Spring中AOP相关术语
Spring中AOP的代理选择
Spring 实现AOP思想使⽤的是动态代理技术
默认情况下,Spring会根据被代理对象是否实现接⼝来选择使⽤JDK还是CGLIB。当被代理对象没有实现
任何接⼝时,Spring会选择CGLIB。当被代理对象实现了接⼝,Spring会选择JDK官⽅的代理技术,不过
我们可以通过配置的⽅式,让Spring强制使⽤CGLIB。
Spring中AOP的配置⽅式
在Spring的AOP配置中,也和IoC配置⼀样,⽀持3类配置⽅式。
第⼀类:使⽤XML配置
第⼆类:使⽤XML+注解组合配置
第三类:使⽤纯注解配置
Spring中AOP实现
XML 模式
Spring是模块化开发的框架,使⽤aop就引⼊aop的jar
1、坐标
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>5.1.12.RELEASE</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.4</version> </dependency>
package com.lagou.edu.utils;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
/**
* @Author: denghy
* @DateTime: 2021/3/19 11:39
* @Description: TODO
* @package: com.lagou.edu.utils
*/
public class LogUtils {
// @Pointcut("execution(* com.lagou.edu.service.impl.TransferServiceImpl.*(..))")
// public void pt1(){
//
// }
/**
* 业务逻辑开始之前执行
*/
// @Before("pt1()")
public void beforeMethod(JoinPoint joinPoint) {
Object[] args = joinPoint.getArgs();
for (int i = 0; i < args.length; i++) {
Object arg = args[i];
System.out.println(arg);
}
System.out.println("业务逻辑开始执行之前执行.......");
}
/**
* 业务逻辑结束时执行(无论异常与否)
*/
// @After("pt1()")
public void afterMethod() {
System.out.println("业务逻辑结束时执行,无论异常与否都执行.......");
}
/**
* 异常时时执行
*/
// @AfterThrowing("pt1()")
public void exceptionMethod() {
System.out.println("异常时执行.......");
}
/**
* 业务逻辑正常时执行
*/
// @AfterReturning(value = "pt1()",returning = "retVal")
public void successMethod(Object retVal) {
System.out.println("业务逻辑正常时执行.......");
}
/**
* 环绕通知
*
*/
/*@Around("pt1()")*/
public Object arroundMethod(ProceedingJoinPoint proceedingJoinPoint) throws Throwable {
System.out.println("环绕通知中的beforemethod....");
Object result = null;
try{
// 控制原有业务逻辑是否执行
// result = proceedingJoinPoint.proceed(proceedingJoinPoint.getArgs());
}catch(Exception e) {
System.out.println("环绕通知中的exceptionmethod....");
}finally {
System.out.println("环绕通知中的after method....");
}
return result;
}
}
2、AOP 核⼼配置
<!-- Spring基于XML的AOP配置前期准备: 在spring的配置⽂件中加⼊aop的约束 xmlns:aop="http://www.springframework.org/schema/aop" http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd Spring基于XML的AOP配置步骤: 第⼀步:把通知Bean交给Spring管理 第⼆步:使⽤aop:config开始aop的配置 第三步:使⽤aop:aspect配置切⾯ 第四步:使⽤对应的标签配置通知的类型 ⼊⻔案例采⽤前置通知,标签为aop:before --> <!--进行aop相关的xml配置,配置aop的过程其实就是把aop相关术语落地--> <!--横切逻辑bean--> <bean id="logUtils" class="com.lagou.edu.utils.LogUtils"></bean> <!--使用config标签表明开始aop配置,在内部配置切面aspect--> <!--aspect = 切入点(锁定方法) + 方位点(锁定方法中的特殊时机)+ 横切逻辑 --> <aop:config> <aop:aspect id="logAspect" ref="logUtils"> <!--切入点锁定我们感兴趣的方法,使用aspectj语法表达式--> <!--<aop:pointcut id="pt1" expression="execution(* *..*.*(..))"/>--> <aop:pointcut id="pt1" expression="execution(* com.lagou.edu.service.impl.TransferServiceImpl.*(..))"/> <!--方位信息,pointcut-ref关联切入点--> <!--aop:before前置通知/增强--> <aop:before method="beforeMethod" pointcut-ref="pt1"/> <!--aop:after,最终通知,无论如何都执行--> <!--aop:after-returnning,正常执行通知--> <!--<aop:after-returning method="successMethod" returning="retValue"/>--> <!--aop:after-throwing,异常通知--> <!--<aop:around method="arroundMethod" pointcut-ref="pt1"/>--> </aop:aspect> </aop:config>
3、测试类
/**
* 测试xml-anno aop
*/
@Test
public void testXmlAnnoAop() throws Exception {
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext("classpath:beans.xml");
TransferService transferService = applicationContext.getBean(TransferService.class);
transferService.transfer("6029621011000","6029621011001",100);
}
在前⾯我们已经说了,Spring在选择创建代理对象时,会根据被代理对象的实际情况来选择的。被代理对象实现了接⼝,则采⽤基于接⼝的动态代理。当被代理对象没有实现任何接⼝
的时候,Spring会⾃动切换到基于⼦类的动态代理⽅式。但是我们都知道,⽆论被代理对象是否实现接⼝,只要不是fifinal修饰的类都可以采⽤cglib提
供的⽅式创建代理对象。所以Spring也考虑到了这个情况,提供了配置的⽅式实现强制使⽤基于⼦类的动态代理(即cglib的⽅式),配置的⽅式有两种
使⽤aop:confifig标签配置
<aop:config proxy-target-class="true">
使⽤aop:aspectj-autoproxy标签配置
<!--此标签是基于XML和注解组合配置AOP时的必备标签,表示Spring开启注解配置AOP的⽀持-->
<!--此标签是基于XML和注解组合配置AOP时的必备标签,表示Spring开启注解配置AOP 的⽀持--> <aop:aspectj-autoproxy proxy-target-class="true"></aop:aspectjautoproxy>
五种通知类型
1、前置通知
配置⽅式:aop:before标签
作⽤:
⽤于配置前置通知。
出现位置:
它只能出现在aop:aspect标签内部
属性:
method:⽤于指定前置通知的⽅法名称
pointcut:⽤于指定切⼊点表达式
pointcut-ref:⽤于指定切⼊点表达式的引⽤
-->
<aop:before method="printLog" pointcut-ref="pointcut1"></aop:before>
执⾏时机
前置通知永远都会在切⼊点⽅法(业务核⼼⽅法)执⾏之前执⾏。
细节
前置通知可以获取切⼊点⽅法的参数,并对其进⾏增强。
2、正常执⾏时通知
<!-- 作⽤: ⽤于配置正常执⾏时通知 出现位置: 它只能出现在aop:aspect标签内部 属性: method:⽤于指定后置通知的⽅法名称 pointcut:⽤于指定切⼊点表达式 pointcut-ref:⽤于指定切⼊点表达式的引⽤ --> <aop:after-returning method="afterReturningPrintLog" pointcutref="pt1"></aop:after-returning>
3、异常通知
<!-- 作⽤: ⽤于配置异常通知。 出现位置: 它只能出现在aop:aspect标签内部 属性: method:⽤于指定异常通知的⽅法名称 pointcut:⽤于指定切⼊点表达式 pointcut-ref:⽤于指定切⼊点表达式的引⽤ --> <aop:after-throwing method="afterThrowingPrintLog" pointcut-ref="pt1"></aop:after-throwing>
执⾏时机
异常通知的执⾏时机是在切⼊点⽅法(业务核⼼⽅法)执⾏产⽣异常之后,异常通知执⾏。如果切
⼊点⽅法执⾏没有产⽣异常,则异常通知不会执⾏。
细节
异常通知不仅可以获取切⼊点⽅法执⾏的参数,也可以获取切⼊点⽅法执⾏产⽣的异常信息。
4、最终通知
<!-- 作⽤: ⽤于指定最终通知。 出现位置: 它只能出现在aop:aspect标签内部 属性: method:⽤于指定最终通知的⽅法名称 pointcut:⽤于指定切⼊点表达式 pointcut-ref:⽤于指定切⼊点表达式的引⽤ --> <aop:after method="afterPrintLog" pointcut-ref="pt1"></aop:after>
执⾏时机
最终通知的执⾏时机是在切⼊点⽅法(业务核⼼⽅法)执⾏完成之后,切⼊点⽅法返回之前执⾏。
换句话说,⽆论切⼊点⽅法执⾏是否产⽣异常,它都会在返回之前执⾏。
细节
最终通知执⾏时,可以获取到通知⽅法的参数。同时它可以做⼀些清理操作。
5、环绕通知
<!-- 作⽤: ⽤于配置环绕通知。 出现位置: 它只能出现在aop:aspect标签的内部 属性: method:⽤于指定环绕通知的⽅法名称 pointcut:⽤于指定切⼊点表达式 pointcut-ref:⽤于指定切⼊点表达式的引⽤ --> <aop:around method="aroundPrintLog" pointcut-ref="pt1"></aop:around>
**特别说明**
环绕通知,它是有别于前⾯四种通知类型外的特殊通知。前⾯四种通知(前置,后置,异常和最终)它们都是指定何时增强的通知类型。⽽环绕通知,它是Spring框架为我们提供的⼀种可以通过编码的
⽅式,控制增强代码何时执⾏的通知类型。它⾥⾯借助的ProceedingJoinPoint接⼝及其实现类,实现⼿动触发切⼊点⽅法的调⽤。
**ProceedingJoinPoint接⼝介绍
XML+注解模式
1、bean.xml
<!--开启aop注解驱动 proxy-target-class:true强制使用cglib --> <aop:aspectj-autoproxy/>
2、logUtil
package com.lagou.edu.utils;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
import org.springframework.stereotype.Component;
/**
* @Author: denghy
* @DateTime: 2021/3/19 11:39
* @Description: TODO
* @package: com.lagou.edu.utils
*/
@Component //替代 <bean id="logUtils" class="com.lagou.edu.utils.LogUtils"></bean>
@Aspect //替代 <aop:aspect id="logAspect" ref="logUtils">
public class LogUtils {
/*<aop:pointcut id="pt1" expression="execution(* com.lagou.edu.service.impl.TransferServiceImpl.*(..))"/>*/
@Pointcut("execution(* com.lagou.edu.service.impl.TransferServiceImpl.*(..))")
public void pt1(){
}
/**
* 业务逻辑开始之前执行
*/
@Before("pt1()")
public void beforeMethod(JoinPoint joinPoint) {
Object[] args = joinPoint.getArgs();
for (int i = 0; i < args.length; i++) {
Object arg = args[i];
System.out.println(arg);
}
System.out.println("业务逻辑开始执行之前执行.......");
}
/**
* 业务逻辑结束时执行(无论异常与否)
*/
@After("pt1()")
public void afterMethod() {
System.out.println("业务逻辑结束时执行,无论异常与否都执行.......");
}
/**
* 异常时时执行
*/
@AfterThrowing("pt1()")
public void exceptionMethod() {
System.out.println("异常时执行.......");
}
/**
* 业务逻辑正常时执行
*/
@AfterReturning(value = "pt1()",returning = "retVal")
public void successMethod(Object retVal) {
System.out.println("业务逻辑正常时执行.......");
}
/**
* 环绕通知
*
*/
@Around("pt1()")
public Object arroundMethod(ProceedingJoinPoint proceedingJoinPoint) throws Throwable {
System.out.println("环绕通知中的beforemethod....");
Object result = null;
try{
// 控制原有业务逻辑是否执行
System.out.println("环绕通知中的控制原有业务逻辑是否执行....");
result = proceedingJoinPoint.proceed(proceedingJoinPoint.getArgs());
}catch(Exception e) {
System.out.println("环绕通知中的exceptionmethod....");
}finally {
System.out.println("环绕通知中的after method....");
}
return result;
}
}
3、测试
注解模式
在配置类上
@EnableAspectJAutoProxy
Spring 声明式事务的⽀持
编程式事务:在业务代码中添加事务控制代码,这样的事务控制机制就叫做编程式事务
声明式事务:通过xml或者注解配置的⽅式达到事务控制的⽬的,叫做声明式事务
事务回顾
事务的概念
事务指逻辑上的⼀组操作,组成这组操作的各个单元,要么全部成功,要么全部不成功。从⽽确保了数
据的准确与安全。
/*转出账户减钱*/
update account set money=money-100 where name=‘a’;
/**转⼊账户加钱*/
update account set money=money+100 where name=‘b’;
这两条语句的执⾏,要么全部成功,要么全部不成功。
事务的四⼤特性
原⼦性(Atomicity)
原⼦性是指事务是⼀个不可分割的⼯作单位,事务中的操作要么都发⽣,要么都不发⽣。
从操作的⻆度来描述,事务中的各个操作要么都成功要么都失败
⼀致性(Consistency)
事务必须使数据库从⼀个⼀致性状态变换到另外⼀个⼀致性状态。
例如转账前A有1000,B有1000。转账后A+B也得是2000。
⼀致性是从数据的⻆度来说的,(1000,1000) (900,1100),不应该出现(900,1000)
隔离性(Isolation)
事务的隔离性是多个⽤户并发访问数据库时,数据库为每⼀个⽤户开启的事务,每个事务不能被其他事务的操作数据所⼲扰,多个并发事务之间要相互隔离。
⽐如:事务1给员⼯涨⼯资2000,但是事务1尚未被提交,员⼯发起事务2查询⼯资,发现⼯资涨了2000
块钱,读到了事务1尚未提交的数据(脏读)
持久性(Durability)
持久性是指⼀个事务⼀旦被提交,它对数据库中数据的改变就是永久性的,接下来即使数据库发⽣故障
也不应该对其有任何影响
事务的隔离级别
不考虑隔离级别,会出现以下情况:(以下情况全是错误的),也即为隔离级别在解决事务并发问题
脏读:⼀个线程中的事务读到了另外⼀个线程中未提交的数据。
场景:
事务1给员⼯涨⼯资2000,但是事务1尚未被提交,员⼯发起事务2查询⼯资,发现⼯资涨了2000块钱,读到了事务1尚未提交的数据(脏读)
不可重复读:⼀个线程中的事务读到了另外⼀个线程中已经提交的update的数据(前后内容不⼀样)
场景:
员⼯A发起事务1,查询⼯资,⼯资为1w,此时事务1尚未关闭
财务⼈员发起了事务2,给员⼯A张了2000块钱,并且提交了事务
员⼯A通过事务1再次发起查询请求,发现⼯资为1.2w,原来读出来1w读不到了,叫做不可重复读
虚读(幻读):⼀个线程中的事务读到了另外⼀个线程中已经提交的insert或者delete的数据(前后条数不⼀样)
场景:
事务1查询所有⼯资为1w的员⼯的总数,查询出来了10个⼈,此时事务尚未关闭
事务2财务⼈员发起,新来员⼯,⼯资1w,向表中插⼊了2条数据,并且提交了事务
事务1再次查询⼯资为1w的员⼯个数,发现有12个⼈,⻅了⻤了
数据库共定义了四种隔离级别:
Serializable(串⾏化):可避免脏读、不可重复读、虚读情况的发⽣。(串⾏化) 最⾼
事务隔离一个一个排队处理,类似 ATM机 一个个处理
Repeatable read(可重复读):可避免脏读、不可重复读情况的发⽣。(幻读有可能发⽣) 第⼆
该机制下会对要update的⾏,进⾏加锁,幻读是,insert /delete 造成的
Read committed(读已提交):可避免脏读情况发⽣。不可重复读和幻读⼀定会发⽣。 第三
Read uncommitted(读未提交):最低级别,以上情况均⽆法保证。(读未提交) 最低
未提交的都进行提交
注意:级别依次升⾼,效率依次降低
MySQL的默认隔离级别是:REPEATABLE READ
查询当前使⽤的隔离级别: select @@tx_isolation;
设置MySQL事务的隔离级别: set session transaction isolation level xxx; (设置的是当前
mysql连接会话的,并不是永久改变的)
事务的传播⾏为
事务往往在service层进⾏控制,如果出现service层⽅法A调⽤了另外⼀个service层⽅法B,A和B⽅法本
身都已经被添加了事务控制,那么A调⽤B的时候,就需要进⾏事务的⼀些协商,这就叫做事务的传播⾏为。
A调⽤B,我们站在B的⻆度来观察来定义事务的传播⾏为
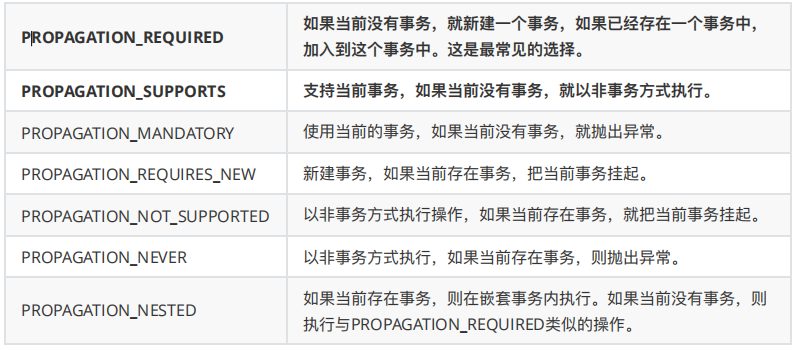
Spring中事务的API
mybatis: sqlSession.commit();
hibernate: session.commit();
PlatformTransactionManager
public interface PlatformTransactionManager {
/* 获取事务状态信息
*/
TransactionStatus getTransaction(@Nullable TransactionDefinition definition)
throws TransactionException;
/**
* 提交事务
*/
void commit(TransactionStatus status) throws TransactionException;
/**
* 回滚事务
*/
void rollback(TransactionStatus status) throws TransactionException; }
此接⼝是Spring的事务管理器核⼼接⼝。
Spring本身并不⽀持事务实现,只是负责提供标准,应⽤底层⽀持什么样的事务,需要提供具体实现类。
此处也是策略模式的具体应⽤。在Spring框架中,也为我们内置了⼀些具体策略,
例如:DataSourceTransactionManager , HibernateTransactionManager 等等。( 和 HibernateTransactionManager 事务管理器在 spring-orm-5.1.12.RELEASE.jar 中)
Spring JdbcTemplate(数据库操作⼯具)、Mybatis(mybatis-spring.jar)————>DataSourceTransactionManagerHibernate框架 ——————> HibernateTransactionManagerDataSourceTransactionManager
归根结底是横切逻辑代码,声明式事务要做的就是使⽤Aop(动态代理)来将事务控制逻辑织⼊到业务代码
Spring 声明式事务配置
纯xml模式
1、添加依赖
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.1.12.RELEASE</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.4</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>5.1.12.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-tx</artifactId> <version>5.1.12.RELEASE</version> </dependency>
2、bean.xml配置
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd http://www.springframework.org/schema/tx https://www.springframework.org/schema/tx/spring-tx.xsd "> <!--开启注解扫描,base-package指定扫描的包路径--> <context:component-scan base-package="com.lagou.edu"/> <!--引入外部资源文件--> <context:property-placeholder location="classpath:jdbc.properties"/> <!--第三方jar中的bean定义在xml中--> <bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource"> <property name="driverClassName" value="${jdbc.driver}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </bean> <bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate"> <constructor-arg name="dataSource" ref="dataSource"/> </bean> <!--spring声明式事务配置,声明式事务无非就是配置一个aop,只不过有些标签不一样罢了--> <!--横切逻辑--> <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <constructor-arg name="dataSource" ref="dataSource"></constructor-arg> </bean> <tx:advice id="txAdvice" transaction-manager="transactionManager"> <!-- 定制事务细节,传播行为、隔离级别等 --> <tx:attributes> <!-- 一般性配置--> <tx:method name="*" read-only="false" propagation="REQUIRED" isolation="DEFAULT" timeout="-1"/> <!--针对查询的覆盖性配置--> <tx:method name="query*" read-only="true" propagation="SUPPORTS"/> </tx:attributes> </tx:advice> <aop:config> <!--advice-ref指向增强=横切逻辑+方位--> <aop:advisor advice-ref="txAdvice" pointcut="execution(* com.lagou.edu.service.impl.TransferServiceImpl.*(..))"/> </aop:config> </beans>
3、测试
事务得到控制
基于XML+注解
1.beans.xml配置文件
<!--spring声明式事务配置,声明式事务无非就是配置一个aop,只不过有些标签不一样罢了--> <!--横切逻辑--> <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <constructor-arg name="dataSource" ref="dataSource"></constructor-arg> </bean> <!--开启spring对注解事务的⽀持--> <tx:annotation-driven transaction-manager="transactionManager"/> <!--<tx:advice id="txAdvice" transaction-manager="transactionManager"> <!– 定制事务细节,传播行为、隔离级别等 –> <tx:attributes> <!– 一般性配置–> <tx:method name="*" read-only="false" propagation="REQUIRED" isolation="DEFAULT" timeout="-1"/> <!–针对查询的覆盖性配置–> <tx:method name="query*" read-only="true" propagation="SUPPORTS"/> </tx:attributes> </tx:advice>
2、业务实现类添加注解
@Transactional
基于纯注解
Spring基于注解驱动开发的事务控制配置,只需要把 xml 配置部分改为注解实现。只是需要⼀个
注解替换掉xml配置⽂件中的 <tx:annotation-driven transaction
manager="transactionManager"/> 配置。
在 Spring 的配置类上添加 @EnableTransactionManagement 注解即可
@EnableTransactionManagement//开启spring注解事务的⽀持
public class SpringConfiguration {
}