POI使用流程
1、导入jar包
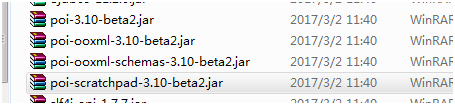
2、引入插件
<script type="text/javascript" src="${pageContext.request.contextPath}/jquery-2.1.1.min.js"></script>
3、在前台写一个button按钮(考虑到服务器不在本地,所以做成下载的功能)
<button onclick="toout()">导出</button>
//按钮有一个点击事件,触发后台的action
<script type="text/javascript">
function toout(){
window.location.href = "${pageContext.request.contextPath}/areaAction!areaList.jhtml";
}
//两表导出
function exportOut(){
/* window.location.href = "${pageContext.request.contextPath }/userAction!exportList.jhtml"; */
var data = $("#index_form").serialize();
console.info(data);
window.location.href= "${pageContext.request.contextPath }/userAction!exportTileList.jhtml?" + data;
}
</script>
4.进入后台(dao—-service—action)
dao和service主要查询功能(ssh注解版框架)
action类中:
导出——>
在util中引入工具类:ExportExcel.java、BaseAction.java
public void userList(){ //定义一个String类型的字符串去接收标题名称,sheet页名称 String title = "用户信息"; //定义String类型 数组,把表头信息拼接进去 String[] rowName = new String[]{"序号","姓名","密码","创建时间","修改时间"}; //由于excel是多种数据类型,所以我们定义一个object数组接收,这样减少代码冗余,提高重用率 List<Object[]> dataList = new ArrayList<Object[]>(); try { //将导出的数据放入List集合 (多表的集合) List<User> userList = userService.userList(user); //遍历list集合放入对象里 for (int i = 0; i < userList.size(); i++) { //定义对象数组[] Object[] obj = new Object[rowName.length]; //根据表头rowName的长度,给对象赋值 obj[0] = userList.get(i).getId(); obj[1] = userList.get(i).getName(); obj[2] = userList.get(i).getPwd(); obj[3] = userList.get(i).getCreatedatetime(); obj[4] = userList.get(i).getModifydatetime(); //将赋完值的obj对象放入刚才定义的dataList里 dataList.add(obj); } //已经得到title, rowName, dataList;放入到我写的工具类里 ,工具类有title, rowName, dataList //全局变量 ExportExcel exportExcel = new ExportExcel(title, rowName, dataList); //运行导出export方法 exportExcel.export(); } catch (Exception e) { e.printStackTrace(); } }
导入<——
在util中引入工具类:ExportExcel.java、BaseAction.java、fileUtil.java
1、在前台写一个按钮
<form id="input_form" action="${pageContext.request.contextPath }/userAction!inputUserFile.jhtml" method="post" enctype="multipart-form-data"> <input type="file" name="excleFile"> <input type="submit" value="导入文件"> </form>
2、在<script>中写方法
function detailUser(){ var data = $("#input_form").serialize(); console.info(data); window.location.href= "${pageContext.request.contextPath }/userAction!exportTileList.jhtml?" + data;
3、action 类
private File excleFile;(生成get set方法) private String excleFileFileName;(生成get set方法) public void inputUserFile(){ //获得绝对路径至文件夹 String realPath = ServletActionContext.getServletContext().getRealPath(""); //获得上传后的文件名 String upLoadFile = FileUtil.upLoadFile(excleFile, excleFileFileName, "aa");//aa是tomcat下的文件夹 //文件在服务器的绝对路径 String FilePath = realPath + "/" + upLoadFile; System.out.println(FilePath); List<User> list = new ArrayList<User>(); //判断是否是.xls文件 版本不同(.xls为2003版,.xlsx为2007版) if(upLoadFile.endsWith(".xls")){ try { //创建工作簿 HSSFWorkbook book = new HSSFWorkbook(new FileInputStream(new File(FilePath))); //获得当前sheet页 book.getSheetAt(0); //遍历sheet页 for (int i = 0; i < book.getNumberOfSheets(); i++) { //创建sheet页 HSSFSheet sheet = book.getSheetAt(i); //遍历当前第4行下表为3(前面3行为表头信息) for (int j = 3; j < sheet.getPhysicalNumberOfRows(); j++) { //创建行 HSSFRow row = sheet.getRow(j); //创建对象,把对象放入list User user1 = new User(); SimpleDateFormat sdf= new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); //通过id赋值 if(UserAction.getCellValue(row.getCell(0)) !=null &&!UserAction.getCellValue(row.getCell(0)).equals("")){ user1.setId(Long.valueOf(UserAction.getCellValue(row.getCell(0)))); } //姓名 user1.setName(UserAction.getCellValue(row.getCell(1))); user1.setPwd(UserAction.getCellValue(row.getCell(2))); //创建时间 if(UserAction.getCellValue(row.getCell(3))!=null&&!UserAction.getCellValue(row.getCell(3)).equals("")){ user1.setCreateDate(sdf.parse(UserAction.getCellValue(row.getCell(3)))); } //修改时间 if(UserAction.getCellValue(row.getCell(4))!=null&&!UserAction.getCellValue(row.getCell(4)).equals("")){ user1.setModifyDate(sdf.parse(UserAction.getCellValue(row.getCell(4)))); } //放入list集合 list.add(user1); } } //遍历集合 for (User user : list) { userService.addUser(user); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } catch (ParseException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } }else if(upLoadFile.endsWith(".xlsx")){ } } ———————————格式设置——————————— // 判断从Excel文件中解析出来数据的格式 private static String getCellValue(HSSFCell cell) { String value = null; // 简单的查检列类型 switch (cell.getCellType()) { case HSSFCell.CELL_TYPE_STRING:// 字符串 value = cell.getRichStringCellValue().getString(); break; case HSSFCell.CELL_TYPE_NUMERIC:// 数字 long dd = (long) cell.getNumericCellValue(); value = dd + ""; break; case HSSFCell.CELL_TYPE_BLANK: value = ""; break; case HSSFCell.CELL_TYPE_FORMULA: value = String.valueOf(cell.getCellFormula()); break; case HSSFCell.CELL_TYPE_BOOLEAN:// boolean型值 value = String.valueOf(cell.getBooleanCellValue()); break; case HSSFCell.CELL_TYPE_ERROR: value = String.valueOf(cell.getErrorCellValue()); break; default: break; } return value; }