Time Limit: 10000MS | Memory Limit: 131072K | |
Total Submissions: 5409 | Accepted: 1371 | |
Case Time Limit: 3000MS |
Description
flymouse’s sister wc is very capable at sports and her favorite event is walking race. Chasing after the championship in an important competition, she comes to a training center to attend a training course. The center has N check-points numbered 1 through N. Some pairs of check-points are directly connected by two-way paths. The check-points and the paths form exactly a tree-like structure. The course lasts N days. On the i-th day, wc picks check-point i as the starting point and chooses another check-point as the finishing point and walks along the only simple path between the two points for the day’s training. Her choice of finishing point will make it that the resulting path will be the longest among those of all possible choices.
After every day’s training, flymouse will do a physical examination from which data will obtained and analyzed to help wc’s future training be better instructed. In order to make the results reliable, flymouse is not using data all from N days for analysis. flymouse’s model for analysis requires data from a series of consecutive days during which the difference between the longest and the shortest distances wc walks cannot exceed a bound M. The longer the series is, the more accurate the results are. flymouse wants to know the number of days in such a longest series. Can you do the job for him?
Input
The input contains a single test case. The test case starts with a line containing the integers N (N ≤ 106) and M (M < 109). Then follow N − 1 lines, each containing two integers fi and di (i = 1, 2, …, N − 1), meaning the check-points i + 1 and fi are connected by a path of length di.
Output
Output one line with only the desired number of days in the longest series.
Sample Input
3 2 1 1 1 3
Sample Output
3
Hint
Explanation for the sample:
There are three check-points. Two paths of lengths 1 and 3 connect check-points 2 and 3 to check-point 1. The three paths along with wc walks are 1-3, 2-1-3 and 3-1-2. And their lengths are 3, 4 and 4. Therefore data from all three days can be used for analysis.
Source
1 2
2 4
2 2
1 6
1 5
6 1
6 1
7 2
7 3
10 1
11 4
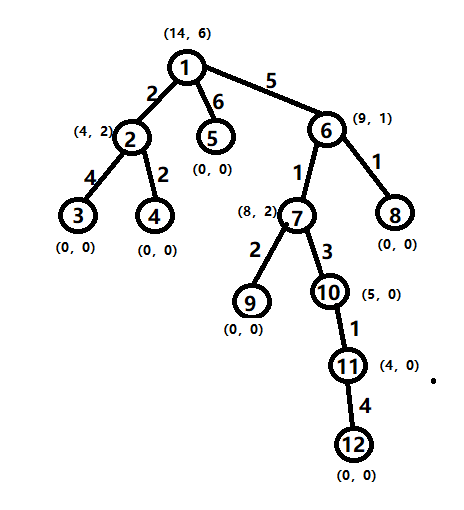
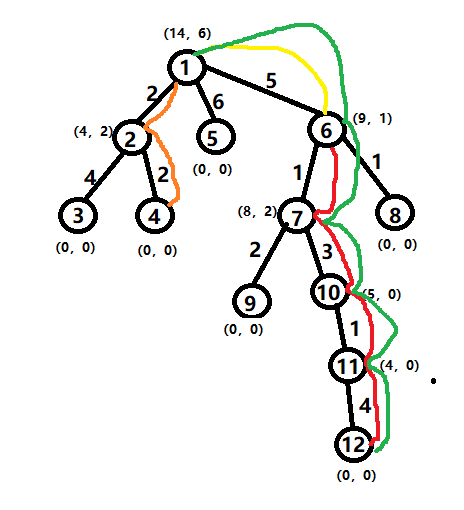
#include<cstdio> #include<algorithm> #include<queue> #define ll long long using namespace std; struct{ int v,w,next; }edge[2000010];//前向星存树 int head[1000010]; int cnt; deque<ll> mx,mn;//双向队列求连续天数 struct{ ll lfst;//最长距离 ll lsnd;//次长距离 }dp[1000010]; ll ans[1000010];//存实际最长距离 void add(int u,int v,int w){//加边 edge[cnt].v=v; edge[cnt].w=w; edge[cnt].next=head[u]; head[u]=cnt++; } void dfs1(int k,int fz){//(当前节点,父亲节点) //printf("%d ",k); for(int i=head[k];i!=-1;i=edge[i].next){ int v=edge[i].v; if(v==fz) continue; dfs1(v,k); //先往下递归 ll a=dp[v].lfst+edge[i].w; if(a>dp[k].lfst){//更新最长,次长距离 dp[k].lsnd=dp[k].lfst; dp[k].lfst=a; } else if(a>dp[k].lsnd) dp[k].lsnd=a; } } void dfs2(int k,int fz,int w){//(儿子节点,根节点,儿子节点到根节点的距离) //printf("wwww%d %d %d %d ",k,fz,dp[fz].lfst,dp[fz].lsnd); if(dp[k].lfst+w==dp[fz].lfst){//如果当前节点在其父亲节点的最长距离线路上 ll a=w+dp[fz].lsnd;//尝试走父亲节点的次长距离 if(a>dp[k].lfst){ dp[k].lsnd=dp[k].lfst; dp[k].lfst=a; } else if(a>dp[k].lsnd) dp[k].lsnd=a; ans[k]=dp[k].lfst; } else{ ll a=w+dp[fz].lfst;//尝试走父亲节点的最长距离 if(a>dp[k].lfst){//比较并更新 dp[k].lsnd=dp[k].lfst; dp[k].lfst=a; } else if(a>dp[k].lsnd) dp[k].lsnd=a; ans[k]=dp[k].lfst;//求出实际最长距离 } for(int i=head[k];i!=-1;i=edge[i].next){ int v=edge[i].v; if(v==fz) continue; dfs2(v,k,edge[i].w); } } void addmn(int k){//加入递增队列 while(mn.size()&&(ans[mn.back()]>ans[k])){//保持加入节点后队列的单调性 mn.pop_back(); } mn.push_back(k); } void addmx(int k){//加入递减队列 while(mx.size()&&(ans[mx.back()]<ans[k])){ mx.pop_back(); } mx.push_back(k); } int main(){ int n,m; int v,w; cnt=0; scanf("%d%d",&n,&m); fill(head,head+n+2,-1);//初始化 dp[0].lfst=dp[0].lsnd=0; dp[1].lfst=dp[1].lsnd=0; for(int i=2;i<=n;i++){ scanf("%d%d",&v,&w); dp[i].lfst=dp[i].lsnd=0;//初始化 add(i,v,w); add(v,i,w); } dfs1(1,0); ans[1]=dp[1].lfst;//我是以1根,那么1的实际最长距离就等于它的最长距离 for(int i=head[1];i!=-1;i=edge[i].next){//往它的儿子节点遍历 dfs2(edge[i].v,1,edge[i].w);//(儿子节点,根节点,儿子节点到根节点的距离) } mx.clear(); mn.clear(); mx.push_back(1);//先放入第一天 mn.push_back(1); int len=0; int len1=1; int lf=1;//左标记,表示以第几天开始来计算 for(int i=2;i<=n;i++){ addmx(i);//将该天加入到队列里 addmn(i); len1++;//长度加1 while(lf<i&&len1&&abs(ans[mn.front()]-ans[mx.front()])>m){//如果绝对差大于m,那么开始天数要往右移 len1--;//当前绝对差大于m,我们要尝试右移开始天数来使得绝对差变小 if(mn.front()==lf)//如果当前的开始天数是最小的距离 mn.pop_front(); if(mx.front()==lf)//如果当前的开始天数是最大距离 mx.pop_front(); lf++; } len=max(len,len1);//更新答案 //printf("wwwwwww%d %lld %lld %d ",i,mn.front(),mx.front(),lf); } printf("%d ",len); return 0; }