A Knight's Journey
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 41972 | Accepted: 14286 |
Description
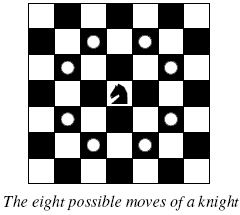
The knight is getting bored of seeing the same black and white squares again and again and has decided to make a journey
around the world. Whenever a knight moves, it is two squares in one direction and one square perpendicular to this. The world of a knight is the chessboard he is living on. Our knight lives on a chessboard that has a smaller area than a regular 8 * 8 board, but it is still rectangular. Can you help this adventurous knight to make travel plans?
Problem
Find a path such that the knight visits every square once. The knight can start and end on any square of the board.
Input
The
input begins with a positive integer n in the first line. The following
lines contain n test cases. Each test case consists of a single line
with two positive integers p and q, such that 1 <= p * q <= 26.
This represents a p * q chessboard, where p describes how many different
square numbers 1, . . . , p exist, q describes how many different
square letters exist. These are the first q letters of the Latin
alphabet: A, . . .
Output
The
output for every scenario begins with a line containing "Scenario #i:",
where i is the number of the scenario starting at 1. Then print a
single line containing the lexicographically first path that visits all
squares of the chessboard with knight moves followed by an empty line.
The path should be given on a single line by concatenating the names of
the visited squares. Each square name consists of a capital letter
followed by a number.
If no such path exist, you should output impossible on a single line.
If no such path exist, you should output impossible on a single line.
Sample Input
3 1 1 2 3 4 3
Sample Output
Scenario #1: A1 Scenario #2: impossible Scenario #3: A1B3C1A2B4C2A3B1C3A4B2C4
Source
TUD Programming Contest 2005, Darmstadt, Germany
题意:
象棋中骑士走日,给出一个p*q棋盘,问其实能否一次走遍所有的格子,按照字典序输出路径。
代码:
1 //基础dfs,用vector保存路径。 2 #include<iostream> 3 #include<vector> 4 #include<cstdio> 5 #include<cstring> 6 using namespace std; 7 int p,q,t; 8 const int diry[8]={-1,1,-2,2,-2,2,-1,1}; 9 const int dirx[8]={-2,-2,-1,-1,1,1,2,2}; 10 int sum; 11 bool vis[30][30]; 12 vector<int>loadx; 13 vector<int>loady; 14 void dfs(int x,int y) 15 { 16 vis[x][y]=1; 17 sum++; 18 loadx.push_back(x); 19 loady.push_back(y); 20 if(sum==p*q) 21 return; 22 for(int i=0;i<8;i++) 23 { 24 25 if(x+dirx[i]<=0||x+dirx[i]>q||y+diry[i]<=0||y+diry[i]>p) 26 continue; 27 if(vis[x+dirx[i]][y+diry[i]]) 28 continue; 29 dfs(x+dirx[i],y+diry[i]); 30 if(sum==p*q) 31 return; 32 } 33 vis[x][y]=0; 34 sum--; 35 loadx.pop_back(); 36 loady.pop_back(); 37 } 38 int main() 39 { 40 scanf("%d",&t); 41 for(int k=1;k<=t;k++) 42 { 43 scanf("%d%d",&p,&q); 44 sum=0; 45 memset(vis,0,sizeof(vis)); 46 while(!loadx.empty()) 47 { 48 loadx.pop_back(); 49 loady.pop_back(); 50 } 51 for(int i=1;i<=q;i++) 52 { 53 if(sum==p*q) 54 break; 55 for(int j=1;j<=p;j++) 56 { 57 dfs(i,j); 58 if(sum==p*q) 59 break; 60 } 61 } 62 printf("Scenario #%d: ",k); 63 if(sum==p*q) 64 { 65 for(int i=0;i<loadx.size();i++) 66 { 67 printf("%c%d",loadx[i]+64,loady[i]); 68 } 69 printf(" "); 70 } 71 else printf("impossible "); 72 } 73 return 0; 74 }