Numpy:
NumPy(Numerical Python) 是科学计算基础库,提供大量科学计算相关功能,比如数据统计,随机数生成等。其提供最核心类型为多维数组类型(ndarray),支持大量的维度数组 与矩阵运算,Numpy 支持向量处理 ndarray 对象,提高程序运算速度。
安装 NumPy 最简单的方法就是使用 pip 工具,语法格式如下:pip install numpy
'''
使用array创建一/三维数组 ,返回 ndarray
'''
import numpy as np
a = np.array([1,2,4,6],dtype=float,ndmin =3)
print(a)
print(type(a))
'''
使用arange创建数组
'''
b =np.arange(1, 10, 2, dtype=float)
print(b)
print(type(b))
'''
随机生成 5,10的整数
'''
c =np.random.randint(5,11,size=4)
print(c)
'''
正态分布具有两个参数μ和σ^2的连续型随机变量的分布,
第一参数μ是服从正态分布的随机变量的均值(期望)
第二个参数σ^2是此随机变量的方差,所以正态分布记作N(μ,σ2)。
μ是正态分布的位置参数,描述正态分布的集中趋势位置。
概率规律为取与μ邻近的值的概率大,而取离μ越远的值的概率越小。
正态分布以X=μ为对称轴,左右完全对称。
σ越大,数据分布越分散,σ越小,数据分布越集中。也称为是正态分布的形状参数,
'''
d = np.random.randn(4)#randn表示标准的正态分布,期望0,方差为1
print(d)
e = np.random.normal(loc=2,scale=2,size=(2,3))#自定义期望和方差,越靠近2,越集中;标准差越大,幅度越大,越集中
print(e)
#索引
print("访问第三个元素:", a[2])
#切片:start:stop:step
print(a[2:5:2]) #从索引2开始到索引5结束,步长是2
#拷贝
b = np.arange(12).reshape(3,4)
c =np.copy(b)#深拷贝
c[0,0] = 100
print(b)
print(c)
#数组之间的转化,转化为一维、二维、三维
b = np.arange(12).reshape(3,4) #直接转化成行和列
np.arange(12).reshape((3,4))#转化为元组转化
np.reshape(a,(4,3,2))#将a一维数组转化成多维
b.reshape #将多维转化成一维
b.ravel #将多维转化成一维
b.flatten #将多维转化成一维
#数组的拼接
concatenate((a1,a2),axis =(1,2,4...))hstack(水平)vstack(垂直)
#数组的分割
numpy.split(ary, indices_or_sections, axis) p.hsplit(X,3) p.vsplit(X,2)
#数组的转换
transpose 进行转换
NumPy 算术函数包含简单的加减乘除: add(),subtract(),multiply() 和 divide()。
NumPy 提供了标准的三角函数:sin()、cos()、tan()。
NumPy 提供了:around、floor、ceil的使用
聚合函数:
NumPy 提供了很多统计函数,用于从数组中查找最小元素,最大元素,百分位标准差和方差等。
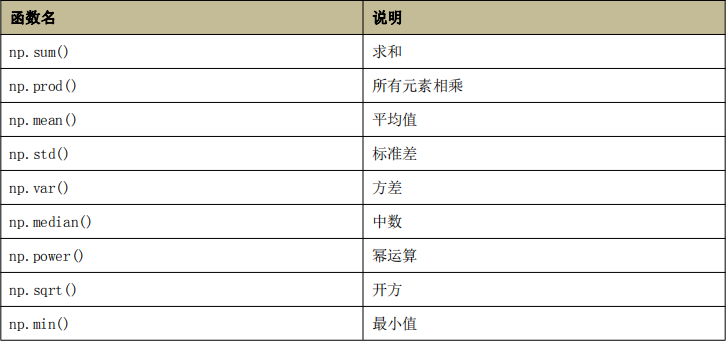
numpy.power() 函数将第一个输入数组中的元素作为底数,计算它与第二个输入数组中相应元素的幂。
numpy. median ()函数的使用,求中位数。
numpy.mean() 函数返回数组中元素的算术平均值。