Description
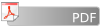
A friend of you is doing research on the Traveling Knight Problem (TKP) where you are to find the shortest closed tour of knight moves that visits each square of a given set of n squares on a chessboard exactly once. He thinks that the most difficult part of the problem is determining the smallest number of knight moves between two given squares and that, once you have accomplished this, finding the tour would be easy.
Of course you know that it is vice versa. So you offer him to write a program that solves the "difficult" part.
Your job is to write a program that takes two squares a and b as input and then determines the number of knight moves on a shortest route from a to b.
Input Specification
The input file will contain one or more test cases. Each test case consists of one line containing two squares separated by one space. A square is a string consisting of a letter (a-h) representing the column and a digit (1-8) representing the row on the chessboard.
Output Specification
For each test case, print one line saying "To get from xx to yy takes n knight moves.".
Sample Input
e2 e4 a1 b2 b2 c3 a1 h8 a1 h7 h8 a1 b1 c3 f6 f6
Sample Output
To get from e2 to e4 takes 2 knight moves. To get from a1 to b2 takes 4 knight moves. To get from b2 to c3 takes 2 knight moves. To get from a1 to h8 takes 6 knight moves. To get from a1 to h7 takes 5 knight moves. To get from h8 to a1 takes 6 knight moves. To get from b1 to c3 takes 1 knight moves. To get from f6 to f6 takes 0 knight moves.
思路:要解这道题,首先必须明白国际象棋中骑士的走法,如图所示

然后可以使用广度优先搜索,广搜在寻求最短路径比深搜好很多,他只需入队列一次,而且只要找到了,即为最短路径,时间性能也优越。
#include"iostream"
#include"cstring"
#include"cstdio"
using namespace std;
struct node
{
int x;
int y;
int s;
};
int nex[8][2]={{-2,1},{-2,-1},{2,-1},{2,1},{1,-2},{-1,-2},{-1,2},{1,2}};
int main()
{
char s1[5],s2[5];
while(scanf("%s%s",s1,s2)!=EOF)
{
struct node que[100];
int a[9][9],book[9][9];
memset(a,0,sizeof(a));
memset(book,0,sizeof(book));
for(int kk=0;kk<100;kk++)
{
que[kk].x=0;
que[kk].y=0;
que[kk].s=0;
}
int head,tail;
int i,j,k,n,m,startx,starty,p,q,tx,ty,flag;
startx=s1[0]-'a'+1;
starty=s1[1]-'0';
p=s2[0]-'a'+1;
q=s2[1]-'0';
//cout<<s1<<endl<<s2<<endl;
//cout<<startx<<' '<<starty<<endl;
//if(startx==p&&starty==q) cout<<"To get from "<<s1[0]<<s1[1]<<" to "<<s2[0]<<s2[1]<<" takes "<<0<<" knight moves"<<endl;
{
head=1;
tail=1;
que[tail].x=startx;
que[tail].y=starty;
que[tail].s=0;
tail++;
book[startx][starty]=1;
flag=0;
while(head<tail)
{
if