link
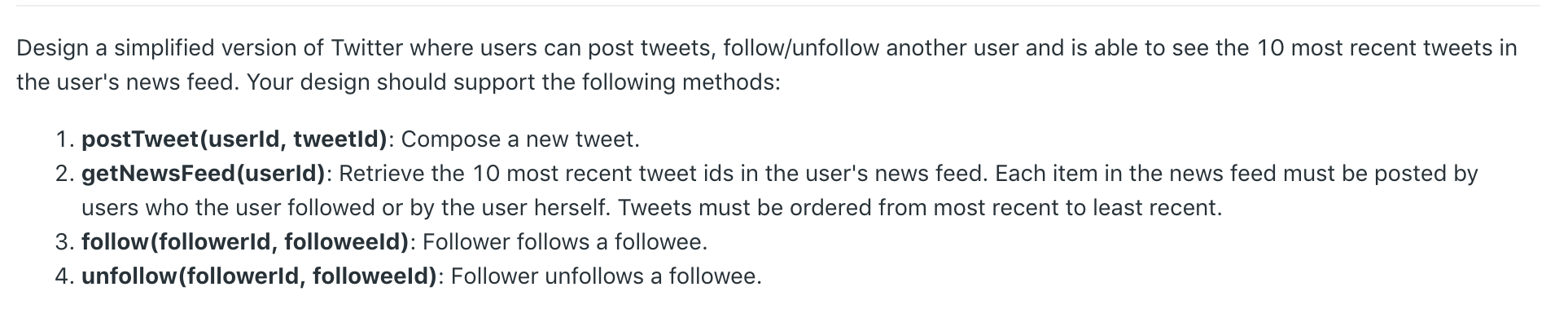
class Twitter {
public:
typedef list<pair<int,int>>::iterator It;
unordered_map<int,list<pair<int,int>>> usertotweet;
unordered_map<int,unordered_set<int>> friends;
int time;
/** Initialize your data structure here. */
Twitter() {
time=0;
}
/** Compose a new tweet. */
void postTweet(int userId, int tweetId) {
usertotweet[userId].push_front({tweetId,++time});
if(usertotweet[userId].size()>10) usertotweet[userId].pop_back();
}
/** Retrieve the 10 most recent tweet ids in the user's news feed. Each item in the news feed must be posted by users who the user followed or by the user herself. Tweets must be ordered from most recent to least recent. */
struct Comp{
bool operator()(pair<It,It> p1, pair<It,It> p2){
return p1.first->second<p2.first->second;
}
};
vector<int> getNewsFeed(int userId) {
priority_queue<pair<It,It>, vector<pair<It,It>>,Comp> pq;
if(usertotweet[userId].begin()!=usertotweet[userId].end()) pq.push({usertotweet[userId].begin(),usertotweet[userId].end()});
for(int f:friends[userId]){
if(f==userId) continue;
if(usertotweet[f].begin()!=usertotweet[f].end()) pq.push({usertotweet[f].begin(),usertotweet[f].end()});
}
vector<int> res;
while(!pq.empty()){
auto cur=pq.top();
pq.pop();
res.push_back(cur.first->first);
if(res.size()==10) break;
++cur.first;
if(cur.first!=cur.second){
pq.push({cur.first,cur.second});
}
}
return res;
}
/** Follower follows a followee. If the operation is invalid, it should be a no-op. */
void follow(int followerId, int followeeId) {
friends[followerId].insert(followeeId);
}
/** Follower unfollows a followee. If the operation is invalid, it should be a no-op. */
void unfollow(int followerId, int followeeId) {
friends[followerId].erase(followeeId);
}
};
/**
* Your Twitter object will be instantiated and called as such:
* Twitter* obj = new Twitter();
* obj->postTweet(userId,tweetId);
* vector<int> param_2 = obj->getNewsFeed(userId);
* obj->follow(followerId,followeeId);
* obj->unfollow(followerId,followeeId);
*/