A Simple Example of C
#include <stdio.h>
int main(void) { /* a simple program */
int num; /* define a variable called num */
num = 1; /* assign a value to num */
printf("I am a simple "); /* use the printf() function */
printf("computer.
");
printf("My favorite number is %d because it is first.
", num);
return 0;
}
If you think this program will print something on your screen, you’re right! Exactly what will be printed might not be apparent, so run the program and see the results. First, use your favorite editor (or your compiler’s favorite editor) to create a file containing the text from Listing 2.1 . Give the file a name that ends in .c and that satisfies your local system’s name requirements. You can use first.c , for example. Now compile and run the program. (Check Chapter 1 , “Getting Ready,” for some general guidelines to this process.) If all went well, the output should look like the following:
I am a simple computer.
My favorite number is 1 because it is first.
Program Adjustments
Did the output for this program briefly flash onscreen and then disappear? Some windowing environments run the program in a separate window and then automatically close the window when the program finishes. In this case, you can supply extra code to make the window stay open until you strike a key. One way is to add the following line before the return statement:
getchar();
This code causes the program to wait for a keystroke, so the window remains open until you press a key.
The Example Explained
Pass 1: Quick Synopsis
This section presents each line from the program followed by a short description; the next section (Pass 2) explores the topics raised here more fully.
#include <stdio.h> include another file
This line tells the compiler to include the information found in the file stdio.h , which is a standard part of all C compiler packages; this file provides support for keyboard input and for displaying output.
int main(void) a function name
C programs consist of one or more functions , the basic modules of a C program. This program consists of one function called main . The parentheses identify main() as a function name. The int indicates that the main() function returns an integer, and the void indicates that main() doesn’t take any arguments. These are matters we’ll go into later. Right now, just accept both int and void as part of the standard ANSI C way for defining main() . (If you have a pre-ANSI C compiler, omit void ; you may want to get something more recent to avoid incompatibilities.)
/* a simple program */ this is a comment
The symbols /* and */ enclose comments—remarks that help clarify a program. They are intended for the reader only and are ignored by the compiler.
{ beginning of the body of the function
This opening brace marks the start of the statements that make up the function. A closing brace ( } ) marks the end of the function definition.
int num; a declaration statement
This statement announces that you are using a variable called num and that num will be an int (integer) type.
num = 1; an assignment statement
The statement num = 1; assigns the value 1 to the variable called num .
printf("I am a simple "); a function call statement
The first statement using printf() displays the phrase I am a simple on your screen, leaving the cursor on the same line. Here printf() is part of the standard C library. It’s termed a function , and using a function in the program is termed calling a function .
printf("computer.
"); another function call statement
The next call to the printf() function tacks on computer to the end of the last phrase printed. The is code telling the computer to start a new line—that is, to move the cursor to the beginning of the next line.
printf("My favorite number is %d because it is first.
", num);
The last use of printf() prints the value of num (which is 1 ) embedded in the phrase in quotes. The %d instructs the computer where and in what form to print the value of num .
return 0; // a return statement
A C function can furnish, or return , a number to the agency that used it. For the present, just regard this line as the appropriate closing for a main() function.
} the end
As promised, the program ends with a closing brace.
Pass 2: Program Details
#include Directives and Header Files
#include <stdio.h>
This is the line that begins the program. The effect of #include <stdio.h> is the same as if you had typed the entire contents of the stdio.h file into your file at the point where the #include line appears. In effect, it’s a cut-and-paste operation. include files provide a convenient way to share information that is common to many programs.
The #include statement is an example of a C preprocessor directive . In general, C compilers perform some preparatory work on source code before compiling; this is termed preprocessing .
The stdio.h file is supplied as part of all C compiler packages. It contains information about input and output functions, such as printf() , for the compiler to use. The name stands for standard input/output header . C people call a collection of information that goes at the top of a file a header , and C implementations typically come with several header files.
For the most part, header files contain information used by the compiler to build the final executable program. For example, they may define constants or indicate the names of functions and how they should be used. But the actual code for a function is in a library file of precompiled code, not in a header file. The linker component of the compiler takes care of finding the library code you need. In short, header files help guide the compiler in putting your program together correctly.
ANSI/ISO C has standardized which header files a C compiler must make available. Some programs need to include stdio.h , and some don’t. The documentation for a particular C implementation should include a description of the functions in the C library. These function descriptions identify which header files are needed. For example, the description for printf() says to use stdio.h . Omitting the proper header file might not affect a particular program, but it is best not to rely on that. Each time this book uses library functions, it will use the include files specified by the ANSI/ISO standard for those functions.
Note Why Input and Output Are Not Built In
Perhaps you are wondering why facilities as basic as input and output aren’t included automatically. One answer is that not all programs use this I/O (input/output) package, and part of the C philosophy is to avoid carrying unnecessary weight. This principle of economic use of resources makes C popular for embedded programming—for example, writing code for a chip that controls an automotive fuel system or a Blu-ray player. Incidentally, the #include line is not even a C language statement! The # symbol in column 1 identifies the line as one to be handled by the C preprocessor before the compiler takes over. You will encounter more examples of preprocessor instructions later, and Chapter 16 , “The C Preprocessor and the C Library,” discusses this topic more fully.
The main() Function
int main()
This next line from the program proclaims a function by the name of main . True, main is a rather plain name, but it is the only choice available. A C program (with some exceptions we won’t worry about) always begins execution with the function called main() . You are free to choose names for other functions you use, but main() must be there to start things. What about the parentheses? They identify main() as a function. You will learn more about functions soon. For now, just remember that functions are the basic modules of a C program.
The int is the main() function’s return type. That means that the kind of value main() can return is an integer. Return where? To the operating system—we’ll come back to this question in C hapter 6 , “C Control Statements: Looping.”
The parentheses following a function name generally enclose information being passed along to the function. For this simple example, nothing is being passed along, so the parentheses contain the word void . (C hapter 11 , “Character Strings and String Functions,” introduces a second format that allows information to be passed to main() from the operating system.)
The int is the main() function’s return type. That means that the kind of value main() can return is an integer. Return where? To the operating system.
The parentheses following a function name generally enclose information being passed along to the function. For this simple example, nothing is being passed along, so the parentheses contain the word void.
Comments
/* a simple program */
The parts of the program enclosed in the /* */ symbols are comments. Using comments makes it easier for someone (including yourself) to understand your program. One nice feature of C comments is that they can be placed anywhere, even on the same line as the material they explain. A longer comment can be placed on its own line or even spread over more than one line. Everything between the opening /* and the closing */ is ignored by the compiler. The following are some valid and invalid comment forms:
/* This is a C comment. */
/* This comment, being somewhat wordy, is spread over two lines. */
/*
You can do this, too.
*/
/* But this is invalid because there is no end marker.
C99 added a second style of comments, one popularized by C++ and Java. The new style uses the symbols // to create comments that are confined to a single line:
// Here is a comment confined to one line.
int rigue; // Such comments can go here, too.
Declarations
int num;
This line from the program is termed a declaration statement . The declaration statement is one of C’s most important features. This particular example declares two things. First, somewhere in the function, you have a variable called num . Second, the int proclaims num as an integer—that is, a number without a decimal point or fractional part. ( int is an example of a data type .) The compiler uses this information to arrange for suitable storage space in memory for the num variable. The semicolon at the end of the line identifies the line as a C statement or instruction. The semicolon is part of the statement, not just a separator between statements as it is in Pascal.
The word int is a C keyword identifying one of the basic C data types. Keywords are the words used to express a language, and you can’t use them for other purposes. For instance, you can’t use int as the name of a function or a variable. These keyword restrictions don’t apply outside the language, however, so it is okay to name a cat or favorite child int . (Local custom or law may void this option in some locales.)
The word num in this example is an identifier —that is, a name you select for a variable, a function, or some other entity. So the declaration connects a particular identifier with a particular location in computer memory, and it also establishes the type of information, or data type, to be stored at that location.
In C, all variables must be declared before they are used. This means that you have to provide lists of all the variables you use in a program and that you have to show which data type each variable is. Declaring variables is considered a good programming technique, and, in C, it is mandatory.
Traditionally, C has required that variables be declared at the beginning of a block with no other kind of statement allowed to come before any of the declarations. That is, the body of main() might look like the following:
int main() { //traditional rules
int doors;
int dogs;
doors = 5;
dogs = 3;
// other statements
}
Data Types
C deals with several kinds (or types) of data: integers, characters, and floating point, for example. Declaring a variable to be an integer or a character type makes it possible for the computer to store, fetch, and interpret the data properly.
Name Choice
You should use meaningful names (or identifiers) for variables (such as sheep_count instead of
x3 if your program counts sheep). If the name doesn’t suffice, use comments to explain what the variables represent. Documenting a program in this manner is one of the basic techniques of good programming.
With C99 and C11 you can make the name of an identifier as long as you want, but the compiler need only consider the first 63 characters as significant. For external identifiers (see Chapter 12 , “Storage Classes, Linkage, and Memory Management”) only 31 characters need to be recognized. This is a substantial increase from the C90 requirement of 31 characters and six characters, respectively, and older C compilers often stopped at eight characters max. Actually, you can use more than the maximum number of characters, but the compiler isn’t required to pay attention to the extra characters. What does this mean? If you have two identifiers each 63 characters long and identical except for one character, the compiler is required to recognize them as distinct from one another. If you have two identifiers 64 characters long and identical except for the final character, the compiler might recognize them as distinct, or it might not; the standard doesn’t define what should happen in that case.
The characters at your disposal are lowercase letters, uppercase letters, digits, and the underscore ( _ ). The first character must be a letter or an underscore. The following are some examples:
Operating systems and the C library often use identifiers with one or two initial underscore characters, such as in _kcab , so it is better to avoid that usage yourself. The standard labels beginning with one or two underscore characters, such as library identifiers, are reserved . This means that although it is not a syntax error to use them, it could lead to name conflicts.
C names are case sensitive , meaning an uppercase letter is considered distinct from the corresponding lowercase letter. Therefore, stars is different from Stars and STARS .
To make C more international, C99 and C11 make an extensive set of characters available for use by the Universal Character Names (or UMC ) mechanism. Reference Section VII, “Expanded Character Support,” in A ppendix B discusses this addition. This makes available characters that are not part of the English alphabet.
Assignment
num = 1; // this is a assignment statement
The next program line is an assignment statement , one of the basic operations in C. This particular example means “assign the value 1 to the variable num .” The earlier int num; line set aside space in computer memory for the variable num , and the assignment line stores a value in that location. You can assign num a different value later, if you want; that is why num is termed a variable . Note that the assignment statement assigns a value from the right side to the left side. Also, the statement is completed with a semicolon, as shown in F igure 2.2 .
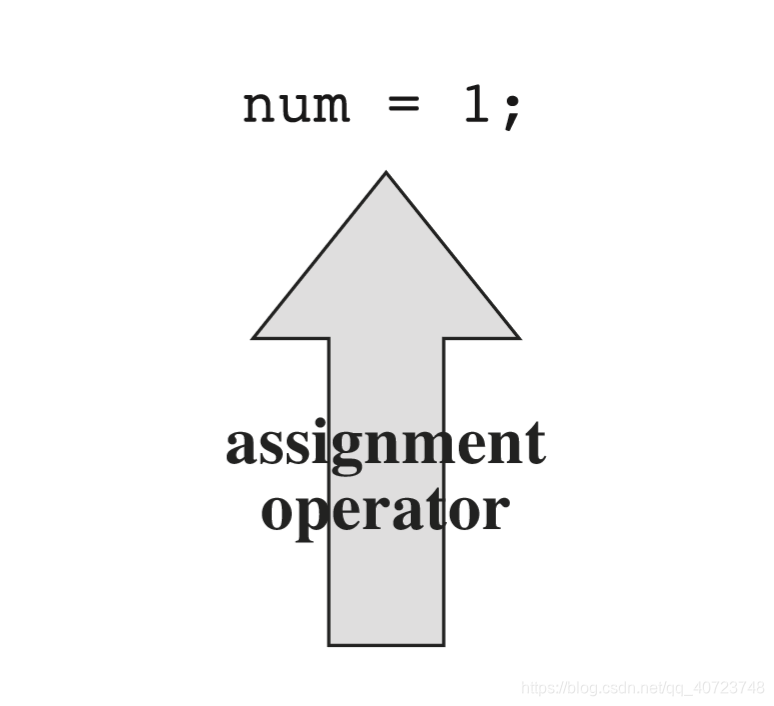
The printf() Function
printf("I am a simple ");
printf("computer.
");
printf("My favorite number is %d because it is first.
", num);
These lines all use a standard C function called printf() . The parentheses signify that printf is a function name. The material enclosed in the parentheses is information passed from the
main() function to the printf() function. For example, the first line passes the phrase I am a simple to the printf() function. Such information is called the argument or, more fully, the actual argument of a function (see F igure 2.3 ). (C uses the terms actual argument and formal argument to distinguish between a specific value sent to a function and a variable in the function used to hold the value; C hapter 5 “Operators, Expressions, and Statements,” goes into this matter in more detail.) What does the function printf() do with this argument? It looks at whatever lies between the double quotation marks and prints that text onscreen.
This first printf() line is an example of how you call or invoke a function in C. You need type only the name of the function, placing the desired argument(s) within the parentheses. When the program reaches this line, control is turned over to the named function ( printf() in this case). When the function is finished with whatever it does, control is returned to the original (the calling ) function— main() , in this example.
What about this next printf() line? It has the characters
included in the quotes, and they didn’t get printed! What’s going on? The
symbol means to start a new line. The
combination (typed as two characters) represents a single character called the newline character . To printf() , it means “start a new line at the far-left margin.” In other words, printing the newline character performs the same function as pressing the Enter key of a typical keyboard. Why not just use the Enter key when typing the printf() argument? That would be interpreted as an immediate command to your editor, not as an instruction to be stored in your source code. In other words, when you press the Enter key, the editor quits the current line on which you are working and starts a new one. The newline character, however, affects how the output of the program is displayed.
The newline character is an example of an escape sequence . An escape sequence is used to represent difficult- or impossible-to-type characters. Other examples are for Tab and for Backspace. In each case, the escape sequence begins with the backslash character, . We’ll return to this subject in C hapter 3 , “Data and C.”
Well, that explains why the three printf() statements produced only two lines: The first print instruction didn’t have a newline character in it, but the second and third did.
The final printf() line brings up another oddity: What happened to the %d when the line was printed? As you will recall, the output for this line was
My favorite number is 1 because it is first.
Aha! The digit 1 was substituted for the symbol group %d when the line was printed, and 1 was the value of the variable num . The %d is a placeholder to show where the value of num is to be printed. This line is similar to the following BASIC statement:
PRINT "My favorite number is "; num; " because it is first."
The C version does a little more than this, actually. The % alerts the program that a variable is to be printed at that location, and the d tells it to print the variable as a decimal (base 10) integer. The printf() function allows several choices for the format of printed variables, including hexadecimal (base 16) integers and numbers with decimal points. Indeed, the f in
printf() is a reminder that this is a formatting print function. Each type of data has its own specifier—as the book introduces new types, it will also introduce the appropriate specifiers.
Return Statement
return 0;
The return statement is the final statement of the program. The int in int main(void) means that the main() function is supposed to return an integer. The C standard requires that main() behave that way. C functions that return values do so with a return statement, which consists of the keyword return , followed by the returned value, followed by a semicolon. If you leave out the return statement for main() , the program will return 0 when it reaches the closing } . So you can omit the return statement at the end of main() . However, you can’t omit it from other functions, so it’s more consistent to use it in main() , too. At this point, you can regard the return statement in main() as something required for logical consistency, but it has a practical use with some operating systems, including Linux and Unix. C hapter 11 will deal further with this topic.
The Structure of a Simple Program
A program consists of a collection of one or more functions, one of which must be called main() . The description of a function consists of a header and a body. The function header contains the function name along with information about the type of information passed to the function and returned by the function. You can recognize a function name by the parentheses, which may be empty. The body is enclosed by braces ( {} ) and consists of a series of statements, each terminated by a semicolon.
While You're are at It-Multiple Functions
//* two_func.c -- a program using two functions in one file */
#include <stdio.h>
void butler(void); /* ANSI/ISO C function prototyping */
int main(void) {
printf("I will summon the butler function.
");
butler();
printf("Yes. Bring me some tea and writeable DVDs.
");
return 0;
}
void butler(void) { /* start of function definition */
printf("You rang, sir?
");
}
The program above shows you how to incorporate a function of your own-besides main() - into a program.
The butler() function appears three times in this program. The first appearance is in the prototype , which informs the compiler about the functions to be used. The second appearance is in main() in the form of a function call . Finally, the program presents the function definition , which is the source code for the function itself. Let’s look at each of these three appearances in turn.
One point to note is that it is the location of the butler() call in main() —not the location of the butler() definition in the file—that determines when the butler() function is executed. You could, for example, put the butler() definition above the main() definition in this program, and the program would still run the same, with the butler() function executed between the two calls to printf() in main() . Remember, all C programs begin execution with main() , no matter where main() is located in the program files. However, the usual C practice is to list main() first because it normally provides the basic framework for a program.
Introducing Debugging
Program errors often are called bugs , and finding and fixing the errors is called debugging .
Syntax Errors
You commit a syntax error when you don’t follow C’s rules. It’s analogous to a grammatical error in English. For instance, consider the following sentence: Bugs frustrate be can . This sentence uses valid English words but doesn’t follow the rules for word order, and it doesn’t have quite the right words, anyway. C syntax errors use valid C symbols in the wrong places.
How do you detect syntax errors? First, before compiling, you can look through the source code and see whether you spot anything obvious. Second, you can examine errors found by the compiler because part of its job is to detect syntax errors. When you attempt to compile this program, the compiler reports back any errors it finds, identifying the nature and location of each error.
However, the compiler can get confused. A true syntax error in one location might cause the compiler to mistakenly think it has found other errors. For instance, because the example does not declare n2 and n3 correctly, the compiler might think it has found further errors whenever those variables are used. In fact, if you can’t make sense of all the reported errors, rather than trying to correct all the reported errors at once, you should correct just the first one or two and then recompile; some of the other errors may go away. Continue in this way until the program works. Another common compiler trick is reporting the error a line late. For instance, the compiler may not deduce that a semicolon is missing until it tries to compile the next line. So if the compiler complains of a missing semicolon on a line that has one, check the line before.
Semantic Errors
Semantic errors are errors in meaning. For example, consider the following sentence: Scornful derivatives sing greenly . The syntax is fine because adjectives, nouns, verbs, and adverbs are in the right places, but the sentence doesn’t mean anything. In C, you commit a semantic error when you follow the rules of C correctly but to an incorrect end.
The compiler does not detect semantic errors, because they don’t violate C rules. The compiler has no way of divining your true intentions. That leaves it to you to find these kinds of errors.
Program State
By tracing the program step-by-step manually, keeping track of each variable, you monitor the program state. The program state is simply the set of values of all the variables at a given point in program execution. It is a snapshot of the current state of computation.
We just discussed one method of tracing the state: executing the program step-by-step yourself. In a program that makes, say, 10,000 iterations, you might not feel up to that task. Still, you can go through a few iterations to see whether your program does what you intend. However, there is always the possibility that you will execute the steps as you intended them to be executed instead of as you actually wrote them, so try to be faithful to the actual code.
Another approach to locating semantic problems is to sprinkle extra printf() statements throughout to monitor the values of selected variables at key points in the program. Seeing how the values change can illuminate what’s happening. After you have the program working to your satisfaction, you can remove the extra statements and recompile.
A third method for examining the program states is to use a debugger. A debugger is a program that enables you to run another program step-by-step and examine the value of that program’s variables. Debuggers come in various levels of ease of use and sophistication. The more advanced debuggers show which line of source code is being executed. This is particularly handy for programs with alternative paths of execution because it is easy to see which particular paths are being followed.
Keywords and Reserved Identifiers
Keywords are the vocabulary of C. Because they are special to C, you can’t use them as identifiers, for example, or as variable names. Many of these keywords specify various types, such as int . Others, such as if , are used to control the order in which program statements are executed. In the following list of C keywords, boldface indicates keywords added by the C90 standard, italics indicates new keywords added by the C99 standard, and boldface italics indicates keywords added by the C11 standard.
If you try to use a keyword, for, say, the name of a variable, the compiler catches that as a syntax error. There are other identifiers, called reserved identifiers , that you shouldn’t use. They don’t cause syntax errors because they are valid names. However, the language already uses them or reserves the right to use them, so it could cause problems if you use these identifiers to mean something else. Reserved identifiers include those beginning with an underscore character and the names of the standard library functions, such as printf() .
Summary
A C program consists of one or more C functions. Every C program must contain a function called main() because it is the function called when the program starts up. A simple function consists of a function header followed by an opening brace, followed by the statements constituting the function body, followed by a terminating, or closing , brace.
Each C statement is an instruction to the computer and is marked by a terminating semicolon. A declaration statement creates a name for a variable and identifies the type of data to be stored in the variable. The name of a variable is an example of an identifier. An assignment statement assigns a value to a variable or, more generally, to a storage area. A function call statement causes the named function to be executed. When the called function is done, the program returns to the next statement after the function call.
The printf() function can be used to print phrases and the values of variables.
The syntax of a language is the set of rules that governs the way in which valid statements in that language are put together. The semantics of a statement is its meaning. The compiler helps you detect syntax errors, but semantic errors show up in a program’s behavior only after it is compiled. Detecting semantic errors may involve tracing the program state—that is, the values of all variables—after each program step.
Finally, keywords are the vocabulary of the C language.