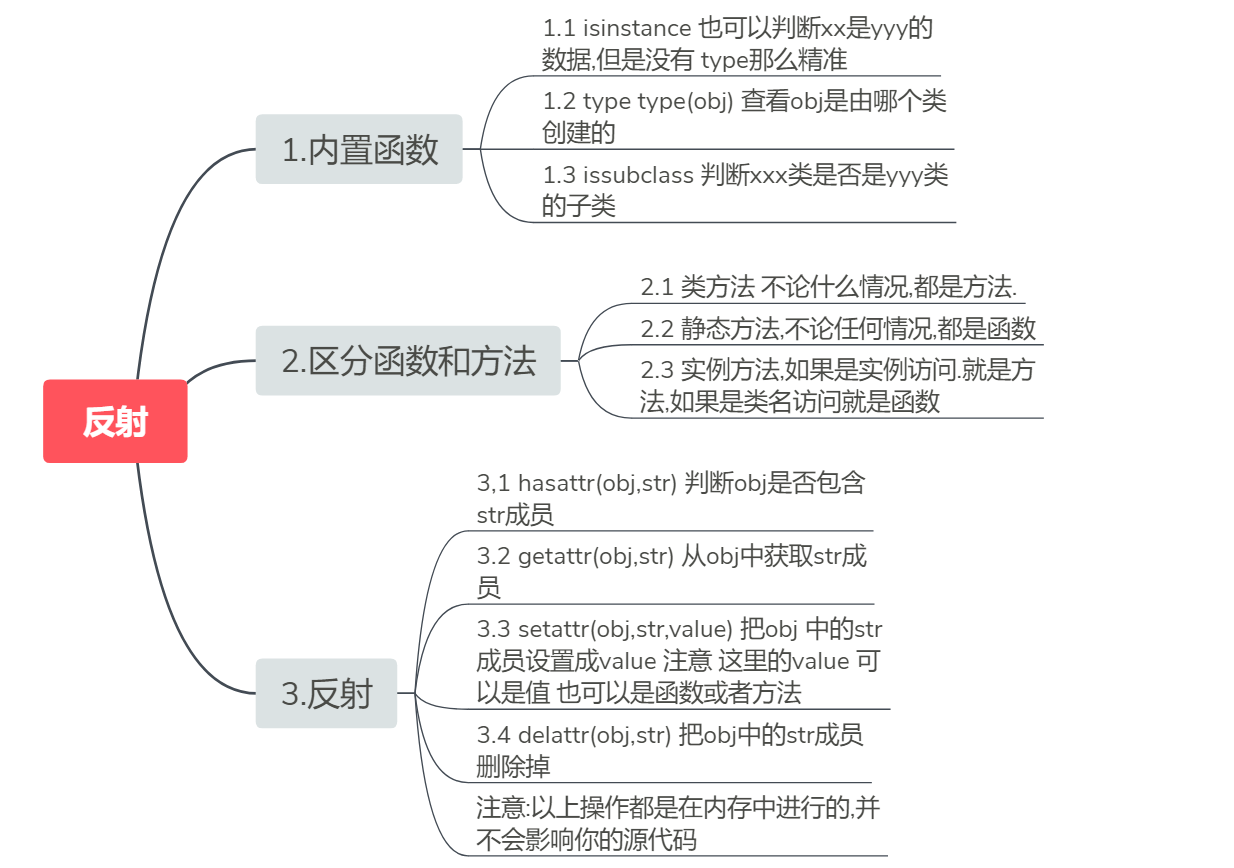
# class Foo(object):
# pass
#
# class Bar(Foo):
# pass
#
# class FooBar(Bar):
# pass
# print(issubclass(Bar, Foo)) # True
# print(issubclass(Foo, Bar)) # False
# print(issubclass(FooBar, Foo)) # True 可以隔代判断
# print(issubclass(Foo, object))
# print(issubclass(Bar, object))
# print(issubclass(FooBar, object))
# object是所有类的根. 面向对象的祖宗
# print(type("你好")) # <class 'str'> 返回该对象的数据类型
# class Animal:
# pass
#
# class Cat(Animal):
# pass
#
# c = Cat()
#
# print(type(c)) # 可以精准的返回数据类型
# 计算a+b的结果 数学运算
# def cul(a, b):
# if (type(a) == int or type(a) == float) and (type(b) == int or type(b) == float):
# return a + b
# else:
# print("不行. 不能帮你计算")
#
# print(cul(10, "胡辣汤"))
# isinstance 判断xxx对象是否是xxx类型的
# class Animal:
# pass
#
# class Cat(Animal): # x是一种y. x继承y
# pass
#
# class BosiCat(Cat):
# pass
#
# kitty = Cat()
# print(isinstance(kitty, BosiCat)) # True xxx是否是一种xxxx(包括对象的父类)
# # type()
# print(type(kitty) == Animal) # False
# # 迭代器
# from collections import Iterator
#python 3.7+ 需要 import collections.abc
# lst = []
# it = lst.__iter__() # list_iterator
# print(isinstance(it, Iterator)) # True
# def chi():
# pass
#
# print(chi) # <function chi at 0x00000220146A8C80>
#
# class Person:
#
# def chi(self):
# pass
#
# @staticmethod
# def he(): # 静态方法
# pass
#
# p = Person()
# print(p.chi) # <bound method Person.chi of <__main__.Person object at 0x0000021252D97240>>
#
# # 通过打印可以看到是方法还是函数
# print(Person.he) # <function Person.he at 0x0000019F4E9A8D08>
#
# 查看类中的方法和函数
# class Car:
# def run(self): # 实例方法
# print("我是车, 我会跑")
#
# @staticmethod
# def cul():
# print("我会计算")
#
# @classmethod
# def jump(cls):
# print("我会jump")
# 实例方法 <bound method Car.run of <__main__.Car object at 0x000001E9166B73C8>>
# c = Car()
# 1
# "str"
# print(c.run) # <bound method Car.run of <__main__.Car object at 0x000001E9166B73C8>>
# Car.run(c) # 通过类名也可以访问实例方法. 不要这么干
# print(Car.run) # <function Car.run at 0x000002454C748AE8>
# 实例方法:
# 1. 用对象.方法 方法
# 2. 类名.方法 函数
# 静态方法
# 都是函数
# print(c.cul) # <function Car.cul at 0x0000024BA2658BF8>
# print(Car.cul) # <function Car.cul at 0x0000024BA2658BF8>
# 类方法都是方法
# print(c.jump) # <bound method Car.jump of <class '__main__.Car'>>
# print(Car.jump) # <bound method Car.jump of <class '__main__.Car'>>
# 对象.方法
# 万事万物皆为对象
# 我们写的类. 也是对象. 可以创建对象
# class Pereson: # type
# pass
from types import FunctionType, MethodType
class Car:
def run(self): # 实例方法
print("我是车, 我会跑")
@staticmethod
def cul():
print("我会计算")
@classmethod
def jump(cls):
print("我会jump")
# 实例方法:
# 1. 用对象.方法 方法
# 2. 类名.方法 函数
c = Car()
# print(isinstance(c.run, FunctionType)) # False
# print(isinstance(Car.run, FunctionType)) # True
# print(isinstance(c.run, MethodType)) # True
# print(isinstance(Car.run, MethodType)) # False
# 静态方法 都是函数
# print(isinstance(c.cul, FunctionType)) # True
# print(isinstance(Car.cul, FunctionType)) # True
# print(isinstance(c.cul, MethodType)) # False
# print(isinstance(Car.cul, MethodType)) # False
# 类方法都是方法
print(isinstance(c.jump, FunctionType)) # False
print(isinstance(Car.jump, FunctionType)) # False
print(isinstance(c.jump, MethodType)) # True
print(isinstance(Car.jump, MethodType)) # True
# FunctionType:函数
# MethodType: 方法
# md5特点: 不可逆的一种加密方式
# 最多用在密码加密上
# cs alex - 534b44a19bf18d20b71ecc4eb77c572f
import hashlib
SALT = b"abcdefghijklmnjklsfdafjklsdjfklsjdak"
#
# 创建md5的对象
obj = hashlib.md5(SALT) # 加盐
# 给obj设置铭文
obj.update("alex".encode("utf-8"))
# 获取到密文
miwen = obj.hexdigest()
# f4c17d1de5723a61286172fd4df5cb83
# 534b44a19bf18d20b71ecc4eb77c572f
print(miwen) # 534b44a19bf18d20b71ecc4eb77c572f
# md5使用
def jiami(content):
obj = hashlib.md5(SALT)
obj.update(content.encode("utf-8"))
return obj.hexdigest()
# 注册
# username = input("请输入你的用户名:") # alex
# password = input("请输入你的密码:")
# password = jiami(password) # c3d4fe3dce88533a8b50cf2e9387c66d
# print(password)
uname = "alex"
upwd = "c3d4fe3dce88533a8b50cf2e9387c66d"
username = input("请输入你的用户名:")
password = input("请输入你的密码:")
if uname == username and upwd == jiami(password):
print("登录成功")
else:
print("失败")
def chi():
print("大牛很能吃")
def he():
print("大牛很能喝")
def la():
print("大牛很能啦")
def shui():
print("大牛一般不睡觉")
def sa():
print("大牛忘了撒")
def play():
print("大牛很喜欢玩儿")
play()
name = "张二蛋"
print("wife")
# import master
# print(getattr(master, "name"))
# setattr(master, "name", "张全蛋")
# print(master.name)
# setattr(master, "wife", "毛蛋")
# print(master.wife)
# def chi():
# print("大牛说的不对. 应该慢慢吃")
#
# # 给xxx模块中的chi替换成我的chi. 和字典一样
# setattr(master, "chi", chi)
#
# delattr(master, "la") # 动态的删除一些内容
#
# while 1:
# s = input("请输入你要测试的功能") # chi he la sa
#
# # 从模块中获取到chi
# if hasattr(master, s): # name 判断xxx中是否包含xxx功能
# fn = getattr(master, s) # 从xxx中获取到xxx功能
# fn()
# else:
# print("没有这个功能")
# setattr(master, "chi", "馒头")
# print(master.chi)
# setattr(对象, 属性(字符串形式), 值) attribute 属性 对象中的属性
# getattr(对象, 属性(字符串形式) )
# delattr(对象, 属性) 从对象中删除一个属性
# hasattr(对象, 属性) 判断对象中会否有xxx属性
# getattr(master, "name")
# getattr(master, "chi")
# class Car:
# pass
# c = Car()
# print(c.color)
# class Car:
# def __init__(self, color, pai, price):
# self.color = color
# self.pai = pai
# self.price = price
#
# def fly(self):
# print("我的车会飞")
#
# c = Car("黄色", "兰博基尼", 188888)
# # delattr(Car, "fly") # 可以操纵我们的类或者对象
# # c.fly()
#
# # setattr(Car, "fly", lambda self:print("我的天啊. 我的车居然会飞"))
# # c.fly()
#
# print(c.color)
# setattr(c, 'color', "黑色")
# print(c.color)
#
# print(getattr(c, "pai"))
# print(c.pai)