Mayor's posters
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 59239 | Accepted: 17157 |
Description
The citizens of Bytetown, AB, could not stand that the candidates in the mayoral election campaign have been placing their electoral posters at all places at their whim. The city council has finally decided to build an electoral wall for placing the posters and introduce the following rules:
They have built a wall 10000000 bytes long (such that there is enough place for all candidates). When the electoral campaign was restarted, the candidates were placing their posters on the wall and their posters differed widely in width. Moreover, the candidates started placing their posters on wall segments already occupied by other posters. Everyone in Bytetown was curious whose posters will be visible (entirely or in part) on the last day before elections.
Your task is to find the number of visible posters when all the posters are placed given the information about posters' size, their place and order of placement on the electoral wall.
-
Every candidate can place exactly one poster on the wall.
-
All posters are of the same height equal to the height of the wall; the width of a poster can be any integer number of bytes (byte is the unit of length in Bytetown).
-
The wall is divided into segments and the width of each segment is one byte.
- Each poster must completely cover a contiguous number of wall segments.
They have built a wall 10000000 bytes long (such that there is enough place for all candidates). When the electoral campaign was restarted, the candidates were placing their posters on the wall and their posters differed widely in width. Moreover, the candidates started placing their posters on wall segments already occupied by other posters. Everyone in Bytetown was curious whose posters will be visible (entirely or in part) on the last day before elections.
Your task is to find the number of visible posters when all the posters are placed given the information about posters' size, their place and order of placement on the electoral wall.
Input
The first line of input contains a number c giving the number of cases that follow. The first line of data for a single case contains number 1 <= n <= 10000. The subsequent n lines describe the posters in the order in which they were placed. The i-th line among the n lines contains two integer numbers li and ri which are the number of the wall segment occupied by the left end and the right end of the i-th poster, respectively. We know that for each 1 <= i <= n, 1 <= li <= ri <= 10000000. After the i-th poster is placed, it entirely covers all wall segments numbered li, li+1 ,... , ri.
Output
For each input data set print the number of visible posters after all the posters are placed.
The picture below illustrates the case of the sample input.
The picture below illustrates the case of the sample input.
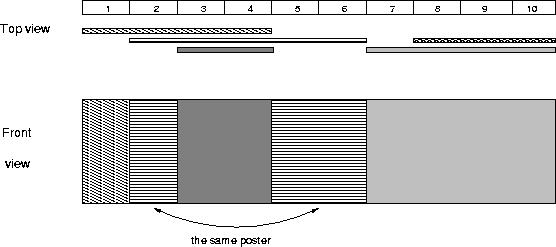
Sample Input
1 5 1 4 2 6 8 10 3 4 7 10
Sample Output
4
题目链接:POJ 2528
这题离散化不是很难容易想到我感觉麻烦的是对于query这个函数的查询方式,做了很久的一道题,很经典但是挺坑,数据虽说不是很严,一些不严谨的离散化也可以过,但数据本身应该是正确的。
用single 来表示区间是否单一颜色,最后加入颜色的时候别把颜色0加入即可
离散化我用的方法比较麻烦但感觉比较好理解,先给出现过的所有点排序,然后由于1~2+6~10离散之后会变成1~2 3~4 中间少了一段,因此对排序之后的数组遍历若出现pos[i]-pos[i-1]>1,则在数组末尾加上pos[i]-1(其他在pos[i]~pos[i-1]之间的数任意均可),然后再次对数组算上排序并去重unique。然后用lower_bound把坐标映射离散一下即可。
统计颜色个数,直接从大区间1~pos.size开始查询,直接暴力查询掉所有的叶子节点就行或者判断当前是否有颜色,有颜色就直接返回不用pushdown但是这种做法会超过建树的区间导致color出现0,特判一下0就行。
另外一种来自某kuangbin大牛(膜Orz...)的做法是倒序更新,倒着帖只要当前范围没被全部覆盖就说明张贴成功大,但是更新时要注意,每一次更新必须要pushup到顶端,不然下一次若更新到比较浅的层则会误认为当前区间可更新实际是没有传递信息上来(调试了大半个下午)。
如果用正序写过的推荐尝试一下写逆序更新,速度也比正序快一丢丢
暴力统计代码:
#include <stdio.h> #include <iostream> #include <algorithm> #include <cstdlib> #include <sstream> #include <cstring> #include <bitset> #include <string> #include <deque> #include <stack> #include <cmath> #include <queue> #include <set> #include <map> using namespace std; #define INF 0x3f3f3f3f #define CLR(x,y) memset(x,y,sizeof(x)) #define FAST_IO ios::sync_with_stdio(false);cin.tie(0); #define LC(x) (x<<1) #define RC(x) ((x<<1)+1) #define MID(x,y) ((x+y)>>1) typedef pair<int,int> pii; typedef long long LL; const double PI=acos(-1.0); const int N=10010; struct seg { int l,mid,r; bool single; int color; }; struct info { int l,r; }; seg T[N<<4]; info pos[N]; vector<int>xpos; set<int>Color; void init() { xpos.clear(); Color.clear(); } void pushdown(int k) { if(T[k].single) { T[LC(k)].single=T[RC(k)].single=true; T[LC(k)].color=T[RC(k)].color=T[k].color; T[k].single=false; } } void build(int k,int l,int r) { T[k].l=l; T[k].r=r; T[k].mid=MID(l,r); T[k].color=0; T[k].single=true; if(l==r) return ; build(LC(k),l,T[k].mid); build(RC(k),T[k].mid+1,r); } void update(int k,int l,int r,int color) { if(l<=T[k].l&&T[k].r<=r) { T[k].color=color; T[k].single=true; } else { pushdown(k); if(r<=T[k].mid) update(LC(k),l,r,color); else if(l>T[k].mid) update(RC(k),l,r,color); else update(LC(k),l,T[k].mid,color),update(RC(k),T[k].mid+1,r,color); } } void query(int k) { if(T[k].l==T[k].r||T[k].single) { if(T[k].color) Color.insert(T[k].color); return ; } else { pushdown(k); query(LC(k)); query(RC(k)); } } int main(void) { int tcase,n,i; scanf("%d",&tcase); while (tcase--) { init(); scanf("%d",&n); for (i=1; i<=n; ++i) { scanf("%d%d",&pos[i].l,&pos[i].r); xpos.push_back(pos[i].l); xpos.push_back(pos[i].r); } sort(xpos.begin(),xpos.end()); int sz=xpos.size(); for (i=1; i<sz; ++i) if(xpos[i]-xpos[i-1]>1) xpos.push_back(xpos[i-1]+1); sort(xpos.begin(),xpos.end()); xpos.erase(unique(xpos.begin(),xpos.end()),xpos.end()); build(1,1,xpos.size()); for (i=1; i<=n; ++i) { pos[i].l=lower_bound(xpos.begin(),xpos.end(),pos[i].l)-xpos.begin()+1; pos[i].r=lower_bound(xpos.begin(),xpos.end(),pos[i].r)-xpos.begin()+1; update(1,pos[i].l,pos[i].r,i); } query(1); printf("%d ",Color.size()); } return 0; }
倒序更新代码:
#include<stdio.h> #include<iostream> #include<algorithm> #include<cstdlib> #include<sstream> #include<cstring> #include<bitset> #include<string> #include<deque> #include<stack> #include<cmath> #include<queue> #include<set> #include<map> using namespace std; #define INF 0x3f3f3f3f #define CLR(x,y) memset(x,y,sizeof(x)) #define LC(x) (x<<1) #define RC(x) ((x<<1)+1) #define MID(x,y) ((x+y)>>1) typedef pair<int,int> pii; typedef long long LL; const double PI=acos(-1.0); const int N=40010; const int M=10010; struct info { int l,r; }node[M]; struct seg { int l,mid,r; int color; }T[N*8]; int kind; vector<int>pos; void pushup(int k) { if(T[LC(k)].color!=-1&&T[RC(k)].color!=-1) T[k].color=T[LC(k)].color; else T[k].color=-1; } void build(int k,int l,int r) { T[k].l=l; T[k].r=r; T[k].mid=MID(l,r); T[k].color=-1; if(l==r) return ; build(LC(k),l,T[k].mid); build(RC(k),T[k].mid+1,r); } bool post(int k,int l,int r,int c) { if(T[k].color!=-1) return false; if(T[k].l==l&&T[k].r==r) { T[k].color=c; return true; } else { bool mix;//就是这里 if(r<=T[k].mid) mix=post(LC(k),l,r,c);//直接return的话就不会pushup了 else if(l>T[k].mid) mix=post(RC(k),l,r,c); else { bool check_left=post(LC(k),l,T[k].mid,c); bool check_right=post(RC(k),T[k].mid+1,r,c); mix=check_left||check_right; } pushup(k); return mix; } } void init() { kind=0; pos.clear(); } int main(void) { int tcase,n,i; scanf("%d",&tcase); while (tcase--) { scanf("%d",&n); init(); for (i=0; i<n; ++i) { scanf("%d%d",&node[i].l,&node[i].r); pos.push_back(node[i].l); pos.push_back(node[i].r); } sort(pos.begin(),pos.end()); int SZ=pos.size(); for (i=1; i<SZ; ++i) if(pos[i-1]<pos[i]-1) pos.push_back(pos[i]-1); sort(pos.begin(),pos.end()); pos.erase(unique(pos.begin(),pos.end()),pos.end()); for (i=0; i<n; ++i) { node[i].l=lower_bound(pos.begin(),pos.end(),node[i].l)-pos.begin()+1; node[i].r=lower_bound(pos.begin(),pos.end(),node[i].r)-pos.begin()+1; } build(1,1,pos.size()); for (i=n-1; i>=0; --i)//这里循环要改成逆序 kind+=post(1,node[i].l,node[i].r,i+1); printf("%d ",kind); } return 0; }