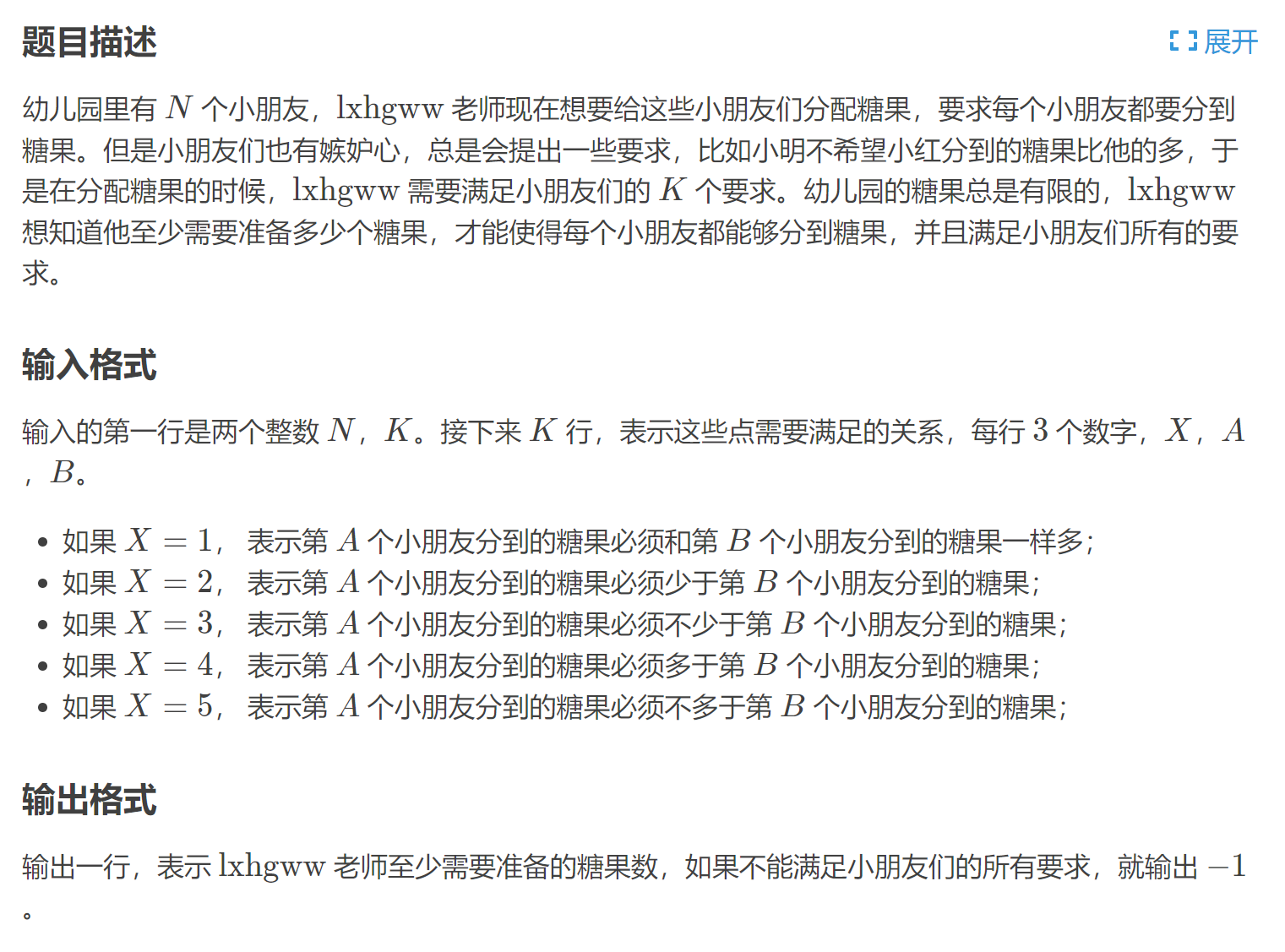
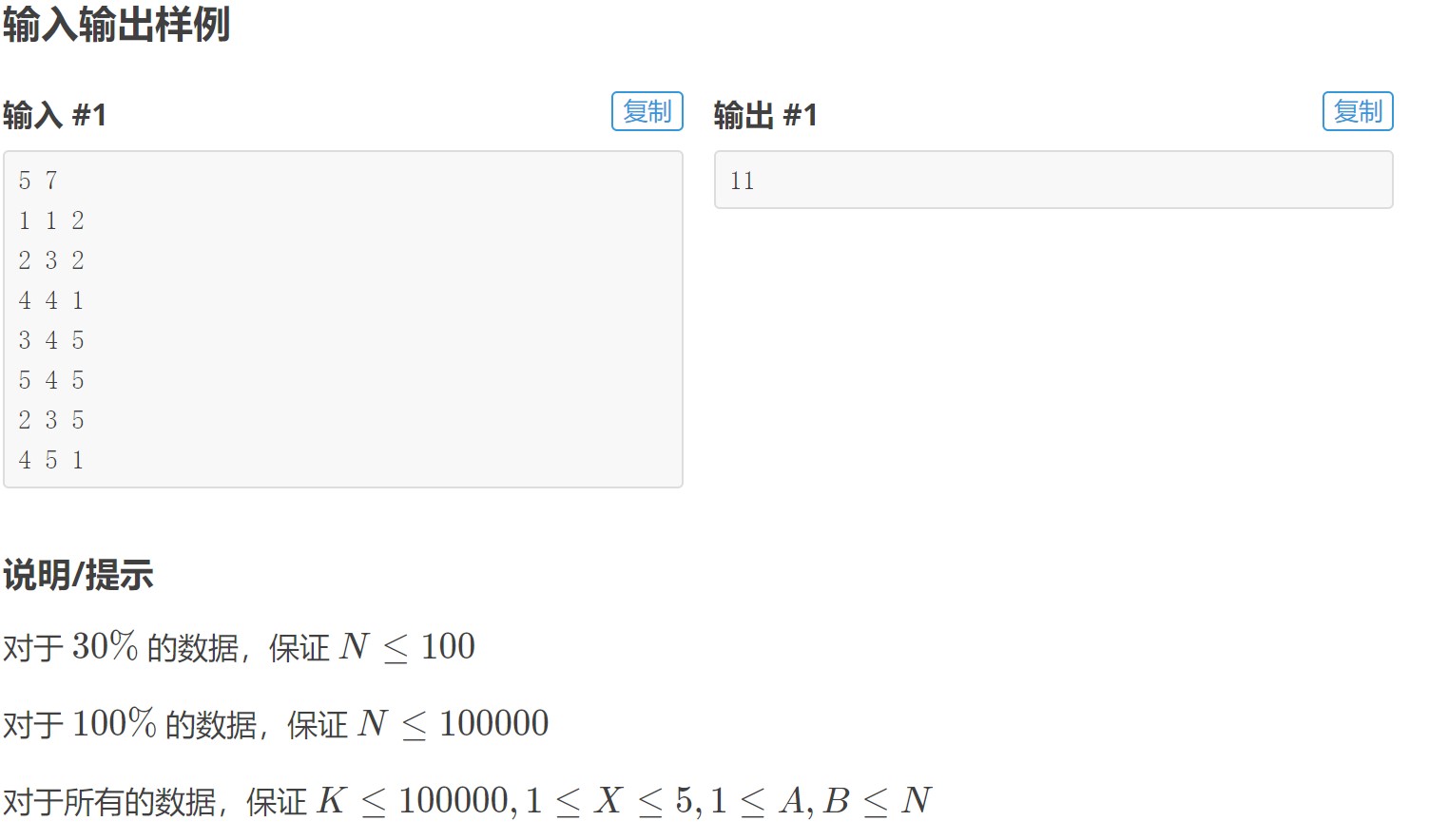
题目思路
差分约束。由于求的是最小值,所以我们需要求最长路径。题目中有五种情况
- X = 1,A和B的糖果一样多表示关系为(A>=B,B>=A)
- X = 2,A的糖果必须比B少,表示为(B>=A+1)
- X = 3,A的糖果不少于B,表示为(A>=B)
- X = 4,A的糖果必须多余B,表示为(A >= B +1)
- X = 5,A的糖果不多余B,表示为A<=B
由于每个小朋友都要分到糖果,所以对于每个X都要大于等于1,所以我们建立一个超级源点(X_0),每个点的取值为(X >= X_0 + 1)
注意
题目的数据范围最大的时候,需要用long long,无解的情况就是存在负环,数据量太大spfa会超时,可以把队列换成栈
实现代码
#include <iostream>
#include <cstring>
#include <cstdio>
using namespace std;
const int N = 100010, M = 300010;
typedef long long LL;
int h[N], e[M], ne[M], w[M], idx;
int n, m;
LL dist[N];
bool st[N];
int q[N];
int cnt[N];
void add(int a,int b,int c)
{
e[idx] = b, ne[idx] = h[a], w[idx] = c, h[a] = idx ++;
}
bool spfa()
{
memset(dist,-0x3f,sizeof dist);
dist[0] = 0;
st[0] = true;
int hh = 0, tt = 1;
q[0] = 0;
while(hh != tt)
{
int t = q[-- tt];
st[t] = false;
for(int i = h[t];~i; i = ne[i])
{
int j = e[i];
if(dist[j] < dist[t] + w[i])
{
dist[j] = dist[t] + w[i];
cnt[j] = cnt[t] + 1;
if(cnt[j] >= n + 1) return false;
if(!st[j])
{
q[ tt ++] = j;
st[j] = true;
}
}
}
}
return true;
}
int main()
{
scanf("%d%d",&n, &m);
memset(h,-1,sizeof h);
for(int i = 0;i < m;i ++)
{
int x, a, b;
scanf("%d%d%d",&x,&a,&b);
if(x == 1) add(a,b,0),add(b,a,0);
else if(x == 2) add(a,b,1);
else if(x == 3) add(b,a,0);
else if(x == 4) add(b,a,1);
else add(a,b,0);
}
for(int i = 1;i <= n;i++) add(0,i,1);
long long res = 0;
if(!spfa()) puts("-1");
else
{
for(int i = 1;i <= n;i++) res += dist[i];
printf("%lld", res);
}
return 0;
}