{相关结构:} TDSFXCompressor = = packed record fGain: Single; // -60 .. 60 : 0 (dB) fAttack: Single; //0.01 .. 500 : 10 (ms) fRelease: Single; // 50 .. 3000 : 200(ms) fThreshold: Single; // -60 .. 0 : -20(dB) fRatio: Single; // 1 .. 100 : 3 (%) fPredelay: Single; // 0 .. 4 : 4 (ms) end; //该结构相关常量: DSFXCOMPRESSOR_GAIN_MIN = -60.0; DSFXCOMPRESSOR_GAIN_MAX = 60.0; DSFXCOMPRESSOR_ATTACK_MIN = 0.01; DSFXCOMPRESSOR_ATTACK_MAX = 500.0; DSFXCOMPRESSOR_RELEASE_MIN = 50.0; DSFXCOMPRESSOR_RELEASE_MAX = 3000.0; DSFXCOMPRESSOR_THRESHOLD_MIN = -60.0; DSFXCOMPRESSOR_THRESHOLD_MAX = 0.0; DSFXCOMPRESSOR_RATIO_MIN = 1.0; DSFXCOMPRESSOR_RATIO_MAX = 100.0; DSFXCOMPRESSOR_PREDELAY_MIN = 0.0; DSFXCOMPRESSOR_PREDELAY_MAX = 4.0;
测试代码:
unit Unit1; interface uses Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms, Dialogs, StdCtrls, ExtCtrls; type TForm1 = class(TForm) Button1: TButton; Button2: TButton; LabeledEdit1: TLabeledEdit; LabeledEdit2: TLabeledEdit; LabeledEdit3: TLabeledEdit; LabeledEdit4: TLabeledEdit; LabeledEdit5: TLabeledEdit; LabeledEdit6: TLabeledEdit; procedure FormCreate(Sender: TObject); procedure FormDestroy(Sender: TObject); procedure Button1Click(Sender: TObject); procedure Button2Click(Sender: TObject); procedure LabeledEdit1Change(Sender: TObject); end; var Form1: TForm1; implementation {$R *.dfm} uses DirectSound, ReadWave2; //ReadWave2 是前面(9)自定义的单元 var myDSound: IDirectSound8; buf8: IDirectSoundBuffer8; fxCompressor: IDirectSoundFXCompressor8; //IDirectSoundFXCompressor8 效果器 procedure TForm1.FormCreate(Sender: TObject); begin System.ReportMemoryLeaksOnShutdown := True; DirectSoundCreate8(nil, myDSound, nil); myDSound.SetCooperativeLevel(Handle, DSSCL_NORMAL); Button1.Caption := '打开并播放'; Button2.Caption := '停止播放'; LabeledEdit1.EditLabel.Caption := 'fGain: -60 - 60'; LabeledEdit1.Text := '0.0'; LabeledEdit1.Tag := 1; LabeledEdit2.EditLabel.Caption := 'fAttack: 0.01 - 500'; LabeledEdit2.Text := '10.0'; LabeledEdit2.Tag := 2; LabeledEdit3.EditLabel.Caption := 'fRelease: 50 - 3000'; LabeledEdit3.Text := '200.0'; LabeledEdit3.Tag := 3; LabeledEdit4.EditLabel.Caption := 'fThreshold: -60 - 0'; LabeledEdit4.Text := '-20.0'; LabeledEdit4.Tag := 4; LabeledEdit5.EditLabel.Caption := 'fRatio: 1 - 100'; LabeledEdit5.Text := '3.0'; LabeledEdit5.Tag := 5; LabeledEdit6.EditLabel.Caption := 'fPredelay: 0 - 4'; LabeledEdit6.Text := '4.0'; LabeledEdit6.Tag := 6; LabeledEdit2.OnChange := LabeledEdit1.OnChange; LabeledEdit3.OnChange := LabeledEdit1.OnChange; LabeledEdit4.OnChange := LabeledEdit1.OnChange; LabeledEdit5.OnChange := LabeledEdit1.OnChange; LabeledEdit6.OnChange := LabeledEdit1.OnChange; end; procedure TForm1.Button1Click(Sender: TObject); var buf: IDirectSoundBuffer; bufDesc: TDSBufferDesc; rEffect: TDSEffectDesc; wav: TReadWave; p1: Pointer; n1: DWORD; begin wav := TReadWave.Create; if not wav.OpenDialog then begin wav.Free; Exit; end; ZeroMemory(@bufDesc, SizeOf(TDSBufferDesc)); bufDesc.dwSize := SizeOf(TDSBufferDesc); bufDesc.dwFlags := DSBCAPS_CTRLFX; bufDesc.dwBufferBytes := wav.Size; bufDesc.lpwfxFormat := @wav.Format; myDSound.CreateSoundBuffer(bufDesc, buf, nil); buf.QueryInterface(IID_IDirectSoundBuffer8, buf8); buf8.Lock(0, 0, @p1, @n1, nil, nil, DSBLOCK_ENTIREBUFFER); wav.Read(p1, n1); wav.Free; buf8.Unlock(p1, n1, nil, 0); ZeroMemory(@rEffect, SizeOf(TDSEffectDesc)); rEffect.dwSize := SizeOf(TDSEffectDesc); rEffect.dwFlags := 0; rEffect.guidDSFXClass := GUID_DSFX_STANDARD_COMPRESSOR; buf8.SetFX(1, @rEffect, nil); buf8.GetObjectInPath(GUID_DSFX_STANDARD_COMPRESSOR, 0, IID_IDirectSoundFXCompressor8, fxCompressor); buf8.Play(0, 0, DSBPLAY_LOOPING); end; procedure TForm1.Button2Click(Sender: TObject); begin if Assigned(buf8) then buf8.Stop; end; procedure TForm1.LabeledEdit1Change(Sender: TObject); var rCompressor: TDSFXCompressor; f: Single; obj: TLabeledEdit; begin obj := Sender as TLabeledEdit; if obj.Text = '-' then Exit; f := StrToFloatDef(obj.Text, MaxInt); case obj.Tag of 1: if (f < -60) or (f > 60) then obj.Text := '0.0'; 2: if (f < 0.01) or (f > 500) then obj.Text := '10.0'; 3: if (f < 50) then Exit else if (f > 3000) then obj.Text := '200.0'; 4: if (f < -60) or (f > 0) then obj.Text := '-20.0'; 5: if (f < 1) or (f > 100) then obj.Text := '3.0'; 6: if (f < 0) or (f > 4) then obj.Text := '4.0'; end; if buf8 = nil then Exit; rCompressor.fGain := StrToFloat(LabeledEdit1.Text); rCompressor.fAttack := StrToFloat(LabeledEdit2.Text); rCompressor.fRelease := StrToFloat(LabeledEdit3.Text); rCompressor.fThreshold := StrToFloat(LabeledEdit4.Text); rCompressor.fRatio := StrToFloat(LabeledEdit5.Text); rCompressor.fPredelay := StrToFloat(LabeledEdit6.Text); fxCompressor.SetAllParameters(rCompressor); end; procedure TForm1.FormDestroy(Sender: TObject); begin buf8 := nil; myDSound := nil; end; end.
窗体设计:
object Form1: TForm1 Left = 0 Top = 0 Caption = 'Form1' ClientHeight = 307 ClientWidth = 306 Color = clBtnFace Font.Charset = DEFAULT_CHARSET Font.Color = clWindowText Font.Height = -11 Font.Name = 'Tahoma' Font.Style = [] OldCreateOrder = False OnCreate = FormCreate OnDestroy = FormDestroy PixelsPerInch = 96 TextHeight = 13 object Button1: TButton Left = 32 Top = 28 Width = 75 Height = 25 Caption = 'Button1' TabOrder = 0 OnClick = Button1Click end object Button2: TButton Left = 32 Top = 75 Width = 75 Height = 25 Caption = 'Button2' TabOrder = 1 OnClick = Button2Click end object LabeledEdit1: TLabeledEdit Left = 144 Top = 30 Width = 121 Height = 21 EditLabel.Width = 61 EditLabel.Height = 13 EditLabel.Caption = 'LabeledEdit1' TabOrder = 2 OnChange = LabeledEdit1Change end object LabeledEdit2: TLabeledEdit Left = 144 Top = 77 Width = 121 Height = 21 EditLabel.Width = 61 EditLabel.Height = 13 EditLabel.Caption = 'LabeledEdit2' TabOrder = 3 end object LabeledEdit3: TLabeledEdit Left = 144 Top = 125 Width = 121 Height = 21 EditLabel.Width = 61 EditLabel.Height = 13 EditLabel.Caption = 'LabeledEdit3' TabOrder = 4 end object LabeledEdit4: TLabeledEdit Left = 144 Top = 173 Width = 121 Height = 21 EditLabel.Width = 61 EditLabel.Height = 13 EditLabel.Caption = 'LabeledEdit4' TabOrder = 5 end object LabeledEdit5: TLabeledEdit Left = 144 Top = 221 Width = 121 Height = 21 EditLabel.Width = 61 EditLabel.Height = 13 EditLabel.Caption = 'LabeledEdit5' TabOrder = 6 end object LabeledEdit6: TLabeledEdit Left = 144 Top = 269 Width = 121 Height = 21 EditLabel.Width = 61 EditLabel.Height = 13 EditLabel.Caption = 'LabeledEdit6' TabOrder = 7 end end
运行效果图:
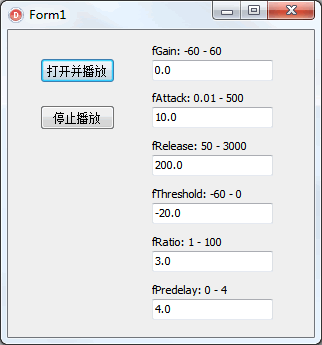