Wall
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 25412 | Accepted: 8456 |
Description
Once upon a time there was a greedy King who ordered his chief Architect to build a wall around the King's castle. The King was so greedy, that he would not listen to his Architect's proposals to build a beautiful brick wall with a perfect shape and nice tall towers. Instead, he ordered to build the wall around the whole castle using the least amount of stone and labor, but demanded that the wall should not come closer to the castle than a certain distance. If the King finds that the Architect has used more resources to build the wall than it was absolutely necessary to satisfy those requirements, then the Architect will loose his head. Moreover, he demanded Architect to introduce at once a plan of the wall listing the exact amount of resources that are needed to build the wall.
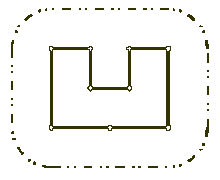
Your task is to help poor Architect to save his head, by writing a program that will find the minimum possible length of the wall that he could build around the castle to satisfy King's requirements.
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
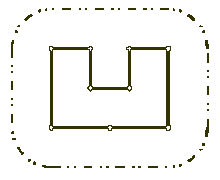
Your task is to help poor Architect to save his head, by writing a program that will find the minimum possible length of the wall that he could build around the castle to satisfy King's requirements.
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
Input
The first line of the input file contains two integer numbers N and L separated by a space. N (3 <= N <= 1000) is the number of vertices in the King's castle, and L (1 <= L <= 1000) is the minimal number of feet that King allows for the wall to come close to the castle.
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Output
Write to the output file the single number that represents the minimal possible length of the wall in feet that could be built around the castle to satisfy King's requirements. You must present the integer number of feet to the King, because the floating numbers are not invented yet. However, you must round the result in such a way, that it is accurate to 8 inches (1 foot is equal to 12 inches), since the King will not tolerate larger error in the estimates.
Sample Input
9 100 200 400 300 400 300 300 400 300 400 400 500 400 500 200 350 200 200 200
Sample Output
1628
题目意思: 本题目给你N,L,N代表有这个城堡有N个点组成,L是你现在建的墙离城堡的最小距离。 题解 :求出凸包 凸包的周长 加上 以L 为半径的圆周长
#include<stdio.h> #include<string> #include<cmath> #include<algorithm> using namespace std; #define MAX 1001 struct Node { double x; double y; }point[MAX]; int n,top; int stack[MAX];//用来存遍历判断时的点下标 bool cmp(Node a,Node b) { if(a.y==b.y) return a.x<b.x; else return a.y<b.y; } int xchen(Node& p1,Node& p2,Node& p3)//判定旋转方向,当p2-p1在p2-p3的下面时,是顺时针,返回大于0 { return (p1.x-p2.x)*(p3.y-p2.y)-(p1.y-p2.y)*(p3.x-p2.x); } double dis(Node& a,Node& b) { return sqrt((a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y)); } Node p11; bool cmpPolor(Node& p1,Node& p2) { double t=xchen(p1,p11,p2); if(t==0) return dis(p1,p11)<=dis(p2,p11); else if(t>0) return 1; else return 0; } double Con() { int i; sort(point,point+n,cmp);//第一次排序,找出原点 p11=point[0]; sort(point+1,point+n,cmpPolor);//第二次排序,按照副角大小排序 top=2; stack[0]=0; stack[1]=1; for(i=2;i<n;++i)//一共两次遍历判断 { while(top>1&&xchen(point[i],point[stack[top-1]],point[stack[top-2]])>=0)//等于0,去除同一边的点 top--; stack[top++]=i; } int t=top; stack[top++]=n-2; for(i=n-3;i>=0;i--) { while(top>t&&xchen(point[i],point[stack[top-1]],point[stack[top-2]])>=0) top--; stack[top++]=i; } double sum=0; for(i=0;i<top-1;i++) { sum+=dis(point[stack[i]],point[stack[i+1]]); // printf("%lf %lf\n",point[stack[i]].x,point[stack[i]].y); } sum+=dis(point[stack[top-1]],point[stack[0]]);//把这些凸包点,两两相连,最后要首尾相连 return sum; } int main() { int i,L; while(scanf("%d%d",&n,&L)!=EOF) { for(i=0;i<n;++i) scanf("%lf%lf",&point[i].x,&point[i].y); double disn; disn=2*L*3.1415926535897; disn+=Con(); printf("%.0lf\n",disn); } return 0; }
刚开始研究凸包,看的时候觉得还比较简单,但是具体操作的时候,就有很多部理解的地方,比如,理论讲的是按副角大小排序,但是操作的时候就有一定的技巧,网上的代码又不统一,造成我这种菜鸟一头雾水。还有就是那个找原点的操作,书上说是找最坐,横坐标最小,但是写的时候就不用for循环去找,可以用排序,也可以在输入时就找点。还有就是那个distance函数,这是个坑爹的函数,用的时候一定不能写小写,因为他本来是一个c++库函数,要是用了,会编辑错误,谨记!!!载着就是大家要注意,理论讲的时候是招大于0的点,但是在操作的时候,讲点顺序颠倒,就成了大于0不进栈。对于判定旋转的操作网上还有别的方法,暂不列举。
凸包入门搞了一天,头都晕了,错误不断,也没有人指点,网上的也是只贴代码和基本思想,对于里面调用函数的讲解甚少,很难理解。终于搞定,但还是不够熟练,需要练习