已经很久没有更新博客了,一是因为换了公司,完全是断网开发了,没有时间来写博客,最主要的就是温水煮青蛙,自己在舒适的环境中越来越懒了,最近打算强制自己更新一波。不知道能坚持多久。由于目前没有具体的Qt项目,智能更具书本内容来写博客。最为一种数据搬运和记录。本文内容来之Qt开发及实例第三版
QPalette类主要有两个基本概念,一个是ColorGroup,另一个是ColorRole。其中ColorGroup指的是一下三种不同的状态
1. QPalette::Active: 获得焦点的状态
2. QPalette::Inactive: 未获得焦点的状态
3. QPalette::Disable:不可用状态
其中Active和Inactive状态在通常情况下,颜色显示是一致的。也可以根据需求设置为不一样的颜色。
1. QPalette::Active: 获得焦点的状态
2. QPalette::Inactive: 未获得焦点的状态
3. QPalette::Disable:不可用状态
其中Active和Inactive状态在通常情况下,颜色显示是一致的。也可以根据需求设置为不一样的颜色。
ColorRole指的是颜色的主题,即对窗体中不同部位颜色的分类。例如QPalette::Window是指背景色QPalette::WindowText指的是前景色,等等。
下面是运行代码截图
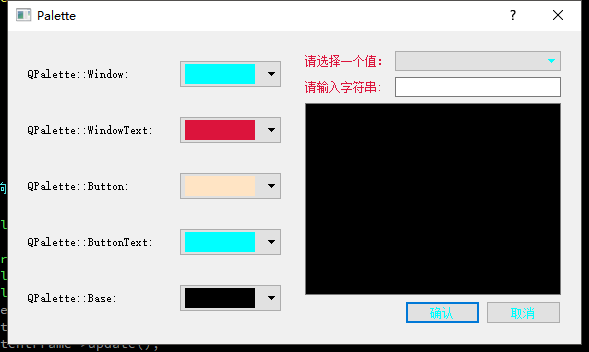
下面是源代码分享
palette.h
palette.h
#ifndef PALETTE_H #define PALETTE_H #include <QDialog> #include <QComboBox> #include <QLabel> #include <QTextEdit> #include <QPushButton> #include <QLineEdit> class Palette : public QDialog { Q_OBJECT public: Palette(QWidget *parent = 0); ~Palette(); void createCtrlFrame(); //完成窗体左半部分颜色选择区的创建 void createContentFrame(); //完成窗体右半部分的创建 void fillColorList(QComboBox *comboBox); //完成向颜色下拉列表框中插入颜色的工作 private slots: void ShowWindow(); void ShowWindowText(); void ShowButton(); void ShowButtonText(); void ShowBase(); private: QFrame *ctrlFrame; //颜色选择面板 QLabel *windowLabel; QComboBox *windowComboBox; QLabel *windowTextLabel; QComboBox *windowTextComboBox; QLabel *buttonLabel; QComboBox *buttonComboBox; QLabel *buttonTextLabel; QComboBox *buttonTextComboBox; QLabel *baseLabel; QComboBox *baseComboBox; QFrame *contentFrame; //具体显示面板 QLabel *label1; QComboBox *comboBox1; QLabel *label2; QLineEdit *lineEdit2; QTextEdit *textEdit; QPushButton *OkBtn; QPushButton *CancelBtn; }; #endif // PALETTE_H
palette.cpp
#include "palette.h" #include <QHBoxLayout> #include <QGridLayout> Palette::Palette(QWidget *parent) : QDialog(parent) { createCtrlFrame(); createContentFrame(); QHBoxLayout *mainLayout =new QHBoxLayout(this); mainLayout->addWidget(ctrlFrame); mainLayout->addWidget(contentFrame); } Palette::~Palette() { } void Palette::createCtrlFrame() { ctrlFrame =new QFrame; //颜色选择面板 windowLabel =new QLabel(tr("QPalette::Window: ")); windowComboBox =new QComboBox; //创建一个QComboBox对象 fillColorList(windowComboBox); connect(windowComboBox,SIGNAL(activated(int)),this,SLOT(ShowWindow())); windowTextLabel =new QLabel(tr("QPalette::WindowText: ")); windowTextComboBox =new QComboBox; fillColorList(windowTextComboBox); connect(windowTextComboBox,SIGNAL(activated(int)),this,SLOT(ShowWindowText())); buttonLabel =new QLabel(tr("QPalette::Button: ")); buttonComboBox =new QComboBox; fillColorList(buttonComboBox); connect(buttonComboBox,SIGNAL(activated(int)),this,SLOT(ShowButton())); buttonTextLabel =new QLabel(tr("QPalette::ButtonText: ")); buttonTextComboBox =new QComboBox; fillColorList(buttonTextComboBox); connect(buttonTextComboBox,SIGNAL(activated(int)),this,SLOT(ShowButtonText())); baseLabel =new QLabel(tr("QPalette::Base: ")); baseComboBox =new QComboBox; fillColorList(baseComboBox); connect(baseComboBox,SIGNAL(activated(int)),this,SLOT(ShowBase())); QGridLayout *mainLayout=new QGridLayout(ctrlFrame); mainLayout->setSpacing(20); mainLayout->addWidget(windowLabel,0,0); mainLayout->addWidget(windowComboBox,0,1); mainLayout->addWidget(windowTextLabel,1,0); mainLayout->addWidget(windowTextComboBox,1,1); mainLayout->addWidget(buttonLabel,2,0); mainLayout->addWidget(buttonComboBox,2,1); mainLayout->addWidget(buttonTextLabel,3,0); mainLayout->addWidget(buttonTextComboBox,3,1); mainLayout->addWidget(baseLabel,4,0); mainLayout->addWidget(baseComboBox,4,1); } void Palette::createContentFrame() { contentFrame =new QFrame; //具体显示面板 label1 =new QLabel(tr("请选择一个值:")); comboBox1 =new QComboBox; label2 = new QLabel(tr("请输入字符串: ")); lineEdit2 =new QLineEdit; textEdit =new QTextEdit; QGridLayout *TopLayout =new QGridLayout; TopLayout->addWidget(label1,0,0); TopLayout->addWidget(comboBox1,0,1); TopLayout->addWidget(label2,1,0); TopLayout->addWidget(lineEdit2,1,1); TopLayout->addWidget(textEdit,2,0,1,2); OkBtn =new QPushButton(tr("确认")); CancelBtn =new QPushButton(tr("取消")); QHBoxLayout *BottomLayout =new QHBoxLayout; BottomLayout->addStretch(1); BottomLayout->addWidget(OkBtn); BottomLayout->addWidget(CancelBtn); QVBoxLayout *mainLayout =new QVBoxLayout(contentFrame); mainLayout->addLayout(TopLayout); mainLayout->addLayout(BottomLayout); } void Palette::fillColorList(QComboBox *comboBox) { QStringList colorList = QColor::colorNames(); QString color; foreach(color,colorList) { QPixmap pix(QSize(70,20)); pix.fill(QColor(color)); comboBox->addItem(QIcon(pix),NULL); comboBox->setIconSize(QSize(70,20)); comboBox->setSizeAdjustPolicy(QComboBox::AdjustToContents); //(f) } } /* * 用于响应对背景颜色的选择 */ void Palette::ShowWindow() { QStringList colorList = QColor::colorNames(); QColor color = QColor(colorList[windowComboBox->currentIndex()]); QPalette p = contentFrame->palette(); p.setColor(QPalette::Window,color); contentFrame->setPalette(p); contentFrame->update(); } /* * 用于响应对应文字颜色的选择 */ void Palette::ShowWindowText() { QStringList colorList = QColor::colorNames(); QColor color = colorList[windowTextComboBox->currentIndex()]; QPalette p = contentFrame->palette(); p.setColor(QPalette::WindowText,color); contentFrame->setPalette(p); } void Palette::ShowButton() { QStringList colorList = QColor::colorNames(); QColor color =QColor(colorList[buttonComboBox->currentIndex()]); QPalette p = contentFrame->palette(); p.setColor(QPalette::Button,color); contentFrame->setPalette(p); contentFrame->update(); } void Palette::ShowButtonText() { QStringList colorList = QColor::colorNames(); QColor color = QColor(colorList[buttonTextComboBox->currentIndex()]); QPalette p =contentFrame->palette(); p.setColor(QPalette::ButtonText,color); contentFrame->setPalette(p); } void Palette::ShowBase() { QStringList colorList = QColor::colorNames(); QColor color = QColor(colorList[baseComboBox->currentIndex()]); QPalette p = contentFrame->palette(); p.setColor(QPalette::Base,color); contentFrame->setPalette(p); }