1.1 命名方法委托,匿名方法委托与Lambda表达式
委托的基本概念在上篇文章已经总结,本篇文章主要描述三种方式的写法
1.1.1 命名方法委托:
代码
class MyDelegateTest
{
//步骤1,声明delegate对象
public delegate void MyDelegate(string name);
//// 这是我们欲传递的方法,它与MyDelegate具有相同的参数和返回值类型
public static void MyDelegateMethod(string name)
{
Console.WriteLine("Hello,{0}", name);
}
public static void Main()
{
// 步骤2,创建delegate对象
MyDelegate md = new MyDelegate(MyDelegateTest.MyDelegateMethod);
// 步骤3,调用delegate
md("Michael");
Console.ReadLine();
}
}
1.1.2 匿名方法委托
代码
class AnonymousDelegate
{
private delegate void MyDelegate(string name);
public static void Main()
{
MyDelegate myDelegate = delegate(string name)
{
Console.WriteLine(name);
};
myDelegate("Michael");
Console.ReadLine();
}
}
1.1.3 Lambda表达式
Lambda表达式是C#3.0的一种新语法,语法简洁为编写匿名方法提供了更简明的函数式的句法.
代码
class LambdaTest
{
private delegate void MyDelegate(string name);
public static void Main()
{
//匿名委托
MyDelegate myDelegate = (name) => { Console.WriteLine(name); };
//调用委托
myDelegate("Michael");
Console.ReadLine();
}
}
使用Lambda表达式更简洁,为什么那么简洁.其实是编译器为我们做了很多事情. MyDelegate myDelegate = (name) => { Console.WriteLine(name); }; 这句话编译器在编译的时候会为我们生成一个私有的静态方法.透过ILDASM可以看到。
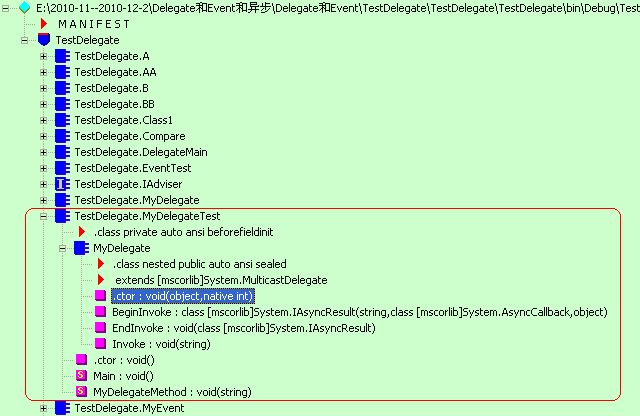
1.2 泛型委托 Predicate/Func/Action
1.2.1 Predicate 泛型委托
表示定义一组条件并确定指定对象是否符合这些条件的方法。此委托由 Array 和 List 类的几种方法使用,用于在集合中搜索元素。
看看下面它的定义:
// Summary:
// Represents the method that defines a set of criteria and determines whether
// the specified object meets those criteria.
//
// Parameters:
// obj:
// The object to compare against the criteria defined within the method represented
// by this delegate.
//
// Type parameters:
// T:
// The type of the object to compare.
//
// Returns:
// true if obj meets the criteria defined within the method represented by this
// delegate; otherwise, false.
public delegate bool Predicate<T>(T obj);
类型参数介绍:
T: 要比较的对象的类型。
obj: 要按照由此委托表示的方法中定义的条件进行比较的对象。
返回值:如果 obj 符合由此委托表示的方法中定义的条件,则为 true;否则为 false。
使用到Predicate 有Array.Find , Array.FindAll , Array.Exists ..... List<T>.Find , List<T>.FindAll , List<T>.Exists 等
代码
class ListToFindMethod
{
static void Main()
{
List<Person> personList = new List<Person>();
personList.Add(new Person { Name = "Quincy", Age = 20 });
personList.Add(new Person { Name = "cqs", Age = 25 });
personList.Add(new Person { Name = "Liu", Age = 23 });
personList.Add(new Person { Name = "Cheng", Age = 23 });
#region 使用Find搜索单个匹配值
Person FindPerson = personList.Find(delegate(Person x)
{
return x.Name == "Quincy";
});
Console.WriteLine(FindPerson.Age);
Console.WriteLine("----------------华丽分割线------------------");
Person FindPersonLambda = personList.Find((x)=>{return x.Name == "Quincy";});
Console.WriteLine(FindPersonLambda.Age);
Console.WriteLine("----------------华丽分割线------------------");
#endregion
}
public class Person
{
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private int age;
public int Age
{
get { return age; }
set { age = value; }
}
}
1.2.2 Action 泛型委托
// 摘要:
// Encapsulates a method that takes a single parameter and does not return a
// value.
//
// 参数:
// obj:
// The parameter of the method that this delegate encapsulates.
//
// 类型参数:
// T:
// The type of the parameter of the method that this delegate encapsulates.
public delegate void Action<T>(T obj);
Action<T>委托表示引用一个返回类型为Void的方法。这个委托存在不同的变体,可以传递至多4个不同的参数类型。
1.2.3 Fun 泛型委托
// 摘要:
// Encapsulates a method that has no parameters and returns a value of the type
// specified by the TResult parameter.
//
// 类型参数:
// TResult:
// The type of the return value of the method that this delegate encapsulates.
//
// 返回结果:
// The return value of the method that this delegate encapsulates.
public delegate TResult Func<TResult>();
Func<T>允许调用带返回参数的方法。Func<T>也有不同的变体,至多可以传递4个参数和一个返回类型。例如:Func<TResult>委托类型可以无参的带返回类型的方法,Func< T1,T2,TResult>表示带两个参数和一个返回类型的方法。
1.3 泛型委托在项目中的应用
我们经常要在UI端下Try-catch语句,我们可以通过泛型委托的方式简化我们的代码,如下面的公共函数:
代码
protected TReturn Invoke<TReturn>(Func<TReturn> func)
{
try
{
return func();
}
catch (TPException)
{
throw;
}
catch (Exception ex)
{
LogHelper.LogWrite(ex, Category.Exception, Priority.High);
throw GetTPException();
}
}
protected void Invoke(Guid sessionId, Action<SessionInformation> action)
{
try
{
SessionInformation si = SessionOperate.CreateInstance(sessionId);
if (si == null)
throw new TPException() { ErrorCode = ExceptionResource.SYS_SESSIONINVALID };
action();
}
catch (TPException)
{
throw;
}
catch (Exception ex)
{
LogHelper.LogWrite(ex, Category.Exception, Priority.High);
throw GetTPException();
}
}
在具体的调用:
代码
public List<ReqSource> GetReqSourceType( Guid sessionID, Guid CollectionID, Guid configID)
{
return this.Invoke(sessionID,si => m_requirementManager.GetReqSourceByType(configID, CollectionID));
}
委托的基本概念在上篇文章已经总结,本篇文章主要描述三种方式的写法
1.1.1 命名方法委托:

{
//步骤1,声明delegate对象
public delegate void MyDelegate(string name);
//// 这是我们欲传递的方法,它与MyDelegate具有相同的参数和返回值类型
public static void MyDelegateMethod(string name)
{
Console.WriteLine("Hello,{0}", name);
}
public static void Main()
{
// 步骤2,创建delegate对象
MyDelegate md = new MyDelegate(MyDelegateTest.MyDelegateMethod);
// 步骤3,调用delegate
md("Michael");
Console.ReadLine();
}
}
1.1.2 匿名方法委托
代码
class AnonymousDelegate
{
private delegate void MyDelegate(string name);
public static void Main()
{
MyDelegate myDelegate = delegate(string name)
{
Console.WriteLine(name);
};
myDelegate("Michael");
Console.ReadLine();
}
}

{
private delegate void MyDelegate(string name);
public static void Main()
{
MyDelegate myDelegate = delegate(string name)
{
Console.WriteLine(name);
};
myDelegate("Michael");
Console.ReadLine();
}
}
1.1.3 Lambda表达式
Lambda表达式是C#3.0的一种新语法,语法简洁为编写匿名方法提供了更简明的函数式的句法.

{
private delegate void MyDelegate(string name);
public static void Main()
{
//匿名委托
MyDelegate myDelegate = (name) => { Console.WriteLine(name); };
//调用委托
myDelegate("Michael");
Console.ReadLine();
}
}
使用Lambda表达式更简洁,为什么那么简洁.其实是编译器为我们做了很多事情. MyDelegate myDelegate = (name) => { Console.WriteLine(name); }; 这句话编译器在编译的时候会为我们生成一个私有的静态方法.透过ILDASM可以看到。
1.2 泛型委托 Predicate/Func/Action
1.2.1 Predicate 泛型委托
表示定义一组条件并确定指定对象是否符合这些条件的方法。此委托由 Array 和 List 类的几种方法使用,用于在集合中搜索元素。
看看下面它的定义:
// Summary:
// Represents the method that defines a set of criteria and determines whether
// the specified object meets those criteria.
//
// Parameters:
// obj:
// The object to compare against the criteria defined within the method represented
// by this delegate.
//
// Type parameters:
// T:
// The type of the object to compare.
//
// Returns:
// true if obj meets the criteria defined within the method represented by this
// delegate; otherwise, false.
public delegate bool Predicate<T>(T obj);
类型参数介绍:
T: 要比较的对象的类型。
obj: 要按照由此委托表示的方法中定义的条件进行比较的对象。
返回值:如果 obj 符合由此委托表示的方法中定义的条件,则为 true;否则为 false。
使用到Predicate 有Array.Find , Array.FindAll , Array.Exists ..... List<T>.Find , List<T>.FindAll , List<T>.Exists 等

{
static void Main()
{
List<Person> personList = new List<Person>();
personList.Add(new Person { Name = "Quincy", Age = 20 });
personList.Add(new Person { Name = "cqs", Age = 25 });
personList.Add(new Person { Name = "Liu", Age = 23 });
personList.Add(new Person { Name = "Cheng", Age = 23 });
#region 使用Find搜索单个匹配值
Person FindPerson = personList.Find(delegate(Person x)
{
return x.Name == "Quincy";
});
Console.WriteLine(FindPerson.Age);
Console.WriteLine("----------------华丽分割线------------------");
Person FindPersonLambda = personList.Find((x)=>{return x.Name == "Quincy";});
Console.WriteLine(FindPersonLambda.Age);
Console.WriteLine("----------------华丽分割线------------------");
#endregion
}
public class Person
{
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private int age;
public int Age
{
get { return age; }
set { age = value; }
}
}
1.2.2 Action 泛型委托
// 摘要:
// Encapsulates a method that takes a single parameter and does not return a
// value.
//
// 参数:
// obj:
// The parameter of the method that this delegate encapsulates.
//
// 类型参数:
// T:
// The type of the parameter of the method that this delegate encapsulates.
public delegate void Action<T>(T obj);
1.2.3 Fun 泛型委托
// 摘要:
// Encapsulates a method that has no parameters and returns a value of the type
// specified by the TResult parameter.
//
// 类型参数:
// TResult:
// The type of the return value of the method that this delegate encapsulates.
//
// 返回结果:
// The return value of the method that this delegate encapsulates.
public delegate TResult Func<TResult>();
Func<T>允许调用带返回参数的方法。Func<T>也有不同的变体,至多可以传递4个参数和一个返回类型。例如:Func<TResult>委托类型可以无参的带返回类型的方法,Func< T1,T2,TResult>表示带两个参数和一个返回类型的方法。
1.3 泛型委托在项目中的应用
我们经常要在UI端下Try-catch语句,我们可以通过泛型委托的方式简化我们的代码,如下面的公共函数:

{
try
{
return func();
}
catch (TPException)
{
throw;
}
catch (Exception ex)
{
LogHelper.LogWrite(ex, Category.Exception, Priority.High);
throw GetTPException();
}
}
protected void Invoke(Guid sessionId, Action<SessionInformation> action)
{
try
{
SessionInformation si = SessionOperate.CreateInstance(sessionId);
if (si == null)
throw new TPException() { ErrorCode = ExceptionResource.SYS_SESSIONINVALID };
action();
}
catch (TPException)
{
throw;
}
catch (Exception ex)
{
LogHelper.LogWrite(ex, Category.Exception, Priority.High);
throw GetTPException();
}
}
在具体的调用:

{
return this.Invoke(sessionID,si => m_requirementManager.GetReqSourceByType(configID, CollectionID));
}