题目链接:http://oj.ecustacm.cn/problem.php?id=1284
题目描述
如下的10个格子,填入0~9的数字。要求:连续的两个数字不能相邻。
(左右、上下、对角都算相邻)一共有多少种可能的填数方案?
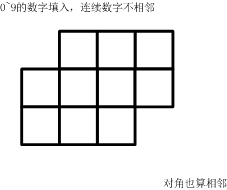
(左右、上下、对角都算相邻)一共有多少种可能的填数方案?
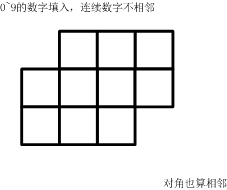
输出
请填写表示方案数目的整数。
思路:
采取 DFS 的方法去遍历每个方格 , 遇到每个方格我们都去判断一下它的 左右、上下、对角 是否符合题意,符合的话才放。
然后的话对于两个缺口我们采取一个是 入口 一个是 出口 的方式进行遍历就好了
#include <iostream> #include <algorithm> #include <string> #include <string.h> #include <vector> #include <map> #include <stack> #include <set> #include <queue> #include <math.h> #include <cstdio> #include <iomanip> #include <time.h> #define LL long long #define INF 0x3f3f3f3f #define ls nod<<1 #define rs (nod<<1)+1 const int maxn = 1e5 + 10 ; const LL mod = 20010905; const int r = 3,c = 4; int map[10][10],vis[20],cnt; int dir[8][2] = {0,-1,-1,-1,-1,0,-1,1,0,1,1,1,1,0,1,-1}; bool check(int x,int y,int n) { for (int i = 0;i < 8;i++) { int nx = x + dir[i][0]; int ny = y + dir[i][1]; if (nx >= 0 && nx < r && ny >= 0 && ny < c) { if (map[nx][ny] == n-1 || map[nx][ny] == n+1) return false; } } return true; } void dfs(int dep,int pos) { if (dep == 2 && pos == 3) { cnt++; return ; } if (pos >= c) dfs(dep+1,0); else { for (int i = 0;i <= 9;i++) { if (!vis[i] && check(dep,pos,i)) { vis[i] = 1; map[dep][pos] = i; dfs(dep,pos+1); map[dep][pos] = -10; vis[i] = 0; } } } } int main() { for (int i = 0;i < 10;i++) { for (int j = 0;j < 10;j++) map[i][j] = -10; } memset(vis,0, sizeof(vis)); cnt = 0; dfs(0,1); printf("%d ",cnt); return 0; }
当然 暴力杯 肯定有暴力的方法:
#include<bits/stdc++.h> using namespace std; int main() { int a[10] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9}; int ans = 0; do { if((abs(a[0]-a[1])!=1)&&(abs(a[1]-a[2])!=1)&&(abs(a[3]-a[4])!=1)&&(abs(a[4]-a[5])!=1)&& (abs(a[5]-a[6])!=1)&&(abs(a[7]-a[8])!=1)&&(abs(a[8]-a[9])!=1)&& (abs(a[0]-a[4])!=1)&&(abs(a[1]-a[5])!=1)&&(abs(a[2]-a[6])!=1)&& (abs(a[3]-a[7])!=1)&&(abs(a[4]-a[8])!=1)&&(abs(a[5]-a[9])!=1)&& (abs(a[0]-a[3])!=1)&&(abs(a[0]-a[5])!=1)&&(abs(a[1]-a[4])!=1)&& (abs(a[1]-a[6])!=1)&&(abs(a[2]-a[5])!=1)&&(abs(a[4]-a[7])!=1)&&(abs(a[4]-a[9])!=1)&& (abs(a[3]-a[8])!=1)&&(abs(a[5]-a[8])!=1)&&(abs(a[6]-a[9])!=1)) ans++; }while(next_permutation(a,a+10)); cout<<ans<<endl; }