webdriver有哪些鼠标操作方法呢?
可以到Python安装的目录下去查看action_chains.py文件,路径如下(我的python安装在D盘):
D:Python27Libsite-packagesseleniumwebdrivercommonaction_chains.py
或者:
在python shell中help(ActionChains)查看帮助文档
鼠标的操作:
click(self, on_element=None)
| Clicks an element.
|
| :Args:
| - on_element: The element to click.
| If None, clicks on current mouse position.
|
| click_and_hold(self, on_element=None)
| Holds down the left mouse button on an element.
|
| :Args:
| - on_element: The element to mouse down.
| If None, clicks on current mouse position.
|
| context_click(self, on_element=None)
| Performs a context-click (right click) on an element.
|
| :Args:
| - on_element: The element to context-click.
| If None, clicks on current mouse position.
|
| double_click(self, on_element=None)
| Double-clicks an element.
|
| :Args:
| - on_element: The element to double-click.
| If None, clicks on current mouse position.
|
| drag_and_drop(self, source, target)
| Holds down the left mouse button on the source element,
| then moves to the target element and releases the mouse button.
|
| :Args:
| - source: The element to mouse down.
| - target: The element to mouse up.
|
| drag_and_drop_by_offset(self, source, xoffset, yoffset)
| Holds down the left mouse button on the source element,
| then moves to the target offset and releases the mouse button.
|
| :Args:
| - source: The element to mouse down.
| - xoffset: X offset to move to.
| - yoffset: Y offset to move to.
|
| key_down(self, value, element=None)
| Sends a key press only, without releasing it.
| Should only be used with modifier keys (Control, Alt and Shift).
|
| :Args:
| - value: The modifier key to send. Values are defined in `Keys` class.
| - element: The element to send keys.
| If None, sends a key to current focused element.
|
| Example, pressing ctrl+c::
|
| ActionChains(driver).key_down(Keys.CONTROL).send_keys('c').key_up(Keys.CONTROL).perform()
|
| key_up(self, value, element=None)
| Releases a modifier key.
|
| :Args:
| - value: The modifier key to send. Values are defined in Keys class.
| - element: The element to send keys.
| If None, sends a key to current focused element.
|
| Example, pressing ctrl+c::
|
| ActionChains(driver).key_down(Keys.CONTROL).send_keys('c').key_up(Keys.CONTROL).perform()
|
| move_by_offset(self, xoffset, yoffset)
| Moving the mouse to an offset from current mouse position.
|
| :Args:
| - xoffset: X offset to move to, as a positive or negative integer.
| - yoffset: Y offset to move to, as a positive or negative integer.
|
| move_to_element(self, to_element)
| Moving the mouse to the middle of an element.
|
| :Args:
| - to_element: The WebElement to move to.
|
| move_to_element_with_offset(self, to_element, xoffset, yoffset)
| Move the mouse by an offset of the specified element.
| Offsets are relative to the top-left corner of the element.
|
| :Args:
| - to_element: The WebElement to move to.
| - xoffset: X offset to move to.
| - yoffset: Y offset to move to.
|
| perform(self)
| Performs all stored actions.
|
| release(self, on_element=None)
| Releasing a held mouse button on an element.
|
| :Args:
| - on_element: The element to mouse up.
| If None, releases on current mouse position.
|
| send_keys(self, *keys_to_send)
| Sends keys to current focused element.
|
| :Args:
| - keys_to_send: The keys to send. Modifier keys constants can be found in the
| 'Keys' class.
|
| send_keys_to_element(self, element, *keys_to_send)
| Sends keys to an element.
|
| :Args:
| - element: The element to send keys.
| - keys_to_send: The keys to send. Modifier keys constants can be found in the
| 'Keys' class.
|
鼠标操作使用方法:
ActionChains can be used in a chain pattern::
|
| menu = driver.find_element_by_css_selector(".nav")
| hidden_submenu = driver.find_element_by_css_selector(".nav #submenu1")
|
| ActionChains(driver).move_to_element(menu).click(hidden_submenu).perform()
注意:ActionChains(driver).move_to_element(menu).click(hidden_submenu).perform()
看着这个一串操作是不是一脸懵逼,其实可以去看下具体的操作方法,如move_to_element()方法
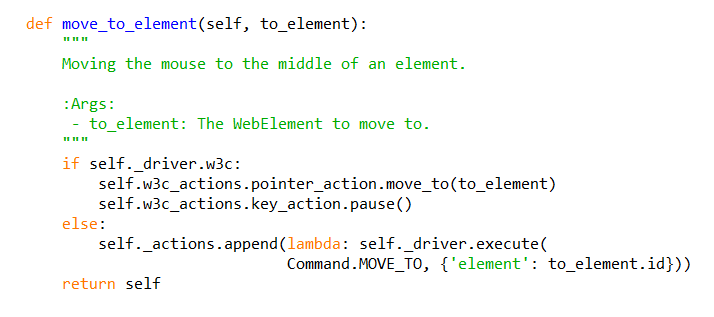
根据这个方法可以看出来,它只是把move_to_element操作存到一个变量中然后返回ActionChains对象本身。
也可以把每个步骤分开写:
|
| actions can be queued up one by one, then performed.::
|
| menu = driver.find_element_by_css_selector(".nav")
| hidden_submenu = driver.find_element_by_css_selector(".nav #submenu1")
|
| actions = ActionChains(driver)
| actions.move_to_element(menu)
| actions.click(hidden_submenu)
| actions.perform()