一、String 常用方法
1 import java.util.Arrays;
2
3 public class StringDemo {
4 public static void main(String[] args) {
5 String str = "String 方法";
6 // 判断字符串是否为空
7 str.isEmpty();
8 // 获取字符串长度
9 str.length();
10 // 删掉开头和结尾的空格
11 str.trim();
12
13 // 在字符串中 0 到结尾 查找第一个找到的字符或字符串索引位置,没有返回 -1
14 // public int indexOf(String str)
15 // public int indexOf(int ch)
16 int index = str.indexOf("ing"); // 3
17 index = str.indexOf('i'); // 3
18 // fromIndex 从这个索引(包含)进行位置寻找 ,也就是 fromIndex 到 结尾
19 // public int indexOf(int ch, fromIndex)
20 // public int indexOf(String ch, fromIndex)
21 index = str.indexOf('i', 3); // 3
22 // lastIndexOf 与 indexOf 方法类似,从后面向 索引 0 查找
23 index = str.lastIndexOf('i');
24
25 // 字符串中是否包含指定的字符序列,String 就是实现了接口 CharSequence
26 // public boolean contains(CharSequence s)
27 // 内部是实现: return indexOf(s.toString()) > -1;
28 boolean ct = str.contains("ing"); // true
29
30 // 判断字符串是否以给定子字符串开头
31 // public boolean startsWith(String prefix)
32 boolean st = str.startsWith("Str");// true
33 // public boolean startsWith(String prefix, int toffset)
34 // toffset 表示开始的位置
35 st = str.startsWith("Str", 2); // false
36 // 判断字符串是否以给定子字符串结尾
37 // public boolean endsWith(String suffix)
38 boolean ed = str.endsWith("法"); // true
39
40 // 与其他字符串比较,看内容是否相同
41 // public boolean equals(Object anObject)
42
43 // 忽略大小写,与其他字符串进行比较,看内容是否相同
44 // public boolean equalsIgnoreCase(String anotherString)
45 "abc".equals("Abc"); // false
46 "abc".equalsIgnoreCase("ABc"); // true
47
48 // 转大小写,返回新字符串,原字符串不变
49 str.toLowerCase(); // 小写
50 str.toUpperCase(); // 大写
51
52 // 字符串比较大小,按照编码值
53 // public int compareTo(String anotherString)
54 "abc".compareTo("Abc"); // => return 'a' - 'A' 字符逐一进行比较
55 // 忽略字母大小写进行比较 (都转大写再比较)
56 // public int compareToIgnoreCase(String str)
57
58 // 截取字符串返回新的字符串
59 // public String substring(int beginIndex)
60 // public String substring(int beginIndex, int endIndex)
61 // beginIndex 开始的索引 endIndex 结束的索引
62 // beginIndex 包括在截取的范围内,而endIndex不包括
63 String sub = str.substring(1); // tring 方法
64 String sube = str.substring(1, 2); // t
65
66 // 字符串连接,返回当前字符串和参数字符串合并后的字符串,原字符串不变
67 // public String concat(String str)
68 String cc = str.concat(" --> concat 连接方法");
69 // String 方法 --> concat 连接方法
70
71 // 字符串替换 ,返回新字符串,原字符串不变
72 // 替换单个字符
73 // public String replace(char oldChar, char newChar)
74 String rp = str.replace('方', '圆');// String 圆法
75 // 替换字符序列
76 // public String replace(CharSequence target, CharSequence replacement)
77 rp = str.replace("String", "replace"); // replace 方法
78 // 利用正则选择需要替换的字符
79 // regex --> 正则表达式的字符串
80 // replacement 替换的元素
81 // public String replaceAll(String regex, String replacement)
82 rp = str.replaceAll("[a-z]", "i"); // Siiiii 方法
83 // 表示:将匹配到每个小写字母,变为 i
84 // replaceFirst 改变第一次符合要求的字符串
85 rp = str.replaceFirst("[a-z]", "i");
86
87 // 分隔字符串,返回分隔后的子字符串数组,原字符串不变
88 // regex 根据字符串分隔,匹配到的字符串将被移除
89 // public String[] split(String regex)
90 String[] strs = "HELLO WORLD THIS NIGHT".split(" ");
91 System.out.println(Arrays.asList(strs));
92 // [HELLO, WORLD, THIS, NIGHT]
93 strs = "HELLO WORLD THIS NIGHT".split("H");
94 System.out.println(Arrays.asList(strs));
95 // [, ELLO WORLD T, IS NIG, T]
96
97 // limit 限制分隔的次数(limit - 1)
98 // 也可以理解匹配到 regex 的次数为 limit - 1
99 // 如果限制的次数(limit - 1)大于实际分隔的次数
100 // 或者 limit - 1 < 0
101 // 那就按正常分隔无限制。
102 // "HELLO WORLD THIS NIGHT".split(" ") 这样的分隔
103 // public String[] split(String regex, String limit);
104 for (int i = -1; i < 6; i++) {
105 strs = "HELLO WORLD THIS NIGHT".split(" ", i);
106 System.out.println(Arrays.asList(strs));
107 }
108 /**
109 * 实际可以分隔3次,可得到数组长度为4
110 *
111 * -1 [HELLO, WORLD, THIS, NIGHT]
112 * 0 [HELLO, WORLD, THIS, NIGHT]
113 * 1 [HELLO WORLD THIS NIGHT]
114 * 2 [HELLO, WORLD THIS NIGHT]
115 * 3 [HELLO, WORLD, THIS NIGHT]
116 * 4 [HELLO, WORLD, THIS, NIGHT]
117 * 5 [HELLO, WORLD, THIS, NIGHT]
118 */
119 }
120
121 }
二、String 的构造方法
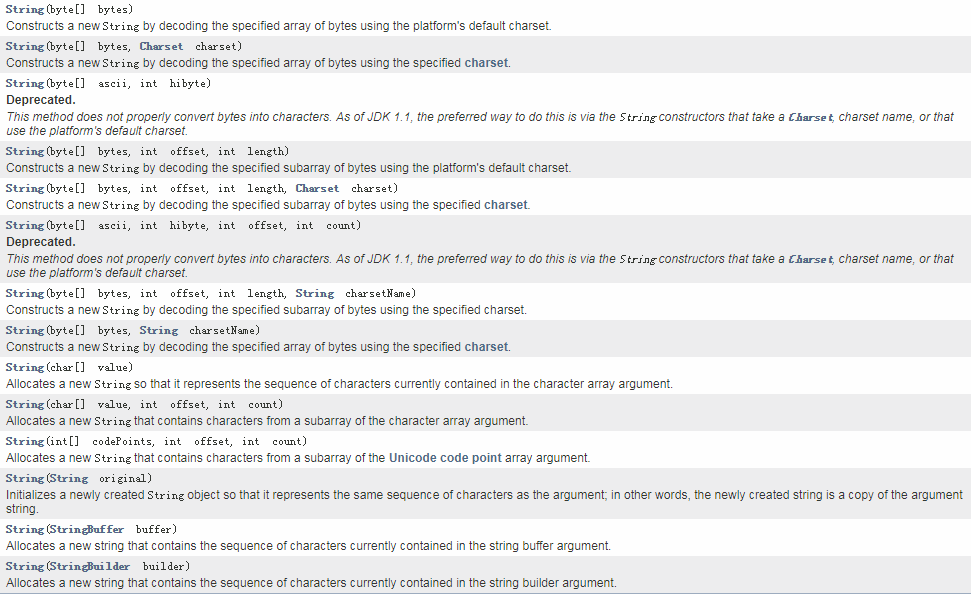