A tree is an undirected graph in which any two vertices are connected by exactly one path. In other words, any connected graph without simple cycles is a tree.
Given a tree of n
nodes labelled from 0
to n - 1
, and an array of n - 1
edges
where edges[i] = [ai, bi]
indicates that there is an undirected edge between the two nodes ai
and bi
in the tree, you can choose any node of the tree as the root. When you select a node x
as the root, the result tree has height h
. Among all possible rooted trees, those with minimum height (i.e. min(h)
) are called minimum height trees (MHTs).
Return a list of all MHTs' root labels. You can return the answer in any order.
The height of a rooted tree is the number of edges on the longest downward path between the root and a leaf.
Example 1:
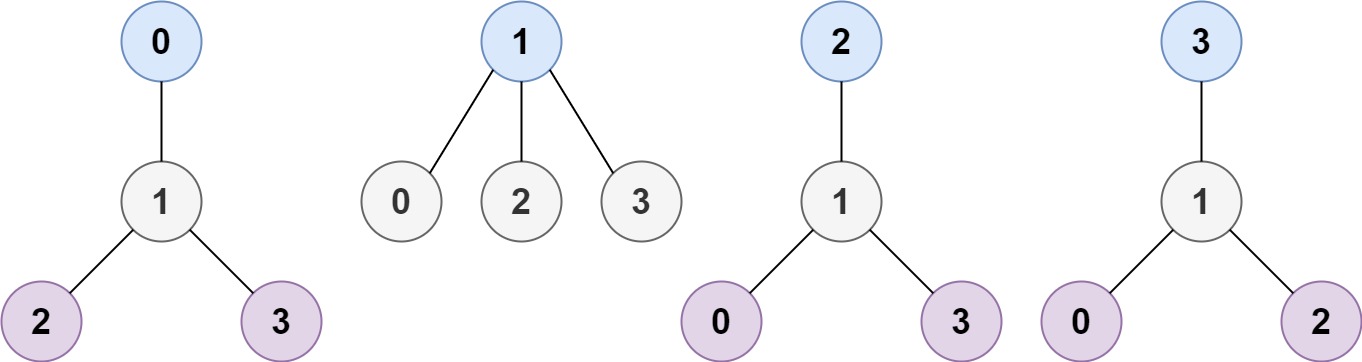
Input: n = 4, edges = [[1,0],[1,2],[1,3]] Output: [1] Explanation: As shown, the height of the tree is 1 when the root is the node with label 1 which is the only MHT.
Example 2:
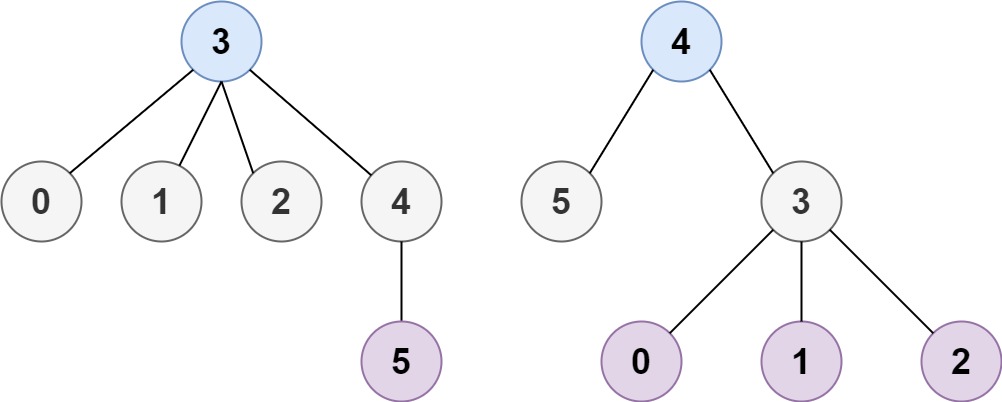
Input: n = 6, edges = [[3,0],[3,1],[3,2],[3,4],[5,4]] Output: [3,4]
Example 3:
Input: n = 1, edges = [] Output: [0]
Example 4:
Input: n = 2, edges = [[0,1]] Output: [0,1]
Constraints:
1 <= n <= 2 * 104
edges.length == n - 1
0 <= ai, bi < n
ai != bi
- All the pairs
(ai, bi)
are distinct. - The given input is guaranteed to be a tree and there will be no repeated edges.
basic idea: delete all the leaves at the same time, until one/two leaves are left
(why this method works: any node that has already been a leaf cannot be the root of a MHT,
because its adjacent non-leaf node will always be a better candidate)
for leaf nodes, the degree = 1, which means each of them is only connected to one node
in each loop, delete the leaf nodes from the graph, and add new leaves to the queue
so basically in the end, the nodes in the queue would be connected to no other nodes but each other, (when there're 2 or 1 nodes left). they should be the answer
time = O(V + E), space = O(V + E)
class Solution { public List<Integer> findMinHeightTrees(int n, int[][] edges) { if(n == 1) { return Arrays.asList(0); } List<Set<Integer>> graph = new ArrayList<>(); for(int i = 0; i < n; i++) { graph.add(new HashSet<>()); } for(int[] e : edges) { graph.get(e[0]).add(e[1]); graph.get(e[1]).add(e[0]); } List<Integer> leaves = new ArrayList<>(); for(int i = 0; i < n; i++) { if(graph.get(i).size() == 1) { leaves.add(i); } } while(n > 2) { List<Integer> newLeaves = new ArrayList<>(); for(int leaf : leaves) { for(int nei : graph.get(leaf)) { graph.get(nei).remove(leaf); if(graph.get(nei).size() == 1) { newLeaves.add(nei); } } } n -= leaves.size(); leaves = newLeaves; } return leaves; } }
class Solution { public List<Integer> findMinHeightTrees(int n, int[][] edges) { if(n == 1) { return Arrays.asList(0); } Map<Integer, Set<Integer>> graph = new HashMap<>(); for(int[] e : edges) { graph.putIfAbsent(e[0], new HashSet<>()); graph.putIfAbsent(e[1], new HashSet<>()); graph.get(e[0]).add(e[1]); graph.get(e[1]).add(e[0]); } List<Integer> leaves = new ArrayList<>(); for(Map.Entry<Integer, Set<Integer>> entry : graph.entrySet()) { if(entry.getValue().size() == 1) { leaves.add(entry.getKey()); } } while(n > 2) { List<Integer> newLeaves = new ArrayList<>(); for(int leaf : leaves) { for(int nei : graph.get(leaf)) { graph.get(nei).remove(leaf); if(graph.get(nei).size() == 1) { newLeaves.add(nei); } } } n -= leaves.size(); leaves = newLeaves; } return leaves; } }