1.Dao层
1.1 添加方法
package com.csj2018.o2o.dao;
import java.util.List;
import com.csj2018.o2o.entity.ProductCategory;
public interface ProductCategoryDao {
/**
* 查询某个店铺下的商品分类
* @param shopId
* @return
*/
List<ProductCategory> queryProductCategoryList(long shopId);
/**
* 批量添加商品分类
* @param productCategoryList
* @return
*/
int batchInsertProductCategory(List<ProductCategory> productCategoryList);
}
1.2.添加mapper方法
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.csj2018.o2o.dao.ProductCategoryDao">
<select id="queryProductCategoryList" resultType="com.csj2018.o2o.entity.ProductCategory" parameterType="Long">
SELECT
product_category_id,
product_category_name,
priority,
create_time,
shop_id
from tb_product_category
where
shop_id = #{shopId}
order by priority desc
</select>
<!-- 7.3新增 -->
<insert id="batchInsertProductCategory" parameterType="java.util.List">
insert into
tb_product_category(product_category_name,priority,create_time,shop_id)
values
<foreach collection="list" item="productCategory" index="index" separator=",">
(
#{productCategory.productCategoryName},
#{productCategory.priority},
#{productCategory.createTime},
#{productCategory.shopId}
)
</foreach>
</insert>
</mapper>
1.3.单元测试
package com.csj2018.o2o.dao;
import static org.junit.Assert.assertEquals;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.junit.Ignore;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.entity.ProductCategory;
public class ProductCategoryDaoTest extends BaseTest {
@Autowired
private ProductCategoryDao productCategoryDao;
@Test
@Ignore
public void testQueryByShopId() throws Exception {
long shopId = 1;
List<ProductCategory> productCategoryList = productCategoryDao.queryProductCategoryList(shopId);
System.out.println("该店铺自定义商品类别数为:"+productCategoryList.size());
}
@Test
public void testBatchInsertProductCategory() {
ProductCategory productCategory1 = new ProductCategory();
productCategory1.setProductCategoryName("批量插入商品类别1");
productCategory1.setPriority(1);
productCategory1.setCreateTime(new Date());
productCategory1.setShopId(1L);
ProductCategory productCategory2 = new ProductCategory();
productCategory2.setProductCategoryName("批量插入商品类别2");
productCategory2.setPriority(2);
productCategory2.setCreateTime(new Date());
productCategory2.setShopId(1L);
List<ProductCategory> productCategoryList = new ArrayList<ProductCategory>();
productCategoryList.add(productCategory1);
productCategoryList.add(productCategory2);
int count = productCategoryDao.batchInsertProductCategory(productCategoryList);
assertEquals(2,count);
}
}
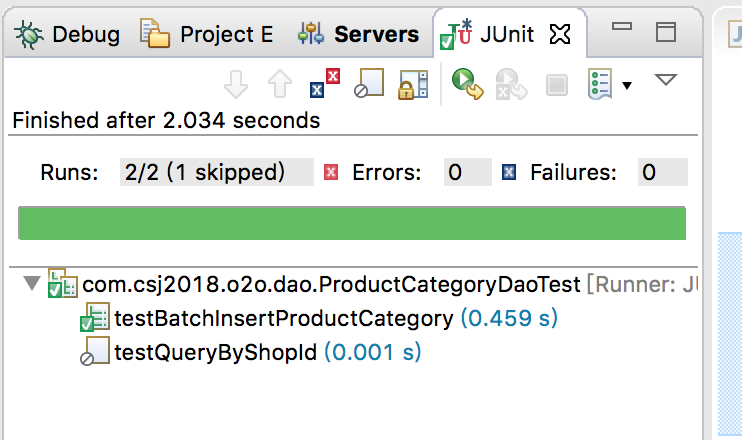
import java.util.List;
import com.csj2018.o2o.dto.ProductCategoryExecution;
import com.csj2018.o2o.entity.ProductCategory;
import com.csj2018.o2o.exceptions.ProductCategoryOperationException;
public interface ProductCategoryService {
/**
* 查询指定某个店铺下的所有商品类别信息
* @param shopId
* @return
/
List
/
* 批量插入商品类别
* @param productCategoryList
* @return
* @throws ProductCategoryOperationException
*/
ProductCategoryExecution batchAddProductCategory(List
}
### 2.2 创建接口中需要的店铺类别操作类
```#java
package com.csj2018.o2o.dto;
import java.util.List;
import com.csj2018.o2o.entity.ProductCategory;
import com.csj2018.o2o.enums.ProductCategoryStateEnum;
public class ProductCategoryExecution {
//结果状态
private int state;
//状态标识
private String stateInfo;
private List<ProductCategory> productCategoryList;
public ProductCategoryExecution() {};
//操作失败的时候使用的构造器
public ProductCategoryExecution(ProductCategoryStateEnum stateEnum) {
this.state = stateEnum.getState();
this.stateInfo = stateEnum.getStateInfo();
}
//操作成功时使用的构造器
public ProductCategoryExecution(ProductCategoryStateEnum stateEnum,List<ProductCategory> productCategoryList) {
this.state = stateEnum.getState();
this.stateInfo = stateEnum.getStateInfo();
this.productCategoryList = productCategoryList;
}
public int getState() {
return state;
}
public void setState(int state) {
this.state = state;
}
public String getStateInfo() {
return stateInfo;
}
public void setStateInfo(String stateInfo) {
this.stateInfo = stateInfo;
}
public List<ProductCategory> getProductCategoryList() {
return productCategoryList;
}
public void setProductCategoryList(List<ProductCategory> productCategoryList) {
this.productCategoryList = productCategoryList;
}
}
2.3商品类别异常类
package com.csj2018.o2o.exceptions;
public class ProductCategoryOperationException extends RuntimeException{
private static final long serialVersionUID = -4280159092691037667L;
public ProductCategoryOperationException(String msg) {
super(msg);
}
}
2.4 Service实现类
package com.csj2018.o2o.service.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.csj2018.o2o.dao.ProductCategoryDao;
import com.csj2018.o2o.dto.ProductCategoryExecution;
import com.csj2018.o2o.entity.ProductCategory;
import com.csj2018.o2o.enums.ProductCategoryStateEnum;
import com.csj2018.o2o.exceptions.ProductCategoryOperationException;
import com.csj2018.o2o.service.ProductCategoryService;
@Service
public class ProductCategoryServiceImpl implements ProductCategoryService{
@Autowired
private ProductCategoryDao productCategoryDao;
@Override
public List<ProductCategory> getProductCategoryList(long shopId) {
// TODO Auto-generated method stub
return productCategoryDao.queryProductCategoryList(shopId);
}
//7.3增加
@Override
@Transactional
public ProductCategoryExecution batchAddProductCategory(List<ProductCategory> productCategoryList)
throws ProductCategoryOperationException {
if(productCategoryList != null && productCategoryList.size() >0) {
try {
int effectedNum = productCategoryDao.batchInsertProductCategory(productCategoryList);
if(effectedNum <= 0) {
throw new ProductCategoryOperationException("店铺类别创建失败");
}else {
return new ProductCategoryExecution(ProductCategoryStateEnum.SUCCESS);
}
}catch (Exception e) {
throw new ProductCategoryOperationException("batchAddProductCategory error:" + e.getMessage());
}
}else {
return new ProductCategoryExecution(ProductCategoryStateEnum.EMPTY_LIST);
}
}
}
3.controller层
package com.csj2018.o2o.web.shopadmin;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.csj2018.o2o.dto.ProductCategoryExecution;
import com.csj2018.o2o.dto.Result;
import com.csj2018.o2o.entity.ProductCategory;
import com.csj2018.o2o.entity.Shop;
import com.csj2018.o2o.enums.ProductCategoryStateEnum;
import com.csj2018.o2o.exceptions.ProductCategoryOperationException;
import com.csj2018.o2o.service.ProductCategoryService;
@Controller
@RequestMapping("/shopadmin")
public class ProductCategoryManagementController {
@Autowired
private ProductCategoryService productCategoryService;
@RequestMapping(value="/getproductcategorylist",method=RequestMethod.GET)
@ResponseBody
private Result<List<ProductCategory>> getProductCategoryList(HttpServletRequest request){
//测试用,可删除
// Shop shop = new Shop();
// shop.setShopId(1L);
// request.getSession().setAttribute("currentShop", shop);
Shop currentShop = (Shop) request.getSession().getAttribute("currentShop");
List<ProductCategory> list = null;
if(currentShop.getShopId() != null && currentShop.getShopId()>0){
list = productCategoryService.getProductCategoryList(currentShop.getShopId());
return new Result<List<ProductCategory>>(true,list);
}else {
ProductCategoryStateEnum ps = ProductCategoryStateEnum.INNER_ERROR;
return new Result<List<ProductCategory>>(false,ps.getState(),ps.getStateInfo());
}
}
//7-3新增
@RequestMapping(value="/getproductcategorylist",method=RequestMethod.POST)
@ResponseBody
private Map<String,Object> addProductCategorys(@RequestBody List<ProductCategory> productCategoryList,HttpServletRequest request){
Map<String,Object> modelMap = new HashMap<String,Object>();
Shop currentShop = (Shop) request.getSession().getAttribute("currentShop");
for(ProductCategory pc:productCategoryList) {
pc.setShopId(currentShop.getShopId());
}
if(productCategoryList != null && productCategoryList.size()>0) {
try {
ProductCategoryExecution pe = productCategoryService.batchAddProductCategory(productCategoryList);
if(pe.getState() == ProductCategoryStateEnum.SUCCESS.getState()) {
modelMap.put("success", true);
}else {
modelMap.put("success", false);
modelMap.put("errMsg", pe.getStateInfo());
}
}catch (ProductCategoryOperationException e) {
modelMap.put("success", false);
modelMap.put("errMsg", e.toString());
}
}else {
modelMap.put("success", false);
modelMap.put("errMsg", "请输入至少一个商品类别");
}
return modelMap;
}
}
5.前端
/**
*
*/
$(function(){
var listUrl = "/o2o/shopadmin/getproductcategorylist";
var addUrl = "/o2o/shopadmin/addproductcategory";
var deleteUrl = "/o2o/shopadmin/removecategory";
getList();
function getList(){
$.getJSON(listUrl,function(data){
if(data.success){
var dataList = data.data;
$('.category-wrap').html('');
var tempHtml = '';
dataList.map(function(item,index){
tempHtml += ''
+'<div class="row row-product-category now">'
+'<div class="col-33 product-category-name">' + item.productCategoryName + '</div>'
+'<div class="col-33">' + item.priority +'</div>'
+'<div class="col-33"><a href="#" class="button delete" data-id="' + item.productCategoryId +'">删除</a></div>'
+'</div>';
});
$('.category-wrap').append(tempHtml);
}
});
}
//点击新建,添加一行
$('#new').click(function(){
var tempHtml = '<div class="row row-product-category temp">'
+ '<div class="col-33"><input class="category-input category" type="text" placeholder="分类名" /></div>'
+ '<div class="col-33"><input class="category-input priority" type="number" placeholder="优先级" /></div>'
+ '<div class="col-33"><a href="#" class="button delete">删除</a></div>'
+'</div>';
$('.category-wrap').append(tempHtml);
});
//点击提交,将temp的控件遍历获取,组成列表传到后台
$('#submit').click(function(){
var tempArr = $('.temp');
var productCategoryList = [];
tempArr.map(function(index,item){
var tempObj = {};
tempObj.productCategoryName = $(item).find('.category').val();
tempObj.priority = $(item).find('.priority').val();
if(tempObj.productCategoryName && tempObj.priority){
productCategoryList.push(tempObj);
}
});
$.ajax({
url:addUrl,
type:'POST',
data:JSON.stringify(productCategoryList),
contentType:'application/json',
success:function(data){
if(data.success){
$.toast("提交成功!");
getList();//更新后用于刷新列表
}else{
$.toast("提交失败");
}
}
});
});
})
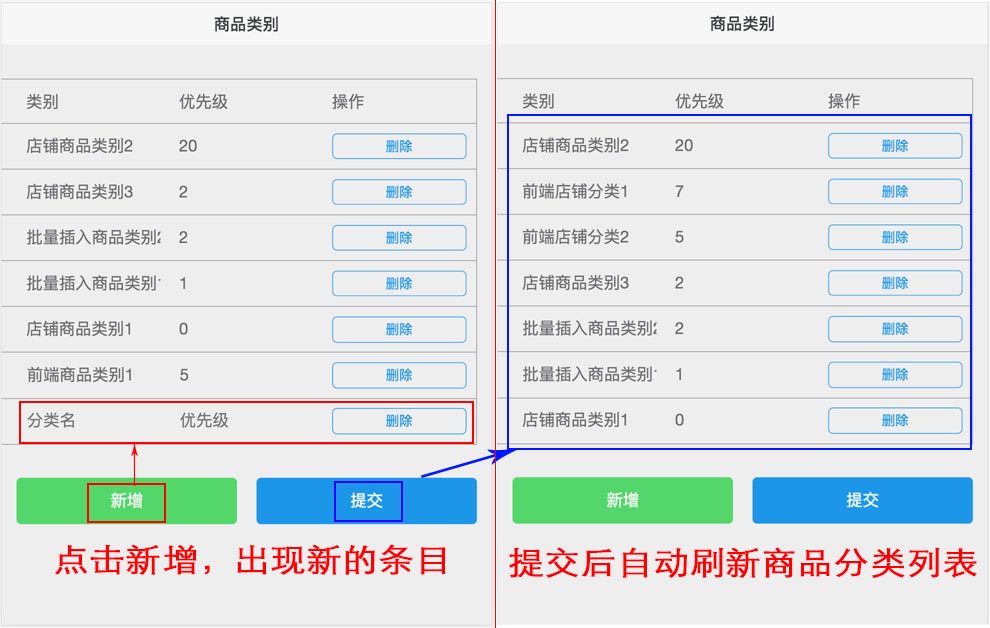