Given a non-empty 2D array grid
of 0's and 1's, an island is a group of 1
's (representing land) connected 4-directionally (horizontal or vertical.) You may assume all four edges of the grid are surrounded by water.
Find the maximum area of an island in the given 2D array. (If there is no island, the maximum area is 0.)
Example 1:
[[0,0,1,0,0,0,0,1,0,0,0,0,0], [0,0,0,0,0,0,0,1,1,1,0,0,0], [0,1,1,0,1,0,0,0,0,0,0,0,0], [0,1,0,0,1,1,0,0,1,0,1,0,0], [0,1,0,0,1,1,0,0,1,1,1,0,0], [0,0,0,0,0,0,0,0,0,0,1,0,0], [0,0,0,0,0,0,0,1,1,1,0,0,0], [0,0,0,0,0,0,0,1,1,0,0,0,0]]Given the above grid, return
6
. Note the answer is not 11, because the island must be connected 4-directionally.
Example 2:
[[0,0,0,0,0,0,0,0]]Given the above grid, return
0
.
Note: The length of each dimension in the given grid
does not exceed 50.
分析:题目翻译一下:要求计算连续1的个数。看题目也是一个DFS问题,当遇到一个1,然后就从上、下、左、又四个方向去搜索。这里要注意搜索边界,如果在边界地方还要搜索,有可能数组越界。
比如
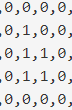
现在加入搜索到第二行第二列的1,然后向上是0,向下是1,再往下还是1,再往下是0。这里要注意如果是1的话,要用一个visited数组来保存访问记录。可以降低重复访问次数。
代码如下:
1 public class maxareaofisland { 2 int maxarea = 0; 3 public int maxAreaOfIsland(int[][] grid) { 4 boolean[][] visited = new boolean[grid.length][grid[0].length]; 5 for ( int i = 0 ; i < grid.length ; i ++ ){ 6 for ( int j = 0 ; j < grid[i].length ; j ++ ){ 7 int temparea = helper(i,j,grid,visited); 8 maxarea = Math.max(maxarea,temparea); 9 } 10 } 11 return maxarea; 12 } 13 14 private int helper(int i, int j, int[][] grid, boolean[][] visited) { 15 if ( i < 0 || j < 0 || i > grid.length - 1 || j > grid.length - 1) return 0; 16 if ( grid[i][j] == 0 || visited[i][j]) return 0; 17 visited[i][j] = true; 18 return 1 + helper(i+1,j,grid,visited)+helper(i-1,j,grid,visited)+helper(i,j+1,grid,visited)+helper(i,j-1,grid,visited); 19 } 20 }
运行时间23ms,击败36.30%的提交。
看了一下其他的方法,基本都是深搜。只是一些技巧问题,就不管了。