"QAQ" is a word to denote an expression of crying. Imagine "Q" as eyes with tears and "A" as a mouth.
Now Diamond has given Bort a string consisting of only uppercase English letters of length n. There is a great number of "QAQ" in the string (Diamond is so cute!).
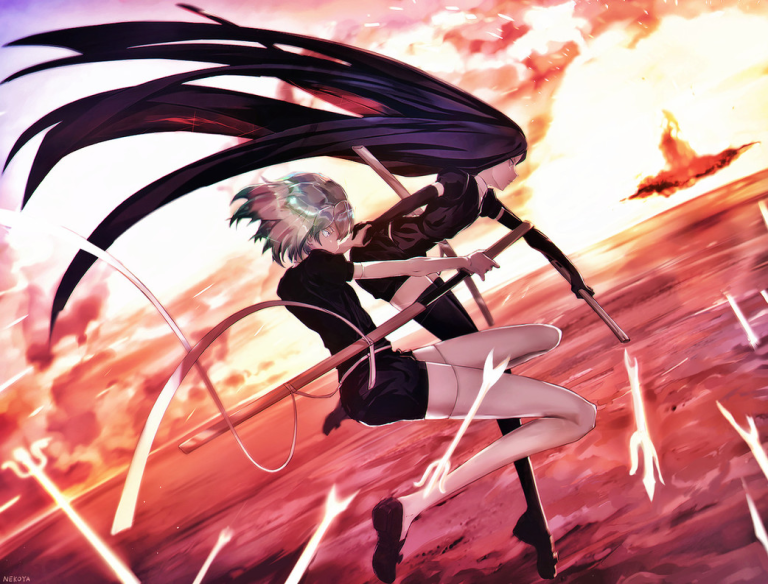
Bort wants to know how many subsequences "QAQ" are in the string Diamond has given. Note that the letters "QAQ" don't have to be consecutive, but the order of letters should be exact.
The only line contains a string of length n (1 ≤ n ≤ 100). It's guaranteed that the string only contains uppercase English letters.
Print a single integer — the number of subsequences "QAQ" in the string.
QAQAQYSYIOIWIN
4
QAQQQZZYNOIWIN
3
In the first example there are 4 subsequences "QAQ": "QAQAQYSYIOIWIN", "QAQAQYSYIOIWIN", "QAQAQYSYIOIWIN", "QAQAQYSYIOIWIN".
记录每个A的位置,左边Q,和右边Q的个数。可以到O(n),就没写了。

#include <bits/stdc++.h> using namespace std; char str[105]; int main() { scanf("%s",str); int len = strlen(str); int ans = 0; for(int i = 0; i < len; i++) { if(str[i]!='A') continue; int cnt1 = 0,cnt2 = 0; for(int j = 0; j < i; j++) { if(str[j]=='Q') cnt1++; } for(int j = i; j < len; j++) { if(str[j]=='Q') cnt2 ++; } ans = ans + (cnt1*cnt2); } printf("%d ",ans); return 0; }
Ralph has a magic field which is divided into n × m blocks. That is to say, there are n rows and m columns on the field. Ralph can put an integer in each block. However, the magic field doesn't always work properly. It works only if the product of integers in each row and each column equals to k, where k is either 1 or -1.
Now Ralph wants you to figure out the number of ways to put numbers in each block in such a way that the magic field works properly. Two ways are considered different if and only if there exists at least one block where the numbers in the first way and in the second way are different. You are asked to output the answer modulo 1000000007 = 109 + 7.
Note that there is no range of the numbers to put in the blocks, but we can prove that the answer is not infinity.
The only line contains three integers n, m and k (1 ≤ n, m ≤ 1018, k is either 1 or -1).
Print a single number denoting the answer modulo 1000000007.
1 1 -1
1
1 3 1
1
3 3 -1
16
In the first example the only way is to put -1 into the only block.
In the second example the only way is to put 1 into every block.
题意:n*m的格子里面填数,使得每一行,每一列的乘积等于k(1,-1)的方案数。
当n,m一奇一偶,k==-1时,没有答案。
然后减掉一行,减掉一列,中间随便填,最后一行,最后一列来定乘积。
#include <bits/stdc++.h> using namespace std; typedef long long ll; const ll MOD = 1000000007; ll pow_mod(ll a,ll n) { if(n==0) return 1; ll x = pow_mod(a,n/2); ll ans = x*x%MOD; if(n%2==1) ans = ans*a%MOD; return ans; } int main() { ll n,m; int k; cin>>n>>m>>k; if(n%2!=m%2&&k==-1) puts("0"); else { n--;m--; cout<< pow_mod(pow_mod(2,n),m)<<endl; } return 0; }
In a dream Marco met an elderly man with a pair of black glasses. The man told him the key to immortality and then disappeared with the wind of time.
When he woke up, he only remembered that the key was a sequence of positive integers of some length n, but forgot the exact sequence. Let the elements of the sequence be a1, a2, ..., an. He remembered that he calculated gcd(ai, ai + 1, ..., aj) for every 1 ≤ i ≤ j ≤ n and put it into a set S. gcd here means the greatest common divisor.
Note that even if a number is put into the set S twice or more, it only appears once in the set.
Now Marco gives you the set S and asks you to help him figure out the initial sequence. If there are many solutions, print any of them. It is also possible that there are no sequences that produce the set S, in this case print -1.
The first line contains a single integer m (1 ≤ m ≤ 1000) — the size of the set S.
The second line contains m integers s1, s2, ..., sm (1 ≤ si ≤ 106) — the elements of the set S. It's guaranteed that the elements of the set are given in strictly increasing order, that means s1 < s2 < ... < sm.
If there is no solution, print a single line containing -1.
Otherwise, in the first line print a single integer n denoting the length of the sequence, n should not exceed 4000.
In the second line print n integers a1, a2, ..., an (1 ≤ ai ≤ 106) — the sequence.
We can show that if a solution exists, then there is a solution with n not exceeding 4000 and ai not exceeding 106.
If there are multiple solutions, print any of them.
4
2 4 6 12
3
4 6 12
2
2 3
-1
In the first example 2 = gcd(4, 6), the other elements from the set appear in the sequence, and we can show that there are no values different from 2, 4, 6 and 12 among gcd(ai, ai + 1, ..., aj) for every 1 ≤ i ≤ j ≤ n.
题意:
一个序列a,他的任意子序列的GCD构成一个集合,现在要用这个集合还原出那个序列。
首先集合中最小的元素,一定是全局gcd,那么它一定能被所有元素整除。
然后,防止序列产生新的GCD,把这个GCD插入到所有空隙中去。
#include <bits/stdc++.h> using namespace std; const int MAXN = 1005; int a[MAXN]; int main() { int n; scanf("%d",&n); for(int i = 0; i < n; i++) { scanf("%d",&a[i]); } int gcd = a[0]; bool flag = true; for(int i =1 ; i < n; i++) { if(a[i]%gcd!=0) { flag = false; break; } } if(flag==false) puts("-1"); else { vector<int> ans; ans.push_back(gcd); for(int i = 1; i < n; i++) { ans.push_back(a[i]); ans.push_back(gcd); } printf("%d ",ans.size()); for(int i = 0; i < (int)ans.size(); i++) printf("%d ",ans[i]); puts(""); } return 0; }
嗯,CF还是得打,脑子有点僵化~~~