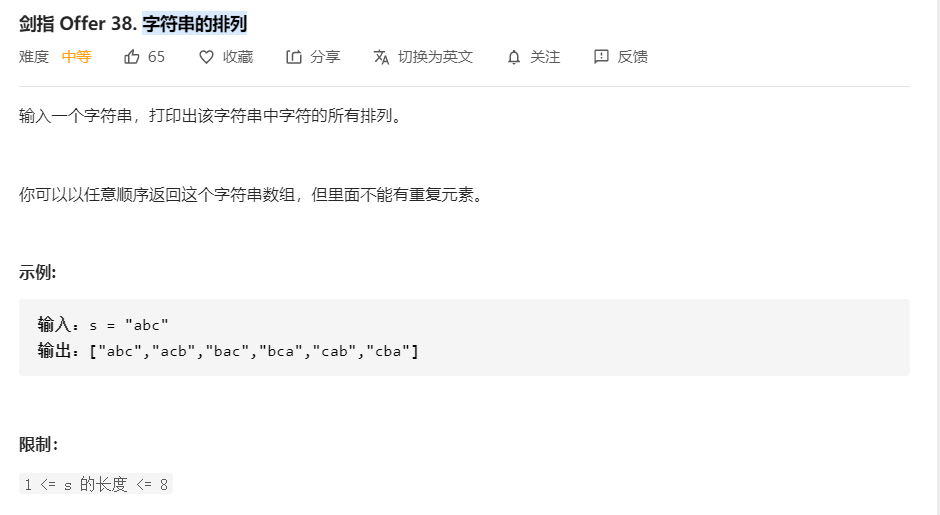
class Solution {
//全排列问题
Set<List<Character>> res = new HashSet<>();
public String[] permutation(String s) {
char []strs = s.toCharArray();
int len = strs.length;
boolean []used = new boolean[len];
LinkedList<Character> list = new LinkedList<>();
dfs(0,used,list,strs);
String[] result = new String[res.size()];
int top=0;
for(List<Character> elem : res){
String tmp = "";
LinkedList<Character> e = (LinkedList<Character>)elem;
for(int i=0;i<len;i++){
tmp+=e.get(i)+"";
}
result[top++] = tmp;
}
return result;
}
void dfs(int u,boolean []used,LinkedList list,char[] strs){
if(u == strs.length){
res.add(new LinkedList<>(list));
return;
}
for(int i=0;i<strs.length;i++){
if(!used[i]){
used[i] = true;
list.add(strs[i]);
dfs(u+1,used,list,strs);
used[i] = false;
list.removeLast();
}
}
}
}