一、建立数据库
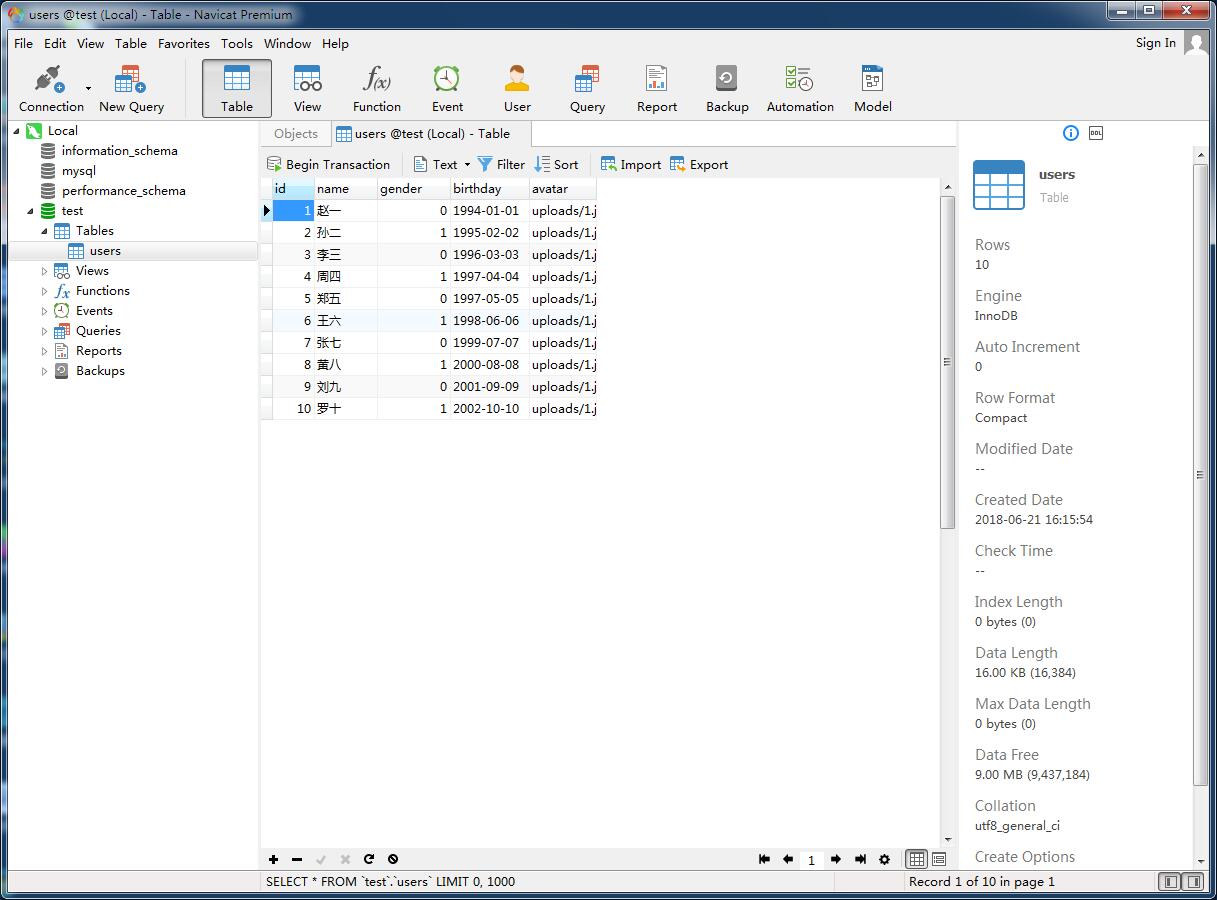
二、首页展示
<?php
//1.建立连接
$conn=mysqli_connect('localhost','root','0000','test');
if(!$conn){
exit('<h1>数据库连接失败</h1>');
}
//2.开始查询
$query=mysqli_query($conn,'select * from users;');
if(!$query){
exit('<h1>查询过程失败</h1>');
}
///3.遍历结果集
// while($item=mysqli_fetch_assoc($query)){
// var_dump($item);
// }
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>管理系统</title>
<link rel="stylesheet" href="bootstrap.css">
</head>
<body>
<nav class="navbar navbar-expand navbar-dark bg-dark fixed-top">
<a class="navbar-brand" href="#">管理系统</a>
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a class="nav-link" href="index.php">用户管理</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">商品管理</a>
</li>
</ul>
</nav>
<main class="container mt-5">
<h1 class="alert-heading">用户管理
<a class="btn btn-link btn-sm" href="add.php">添加</a>
</h1>
<table class="table table-hover">
<thead>
<tr>
<th>#</th>
<th>头像</th>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
<th class="text-center" width="140">操作</th>
</tr>
</thead>
<tbody>
<?php while($item=mysqli_fetch_assoc($query)): ?>
<tr>
<th scope="row"><?php echo $item['id'] ?></th>
<td>
<img src="<?php echo $item['avatar']; ?>" class="rounded" alt="<?php echo $item['name']; ?>">
</td>
<td><?php echo $item['name']; ?></td>
<td><?php echo $item['gender']==0?'男':'女'; ?></td>
<td><?php echo $item['birthday']; ?></td>
<td class="text-center">
<a class="btn btn-info btn-sm" href="edit.php?id=<?php echo $item['id']?>">编辑</a>
<a class="btn btn-danger btn-sm" href="delete.php?id=<?php echo $item['id']?>">删除</a>
</td>
</tr>
<?php endwhile ?>
</tbody>
</table>
<ul class="pagination justify-content-center">
<li class="page-item"><a class="page-link" href="#">«</a></li>
<li class="page-item"><a class="page-link" href="#">1</a></li>
<li class="page-item"><a class="page-link" href="#">2</a></li>
<li class="page-item"><a class="page-link" href="#">3</a></li>
<li class="page-item"><a class="page-link" href="#">»</a></li>
</ul>
</main>
</body>
</html>
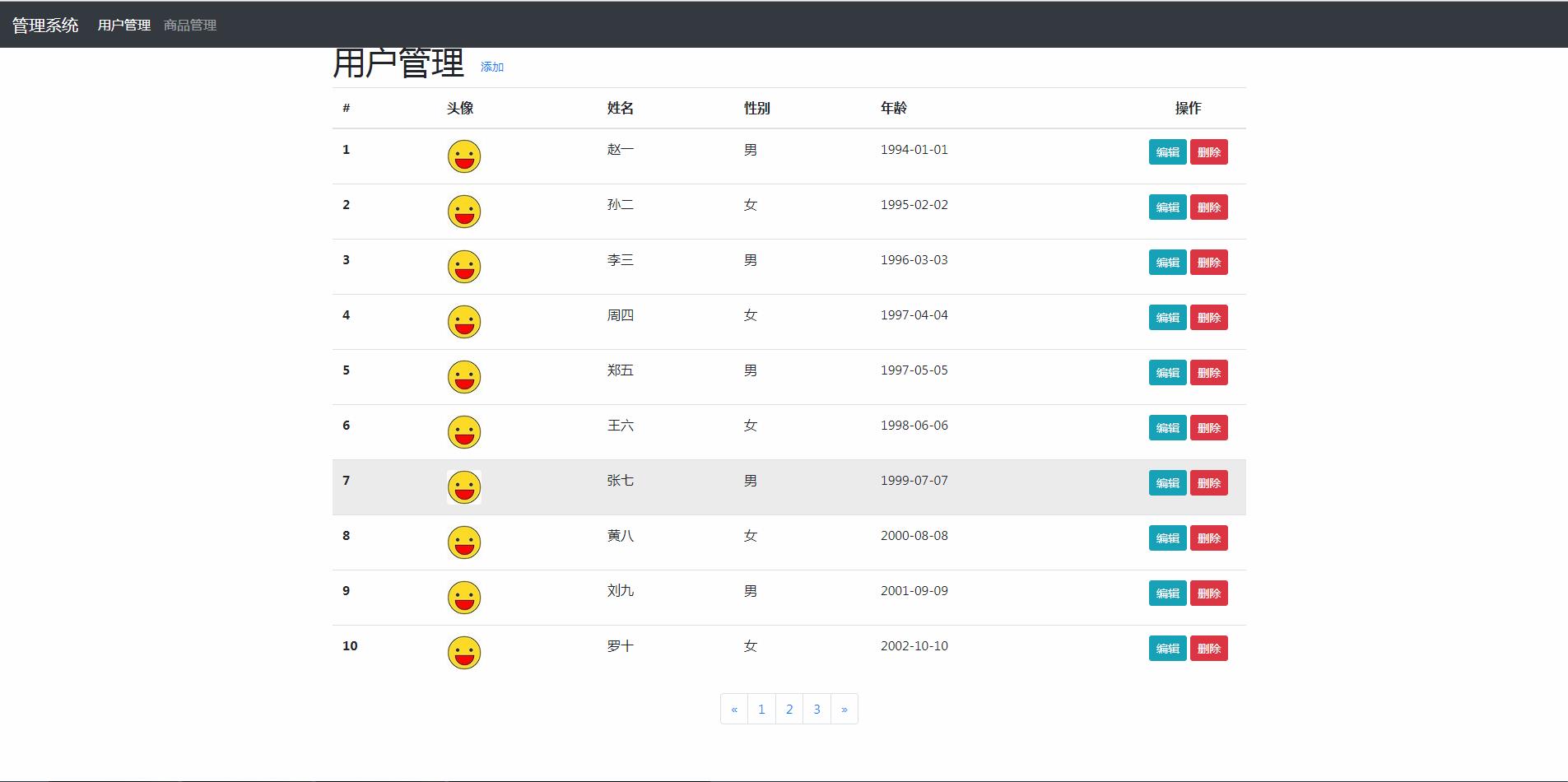
三、删除功能实现
<?php
//接收要删除的数据 ID
if(empty($_GET['id'])){
exit('<h1>必须传入指定参数</h1>');
}
$id=$_GET['id'];
//建立连接
$conn=mysqli_connect('localhost','root','0000','test');
if(!$conn){
exit('<h1>数据库连接失败</h1>');
}
//开始查询
$query=mysqli_query($conn,'delete from users where id= ' . $id .';');
if(!$query){
exit('<h1>查询数据失败</h1>');
}
$affected_rows=mysqli_affected_rows($conn);
if($affected_rows<=0){
exit('<h1>删除数据失败</h1>');
}
//跳转页面
header('Location: index.php');
?>
四、添加功能的实现
<?php
function add_user(){
//1.验证非空
if(empty($_POST['coding'])){
$GLOBALS['error_message']='请输入编码';
return;
}
if(empty($_POST['name'])){
$GLOBALS['error_message']='请输入姓名';
return;
}
if(!isset($_POST['gender']) && $_POST['gender']!=='-1'){
$GLOBALS['error_message']='请输入性别';
return;
}
if(empty($_POST['birthday'])){
$GLOBALS['error_message']='请输入日期';
return;
}
//2.取值
$coding=$_POST['coding'];
$name=$_POST['name'];
$gender=$_POST['gender'];
$birthday=$_POST['birthday'];
//3.接收文件并验证
if(empty($_FILES['avatar'])){
$GLOBALS['error_message']='请上传头像';
return;
}
$ext=pathinfo($_FILES['avatar']['name'],PATHINFO_EXTENSION);//扩展名==>jpg
$target='uploads/' . uniqid() . '.' .$ext;
if(!move_uploaded_file($_FILES['avatar']['tmp_name'],$target)){
$GLOBALS['error_message']='上传头像失败';
return;
}
$avatar=$target;
//4.保存
$conn=mysqli_connect('localhost','root','0000','test');
if(!$conn){
$GLOBALS['error_message']='连接数据库失败';
return;
}
$query=mysqli_query($conn,"insert into users values({$coding},'{$name}',{$gender},'{$birthday}','{$avatar}');");
if(!$query){
$GLOBALS['error_message']='查询过程失败';
return;
}
$affectd_rows=mysqli_affected_rows($conn);
if($affectd_rows!==1){
$GLOBALS['error_message']='添加数据失败';
return;
}
//5.响应
header('Location: index.php');
}
if($_SERVER['REQUEST_METHOD']==='POST'){
add_user();
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>添加数据</title>
<link rel="stylesheet" href="bootstrap.css">
</head>
<body>
<nav class="navbar navbar-expand navbar-dark bg-dark fixed-top">
<a href="#" class="navbar-brand">管理系统</a>
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a href="index.php" class="nav-link">用户管理</a>
</li>
<li class="nav-item active">
<a href="#" class="nav-link">商品管理</a>
</li>
</ul>
</nav>
<main class="container mt-5">
<h1 class="alert-heading">添加用户</h1>
<?php if(isset($error_message)): ?>
<div class="alert alert-warning">
<?php echo $error_message; ?>
</div>
<?php endif ?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" enctype="multipart/form-data" autocomplete="off">
<div class="form-group">
<label for="avatar">头像</label>
<input type="file" name="avatar" id="avatar" class="form-control">
</div>
<div class="form-group">
<label for="coding">编号</label>
<input type="text" name="coding" id="coding" class="form-control">
</div>
<div class="form-group">
<label for="name">姓名</label>
<input type="text" name="name" id="name" class="form-control">
</div>
<div class="form-group">
<label for="gender">性别</label>
<select name="gender" id="gender" class="form-control">
<option value="-1">请选择性别</option>
<option value="0">男</option>
<option value="1">女</option>
</select>
</div>
<div class="form-group">
<label for="birthday">生日</label>
<input type="date" name="birthday" id="birthday" class="form-control">
</div>
<button class="btn btn-primary">保存</button>
</form>
</main>
</body>
</html>
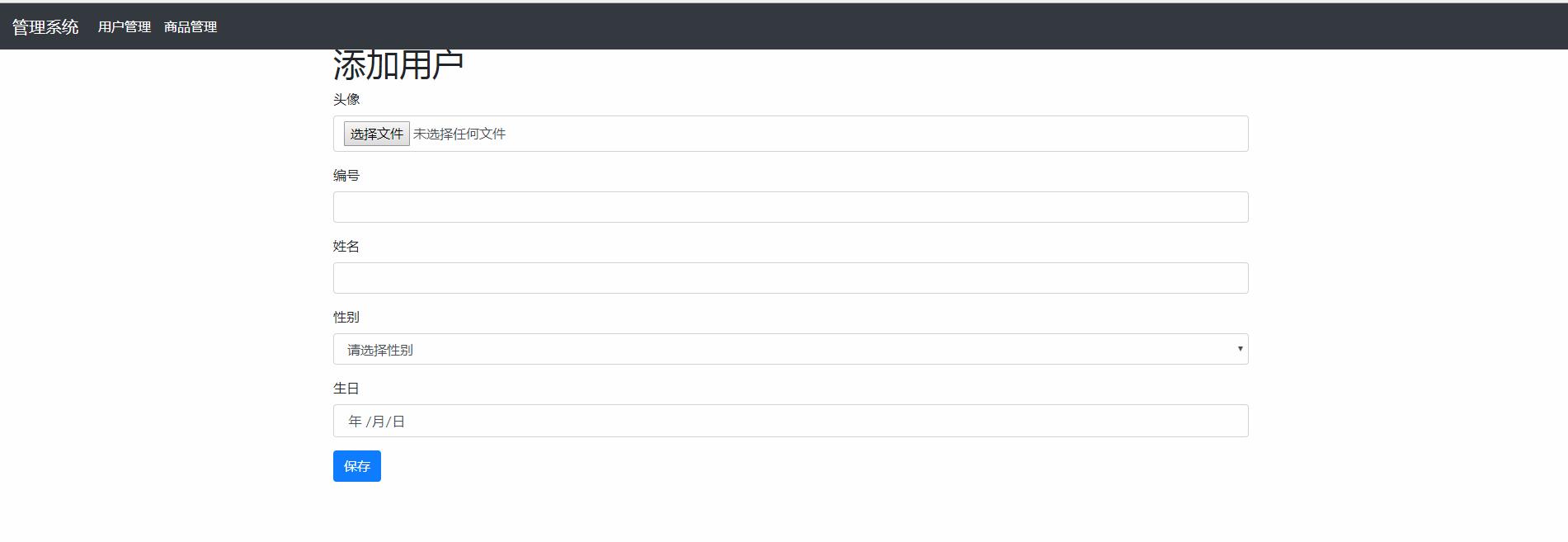
五、编辑功能的实现
<?php
//接收需要修改的ID
if(empty($_GET['id'])){
exit('<h1>必须传入指定参数</h1>');
}
$id=$_GET['id'];
//建立连接
$conn=mysqli_connect('localhost','root','0000','test');
if(!$conn){
exit('<h1>数据库连接失败</h1>');
}
//开始查询(因为ID是唯一的,找到第一个满足条件的就不用继续了)
$query=mysqli_query($conn,"select *from users where id={$id} limit 1;");
if(!$query){
exit('<h1>查询数据失败</h1>');
}
$user=mysqli_fetch_assoc($query);
if(!$user){
exit('<h1>找不到你要编辑的数据</h1>');
}
function edit(){
global $user;
//1.验证非空
if(empty($_POST['name'])){
$GLOBALS['error_message']='请输入姓名';
return;
}
if(!isset($_POST['gender']) && $_POST['gender']!=='-1'){
$GLOBALS['error_message']='请输入性别';
return;
}
if(empty($_POST['birthday'])){
$GLOBALS['error_message']='请输入日期';
return;
}
//2.取值
$user['name']=$_POST['name'];
$user['gender']=$_POST['gender'];
$user['birthday']=$_POST['birthday'];
//3.有上传就修改(用户上传了新头像)
if(isset($_FILES['avatar']) && $_FILES['avatar']['error']===UPLOAD_ERR_OK){
$ext=pathinfo($_FILES['avatar']['name'],PATHINFO_EXTENSION);
$target='uploads/'. uniqid() . '.' .$ext;
if(!move_uploaded_file($_FILES['avatar']['tmp_name'],$target)){
$GLOBALS['error_message']='上传头像失败';
return;
}
$user['avator']=$target;
}
//4.保存
$conn=mysqli_connect('localhost','root','0000','test');
if(!$conn){
$GLOBALS['error_message']='连接数据库失败';
return;
}
$query=mysqli_query($conn,"update users set name='{$user['name']}',gender={$user['gender']},birthday='{$user['birthday']}',avatar='{$user['avatar']}' where id={$user['id']}");
if(!$query){
$GLOBALS['error_message']='查询过程失败';
return;
}
$affectd_rows=mysqli_affected_rows($conn);
if($affectd_rows!==1){
$GLOBALS['error_message']='添加数据失败';
return;
}
//5.响应
header('Location: index.php');
}
if($_SERVER['REQUEST_METHOD']==='POST'){
edit();
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>编辑数据</title>
<link rel="stylesheet" href="bootstrap.css">
</head>
<body>
<nav class="navbar navbar-expand navbar-dark bg-dark fixed-top">
<a href="#" class="navbar-brand">管理系统</a>
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a href="index.php" class="nav-link">用户管理</a>
</li>
<li class="nav-item active">
<a href="#" class="nav-link">商品管理</a>
</li>
</ul>
</nav>
<main class="container mt-5">
<h1 class="alert-heading">编辑"<?php echo $user['name']; ?>"</h1>
<?php if(isset($error_message)): ?>
<div class="alert alert-warning">
<?php echo $error_message; ?>
</div>
<?php endif ?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>?id=<?php echo $user['id']; ?>" method="post" enctype="multipart/form-data" autocomplete="off">
<img src="<?php echo $user['avatar']; ?>" alt="">
<div class="form-group">
<label for="avatar">头像</label>
<input type="file" name="avatar" id="avatar" class="form-control">
</div>
<input type="hidden" name="coding" id="coding" class="form-control" value="<?php echo $user['id']; ?>">
<div class="form-group">
<label for="name">姓名</label>
<input type="text" name="name" id="name" class="form-control" value="<?php echo $user['name']; ?>">
</div>
<div class="form-group">
<label for="gender">性别</label>
<select name="gender" id="gender" class="form-control">
<option value="-1">请选择性别</option>
<option value="0"<?php echo $user['gender']==='0'? 'selected':''; ?>>男</option>
<option value="1"<?php echo $user['gender']==='1'? 'selected':''; ?>>女</option>
</select>
</div>
<div class="form-group">
<label for="birthday">生日</label>
<input type="date" name="birthday" id="birthday" class="form-control" value="<?php echo $user['birthday']; ?>">
</div>
<button class="btn btn-primary">保存</button>
</form>
</main>
</body>
</html>
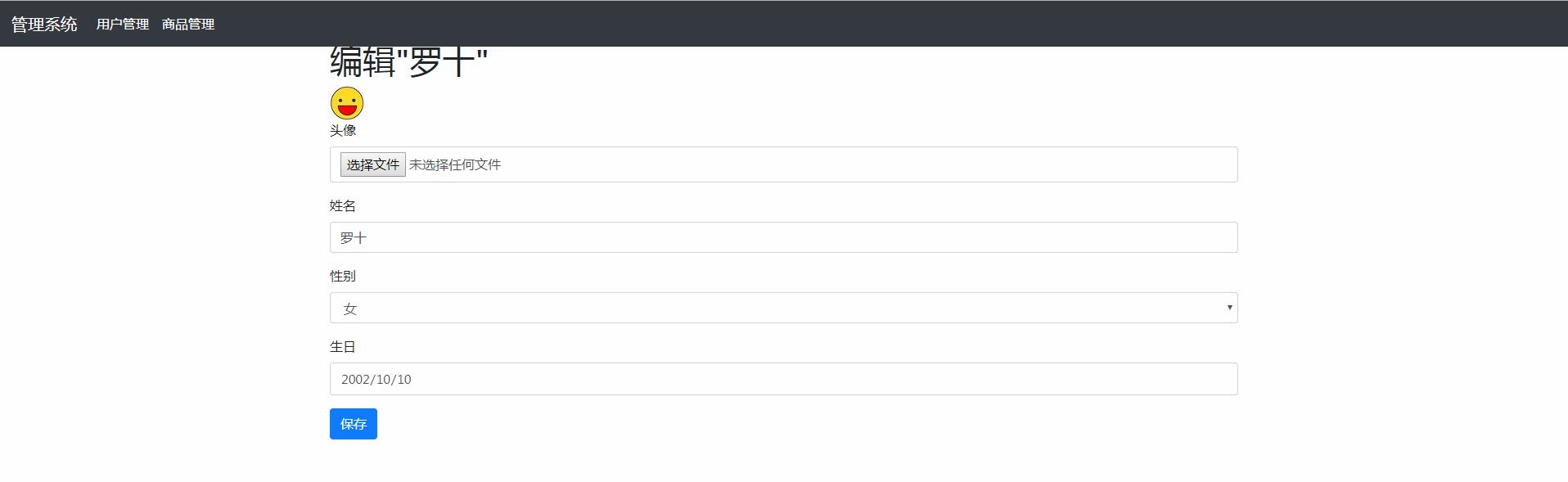
六、文件夹:
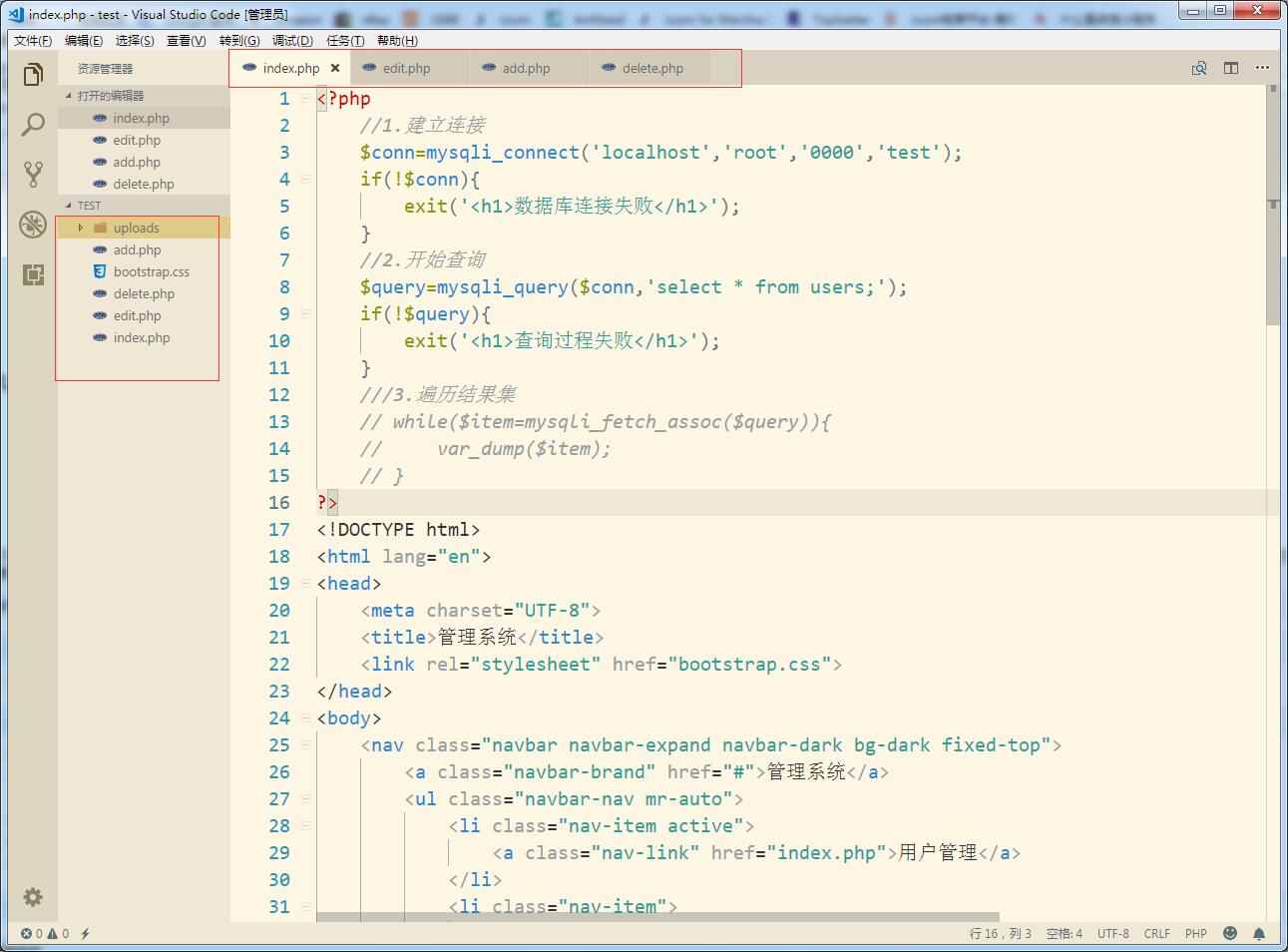
七、效果展示
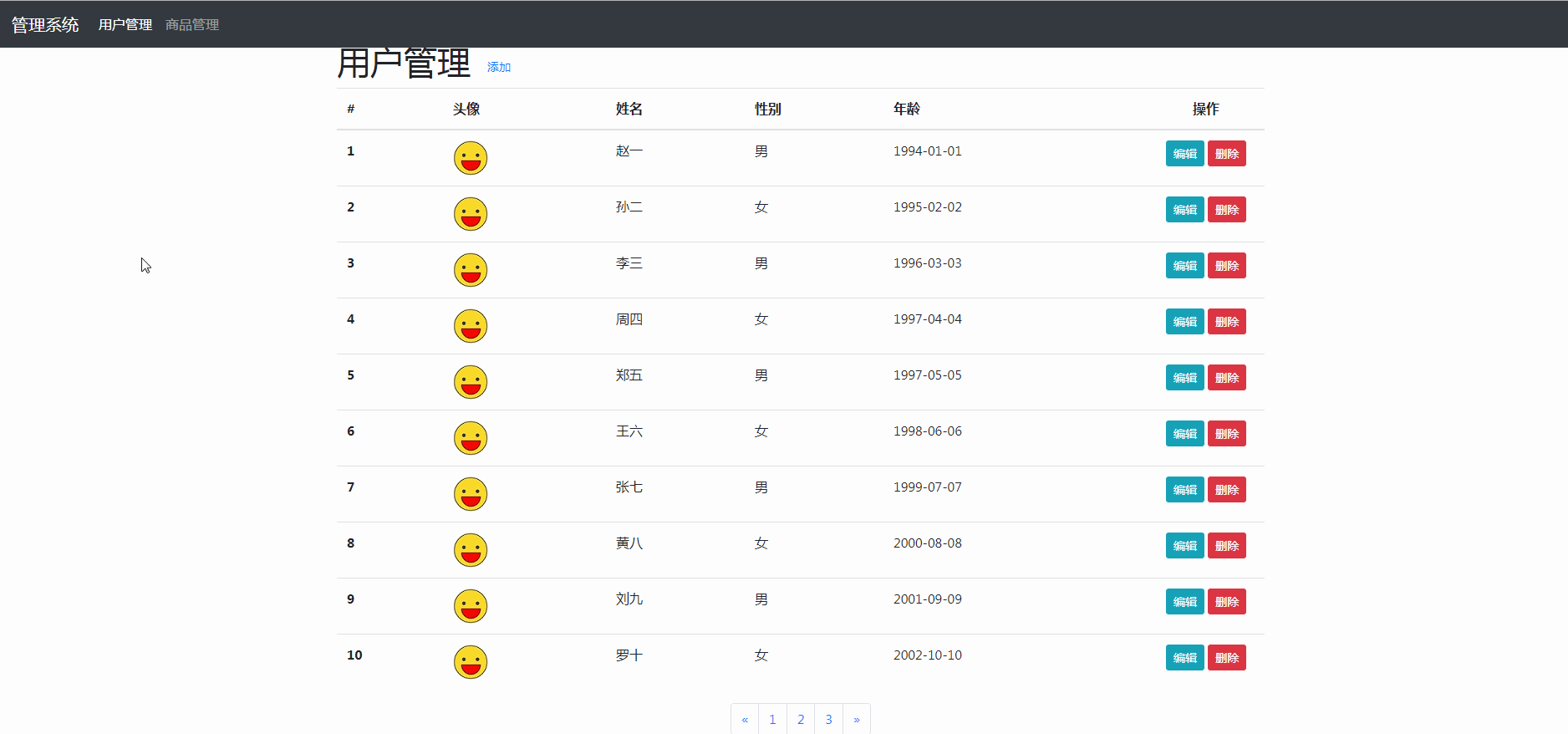