You are given a string ss consisting of nn lowercase Latin letters. nn is even.
For each position ii (1≤i≤n1≤i≤n) in string ss you are required to change the letter on this position either to the previous letter in alphabetic order or to the next one (letters 'a' and 'z' have only one of these options). Letter in every position must be changed exactly once.
For example, letter 'p' should be changed either to 'o' or to 'q', letter 'a' should be changed to 'b' and letter 'z' should be changed to 'y'.
That way string "codeforces", for example, can be changed to "dpedepqbft" ('c' →→ 'd', 'o' →→ 'p', 'd' →→ 'e', 'e' →→ 'd', 'f' →→ 'e', 'o' →→'p', 'r' →→ 'q', 'c' →→ 'b', 'e' →→ 'f', 's' →→ 't').
String ss is called a palindrome if it reads the same from left to right and from right to left. For example, strings "abba" and "zz" are palindromes and strings "abca" and "zy" are not.
Your goal is to check if it's possible to make string ss a palindrome by applying the aforementioned changes to every position. Print "YES" if string ss can be transformed to a palindrome and "NO" otherwise.
Each testcase contains several strings, for each of them you are required to solve the problem separately.
The first line contains a single integer TT (1≤T≤501≤T≤50) — the number of strings in a testcase.
Then 2T2T lines follow — lines (2i−1)(2i−1) and 2i2i of them describe the ii-th string. The first line of the pair contains a single integer nn (2≤n≤1002≤n≤100, nn is even) — the length of the corresponding string. The second line of the pair contains a string ss, consisting of nnlowercase Latin letters.
Print TT lines. The ii-th line should contain the answer to the ii-th string of the input. Print "YES" if it's possible to make the ii-th string a palindrome by applying the aforementioned changes to every position. Print "NO" otherwise.
5
6
abccba
2
cf
4
adfa
8
abaazaba
2
ml
YES
NO
YES
NO
NO
The first string of the example can be changed to "bcbbcb", two leftmost letters and two rightmost letters got changed to the next letters, two middle letters got changed to the previous letters.
The second string can be changed to "be", "bg", "de", "dg", but none of these resulting strings are palindromes.
The third string can be changed to "beeb" which is a palindrome.
The fifth string can be changed to "lk", "lm", "nk", "nm", but none of these resulting strings are palindromes. Also note that no letter can remain the same, so you can't obtain strings "ll" or "mm".
反正每个字母都要改变,那么相对的字母要不相差为二,要不为零.
1 #include <bits/stdc++.h> 2 using namespace std; 3 int main(){ 4 int n; 5 cin>>n; 6 while(n--){ 7 int x; 8 char s[101]; 9 cin>>x>>s; 10 int l = 0,r = x-1; 11 bool prime = true; 12 for(int i=1;i<=x/2;i++){ 13 int xn = s[l]-'a'; 14 int y = s[r]-'a'; 15 if(abs(xn-y)!=2&&abs(xn-y)!=0){ 16 prime = false; 17 break; 18 }else{ 19 l++,r--; 20 } 21 } 22 if(prime){ 23 cout<<"YES"<<endl; 24 }else{ 25 cout<<"NO"<<endl; 26 } 27 } 28 return 0; 29 }
You are given a chessboard of size n×nn×n. It is filled with numbers from 11 to n2n2 in the following way: the first ⌈n22⌉⌈n22⌉ numbers from 11 to ⌈n22⌉⌈n22⌉are written in the cells with even sum of coordinates from left to right from top to bottom. The rest n2−⌈n22⌉n2−⌈n22⌉ numbers from ⌈n22⌉+1⌈n22⌉+1 to n2n2are written in the cells with odd sum of coordinates from left to right from top to bottom. The operation ⌈xy⌉⌈xy⌉ means division xx by yy rounded up.
For example, the left board on the following picture is the chessboard which is given for n=4n=4 and the right board is the chessboard which is given for n=5n=5.
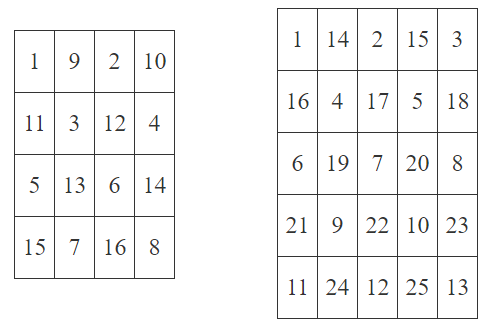
You are given qq queries. The ii-th query is described as a pair xi,yixi,yi. The answer to the ii-th query is the number written in the cell xi,yixi,yi (xixiis the row, yiyi is the column). Rows and columns are numbered from 11 to nn.
The first line contains two integers nn and qq (1≤n≤1091≤n≤109, 1≤q≤1051≤q≤105) — the size of the board and the number of queries.
The next qq lines contain two integers each. The ii-th line contains two integers xi,yixi,yi (1≤xi,yi≤n1≤xi,yi≤n) — description of the ii-th query.
For each query from 11 to qq print the answer to this query. The answer to the ii-th query is the number written in the cell xi,yixi,yi (xixi is the row, yiyi is the column). Rows and columns are numbered from 11 to nn. Queries are numbered from 11 to qq in order of the input.
4 5
1 1
4 4
4 3
3 2
2 4
1
8
16
13
4
5 4
2 1
4 2
3 3
3 4
16
9
7
20
Answers to the queries from examples are on the board in the picture from the problem statement.
看好规律就可以,但是要用longlong,为此哇了一发.
1 #include <bits/stdc++.h> 2 #define ll long long 3 using namespace std; 4 ll n,m; 5 int main(){ 6 cin>>n>>m; 7 while(m--){ 8 ll x,y; 9 cin>>x>>y; 10 ll ans = (x-1)*n+y; 11 ll begin = (n*n+1)/2; 12 if(n%2){ 13 if(x%2){ 14 if(ans%2){ 15 ll xn = (x-1)/2*n; 16 y = (y+1)/2; 17 cout<<xn+y<<endl; 18 }else{ 19 ll xn = (x-1)/2*n; 20 y = y/2; 21 cout<<begin+xn+y<<endl; 22 } 23 }else{ 24 if(ans%2==0){ 25 ll xn = (x)/2*n-(n+1)/2; 26 y = (y+1)/2; 27 cout<<begin+xn+y<<endl; 28 }else{ 29 ll xn = (x)/2*n-n/2; 30 y = y/2; 31 cout<<xn+y<<endl; 32 } 33 } 34 }else{ 35 if(x%2){ 36 if(ans%2){ 37 ll xn = (x-1)/2*n; 38 y = (y+1)/2; 39 cout<<xn+y<<endl; 40 }else{ 41 ll xn = (x-1)/2*n; 42 y = y/2; 43 cout<<begin+xn+y<<endl; 44 } 45 }else{ 46 if(ans%2){ 47 ll xn = (x)/2*n-n/2; 48 y = (y+1)/2; 49 cout<<begin+xn+y<<endl; 50 }else{ 51 ll xn = (x)/2*n-n/2; 52 y = y/2; 53 cout<<xn+y<<endl; 54 } 55 } 56 } 57 } 58 return 0; 59 }
You have nn sticks of the given lengths.
Your task is to choose exactly four of them in such a way that they can form a rectangle. No sticks can be cut to pieces, each side of the rectangle must be formed by a single stick. No stick can be chosen multiple times. It is guaranteed that it is always possible to choose such sticks.
Let SS be the area of the rectangle and PP be the perimeter of the rectangle.
The chosen rectangle should have the value P2SP2S minimal possible. The value is taken without any rounding.
If there are multiple answers, print any of them.
Each testcase contains several lists of sticks, for each of them you are required to solve the problem separately.
The first line contains a single integer TT (T≥1T≥1) — the number of lists of sticks in the testcase.
Then 2T2T lines follow — lines (2i−1)(2i−1) and 2i2i of them describe the ii-th list. The first line of the pair contains a single integer nn (4≤n≤1064≤n≤106) — the number of sticks in the ii-th list. The second line of the pair contains nn integers a1,a2,…,ana1,a2,…,an (1≤aj≤1041≤aj≤104) — lengths of the sticks in the ii-th list.
It is guaranteed that for each list there exists a way to choose four sticks so that they form a rectangle.
The total number of sticks in all TT lists doesn't exceed 106106 in each testcase.
Print TT lines. The ii-th line should contain the answer to the ii-th list of the input. That is the lengths of the four sticks you choose from the ii-th list, so that they form a rectangle and the value P2SP2S of this rectangle is minimal possible. You can print these four lengths in arbitrary order.
If there are multiple answers, print any of them.
3
4
7 2 2 7
8
2 8 1 4 8 2 1 5
5
5 5 5 5 5
2 7 7 2
2 2 1 1
5 5 5 5
There is only one way to choose four sticks in the first list, they form a rectangle with sides 22 and 77, its area is 2⋅7=142⋅7=14, perimeter is 2(2+7)=182(2+7)=18. 18214≈23.14318214≈23.143.
The second list contains subsets of four sticks that can form rectangles with sides (1,2)(1,2), (2,8)(2,8) and (1,8)(1,8). Their values are 622=18622=18, 20216=2520216=25 and 1828=40.51828=40.5, respectively. The minimal one of them is the rectangle (1,2)(1,2).
You can choose any four of the 55 given sticks from the third list, they will form a square with side 55, which is still a rectangle with sides (5,5)(5,5).
这题难点,要是P^2/S化简
最后可以化成x/y+y/x最小,求这个最小,那就是x和y越接近越好.
那么x/y越接近1,越好,所以如果一个为想x/y 一个为xx/yy,如果后者大于前者,那麽就取后者,当然
前提是已经排好序.
1 #include <bits/stdc++.h> 2 #define ll long long 3 #define N 1000006 4 using namespace std; 5 6 ll a[N], cnt = 0, b[N]; 7 int main() { 8 int t, n; 9 cin >> t; 10 while(t--) { 11 cin>>n; 12 cnt = 0; 13 for(int i = 1; i <= n; i ++) scanf("%lld", &a[i]); 14 sort(a+1,a+1+n); 15 for(int i = 1; i < n; i ++) { 16 if(a[i] == a[i+1]) { 17 b[cnt++] = a[i]; 18 i++; 19 } 20 } 21 ll ans1 = b[0], ans2 = b[1]; 22 23 for(int i = 2; i < cnt; i ++) { 24 if(ans1*b[i] < ans2*b[i-1]) { 25 ans1 = b[i-1]; 26 ans2 = b[i]; 27 } 28 } 29 cout<<ans1<<" "<<ans1<<" "<<ans2<<" "<<ans2<<endl; 30 } 31 return 0; 32 }
Medicine faculty of Berland State University has just finished their admission campaign. As usual, about 80%80% of applicants are girls and majority of them are going to live in the university dormitory for the next 44 (hopefully) years.
The dormitory consists of nn rooms and a single mouse! Girls decided to set mouse traps in some rooms to get rid of the horrible monster. Setting a trap in room number ii costs cici burles. Rooms are numbered from 11 to nn.
Mouse doesn't sit in place all the time, it constantly runs. If it is in room ii in second tt then it will run to room aiai in second t+1t+1 without visiting any other rooms inbetween (i=aii=ai means that mouse won't leave room ii). It's second 00 in the start. If the mouse is in some room with a mouse trap in it, then the mouse get caught into this trap.
That would have been so easy if the girls actually knew where the mouse at. Unfortunately, that's not the case, mouse can be in any room from 11 to nn at second 00.
What it the minimal total amount of burles girls can spend to set the traps in order to guarantee that the mouse will eventually be caught no matter the room it started from?
The first line contains as single integers nn (1≤n≤2⋅1051≤n≤2⋅105) — the number of rooms in the dormitory.
The second line contains nn integers c1,c2,…,cnc1,c2,…,cn (1≤ci≤1041≤ci≤104) — cici is the cost of setting the trap in room number ii.
The third line contains nn integers a1,a2,…,ana1,a2,…,an (1≤ai≤n1≤ai≤n) — aiai is the room the mouse will run to the next second after being in room ii.
Print a single integer — the minimal total amount of burles girls can spend to set the traps in order to guarantee that the mouse will eventually be caught no matter the room it started from.
5
1 2 3 2 10
1 3 4 3 3
3
4
1 10 2 10
2 4 2 2
10
7
1 1 1 1 1 1 1
2 2 2 3 6 7 6
2
In the first example it is enough to set mouse trap in rooms 11 and 44. If mouse starts in room 11 then it gets caught immideately. If mouse starts in any other room then it eventually comes to room 44.
In the second example it is enough to set mouse trap in room 22. If mouse starts in room 22 then it gets caught immideately. If mouse starts in any other room then it runs to room 22 in second 11.
Here are the paths of the mouse from different starts from the third example:
- 1→2→2→…1→2→2→…;
- 2→2→…2→2→…;
- 3→2→2→…3→2→2→…;
- 4→3→2→2→…4→3→2→2→…;
- 5→6→7→6→…5→6→7→6→…;
- 6→7→6→…6→7→6→…;
- 7→6→7→…7→6→7→…;
So it's enough to set traps in rooms 22 and 66.
这题感觉找到环就行了.
1 #include <bits/stdc++.h> 2 #define N 200005 3 #define ll long long int 4 using namespace std; 5 ll a[N],b[N]; 6 bool vis[N],val[N]; 7 std::vector<ll> v[N]; 8 ll cnt = 0; 9 ll n; 10 11 void dfs(ll x){ 12 vis[x] = 1; 13 for(ll i=0;i<v[x].size();i++){ 14 ll y = v[x][i]; 15 if(vis[y]==0){ 16 dfs(y); 17 } 18 } 19 } 20 21 void solve(ll x){ 22 val[x] = 1; 23 ll y = b[x]; 24 if(val[y]){ 25 ll ans = a[x]; 26 ll xn = y; 27 while(xn!=x){ 28 ans = min(ans,a[xn]); 29 xn = b[xn]; 30 } 31 cnt += ans; 32 }else{ 33 solve(y); 34 } 35 } 36 37 int main(){ 38 cin>>n; 39 for(ll i=1;i<=n;i++){ 40 cin>>a[i]; 41 } 42 for(ll i=1;i<=n;i++){ 43 cin>>b[i]; 44 v[i].push_back(b[i]); 45 v[b[i]].push_back(i); 46 } 47 for(ll i = 1;i<=n;i++){ 48 if(vis[i]==0){ 49 dfs(i); 50 solve(i); 51 } 52 } 53 cout<<cnt<<endl; 54 return 0; 55 }