ASP.NET Web API和ASP.NET Web MVC中使用Ninject
一、准备工作
1、新建一个名为MvcDemo的空解决方案
2、新建一个名为MvcDemo.WebUI的空MVC应用程序
3、使用NuGet安装Ninject库
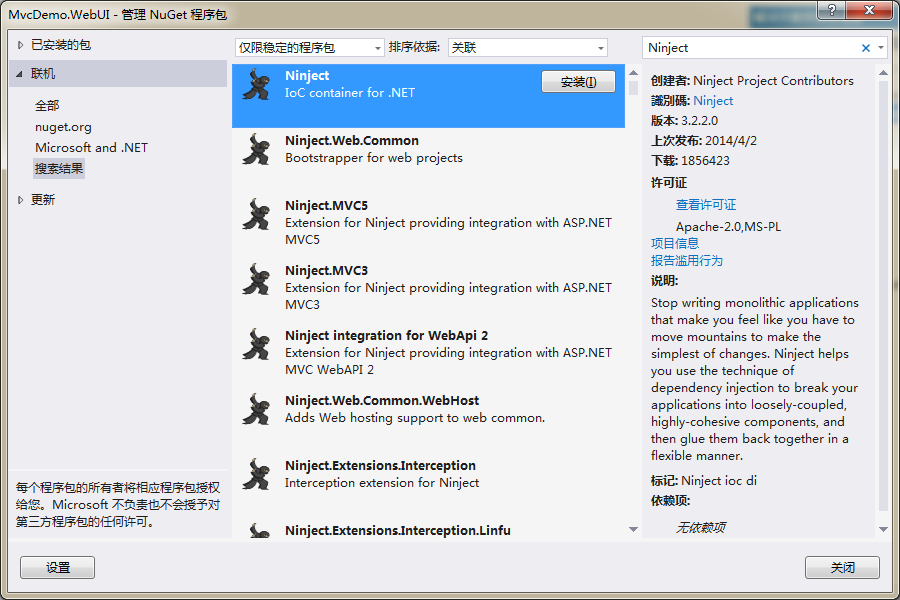
二、在ASP.NET MVC中使用Ninject
1、新建一个Product实体类,代码如下:
public class Product { public int ProductId { get; set ; } public string Name { get; set ; } public string Description { get; set ; } public decimal Price { get; set ; } public string Category { set; get ; } }
2、添加一个IProductRepository接口及实现
public interface IProductRepository { IQueryable <Product > Products { get; } IQueryable <Product > GetProducts(); Product GetProduct(); bool AddProduct(Product product); bool UpdateProduct(Product product); bool DeleteProduct(int productId); } public class ProductRepository : IProductRepository { private List < Product> list; public IQueryable < Product> Products { get { return GetProducts(); } } public IQueryable < Product> GetProducts() { list = new List < Product> { new Product {ProductId = 1,Name = "苹果",Category = "水果" ,Price = 1}, new Product {ProductId = 2,Name = "鼠标",Category = "电脑配件" ,Price = 50}, new Product {ProductId = 3,Name = "洗发水",Category = "日用品" ,Price = 20} }; return list.AsQueryable(); } public Product GetProductById( int productId) { return Products.FirstOrDefault(p => p.ProductId == productId); } public bool AddProduct( Product product) { if (product != null ) { list.Add(product); return true ; } return false ; } public bool UpdateProduct( Product product) { if (product != null ) { if (DeleteProduct(product.ProductId)) { AddProduct(product); return true ; } } return false ; } public bool DeleteProduct( int productId) { var product = GetProductById(productId); if (product != null ) { list.Remove(product); return true ; } return false ; } }
3、添加一个实现了IDependencyResolver接口的Ninject依赖解析器类
public class NinjectDependencyResolverForMvc : IDependencyResolver { private IKernel kernel; public NinjectDependencyResolverForMvc( IKernel kernel) { if (kernel == null ) { throw new ArgumentNullException( "kernel" ); } this .kernel = kernel; } public object GetService( Type serviceType) { return kernel.TryGet(serviceType); } public IEnumerable < object> GetServices( Type serviceType) { return kernel.GetAll(serviceType); } }
4、添加一个NinjectRegister类,用来为MVC和WebApi注册Ninject容器
public class NinjectRegister { private static readonly IKernel Kernel; static NinjectRegister() { Kernel= new StandardKernel (); AddBindings(); } public static void RegisterFovMvc() { DependencyResolver .SetResolver(new NinjectDependencyResolverForMvc (Kernel)); } private static void AddBindings() { Kernel.Bind<IProductRepository >().To< ProductRepository>(); } }
5、在Global.asax文件的Application_Start方法中添加下面代码:
NinjectRegister .RegisterFovMvc(); //为ASP.NET MVC注册IOC容器
6、新建一个名为ProductController的控制器,ProductController的构造函数接受了一个IProductRepository参数,当ProductController 被实例化的时候,Ninject就为其注入了ProductRepository的依赖.
public class ProductController : Controller { private IProductRepository repository; public ProductController(IProductRepository repository) { this .repository = repository; } public ActionResult Index() { return View(repository.Products); } }
7、视图代码用来展示商品列表
@model IQueryable<MvcDemo.WebUI.Models. Product > @{ ViewBag.Title = "MvcDemo Index" ; } @ foreach ( var product in Model) { <div style=" border-bottom :1px dashed silver ;"> < h3> @product.Name </ h3> < p> 价格:@ product.Price </ p > </div > }
8、运行效果如下:

三、在ASP.NET Web Api中使用Ninject
1、添加一个实现了IDependencyResolver接口的Ninject依赖解析器类
namespace MvcDemo.WebUI.AppCode { public class NinjectDependencyResolverForWebApi : NinjectDependencyScope ,IDependencyResolver { private IKernel kernel; public NinjectDependencyResolverForWebApi( IKernel kernel) : base (kernel) { if (kernel == null ) { throw new ArgumentNullException( "kernel" ); } this .kernel = kernel; } public IDependencyScope BeginScope() { return new NinjectDependencyScope(kernel); } } public class NinjectDependencyScope : IDependencyScope { private IResolutionRoot resolver; internal NinjectDependencyScope(IResolutionRoot resolver) { Contract .Assert(resolver != null ); this .resolver = resolver; } public void Dispose() { resolver = null ; } public object GetService( Type serviceType) { return resolver.TryGet(serviceType); } public IEnumerable < object> GetServices( Type serviceType) { return resolver.GetAll(serviceType); } } }
2、在NinjectRegister类中添加注册依赖解析器的方法
public static void RegisterFovWebApi( HttpConfiguration config) { config.DependencyResolver = new NinjectDependencyResolverForWebApi (Kernel); }
3、在Global.asax文件的Application_Start方法中添加下面代码:
NinjectRegister .RegisterFovWebApi( GlobalConfiguration.Configuration); //为WebApi注册IOC容器
4、新建一个名为MyApiController的控制器
public class MyApiController : ApiController { private IProductRepository repository; public MyApiController(IProductRepository repository) { this .repository = repository; } // GET api/MyApi [ HttpGet ] public IEnumerable < Product> Get() { return repository.Products; } // GET api/MyApi/5 [ HttpGet ] public Product Get( int id) { return repository.Products.FirstOrDefault(p => p.ProductId == id); } }
5、视图代码用来展示商品列表
@{ ViewBag.Title = "ApiDemo Index" ; } < script> function GetAll() { $.ajax({ url: "/api/MyApi" , type: "GET" , dataType: "json" , success: function (data) { for (var i = 0; i < data.length; i++) { $( "#list" ).append("<h3>" + data[i].Name + "</h3>"); $( "#list" ).append("<p>价格:" +data[i].Price + "</p>"); } } }); } $( function () { GetAll(); }); </ script> < h2> Api Ninject Demo </h2 > < div id="list" style=" border-bottom :1px dashed silver ;"></ div >
6、运行效果如下:
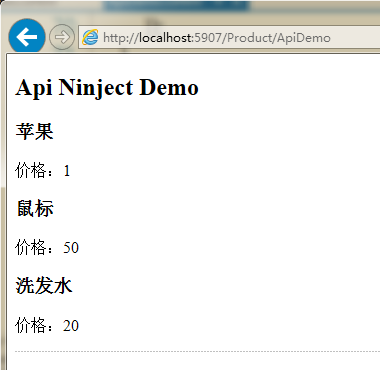
至此,我们就实现了共用一个NinjectRegister类完成了为MVC和Web Api注册Ninject容器