题目链接:http://poj.org/problem?id=3522
Time Limit: 5000MS Memory Limit: 65536K
Description
Given an undirected weighted graph G, you should find one of spanning trees specified as follows.
The graph G is an ordered pair (V, E), where V is a set of vertices {v1, v2, …, vn} and E is a set of undirected edges {e1, e2, …, em}. Each edge e ∈ E has its weight w(e).
A spanning tree T is a tree (a connected subgraph without cycles) which connects all the n vertices with n − 1 edges. The slimness of a spanning tree T is defined as the difference between the largest weight and the smallest weight among the n − 1 edges of T.
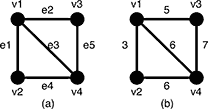
Figure 5: A graph G and the weights of the edges
For example, a graph G in Figure 5(a) has four vertices {v1, v2, v3, v4} and five undirected edges {e1, e2, e3, e4, e5}. The weights of the edges are w(e1) = 3, w(e2) = 5, w(e3) = 6, w(e4) = 6, w(e5) = 7 as shown in Figure 5(b).
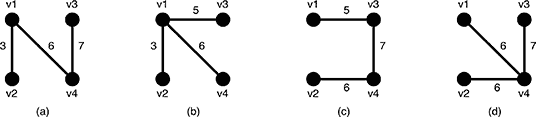
Figure 6: Examples of the spanning trees of G
There are several spanning trees for G. Four of them are depicted in Figure 6(a)~(d). The spanning tree Ta in Figure 6(a) has three edges whose weights are 3, 6 and 7. The largest weight is 7 and the smallest weight is 3 so that the slimness of the tree Ta is 4. The slimnesses of spanning trees Tb, Tc and Td shown in Figure 6(b), (c) and (d) are 3, 2 and 1, respectively. You can easily see the slimness of any other spanning tree is greater than or equal to 1, thus the spanning tree Td in Figure 6(d) is one of the slimmest spanning trees whose slimness is 1.
Your job is to write a program that computes the smallest slimness.
Input
The input consists of multiple datasets, followed by a line containing two zeros separated by a space. Each dataset has the following format.
n | m | |
a1 | b1 | w1 |
⋮ | ||
am | bm | wm |
Every input item in a dataset is a non-negative integer. Items in a line are separated by a space. n is the number of the vertices and m the number of the edges. You can assume 2 ≤ n ≤ 100 and 0 ≤ m ≤ n(n − 1)/2. ak and bk (k = 1, …, m) are positive integers less than or equal to n, which represent the two vertices vak and vbk connected by the kth edge ek. wk is a positive integer less than or equal to 10000, which indicates the weight of ek. You can assume that the graph G = (V, E) is simple, that is, there are no self-loops (that connect the same vertex) nor parallel edges (that are two or more edges whose both ends are the same two vertices).
Output
For each dataset, if the graph has spanning trees, the smallest slimness among them should be printed. Otherwise, −1 should be printed. An output should not contain extra characters.
Sample Input
4 5 1 2 3 1 3 5 1 4 6 2 4 6 3 4 7 4 6 1 2 10 1 3 100 1 4 90 2 3 20 2 4 80 3 4 40 2 1 1 2 1 3 0 3 1 1 2 1 3 3 1 2 2 2 3 5 1 3 6 5 10 1 2 110 1 3 120 1 4 130 1 5 120 2 3 110 2 4 120 2 5 130 3 4 120 3 5 110 4 5 120 5 10 1 2 9384 1 3 887 1 4 2778 1 5 6916 2 3 7794 2 4 8336 2 5 5387 3 4 493 3 5 6650 4 5 1422 5 8 1 2 1 2 3 100 3 4 100 4 5 100 1 5 50 2 5 50 3 5 50 4 1 150 0 0
Sample Output
1 20 0 -1 -1 1 0 1686 50
在所有生成树里,找到“最大边权值 减去 最小边权值”最小的那棵生成树。
那么,对于已经某个确定的最小边的所有生成树,我们找到最小生成树,它的“最大边权值 减去 最小边权值”就是这些生成树里最小的。
然后,我们枚举最小边即可。
1 #include<cstdio> 2 #include<iostream> 3 #include<algorithm> 4 using namespace std; 5 #define N 102 6 #define M 5000 7 #define INF 2147483647 8 int n,m; 9 struct Edge{ 10 int u,v,w; 11 }e[M]; 12 bool cmp(Edge a,Edge b){return a.w<b.w;} 13 int par[N]; 14 int find(int x){return( par[x]==x ? x : par[x]=find(par[x]) );} 15 int kruskal(int st)//获得最小边,作为开始边 16 { 17 int i,cnt=0; 18 for (i=1;i<=n;i++) par[i]=i;//初始化并查集 19 for (i=st;i<m;i++)//遍历后面的每条边 20 { 21 int x=find(e[i].u),y=find(e[i].v); 22 if (x != y){//如果这条边的连接的左右节点还未连通 23 par[y]=x;//将这条边连通 24 if (++cnt==n-1) break;//边计数增加1,如果边数到达了n-1条,那么一棵生成树已完成,跳出 25 } 26 } 27 if (cnt<n-1) return -1; //如果从开始边往后遍历,遍历完了所有边,依然无法产生一颗生成树,那么返回-1 28 return e[i].w-e[st].w; //否则就返回这棵生成树的“最大边权值 减去 最小边权值”的值 29 } 30 int main() 31 { 32 while (scanf("%d%d",&n,&m) && n!=0) 33 { 34 for (int i=0;i<m;i++) scanf("%d%d%d",&e[i].u,&e[i].v,&e[i].w); 35 sort(e,e+m,cmp);//把边按权值按从小到大排序 36 int tmp,ans=INF; 37 for (int i=0;i<m;i++)//枚举最小边 38 { 39 tmp=kruskal(i); 40 if(tmp==-1) break;//如果从这条最小边开始已经无法产生生成树了,之后显然也不会有生成树了,那么我们就直接跳出即可 41 if(tmp<ans) ans=tmp;//记录下最小的那个“最大边权值 减去 最小边权值” 42 } 43 if(ans==INF) printf("-1 "); //如果答案没被更新过,那么显然连一棵生成树都没有,按题目要求打印-1 44 else printf("%d ",ans);//否则就打印出答案即可 45 } 46 return 0; 47 }