前些时间在园子里看到有位朋友写也了一个DropDownTreeList控件,说实话写的很是不错。今天也贴出我在项目中写的DropDownTreeList控件,以此来和园子里喜欢控件开发的朋友共同进步。
是不是有朋友在说:“少废话了,代码贴出来看看先”。好,那就贴出代码先:
1
/// <summary>
2
/// 下拉树形框控件。
3
/// </summary>
4
[DefaultProperty("Text"), ToolboxData("<{0}:DropDownTreeList runat=server></{0}:DropDownTreeList>")]
5
public class DropDownTreeList : WebControl, IPostBackDataHandler, IPostBackEventHandler
6
{
7
8
/// <summary>
9
/// 下拉列表框控件变量
10
/// </summary>
11
public System.Web.UI.WebControls.DropDownList TypeID = new System.Web.UI.WebControls.DropDownList();
12
13
14
/// <summary>
15
/// 构造函数
16
/// </summary>
17
public DropDownTreeList(): base()
18
{
19
this.BorderStyle = BorderStyle.Groove;
20
this.BorderWidth = 1;
21
}
22
23
/// <summary>
24
/// 构造函数
25
/// </summary>
26
/// <param name="dt"></param>
27
public DropDownTreeList(DataTable dt): this()
28
{
29
BuildTree(dt);
30
}
31
32
/// <summary>
33
/// 构造函数
34
/// </summary>
35
/// <param name="selectid">选择项</param>
36
/// <param name="dt"></param>
37
public DropDownTreeList(string selectid,DataTable dt): this(dt)
38
{
39
this.TypeID.SelectedValue = selectid;
40
}
41
42
/// <summary>
43
/// 创建树
44
/// </summary>
45
/// <param name="dt"></param>
46
public void BuildTree(DataTable dt)
47
{
48
if(this.ParentFiledName.Trim()=="")
49
{
50
throw new Exception("父字段名称不能为空");
51
}
52
if (this.NodeTextField.Trim() == "")
53
{
54
throw new Exception("节点文本字段名称不能为空");
55
}
56
if(this.NodeValueField.Trim()=="")
57
{
58
throw new Exception("节点值字段名称不能为空");
59
}
60
string SelectedType = "0";
61
62
TypeID.SelectedValue = SelectedType;
63
this.Controls.Add(TypeID);
64
TypeID.Items.Clear();
65
//加载树
66
TypeID.Items.Add(new ListItem("请选择 ", "0"));
67
DataRow[] drs = dt.Select(this.ParentFiledName + "=0");
68
foreach (DataRow r in drs)
69
{
70
TypeID.Items.Add(new ListItem(r[NodeTextField].ToString(), r[NodeValueField].ToString()));
71
string blank=StyleDropTree.DropDownTreeList_Style(DropTreeStyle);
72
BindNode(r[NodeValueField].ToString(), dt, blank);
73
}
74
75
TypeID.DataBind();
76
}
77
78
private string _nodeValueField="";
79
/// <summary>
80
/// 节点值字段
81
/// </summary>
82
[Bindable(true), Browsable(true), Category("数据"), DefaultValue("")]
83
public string NodeValueField
84
{
85
get
86
{
87
try
88
{
89
return _nodeValueField;
90
}
91
catch
92
{
93
throw new Exception();
94
}
95
96
}
97
set
98
{
99
this._nodeValueField = value;
100
}
101
}
102
103
private string _nodeTextField="";
104
/// <summary>
105
/// 节点文本字段
106
/// </summary>
107
[Bindable(true), Browsable(true), Category("数据"), DefaultValue("")]
108
public string NodeTextField
109
{
110
get
111
{
112
try
113
{
114
return _nodeTextField;
115
}
116
catch
117
{
118
throw new Exception();
119
}
120
121
}
122
set
123
{
124
this._nodeTextField = value;
125
}
126
}
127
128
/// <summary>
129
/// 创建树
130
/// </summary>
131
/// <param name="sqlstring">查询字符串</param>
132
/// <param name="selectid">选取项</param>
133
public void BuildTree(string selectid,DataTable dt)
134
{
135
BuildTree(dt);
136
this.TypeID.SelectedValue = selectid;
137
}
138
139
/// <summary>
140
/// 创建树结点
141
/// </summary>
142
/// <param name="sonparentid">当前数据项</param>
143
/// <param name="dt">数据表</param>
144
/// <param name="blank">空白符</param>
145
private void BindNode(string sonparentid, DataTable dt, string blank)
146
{
147
DataRow[] drs = dt.Select(this.ParentFiledName + "=" + sonparentid);
148
149
foreach (DataRow r in drs)
150
{
151
string nodevalue = r[NodeValueField].ToString();
152
string text = r[NodeTextField].ToString();
153
if (StyleDropTree.DropDownTreeList_Style(DropTreeStyle) == "├─『")
154
{
155
text = blank + "" + text + "』";
156
}
157
else
158
{
159
text = blank + "" + text + "";
160
}
161
TypeID.Items.Add(new ListItem(text, nodevalue));
162
string blankNode = HttpUtility.HtmlDecode(" ") + blank;
163
BindNode(nodevalue, dt, blankNode);
164
}
165
}
166
167
168
/// <summary>
169
/// 当前选择项的值
170
/// </summary>
171
[Bindable(false), Browsable(false), Category("数据"), DefaultValue("")]
172
public string SelectedValue
173
{
174
get
175
{
176
return this.TypeID.SelectedValue;
177
}
178
179
set
180
{
181
this.TypeID.SelectedValue = value;
182
}
183
}
184
185
/// <summary>
186
/// 当前选择项的文本
187
/// </summary>
188
[Bindable(false), Browsable(false), Category("数据"), DefaultValue("")]
189
public string SelectedText
190
{
191
get
192
{
193
try
194
{
195
string NodeText = this.TypeID.SelectedItem.Text.Trim();
196
int i = 0;
197
if (StyleDropTree.DropDownTreeList_Style(DropTreeStyle) == "├─『")
198
{
199
i = NodeText.IndexOf("『");
200
return NodeText.Substring(i + 1, NodeText.Length - i - 2);
201
}
202
else
203
{
204
return NodeText.Trim();
205
}
206
207
}
208
catch
209
{
210
return "";
211
}
212
213
}
214
set
215
{
216
this.TypeID.SelectedItem.Text=value;
217
}
218
}
219
/// <summary>
220
/// 父字段名称
221
/// </summary>
222
private string m_parentid = "";
223
[Bindable(true), Category("数据"), DefaultValue("")]
224
public string ParentFiledName
225
{
226
get
227
{
228
return m_parentid;
229
}
230
231
set
232
{
233
m_parentid = value;
234
}
235
}
236
237
/// <summary>
238
/// 当某选项被选中后,获取焦点的控件ID(如提交按钮等)
239
/// </summary>
240
[Bindable(true), Category("数据"), DefaultValue("")]
241
public string SetFocusButtonID
242
{
243
get
244
{
245
object o = ViewState[this.ClientID + "_SetFocusButtonID"];
246
return (o == null) ? "" : o.ToString();
247
}
248
set
249
{
250
ViewState[this.ClientID + "_SetFocusButtonID"] = value;
251
if (value != "")
252
{
253
this.TypeID.Attributes.Add("onChange", "document.getElementById('" + value + "').focus();");
254
}
255
}
256
}
257
258
/// <summary>
259
/// 获取或设置数据源
260
/// </summary>
261
private object _dataSource;
262
[Bindable(true),Category("Data"),DefaultValue(null),Description("数据"),DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)]
263
public virtual object DataSource
264
{
265
get
266
{
267
return _dataSource;
268
}
269
set
270
{
271
if ((value == null) || (value is IListSource) || (value is IEnumerable))
272
{
273
_dataSource = value;
274
}
275
else
276
{
277
throw new ArgumentException();
278
}
279
}
280
}
281
282
public override void DataBind()
283
{
284
base.OnDataBinding(EventArgs.Empty);
285
DataTable table = (DataTable)this.DataSource;
286
BuildTree(table);
287
}
288
289
290
private StyleLook _styleLook;
291
/// <summary>
292
/// 设置控件的样式
293
/// </summary>
294
[Bindable(true), Category("样式"), DefaultValue("")]
295
public StyleLook DropTreeStyle
296
{
297
298
set { _styleLook = value; }
299
get { return _styleLook; }
300
}
301
/// <summary>
302
/// 输出html,在浏览器中显示控件
303
/// </summary>
304
/// <param name="output"> 要写出到的 HTML 编写器 </param>
305
protected override void Render(HtmlTextWriter output)
306
{
307
if (this.HintInfo != "")
308
{
309
output.WriteBeginTag("span id=\"" + this.ClientID + "\" onmouseover=\"showhintinfo(this," + this.HintLeftOffSet + "," + this.HintTopOffSet + ",'" + this.HintTitle + "','" + this.HintInfo + "','" + this.HintHeight + "','" + this.HintShowType + "');\" onmouseout=\"hidehintinfo();\">");
310
}
311
312
RenderChildren(output);
313
314
if (this.HintInfo != "")
315
{
316
output.WriteEndTag("span");
317
}
318
}
319
320
321
322
IPostBackDataHandler 成员
“连个效果图没有,就让人看你那烂代码。来个图先?”,有朋友又在喊了。
2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185

186

187

188

189

190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

208

209

210

211

212

213

214

215

216

217

218

219

220

221

222

223

224

225

226

227

228

229

230

231

232

233

234

235

236

237

238

239

240

241

242

243

244

245

246

247

248

249

250

251

252

253

254

255

256

257

258

259

260

261

262

263

264

265

266

267

268

269

270

271

272

273

274

275

276

277

278

279

280

281

282

283

284

285

286

287

288

289

290

291

292

293

294

295

296

297

298

299

300

301

302

303

304

305

306

307

308

309

310

311

312

313

314

315

316

317

318

319

320

321

322

好的,图来了。效果1:
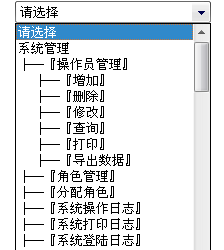
好的,图来了。效果2:

使用方法:
string SQl = "select * from SYS_T_Menu";
DataTable dt = SqlHelper.ReturnDataTable(SQl);
DropDownTreeList1.NodeTextField = "Menu_Name";
DropDownTreeList1.NodeValueField = "Menu_ID";
DropDownTreeList1.ParentFiledName = "Menu_Father_ID";
DropDownTreeList1.DataSource = dt;
DropDownTreeList1.DataBind();