import numpy as np
np.random.seed(1337)
from keras.datasets import mnist
from keras.models import Model
from keras.layers import Dense, Input
import matplotlib.pyplot as plt
(x_train,y_train),(x_test,y_test) = mnist.load_data()
x_train = x_train.astype('float32') / 255.-0.5 #(-0.5,0.5)的区间
x_test = x_test.astype('float32') / 255.-0.5
x_train = x_train.reshape((x_train.shape[0],-1))
x_test = x_test.reshape((x_test.shape[0],-1))
print(x_train.shape)
print(x_test.shape)
# 最终压缩成2个
encoding_dim = 2
# 输入
input_img = Input(shape=(784,))
# encoder layers
encoded = Dense(128, activation='relu')(input_img)
encoded = Dense(64, activation='relu')(encoded)
encoded = Dense(10, activation='relu')(encoded)
encoder_output = Dense(encoding_dim,)(encoded)
# decoder layers
decoded = Dense(10,activation='relu')(encoder_output)
decoded = Dense(64,activation='relu')(decoded)
decoded = Dense(128,activation='relu')(decoded)
decoded = Dense(784,activation='tanh')(decoded)
# 搭建autoencoder模型
autoencoder = Model(input=input_img,output=decoded)
# 搭建encoder model for plotting,encoder是autoencoder的一部分
encoder = Model(input=input_img,output=encoder_output)
# 编译 autoencoder
autoencoder.compile(optimizer='adam',loss='mse')
# 训练
autoencoder.fit(x_train, x_train,
nb_epoch=20,
batch_size=256,
shuffle=True)
# plotting
encoded_imgs = encoder.predict(x_test)
plt.scatter(encoded_imgs[:,0], encoded_imgs[:,1], c=y_test)
plt.show()
E:ProgramDataAnaconda3libsite-packagesh5py\__init__.py:36: FutureWarning: Conversion of the second argument of issubdtype from `float` to `np.floating` is deprecated. In future, it will be treated as `np.float64 == np.dtype(float).type`.
from ._conv import register_converters as _register_converters
Using TensorFlow backend.
(60000, 784)
(10000, 784)
D:/我的python/用keras搭建神经网络/Autoencoder 自编码.py:38: UserWarning: Update your `Model` call to the Keras 2 API: `Model(inputs=Tensor("in..., outputs=Tensor("de...)`
autoencoder = Model(input=input_img,output=decoded)
D:/我的python/用keras搭建神经网络/Autoencoder 自编码.py:41: UserWarning: Update your `Model` call to the Keras 2 API: `Model(inputs=Tensor("in..., outputs=Tensor("de...)`
encoder = Model(input=input_img,output=encoder_output)
D:/我的python/用keras搭建神经网络/Autoencoder 自编码.py:50: UserWarning: The `nb_epoch` argument in `fit` has been renamed `epochs`.
shuffle=True)
Epoch 1/20
60000/60000 [==============================] - 5s 80us/step - loss: 0.0694
Epoch 2/20
60000/60000 [==============================] - 1s 20us/step - loss: 0.0562
Epoch 3/20
60000/60000 [==============================] - 1s 19us/step - loss: 0.0525
Epoch 4/20
60000/60000 [==============================] - 1s 20us/step - loss: 0.0493
Epoch 5/20
60000/60000 [==============================] - 1s 20us/step - loss: 0.0476
Epoch 6/20
60000/60000 [==============================] - 1s 20us/step - loss: 0.0463
Epoch 7/20
60000/60000 [==============================] - 1s 22us/step - loss: 0.0452
Epoch 8/20
60000/60000 [==============================] - 1s 23us/step - loss: 0.0442
Epoch 9/20
60000/60000 [==============================] - 1s 19us/step - loss: 0.0435
Epoch 10/20
60000/60000 [==============================] - 1s 19us/step - loss: 0.0429
Epoch 11/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0424
Epoch 12/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0419
Epoch 13/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0415
Epoch 14/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0412
Epoch 15/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0409
Epoch 16/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0405
Epoch 17/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0402
Epoch 18/20
60000/60000 [==============================] - 1s 19us/step - loss: 0.0401
Epoch 19/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0398
Epoch 20/20
60000/60000 [==============================] - 1s 18us/step - loss: 0.0397
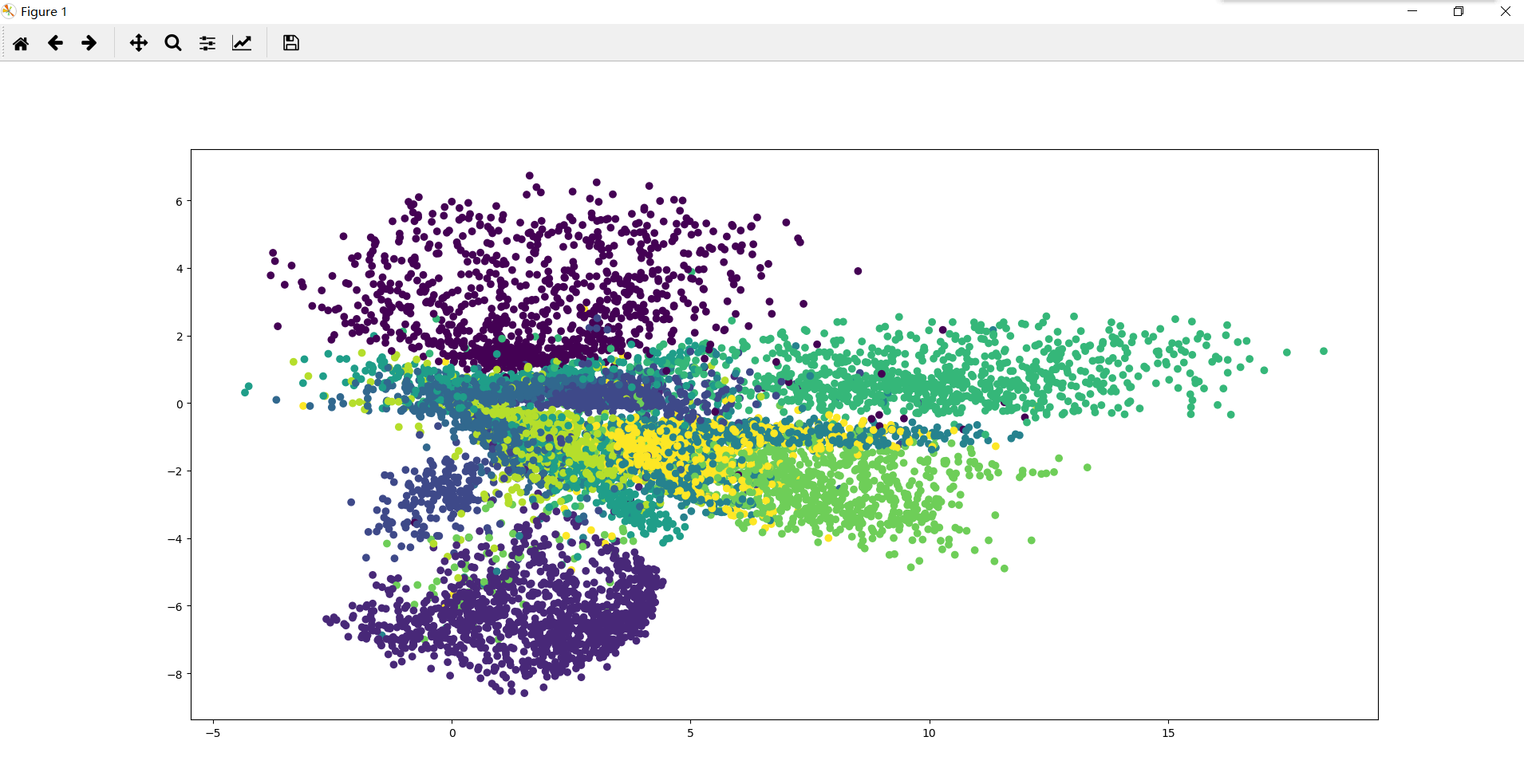