第一章 String类
概述
java.lang.String 类代表字符串。Java程序中所有的字符串文字(例如 "abc" )都可以被看作是实现此类的实例。
类 String 中包括用于检查各个字符串的方法,比如用于比较字符串,搜索字符串,提取子字符串以及创建具有翻
译为大写或小写的所有字符的字符串的副本。
特点
1. 字符串不变:字符串的值在创建后不能被更改。
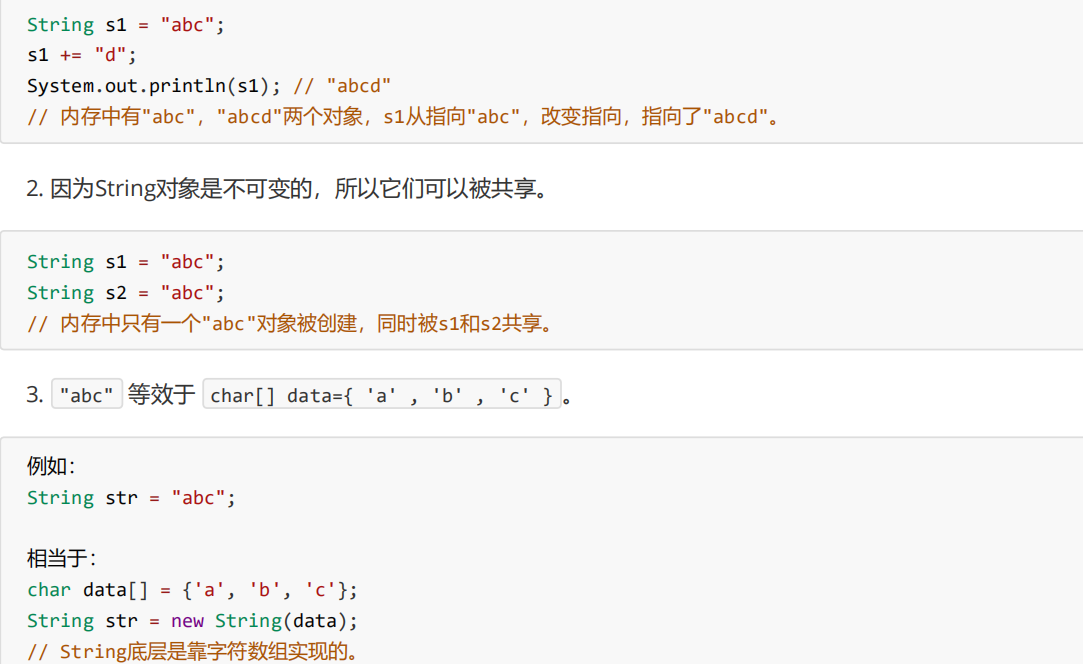
使用步骤
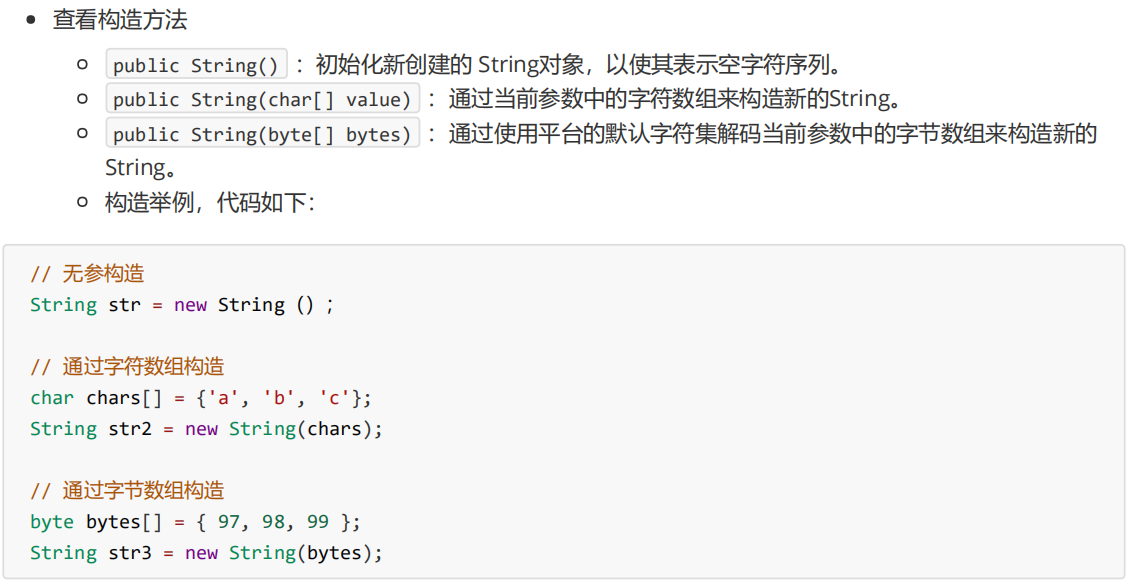
public boolean equals (Object anObject) :将此字符串与指定对象进行比较。
public boolean equalsIgnoreCase (String anotherString) :将此字符串与指定对象进行比较,忽略大小 写。

public void EqualTest(){ //创建字符串的对象 String s1="hello"; String s2="hello"; String s3="HELLO"; System.out.println(s1.equals(s2));//true System.out.println(s1.equals(s3));//flase System.out.println("======================"); System.out.println(s1.equalsIgnoreCase(s2));//true System.out.println(s1.equalsIgnoreCase(s3));//true }
public int length () :返回此字符串的长度。
public String concat (String str) :将指定的字符串连接到该字符串的末尾。
public char charAt (int index) :返回指定索引处的 char值。
public int indexOf (String str) :返回指定子字符串第一次出现在该字符串内的索引。
public String substring (int beginIndex) :返回一个子字符串,
从beginIndex开始截取字符串到字符 串结尾。
public String substring (int beginIndex, int endIndex) :
返回一个子字符串,从beginIndex到 endIndex截取字符串。含beginIndex,不含endIndex。

public void StrMethodTest(){ /* * public int length () :返回此字符串的长度。 * public String concat (String str) :将指定的字符串连接到该字符串的末尾。 * public char charAt (int index) :返回指定索引处的 char值。 * public int indexOf (String str) :返回指定子字符串第一次出现在该字符串内的索引。 * public String substring (int beginIndex) :返回一个子字符串, * 从beginIndex开始截取字符串到字符 串结尾。 *public String substring (int beginIndex, int endIndex) : * 返回一个子字符串,从beginIndex到 endIndex截取字符串。含beginIndex,不含endIndex。 * */ //创建字符串对象 String s="helloworld"; //int length():获取字符串的长度 System.out.println(s.length()); System.out.println("====================="); //String concat(String str):将指定的字符串连接到该字符串的末尾。 String s2=s.concat("**hello itheima"); System.out.println(s2);// helloworld**hello itheima System.out.println("==================="); //获取指定索引处的字符 for(int i=0;i<s.length();i++){ System.out.print(s.charAt(i)+" "); } System.out.println("==================================="); //public int indexOf (String str) :返回指定子字符串第一次出现在该字符串内的索引。 //没有返回-1; System.out.println(s.indexOf("l")); System.out.println(s.indexOf("owo")); System.out.println(s.indexOf("ak")); System.out.println("==========================="); // public String substring (int beginIndex) :返回一个子字符串, // * 从beginIndex开始截取字符串到字符 串结尾。 System.out.println(s.substring(0)); System.out.println(s.substring(5)); System.out.println("=============================="); }
public char[] toCharArray () :将此字符串转换为新的字符数组。
public byte[] getBytes () :使用平台的默认字符集将该 String编码转换为新的字节数组。
public String replace (CharSequence target, CharSequence replacement) :
将与target匹配的字符串使 用replacement字符串替换。

public void StrChangeTest(){ /* * public char[] toCharArray () :将此字符串转换为新的字符数组。 * public byte[] getBytes () :使用平台的默认字符集将该 String编码转换为新的字节数组。 * public String replace (CharSequence target, CharSequence replacement) : * 将与target匹配的字符串使 用replacement字符串替换。 * */ //创建字符串对象 String s="abcde"; //public char[] toCharArray () :将此字符串转换为新的字符数组。 char [] chs=s.toCharArray(); for(int i=0;i<chs.length;i++){ System.out.println(chs[i]); } System.out.println("================================="); //byte[] getBytes () :使用平台的默认字符集将该 String编码转换为新的字节数组。 byte[] bytes=s.getBytes(); for(int x=0;x<bytes.length;x++){ System.out.println(bytes[x]); } System.out.println("==================================="); //替换字母it为大写的IT String str="itcast itheima"; String replace=str.replace("it","IT"); System.out.println(replace);//ITcast ITheima System.out.println("============================"); }
第二章 static关键字
概述
关于 static 关键字的使用,它可以用来修饰的成员变量和成员方法,被修饰的成员是属于类的,而不是单单是属
于某个对象的。也就是说,既然属于类,就可以不靠创建对象来调用了。
定义和使用格式
类变量
当 static 修饰成员变量时,该变量称为类变量。该类的每个对象都共享同一个类变量的值。任何对象都可以更改
该类变量的值,但也可以在不创建该类的对象的情况下对类变量进行操作。
类变量:使用 static关键字修饰的成员变量。
定义格式:
static 数据类型 变量名;
第三章 Arrays类
概述
java.util.Arrays 此类包含用来操作数组的各种方法,比如排序和搜索等。其所有方法均为静态方法,调用起来非常简单
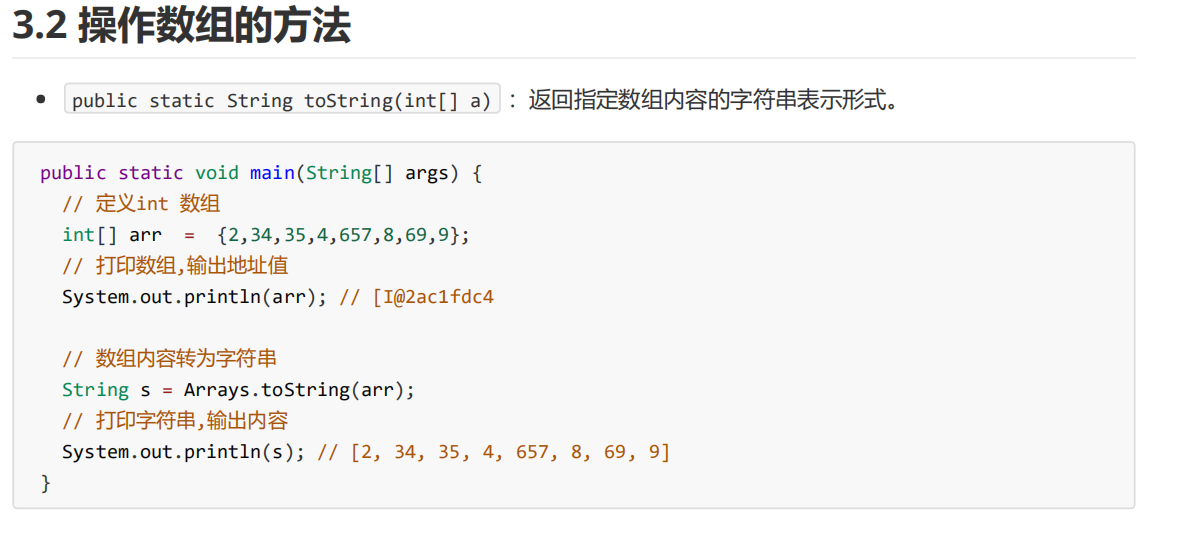
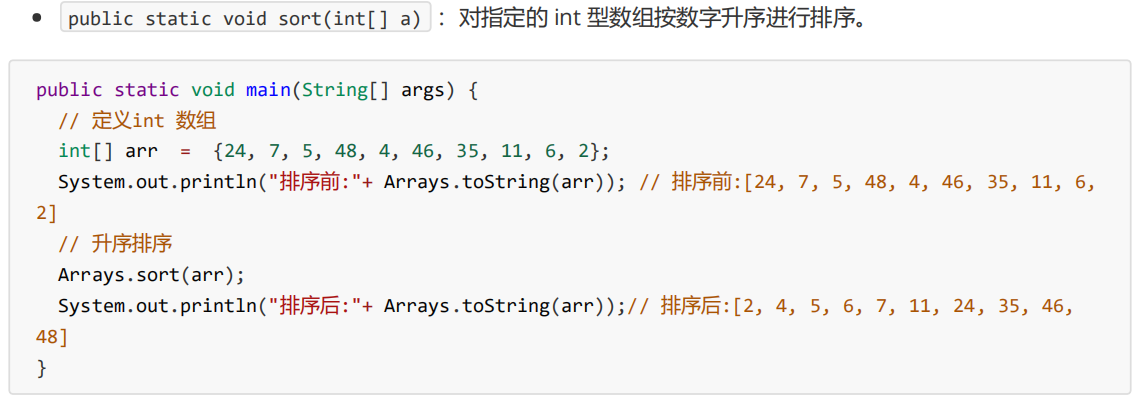
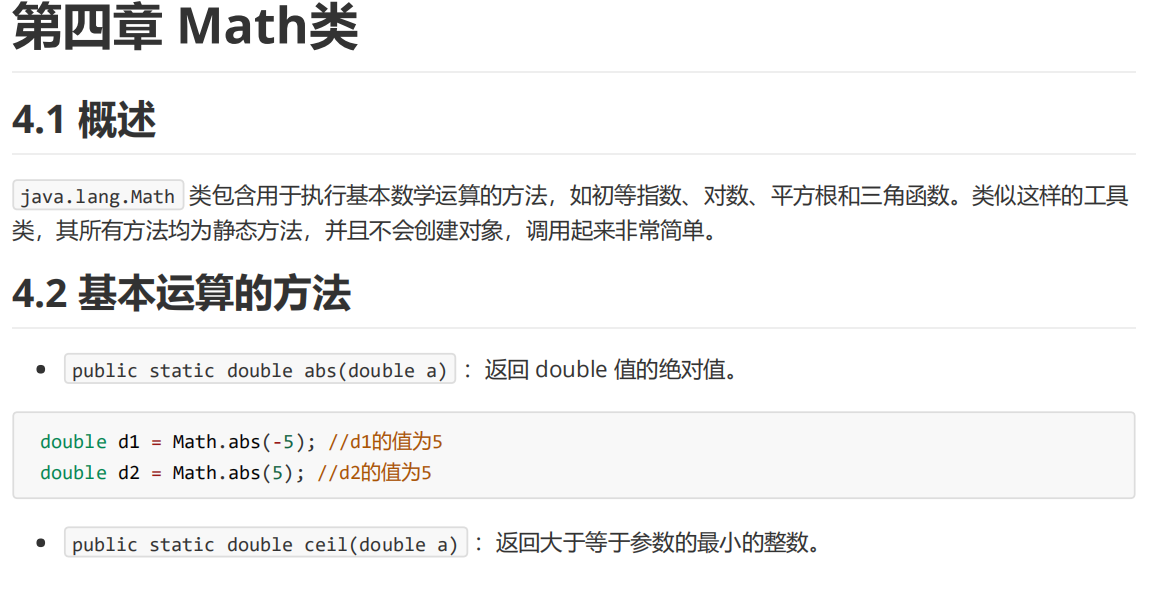
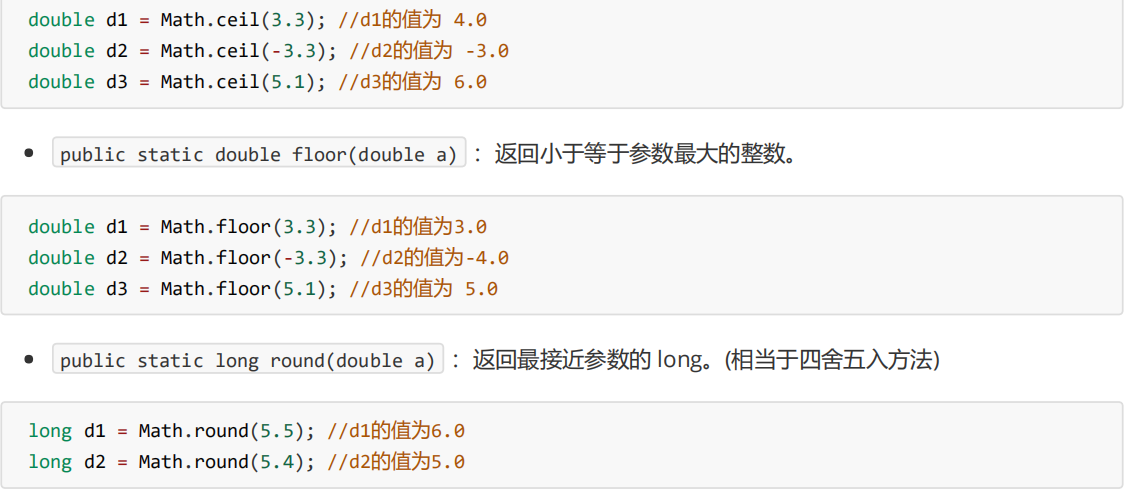