1.效果图
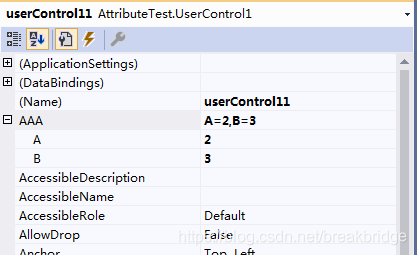
2.对象和控件
using System.ComponentModel;
using System.Drawing;
using System.Windows.Forms;
namespace AttributeTest
{
public partial class UserControl1 : UserControl
{
public UserControl1()
{
InitializeComponent();
this.BackColor = Color.Yellow;
}
public AB AAA { get; set; } = new AB { A = "2", B = "3" };
}
[TypeConverter(typeof(ABConverter))]
public class AB
{
public string A { get; set; }
public string B { get; set; }
}
}
3.类型转换器
using System;
using System.Collections;
using System.ComponentModel;
using System.ComponentModel.Design.Serialization;
using System.Globalization;
using System.Reflection;
namespace AttributeTest
{
public class ABConverter: TypeConverter
{
public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType)
{
if (sourceType == typeof(string))
{
return true;
}
return base.CanConvertFrom(context, sourceType);
}
public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType)
{
if (destinationType == typeof(string))
{
return true;
}
if (destinationType == typeof(InstanceDescriptor))
{
return true;
}
return base.CanConvertTo(context, destinationType);
}
public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value)
{
if (value == null)
{
return base.ConvertFrom(context, culture, value);
}
string s = value as string;
string[] ps = s.Split(',');
if (ps.Length != 2)
{
throw new ArgumentException("error!");
}
return new AB() { A = ps[0], B = ps[1] };
}
public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType)
{
if (destinationType == typeof(string) && value is AB)
{
AB a = (AB)value;
return string.Format("A={0},B={1}", a.A, a.B);
}
if (destinationType == typeof(InstanceDescriptor) && value is AB)
{
AB vd = (AB)value;
ConstructorInfo ctor = typeof(AB).GetConstructor(new Type[] { typeof(string), typeof(string) });
return new InstanceDescriptor(ctor, new object[] { vd.A, vd.B });
}
return base.ConvertTo(context, culture, value, destinationType);
}
/// <summary>
/// 改变数值时重新生成对象
/// </summary>
/// <param name="context"></param>
/// <param name="propertyValues"></param>
/// <returns></returns>
public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues)
{
string aa = propertyValues["A"].ToString();
string bb = propertyValues["B"].ToString();
return new AB() { A = aa, B = bb };
}
/// <summary>
/// 显示属性集合时要以怎样的形式
/// </summary>
/// <param name="context"></param>
/// <param name="value"></param>
/// <param name="attributes"></param>
/// <returns></returns>
public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes)
{
if (value is AB)
{
return TypeDescriptor.GetProperties(value, attributes);
}
return base.GetProperties(context, value, attributes);
}
public override bool GetCreateInstanceSupported(ITypeDescriptorContext context)
{
return true;
}
public override bool GetPropertiesSupported(ITypeDescriptorContext context)
{
return true;
}
}
}