题目链接:http://poj.org/problem?id=2676
Time Limit: 2000MS Memory Limit: 65536K
Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
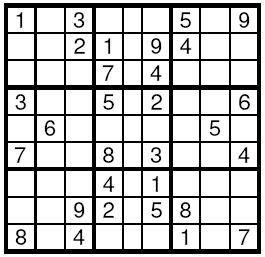
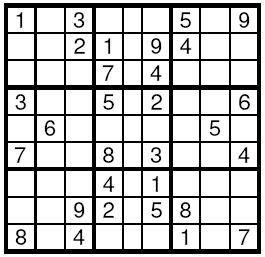
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1 103000509 002109400 000704000 300502006 060000050 700803004 000401000 009205800 804000107
Sample Output
143628579 572139468 986754231 391542786 468917352 725863914 237481695 619275843 854396127
题意:
你一定听说过“数独”游戏。
如【图1.png】,玩家需要根据9×9盘面上的已知数字,推理出所有剩余空格的数字,并满足每一行、每一列、每一个同色九宫内的数字均含1-9,不重复。
数独的答案都是唯一的,所以,多个解也称为无解。
本图的数字据说是芬兰数学家花了3个月的时间设计出来的较难的题目。但对会使用计算机编程的你来说,恐怕易如反掌了。
本题的要求就是输入数独题目,程序输出数独的唯一解。我们保证所有已知数据的格式都是合法的,并且题目有唯一的解。
格式要求:
输入9行,每行9个数字,0代表未知,其它数字为已知。
输出9行,每行9个数字表示数独的解。
题解:
从[1,1]到[9,9]地进行DFS
AC代码:
#include<cstdio> #include<cstring> using namespace std; int num[10][10]; bool rowVis[10][10],colVis[10][10],matVis[10][10]; struct Pos{ int row,col; Pos nextpos() { if(col==9) return (Pos){row+1,1}; else return (Pos){row,col+1}; } int MatID(){return (row-1)/3*3 + (col-1)/3+1;} bool ok(int x) { if(!rowVis[row][x] && !colVis[col][x] && !matVis[MatID()][x]) return 1; else return 0; } }; void mark(Pos pos,int x,bool val) { rowVis[pos.row][x] = val; colVis[pos.col][x] = val; matVis[pos.MatID()][x] = val; } bool dfs(Pos pos) { int row=pos.row,col=pos.col; if(row==10) return 1; if(num[row][col]) return dfs(pos.nextpos()); for(int i=1;i<=9;i++) { if(pos.ok(i)) { num[row][col]=i; mark(pos,i,1); if(dfs(pos.nextpos())) return 1; num[row][col]=0; mark(pos,i,0); } } return 0; } int main() { int t; scanf("%d",&t); while(t--) { memset(rowVis,0,sizeof(rowVis)); memset(colVis,0,sizeof(colVis)); memset(matVis,0,sizeof(matVis)); for(int row=1;row<=9;row++) { char tmp[10]; scanf("%s",tmp); for(int col=1;col<=9;col++) { num[row][col]=tmp[col-1]-'0'; if(num[row][col]) mark((Pos){row,col},num[row][col],1); } } dfs((Pos){1,1}); for(int row=1;row<=9;row++) { for(int col=1;col<=9;col++) printf("%d",num[row][col]); printf(" "); } } }