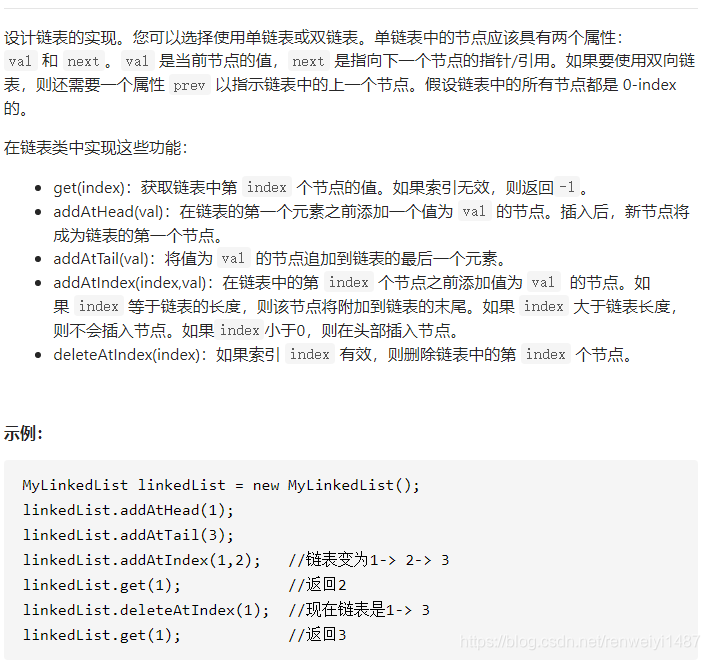
原始链表操作无哑节点
class MyLinkedList {
class Node {
int val;
Node next;
Node (int x) {
val = x;
}
}
private Node first = null;
// 链表长度
private int N = 0;
/** Initialize your data structure here. */
public MyLinkedList() {
}
/** Get the value of the index-th node in the linked list. If the index is invalid, return -1. */
public int get(int index) {
if (index < 0 || index >= N) return -1;
Node cur = first;
while(index-- > 0) {
cur = cur.next;
}
return cur.val;
}
/** Add a node of value val before the first element of the linked list. After the insertion, the new node will be the first node of the linked list. */
public void addAtHead(int val) {
Node lastNode = first;
first = new Node(val);
first.next = lastNode;
N++;
}
/** Append a node of value val to the last element of the linked list. */
public void addAtTail(int val) {
if (first == null) addAtHead(val);
Node cur = first;
while (cur.next != null) {
cur = cur.next;
}
cur.next = new Node(val);
N++;
}
/** Add a node of value val before the index-th node in the linked list. If index equals to the length of linked list, the node will be appended to the end of linked list. If index is greater than the length, the node will not be inserted. */
public void addAtIndex(int index, int val) {
if (index > N) return;
if (index <= 0) {
addAtHead(val);
return;
}
if (index == N) {
addAtTail(val);
return;
}
Node prev = first;
Node cur = first.next;
while (index-- > 1) {
cur = cur.next;
prev = prev.next;
}
Node insert = new Node(val);
prev.next = insert;
insert.next = cur;
N++;
}
/** Delete the index-th node in the linked list, if the index is valid. */
public void deleteAtIndex(int index) {
if (index < 0 || index >= N) return;
if (index == 0) {
first = first.next;
N--;
return;
}
Node prev = first;
Node cur = first.next;
while (index-- > 1) {
cur = cur.next;
prev = prev.next;
}
prev.next = cur.next;
N--;
}
}
/**
* Your MyLinkedList object will be instantiated and called as such:
* MyLinkedList obj = new MyLinkedList();
* int param_1 = obj.get(index);
* obj.addAtHead(val);
* obj.addAtTail(val);
* obj.addAtIndex(index,val);
* obj.deleteAtIndex(index);
*/
使用哑节点
class MyLinkedList {
class Node {
int val;
Node next;
Node (int x) {
val = x;
}
}
private Node dummyHead = null;
// 链表长度
private int N;
/** Initialize your data structure here. */
public MyLinkedList() {
dummyHead = new Node(0);
N = 0;
}
/** Get the value of the index-th node in the linked list. If the index is invalid, return -1. */
public int get(int index) {
if (index < 0 || index >= N) return -1;
Node cur = dummyHead;
while (index-- > 0) {
cur = cur.next;
}
return cur.next.val;
}
/** Add a node of value val before the first element of the linked list. After the insertion, the new node will be the first node of the linked list. */
public void addAtHead(int val) {
Node newNode = new Node(val);
newNode.next = dummyHead.next;
dummyHead.next = newNode;
N++;
}
/** Append a node of value val to the last element of the linked list. */
public void addAtTail(int val) {
Node cur = dummyHead;
while (cur.next != null) {
cur = cur.next;
}
cur.next = new Node(val);
N++;
}
/** Add a node of value val before the index-th node in the linked list. If index equals to the length of linked list, the node will be appended to the end of linked list. If index is greater than the length, the node will not be inserted. */
public void addAtIndex(int index, int val) {
if (index > N) return;
Node cur = dummyHead;
while (index-- > 0) {
cur = cur.next;
}
Node newNode = new Node(val);
newNode.next = cur.next;
cur.next = newNode;
N++;
}
/** Delete the index-th node in the linked list, if the index is valid. */
public void deleteAtIndex(int index) {
if (index < 0 || index >= N) return;
Node cur = dummyHead;
while (index-- > 0) {
cur = cur.next;
}
cur.next = cur.next.next;
N--;
}
}