Chart 支持将其数据系列和类别轴绑定到数组和 Observable。
绑定系列
本节提供有关 Chart 系列的一般绑定方法的信息。
值数组
最简单的数据绑定形式涉及为每个系列提供一组值。
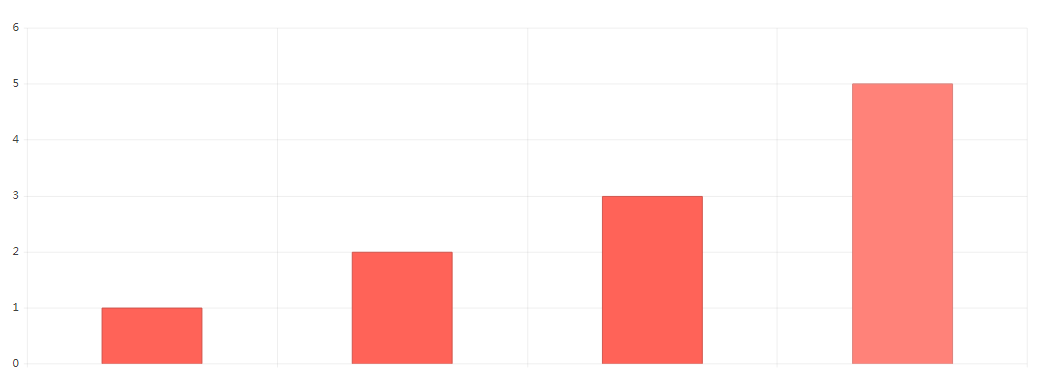
import { Component } from '@angular/core'; @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item [data]="seriesData"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: number[] = [1, 2, 3, 5]; }
数组
某些系列要求每个点有多个值,例如 Scatter(x 和 y)和 Bubble(x、y 和大小)系列。以下示例演示如何将 Bubble 系列绑定到数组。
@Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item type="bubble" [data]="seriesData"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: number[] = [[1, 1, 10], [2, 2, 20], [3, 3, 30]]; }
对象
图表允许您通过指定要使用的字段(值、类别、X 值、Y 值等)将其绑定到对象。 这是最常用的绑定类型,因为它允许您使用模型而只需很少或无需修改。以下示例演示如何使用绑定类别文本和值配置列系列。
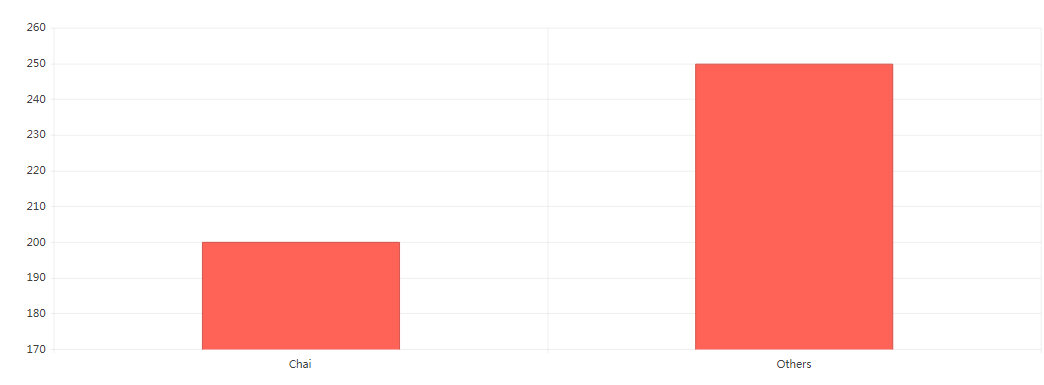
import { Component } from '@angular/core'; interface Model { product: string; sales: number; } @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item type="column" [data]="seriesData" field="sales" categoryField="product"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: Model[] = [{ product: 'Chai', sales: 200 }, { product: 'Others', sales: 250 }]; }
对象组
为数据集中的每个独特类别绘制单独的系列通常很方便。 例如销售报告中每个产品的折线图,通常事先不知道确切的类别数量。
Data Query包提供了一个方便的groupBy方法,您可以使用该方法将记录分成组。它需要数据和一个 GroupDescriptor,输出是一个包含组及其项目的GroupResult。
以下示例演示如何为每个服务绘制折线图。
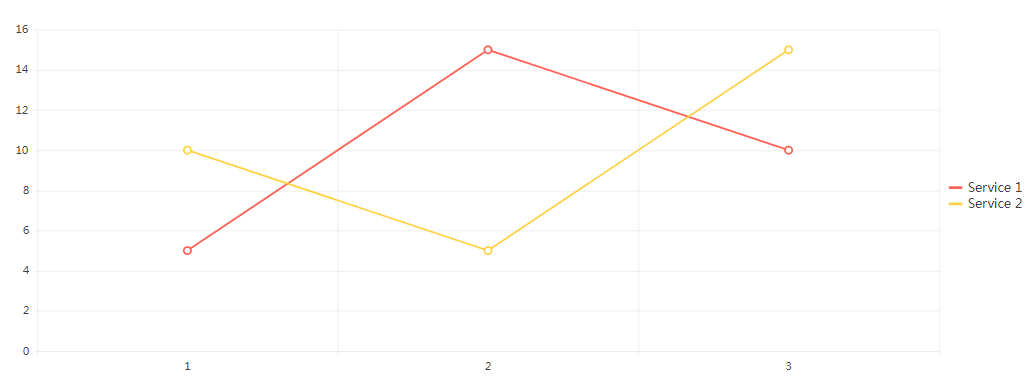
import { Component } from '@angular/core'; import { groupBy, GroupResult } from '@progress/kendo-data-query'; interface Sample { interval: number; service: string; value: number; } @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item *ngFor="let item of series" [data]="item.items" [name]="item.value" field="value" categoryField="interval" type="line"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public data: Sample[] = [{ interval: 1, service: 'Service 1', value: 5 }, { interval: 2, service: 'Service 1', value: 15 }, { interval: 3, service: 'Service 1', value: 10 }, { interval: 1, service: 'Service 2', value: 10 }, { interval: 2, service: 'Service 2', value: 5 }, { interval: 3, service: 'Service 2', value: 15 }]; public series: GroupResult[]; constructor() { this.series = groupBy(this.data, [{ field: 'service' }]) as GroupResult[]; // Inspect the resulting data structure in the console console.log(JSON.stringify(this.series, null, 2)); } }
绑定类别
Categorical Charts 的类别轴是一个数据绑定组件,就像系列一样。它支持以下提供类别列表的基本方法:
- 标签数组
- 类别字段
当 Chart 绑定日期时,类别轴提供专用功能。
标签数组
最简单的数据绑定形式涉及向轴提供类别标签数组,该列表将按原样显示,无需任何修改,系列数据点沿轴顺序定位。
- 类别的顺序与系列数据点的顺序无关。
- 类别的数量必须等于系列中数据点的数量。
- 为了保持顺序,系列中的缺失值必须用 null 表示。
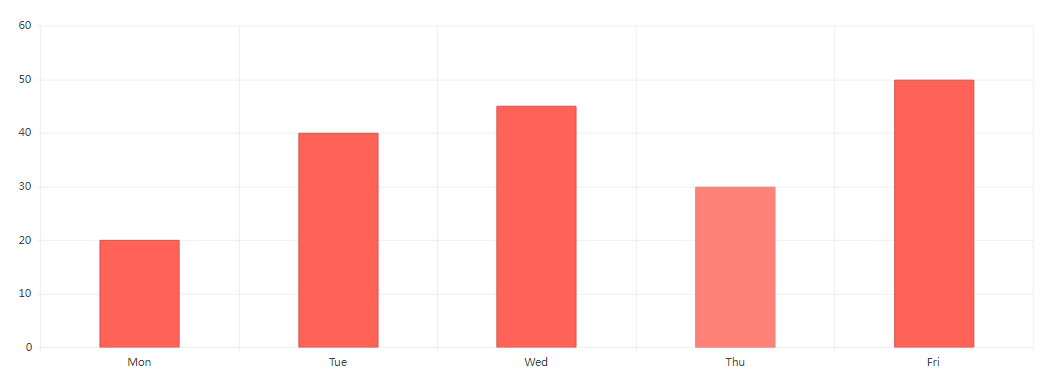
import { Component } from '@angular/core'; @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-category-axis> <kendo-chart-category-axis-item [categories]="categories"> </kendo-chart-category-axis-item> </kendo-chart-category-axis> <kendo-chart-series> <kendo-chart-series-item [data]="seriesData"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: number[] = [20, 40, 45, 30, 50]; public categories: string[] = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri']; }
类别字段
前一种方法容易出错,因为您需要维护类别和特定的数据顺序。 为避免此要求,请将类别绑定到与系列相同的模型对象。 这样,系列点和类别将始终自动匹配。
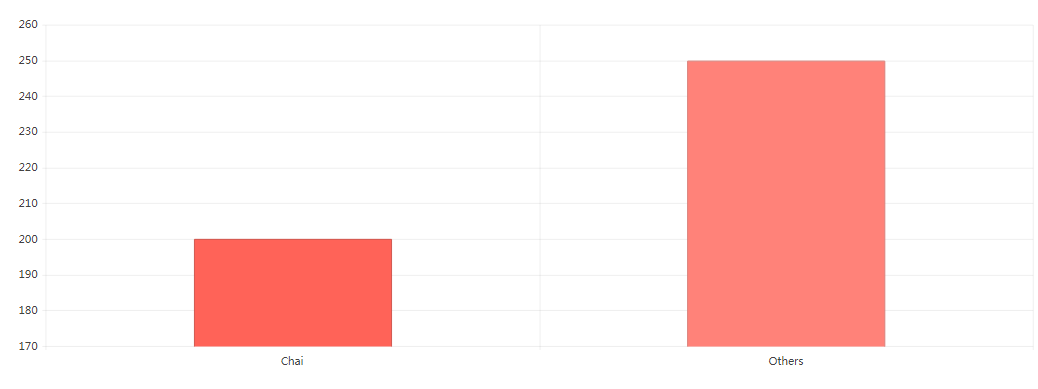
import { Component } from '@angular/core'; interface Model { product: string; sales: number; } @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item type="column" [data]="seriesData" field="sales" categoryField="product"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: Model[] = [{ product: 'Chai', sales: 200 }, { product: 'Others', sales: 250 }]; }
处理重复类别
绑定到类别字段可以让两个数据点具有相同的类别——以下示例演示了为“Chai”声明的两个值。
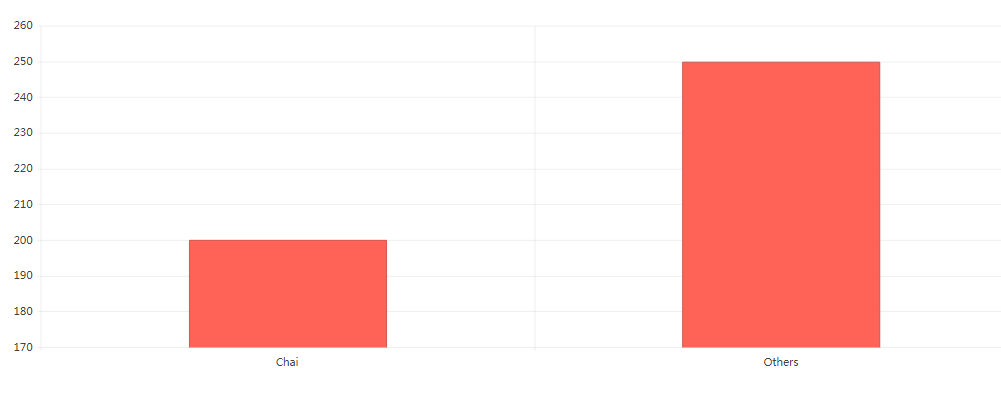
import { Component } from '@angular/core'; interface Model { product: string; sales: number; } @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item type="column" [data]="seriesData" field="sales" categoryField="product"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: Model[] = [{ product: 'Chai', sales: 200 }, { product: 'Chai', sales: 100 }, { product: 'Others', sales: 250 }]; }
在这种情况下,图表从源数据集中获取数据点,并使用聚合函数生成一个新点。
默认情况下,聚合函数返回值字段的最大值。 如果类别只包含一个点,则不加修改地返回它。 即使类别仅包含一个数据点,其他聚合(例如计数和总和)也会产生自己的值。
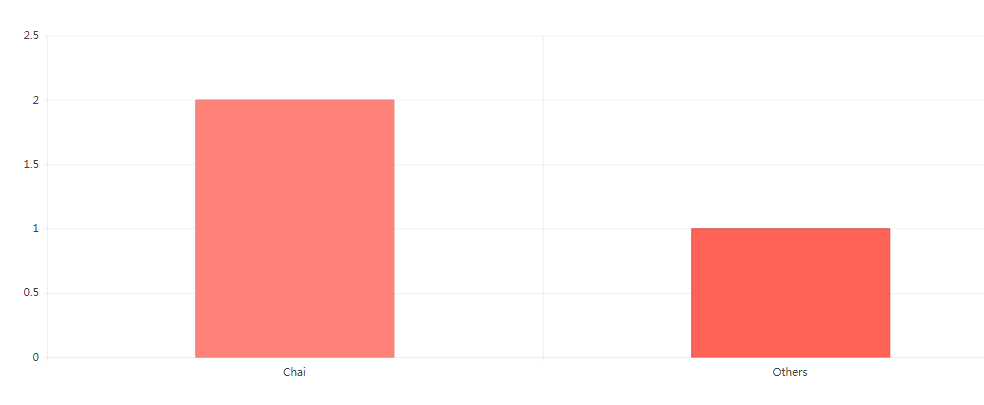
import { Component } from '@angular/core'; interface Model { product: string; sales: number; } @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item aggregate="count" type="column" [data]="seriesData" field="sales" categoryField="product"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: Model[] = [{ product: 'Chai', sales: 200 }, { product: 'Chai', sales: 100 }, { product: 'Others', sales: 250 }]; }
也可以定义自己的聚合函数,如下例所示。
使用类别绑定时,将对所有唯一类别执行聚合函数。
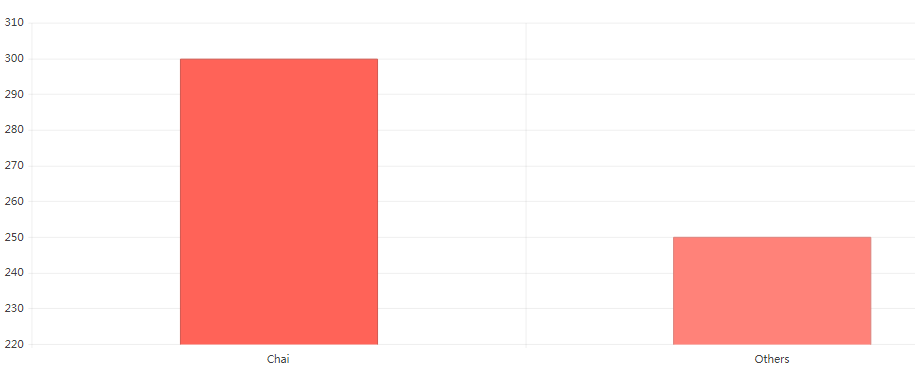
import { Component } from '@angular/core'; interface Model { product: string; sales: number; } @Component({ selector: 'my-app', template: ` <kendo-chart> <kendo-chart-series> <kendo-chart-series-item [aggregate]="myAggregate" type="column" [data]="seriesData" field="sales" categoryField="product"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: Model[] = [{ product: 'Chai', sales: 200 }, { product: 'Chai', sales: 100 }, { product: 'Others', sales: 250 }]; public myAggregate(values: number[], series: any, dataItems: Model[], category: string) { /* Return a sum of the values */ return values.reduce((n, acc) => acc + n, 0); } }
更新数据
替换数组实例
为了提高浏览器的性能,Chart使用了OnPush更改检测策略。这意味着组件不会检测对数组实例的更改——例如,当添加元素时。 要触发更改检测,请为集合创建一个新的数组实例——例如,设置一个this.data = [...this.data, {new item}],来替代this.data.push({new item})数组。
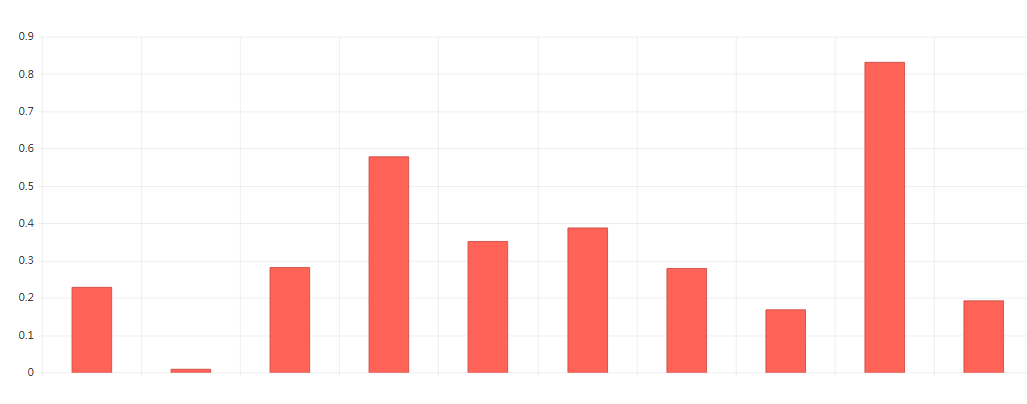
import { Component } from '@angular/core'; @Component({ selector: 'my-app', template: ` <kendo-chart [transitions]="false"> <kendo-chart-series> <kendo-chart-series-item [data]="seriesData"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { public seriesData: number[] = []; constructor() { setInterval(() => { // Clone the array const data = this.seriesData.slice(0); // Produce one random value each 100ms data.push(Math.random()); if (data.length > 10) { // Keep only 10 items in the array data.shift(); } // Replace with the new array instance this.seriesData = data; }, 100); } }
绑定到 Observable
当您将 Chart 绑定到 observable 时,组件会在每次新数据来自 observable 时自动更新它呈现的信息。 要将 Chart 绑定到 observable,请使用异步管道。
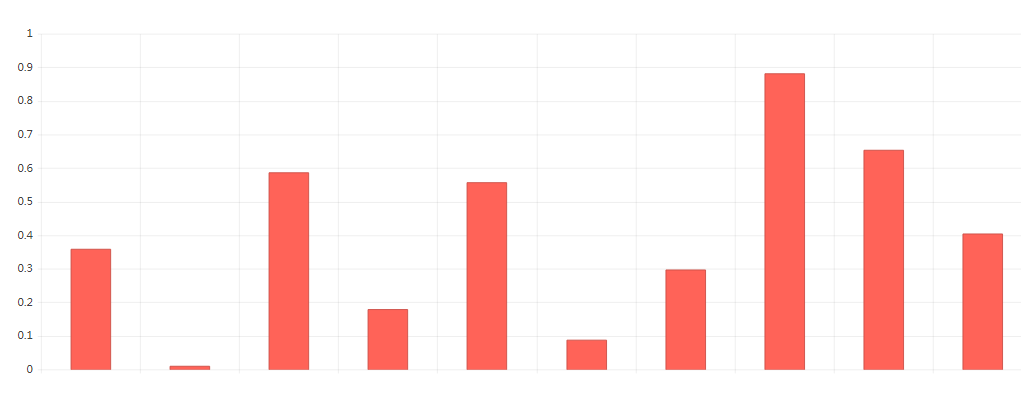
import { Component } from '@angular/core'; import { Observable, interval } from 'rxjs'; import { bufferCount, map } from 'rxjs/operators'; @Component({ selector: 'my-app', template: ` <kendo-chart [transitions]="false"> <kendo-chart-series> <kendo-chart-series-item type="column" [data]="seriesData | async"> </kendo-chart-series-item> </kendo-chart-series> </kendo-chart> ` }) export class AppComponent { /* Start with an empty observable */ public seriesData: Observable<number[]>; constructor() { /* Produce 1 random value each 100ms and emit it in batches of 10. */ this.seriesData = interval(100).pipe( map(i => Math.random()), bufferCount(10) ); } }
Kendo UI for Angular是Kendo UI系列商业产品的最新产品。Kendo UI for Angular是专用于Angular开发的专业级Angular组件。telerik致力于提供纯粹的高性能Angular UI组件,无需任何jQuery依赖关系。