HBase的基本Java_API操作
准备测试单元
BeforeTest
1 private Connection connection;
2 private Admin admin;
3
4 @BeforeTest
5 public void beforeTest() throws IOException {
6 //1.使用HbaseConfiguration.create()创建Hbase配置。
7 Configuration configuration = HBaseConfiguration.create();
8 //2.使用ConnectionFactory.createConnection(创建Hbase连接。
9 connection = ConnectionFactory.createConnection(configuration);
10 //3.要创建表,需要基于Hbase连接获取admin管理对象
11 admin = connection.getAdmin();
12 }
AfterTest
@AfterTest
public void afterTest() throws IOException {
//4.使用admin.close、 connection.close关闭连接
admin.close();
connection.close();
}
表的建立
@Test
//创建表,参数tableName为表的名称,字符串数组fields为存储记录各个字段名称的数组。
// 要求当HBase已经存在名为tableName的表的时候,先删除原有的表,然后再创建新的表。
public void createTable() throws IOException {
/*
* 1. 判断表是否存在。
a) 存在,则退出
2.使用TableDescriptorBuilder.newBuilder构建表描述构建器
3.使用ColumnFamilyDescriptorBuilder.newBuilder构建列蔟描述构建器。
4.构建列蔟描述,构建表描述
5.创建表
*/
TableName tableName = TableName.valueOf("hebei_nt");
if(admin.tableExists(tableName)){
deleteTable(tableName.toString());
return;
}
//构建表
//表描述器
TableDescriptorBuilder tableDescriptorBuilder = TableDescriptorBuilder.newBuilder(tableName);
//列簇构建
String[] fields ={"Student","Course","SC"};
for (int i=0;i<fields.length;i++)
{
//3.创建列簇构造描述器
ColumnFamilyDescriptorBuilder columnFamilyDescriptorBuilder = ColumnFamilyDescriptorBuilder.newBuilder(Bytes.toBytes(fields[i]));
//4.构建列簇描述
ColumnFamilyDescriptor cfDes = columnFamilyDescriptorBuilder.build();
//建立表与列簇的关联关系
tableDescriptorBuilder.setColumnFamily(cfDes);
}
//创建表
TableDescriptor tableDescriptor = tableDescriptorBuilder.build();
admin.createTable(tableDescriptor);
}
数据插入(指定rowKey)
@Test
//向表tableName、行row(用S_Name表示)和字符串数组fields指定的单元格中添加对应的数据values。
// 其中,fields中每个元素如果对应的列族下还有相应的列限定符的话,用“columnFamily:column”表示。
// 例如,同时向“Math”、“Computer Science”、“English”三列添加成绩时,
// 字符串数组fields为{“Score:Math”, ”Score:Computer Science”, ”Score:English”},数组values存储这三门课的成绩。
public void addRecord() throws IOException {
String[] fields = {"Student:S_NO", "Student:S_Name", "Student:S_Sex", "Student:S_Age"};
String[] values = {"2015001", "Zhangsan", "male", "23"};
String row = "Zhangsan";
TableName tableName = TableName.valueOf("hebei_nt");
Table table = connection.getTable(tableName);
String []column=new String[110];
String columnFamily="";
for(int i=0;i<fields.length;i++)
{
String []split=fields[i].split(":");
column[i]=split[1];
columnFamily=split[0];
}
Put put = new Put(Bytes.toBytes(row));
for(int i=0;i<values.length;i++)
{
put.addColumn(Bytes.toBytes(columnFamily),Bytes.toBytes(column[i]),Bytes.toBytes(values[i]));
}
//5.使用htable表执行put操作
table.put(put);
//关闭表
table.close();
}
查看指定rowKey下的某个列簇的值
@Test
// 浏览表tableName某一列的数据,如果某一行记录中该列数据不存在,则返回null。
// 要求当参数column为某一列族名称时,如果底下有若干个列限定符,则要列出每个列限定符代表的列的数据;
// 当参数column为某一列具体名称(例如“Score:Math”)时,只需要列出该列的数据。
public void scanColumn() throws IOException {
TableName tableName = TableName.valueOf("hebei_nt");
String column = "Student:S_Name";
String rowkey = "Zhangsan";
Table table = connection.getTable(tableName);
Get get = new Get(Bytes.toBytes(rowkey));
if(column.contains(":"))
{
//查询指定rowkey和列簇下的指定列名
String[] split = column.split(":");
get.addColumn(Bytes.toBytes(split[0]),Bytes.toBytes(split[1]));
Result result = table.get(get);
byte[] value = result.getValue(Bytes.toBytes(split[0]), Bytes.toBytes(split[1]));
if(Bytes.toString(value)!=null)
System.out.println(Bytes.toString(value));
else
System.out.println("null");
}
else
{
//查询指定rowkey和列簇下的所有数据
get.addFamily(column.getBytes());
Result result = table.get(get);
Cell[] cells = result.rawCells();
for (Cell cell:cells)
{
//获取列簇名称
String cf = Bytes.toString(cell.getFamilyArray(), cell.getFamilyOffset(), cell.getFamilyLength());
//获取列的名称
String colunmName = Bytes.toString(cell.getQualifierArray(), cell.getQualifierOffset(), cell.getQualifierLength());
//获取值
String value = Bytes.toString(cell.getValueArray(), cell.getValueOffset(), cell.getValueLength());
if(value!=null)
System.out.println(cf+":"+colunmName+"=>"+value);
else
System.out.println(cf+":"+colunmName+"=>"+"null");
}
}
table.close();
}
修改rowKey下指定的列簇:列限定符的值
@Test
//修改表tableName,行row(可以用学生姓名S_Name表示),列column指定的单元格的数据。
public void modifyData() throws IOException {
TableName tableName = TableName.valueOf("hebei_nt");
String colum = "Student:S_Name";
String rowkey = "Zhangsan";
String value = "GaoDaShuai";
Table table = connection.getTable(tableName);
//修改操作
Put put = new Put(Bytes.toBytes(rowkey));
String[] split = colum.split(":");
String columnFamily=split[0];
String columnName=split[1];
put.addColumn(Bytes.toBytes(columnFamily),Bytes.toBytes(columnName),Bytes.toBytes(value));
table.put(put);
//查看修改后的数据
Get get = new Get(rowkey.getBytes());
get.addColumn(Bytes.toBytes(columnFamily),Bytes.toBytes(columnName));
Result result = table.get(get);
byte[] value2 = result.getValue(Bytes.toBytes(columnFamily),Bytes.toBytes(columnName));
if(Bytes.toString(value2)!=null)
System.out.println(columnFamily+":"+columnName+"=>"+Bytes.toString(value2));
else
System.out.println("null");
System.out.println("修改成功!!");
table.close();
}
删除一行数据
@Test
public void deleteRow() throws IOException {
TableName tableName = TableName.valueOf("hebei_nt");
String rowkey="Zhangsan";
Table table = connection.getTable(tableName);
//删除一条rowkey记录
Delete delete = new Delete(Bytes.toBytes(rowkey));
table.delete(delete);
table.close();
}
一张图看懂HBase的数据模型
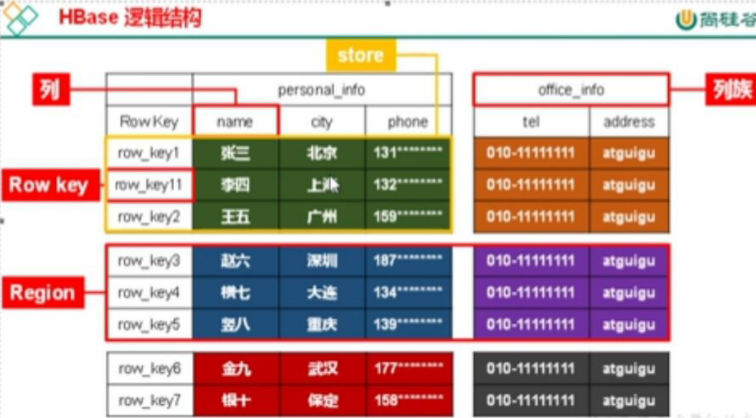
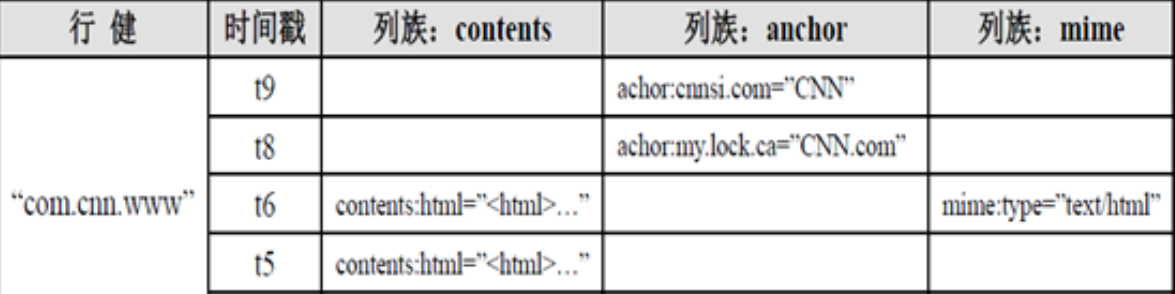