Description
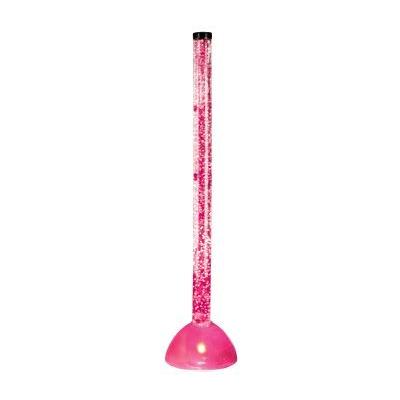
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
算法课学过归并排序求逆序数。
代码:
#include <iostream> #include <cstdio> #include <set> using namespace std; typedef long long ll; int num[500000]; ll merge_sort(int *t,int l,int r) { if(l >= r) return 0; int mid = (l + r) / 2; ll d = merge_sort(t,l,mid) + merge_sort(t,mid + 1,r); int *temp = new int[r - l + 1]; int c = 0,i = l,j = mid + 1; while(i <= mid || j <= r) { if(i <= mid && (t[i] <= t[j] || j > r)) { temp[c ++] = t[i ++]; } else if(j <= r && (t[j] < t[i] || i > mid)) { temp[c ++] = t[j ++]; d += mid - i + 1; } } for(int i = l;i <= r;i ++) { t[i] = temp[i - l]; } delete [] temp; return d; } int main() { int n,d; while(~scanf("%d",&n) && n) { for(int i = 0;i < n;i ++) { scanf("%d",&num[i]); } printf("%lld ",merge_sort(num,0,n - 1)); } }
耗时不少,其实树状数组好一点,只不过不能直接把数作为下标,数很大,但是个数最多500000,可以接受,可以排序后进行。
代码:
#include <iostream> #include <cstdio> #include <cstring> #include <algorithm> using namespace std; typedef long long ll; typedef pair<int,int> pa; pa num[500000]; ll sum[500001]; int n; inline int lowbit(int x) { return x & -x; } inline void update(int x,int y) { while(x <= n) { sum[x] += y; x += lowbit(x); } } inline int getsum(int x) { int ans = 0; while(x > 0) { ans += sum[x]; x -= lowbit(x); } return ans; } bool cmp(pa a,pa b) { if(a.first == b.first) return a.second < b.second; return a.first < b.first; } int main() { int d; while(~scanf("%d",&n) && n) { ll ans = 0; for(int i = 0;i < n;i ++) { sum[i + 1] = 0; scanf("%d",&d); num[i] = pa(d,i + 1); } sort(num,num + n,cmp); for(int i = 0;i < n;i ++) { ans += getsum(num[i].second); update(num[i].second,1); } printf("%lld ",(ll)n * (n - 1) / 2 - ans); } }