转载:https://blog.csdn.net/cumtb2002/article/details/107798767
Modules used:
使用的模块:
For this, we will use the opencv-python module which provides us various functions to work on images.
为此,我们将使用opencv-python模块,该模块为我们提供了处理图像的各种功能。
Download opencv-python
下载opencv-python
-
General Way:
-
pip install opencv-python
-
-
Pycharm Users:
-
Go to the project Interpreter and install this module from there.
opencv-python Module:
opencv-python模块:
opencv-python is a python library that will solve the Computer Vision Problems and provides us various functions to edit the Images.
opencv-python是一个python库,它将解决计算机视觉问题并为我们提供编辑图像的各种功能。
Note: The edge Detection is possible only in grayscale Image.
注意:只能在灰度图像中进行边缘检测。
What we will do in this script?
我们将在此脚本中做什么?
To detect the edges of the images we will use opencv-python various Functions and Provide thresholds.
为了检测图像的边缘,我们将使用opencv-python的各种功能并提供阈值。
In this article we will detect the edge of the Image with the help of various functions and the accuracy of edge increases as we go down,
在本文中,我们将借助各种功能来检测图像的边缘,并且当我们下降时边缘的精度会提高,
-
Sobel Function: This Function will create the Horizontal and vertical edges and after that, we will use the Bitwise or operator to combine them
Sobel函数 :此函数将创建水平边缘和垂直边缘,然后,我们将使用按位或运算符将它们组合
-
Laplacian Function: This Function is the simplest Function in which we just have to put the Grayscale Variable into it, and we will get the edge detected image.
拉普拉斯函数 :此函数是最简单的函数,只需要将灰度变量放入其中,就可以得到边缘检测到的图像。
-
Canny Function: This is the most powerful function for edge detection and most accurate.
Canny功能 :这是边缘检测功能最强大且最准确的功能。
Let's see the code:
让我们看一下代码:
1)使用Sobel函数 (1) Using Sobel Function)
-
# importing the module
-
import cv2
-
-
# read the image and store the data in a variable
-
image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
-
-
# make it grayscale
-
Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
-
-
# Make it with the help of sobel
-
# make the sobel_horizontal
-
# For horizontal x axis=1 and yaxis=0
-
# for vertical x axis=0 and y axis=1
-
Horizontal=cv2.Sobel(Gray,0,1,0,cv2.CV_64F)
-
-
# the thresholds are like
-
# (variable,0,<x axis>,<y axis>,cv2.CV_64F)
-
Vertical=cv2.Sobel(Gray,0,0,1,cv2.CV_64F)
-
-
# DO the Bitwise operation
-
Bitwise_Or=cv2.bitwise_or(Horizontal,Vertical)
-
-
# Show the Edged Image
-
cv2.imshow("Sobel Image",Bitwise_Or)
-
cv2.imshow("Original Image",Gray)
-
cv2.waitKey(0)
-
cv2.destroyAllWindows()
Output:
输出:
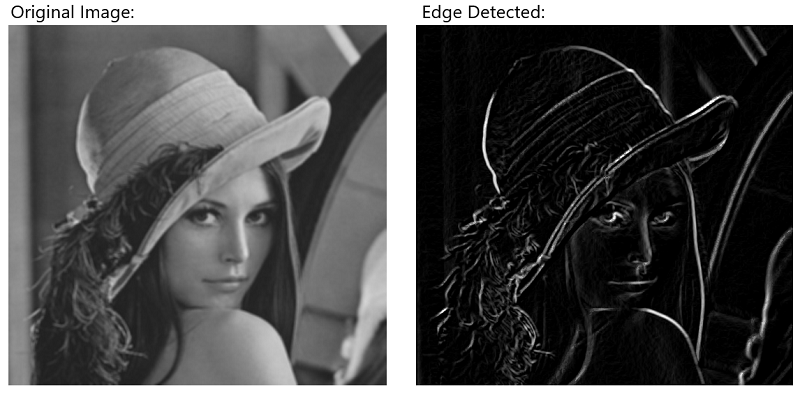
2)拉普拉斯函数 (2) Laplacian Function)
-
# importing the module
-
import cv2
-
-
# read the image and store the data in a variable
-
image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
-
-
# make it grayscale
-
Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
-
-
# Make Laplacian Function
-
Lappy=cv2.Laplacian(Gray,cv2.CV_64F)
-
-
cv2.imshow("Laplacian",Lappy)
-
cv2.imshow("Original",Gray)
-
cv2.waitKey(0)
-
cv2.destroyAllWindows()
Output:
输出:
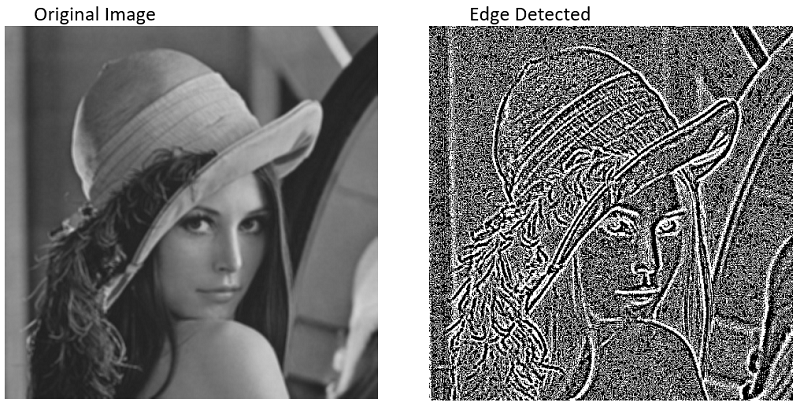
3)使用Canny函数 (3) Using Canny Function)
-
# importing the module
-
import cv2
-
-
# read the image and store the data in a variable
-
image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
-
-
# make it grayscale
-
Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
-
-
# Make canny Function
-
canny=cv2.Canny(Gray,40,140)
-
-
# the threshold is varies bw 0 and 255
-
cv2.imshow("Canny",canny)
-
cv2.imshow("Original",Gray)
-
cv2.waitKey(0)
-
cv2.destroyAllWindows()
Output:
输出:
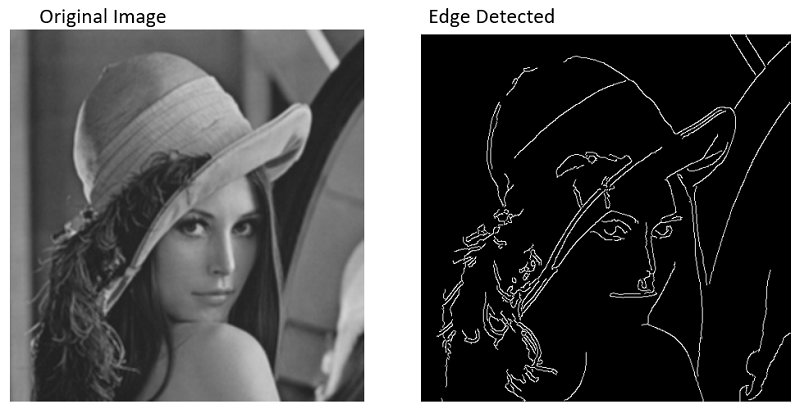
翻译自: https://www.includehelp.com/python/edge-detection-of-image-using-opencv-cv2.aspx