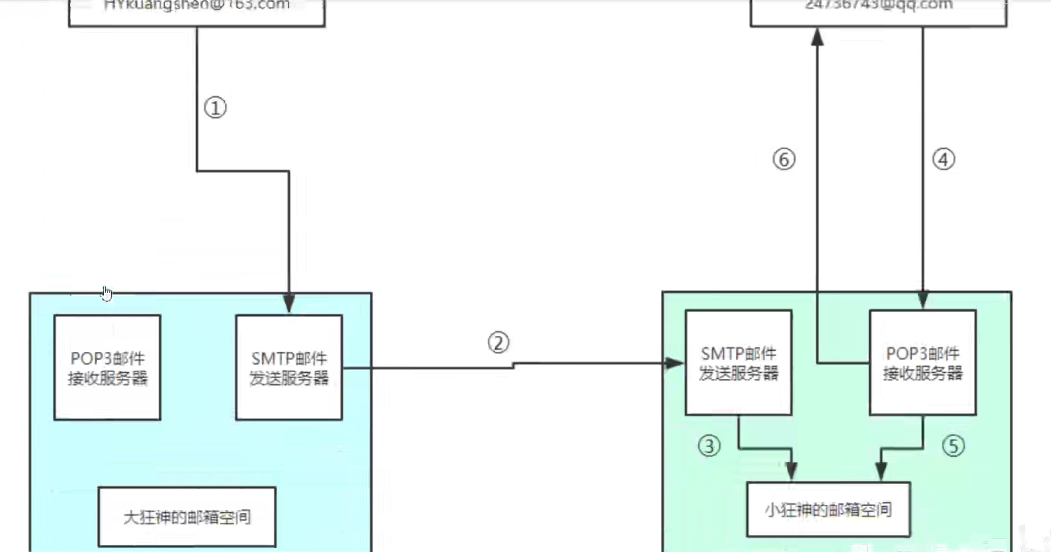
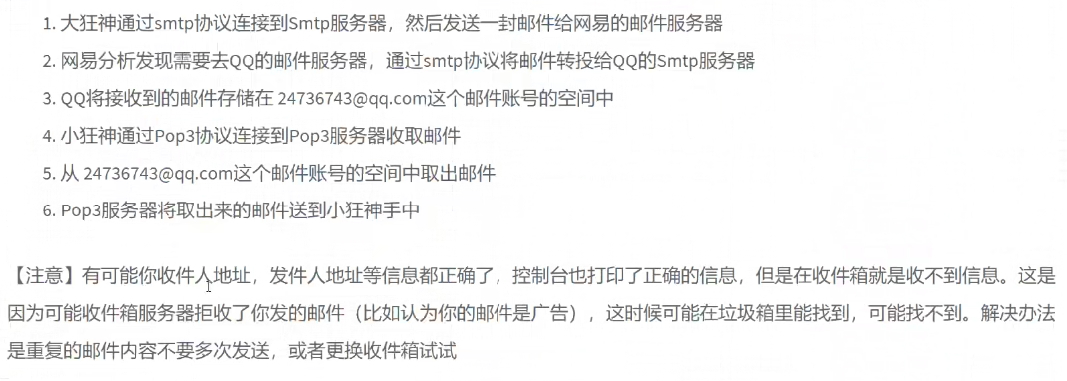
二.开发
1.导入jar包
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4.7</version>
</dependency>
<dependency>
<groupId>javax.activation</groupId>
<artifactId>activation</artifactId>
<version>1.1.1</version>
</dependency>
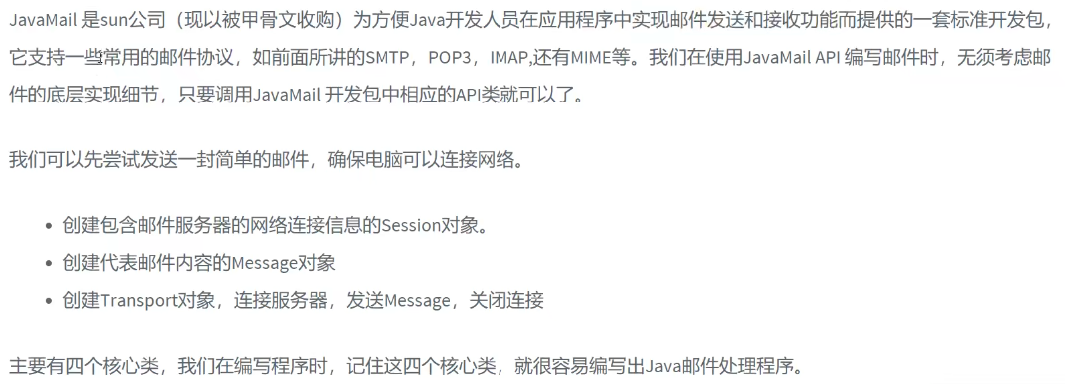
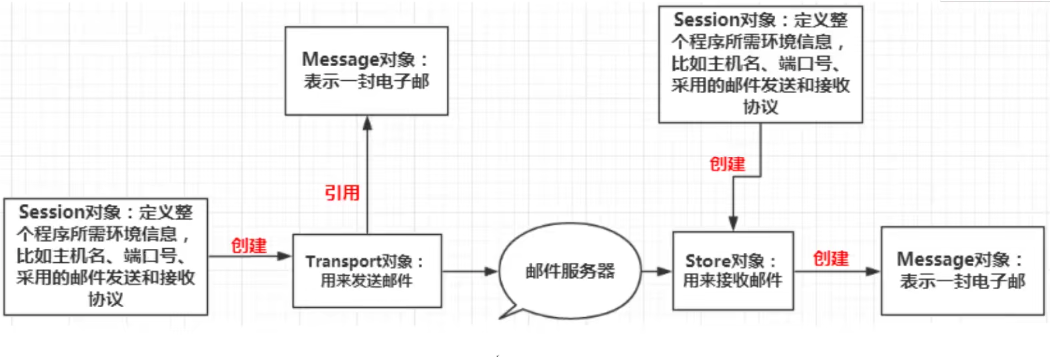
注:QQ邮箱开启POP3和SMTP服务还有授权码
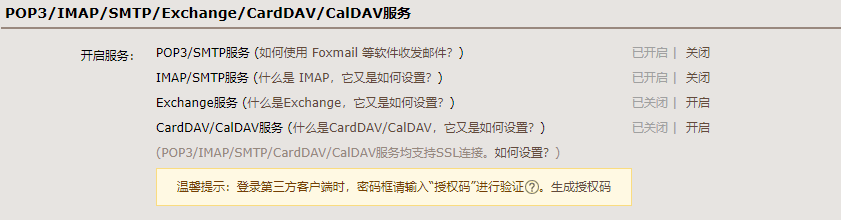
2.案例一:发送简单邮件
1 import com.sun.mail.util.MailSSLSocketFactory;
2
3 import javax.mail.*;
4 import javax.mail.internet.InternetAddress;
5 import javax.mail.internet.MimeMessage;
6 import java.io.UnsupportedEncodingException;
7 import java.security.GeneralSecurityException;
8 import java.util.Properties;
9
10 //发送一份简单的邮件
11 public class MailDemo01 {
12
13 public static void main(String[] args) throws GeneralSecurityException, MessagingException, UnsupportedEncodingException {
14
15 Properties prop = new Properties();
16 prop.setProperty("mail.host","smtp.qq.com"); //设置QQ邮箱服务器
17 prop.setProperty("mail.transport.protocol","smtp"); //邮件发送协议
18 prop.setProperty("mail.smtp.auth","true"); //需要验证用户名和密码
19
20 //关于QQ邮箱,还要设置SSL加密,加上以下代码即可,只有QQ邮箱需要其他邮箱不需要
21 MailSSLSocketFactory sf = new MailSSLSocketFactory();
22 sf.setTrustAllHosts(true);
23 prop.put("mail.smtp.ssl.enable","true");
24 prop.put("mail.smt;.ssl.socketFactory",sf);
25
26
27 //使用JavaMail发送邮件5个步骤
28 //1.创建定义整个应用程序所需的环境信息的Session对象
29
30 //QQ 才需要,其他邮箱不需要
31 Session session = Session.getDefaultInstance(prop, new Authenticator() {
32 @Override
33 protected PasswordAuthentication getPasswordAuthentication() {
34 //发件人邮件用户名和授权码
35 return new PasswordAuthentication("464407764@qq.com","hmiauriqbvzmbhcf");
36 }
37 });
38
39 //开启Session的debug模式,这样就可以查看到程序发送Email的运行状态
40 session.setDebug(true);
41
42 //2.通过Session得到transport对象
43 Transport ts = session.getTransport();
44
45 //3.使用邮箱的用户名和授权码连上邮件服务器
46 ts.connect("smtp.qq.com","464407764@qq.com","hmiauriqbvzmbhcf");
47
48 //4.创建邮件:写邮件
49 MimeMessage message = new MimeMessage(session);
50
51 //指明邮件的发送者
52 message.setFrom(new InternetAddress("464407764@qq.com","海天之恋", "UTF-8"));
53
54 //指明邮件的接收者
55 message.setRecipient(Message.RecipientType.TO,new InternetAddress("18855162320@163.com"));
56
57 //邮件标题
58 message.setSubject("这是简单邮件案例的邮件标题!");
59
60 //邮件内容
61 message.setContent("<h1 style='color: red'>这是简单邮件案例的邮件内容</h1>","text/html;charset=UTF-8");
62
63 //5.发送邮件
64 ts.sendMessage(message,message.getAllRecipients());
65
66 //6.关闭连接
67 ts.close();
68
69 }
70 }
3.案例二:发送复杂邮件(带附件和图片)
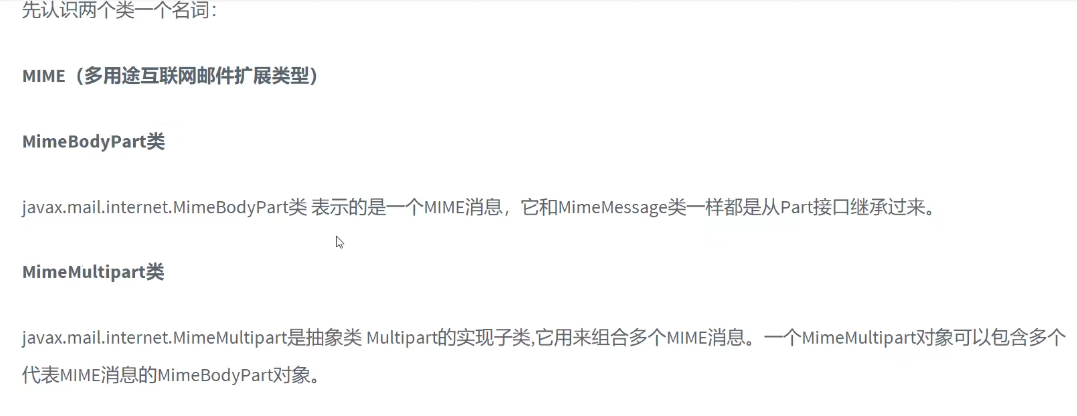
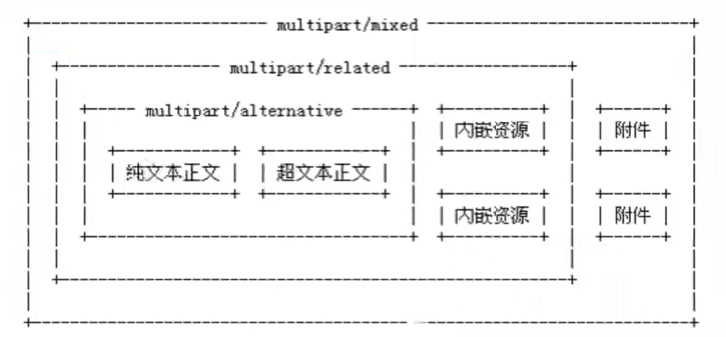
1 import com.sun.mail.util.MailSSLSocketFactory;
2
3 import javax.activation.DataHandler;
4 import javax.activation.FileDataSource;
5 import javax.mail.*;
6 import javax.mail.internet.InternetAddress;
7 import javax.mail.internet.MimeBodyPart;
8 import javax.mail.internet.MimeMessage;
9 import javax.mail.internet.MimeMultipart;
10 import java.io.UnsupportedEncodingException;
11 import java.security.GeneralSecurityException;
12 import java.util.Properties;
13
14 //发送一份复杂的邮件
15 public class MailDemo02 {
16
17 public static void main(String[] args) throws GeneralSecurityException, MessagingException, UnsupportedEncodingException {
18
19 Properties prop = new Properties();
20 prop.setProperty("mail.host","smtp.qq.com"); //设置QQ邮箱服务器
21 prop.setProperty("mail.transport.protocol","smtp"); //邮件发送协议
22 prop.setProperty("mail.smtp.auth","true"); //需要验证用户名和密码
23
24 //关于QQ邮箱,还要设置SSL加密,加上以下代码即可,只有QQ邮箱需要其他邮箱不需要
25 MailSSLSocketFactory sf = new MailSSLSocketFactory();
26 sf.setTrustAllHosts(true);
27 prop.put("mail.smtp.ssl.enable","true");
28 prop.put("mail.smt;.ssl.socketFactory",sf);
29
30
31 //使用JavaMail发送邮件5个步骤
32 //1.创建定义整个应用程序所需的环境信息的Session对象
33
34 //QQ 才需要,其他邮箱不需要
35 Session session = Session.getDefaultInstance(prop, new Authenticator() {
36 @Override
37 protected PasswordAuthentication getPasswordAuthentication() {
38 //发件人邮件用户名和授权码
39 return new PasswordAuthentication("464407764@qq.com","hmiauriqbvzmbhcf");
40 }
41 });
42
43 //开启Session的debug模式,这样就可以查看到程序发送Email的运行状态
44 session.setDebug(true);
45
46 //2.通过Session得到transport对象
47 Transport ts = session.getTransport();
48
49 //3.使用邮箱的用户名和授权码连上邮件服务器
50 ts.connect("smtp.qq.com","464407764@qq.com","hmiauriqbvzmbhcf");
51
52 //4.创建邮件:写邮件
53 MimeMessage message = new MimeMessage(session);
54
55 //指明邮件的发送者
56 message.setFrom(new InternetAddress("464407764@qq.com","海天之恋", "UTF-8"));
57
58 //指明邮件的接收者
59 message.setRecipient(Message.RecipientType.TO,new InternetAddress("18855162320@163.com"));
60
61 //邮件标题
62 message.setSubject("这是复杂邮件案例的邮件标题!");
63
64 //邮件内容
65 //================================================================================
66
67 //发送图片
68 // sendImage(message);
69 //发送附件,图片和文本
70 sendAttachmentsAndImage(message);
71
72 //================================================================================
73
74 //5.发送邮件
75 ts.sendMessage(message,message.getAllRecipients());
76
77 //6.关闭连接
78 ts.close();
79
80 }
81
82 public static void sendImage(MimeMessage message) throws MessagingException {
83 //准备图片数据
84 MimeBodyPart image = new MimeBodyPart();
85 DataHandler dh = new DataHandler(new FileDataSource(MailDemo02.class.getResource("/").getPath()+"/1.jpg"));//图片需要经过数据处理
86 image.setDataHandler(dh);//在Body中放入处理过后的图片
87 image.setContentID("myPicture");//给图片设置一个ID(随意设置)
88
89 //准备正文数据
90 MimeBodyPart text = new MimeBodyPart();
91 text.setContent("这是一封邮件正文带图片<img src='cid:myPicture'>的邮件","text/html;charset=UTF-8");//通过cid获得ContentID
92
93 //描述数据关系
94 MimeMultipart mm = new MimeMultipart();
95 mm.addBodyPart(text);
96 mm.addBodyPart(image);
97 mm.setSubType("related");
98
99 //设置到消息中,保存修改
100 message.setContent(mm);//把最后编辑好的邮件放入消息中
101 message.saveChanges();
102 }
103
104 public static void sendAttachmentsAndImage(MimeMessage message) throws MessagingException {
105 //图片
106 MimeBodyPart body1 = new MimeBodyPart();
107 body1.setDataHandler(new DataHandler(new FileDataSource(MailDemo02.class.getResource("/").getPath()+"/1.jpg")));//在Body中放入处理过后的图片
108 body1.setContentID("myPicture");//给图片设置一个ID(随意设置)
109
110 //文本
111 MimeBodyPart body2 = new MimeBodyPart();
112 body2.setContent("这是一封邮件正文带图片<img src='cid:myPicture'>的邮件","text/html;charset=UTF-8");//通过cid获得ContentID
113
114 //附件
115 MimeBodyPart body3 = new MimeBodyPart();
116 body3.setDataHandler(new DataHandler(new FileDataSource(MailDemo02.class.getResource("/").getPath() + "/1.txt")));
117 body3.setFileName("myAttachments.txt");//设置附件名
118
119 //1.现将文本和图片拼装
120 MimeMultipart multipart1 = new MimeMultipart();
121 multipart1.addBodyPart(body2); //文本
122 multipart1.addBodyPart(body1); //图片
123 multipart1.setSubType("related");
124
125 MimeBodyPart contentText = new MimeBodyPart();
126 contentText.setContent(multipart1);
127
128 //2.拼接附件
129 MimeMultipart allFile = new MimeMultipart();
130 allFile.addBodyPart(body3); //附件
131 allFile.addBodyPart(contentText); //文本+图片
132 allFile.setSubType("mixed");
133
134 //设置到消息中,保存修改
135 message.setContent(allFile);//把最后编辑好的邮件放入消息中
136 message.saveChanges();
137 }
138
139 }
4.总结
-
在应用场景中我们一般使用多线程处理发送邮件,将主要代码放在run方法中,防止发送时卡顿。
-
理解思路其次是程序