python之办公自动化
生成execl表
import pandas as pd
# DataFrame 数据帧 相当于 工作簿中的一个工作表
df = pd.DataFrame({
'Id': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15],
'Name': ['student_1', 'student_2', 'student_3', 'student_4', 'student_5', 'student_6', 'student_7', 'student_8',
'student_9', 'student_10', 'student_11', 'student_12', 'student_13', 'student_14', 'student_15'],
'Score': [21, 26, 47, 55, 66, 45, 46, 78, 89, 98, 87, 77, 44, 45, 78]
})
# 自定义索引
df = df.set_index('Id')
print(df)
df.to_excel(r'F:pythonProject2staticpeople.xlsx')
print('done!')
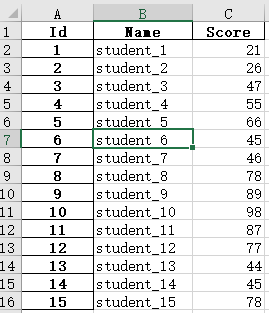
读取execl表
path = 'F:/pythonProject2/static/people.xlsx'
people = pd.read_excel(path, sheet_name='Sheet1', )
print(people)
print('***********************************')
# 跳过前1行
people = pd.read_excel(path, sheet_name='Sheet1', header=1)
print(people)
print('***********************************')
# 指定id为索引,去除默认的索引
people = pd.read_excel(path, sheet_name='Sheet1', index_col='Id', )
print(people.head(2))
print('***********************************')
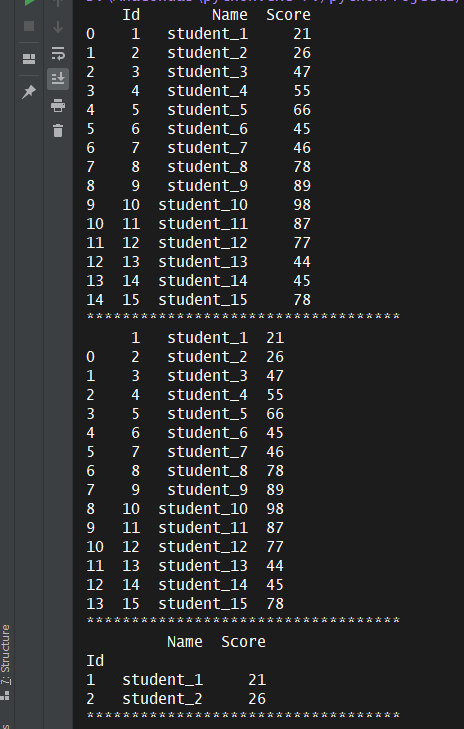
matplotlib绘图
import pandas as pd
import matplotlib.pylab as plt
Students = pd.read_excel(r'F:pythonProject2staticpeople.xlsx')
Students.sort_values(by='Score', inplace=True, ascending=False)
plt.bar(Students['Name'], Students.Score, color='orange')
# 添加中文支持
from matplotlib.font_manager import FontProperties
# 引入字体
font = FontProperties(fname=r"c:windowsfontsSimSun.ttc", size=16)
plt.title('学生分数', fontproperties=font)
plt.xlabel('名字', fontproperties=font, fontsize=14)
plt.ylabel('分数', fontproperties=font, fontsize=14)
plt.xticks(Students.Name, rotation='90')
plt.tight_layout()
plt.show()
![1]()
绘制叠加柱状图
import pandas as pd
import matplotlib.pylab as plt
users = pd.read_excel(r'F:pythonProject2staticdata.xlsx')
# 新计算出一个总量,用于排序‘
users['Total'] = users['Jan'] + users['Feb'] + users['Mar']
# 排序
users.sort_values(by='Total', inplace=True)
# 水平的叠加柱状图, barh 中的 h表示 horizontal 水平的
# 锤子的叠加主张图, bar
# 利用 stacked 就可以实现叠加形式
users.plot.bar(x='Name', y=['Jan', 'Feb', 'Mar'], stacked=True)
plt.tight_layout()
plt.show()

python操作word
from docx import Document
from docx.shared import Inches # 定义插入图像的英寸
document = Document()
# 新增段落
p2 = document.add_paragraph('这是个段落')
# 在p2段落前插入一个段落
p1 = p2.insert_paragraph_before('第一个段落')
# 新增标题,level有1-9种规格
# level=0,新增的标题会有带下划线样式
document.add_heading('这是标题', level=1)
# 新增分页符 -- 后面添加的内容会在新的一页中
document.add_page_break()
# 新增表格 -- 因为前面有分页符,所有这里的内容在新的一页中
table = document.add_table(rows=6, cols=6)
# 第一行第三列
cell = table.cell(0, 2)
cell.text = '第一行第三列'
row = table.rows[1]
row.cells[0].text = '第二行第一列'
row.cells[1].text = '第二行第二列'
document.add_picture('default.png', width=Inches(1.25))
document.save('new.docx')
生成工作报告简单案例
# -*- coding:utf-8 -*-
# @File :practice.py
# @Author:yx yao
# @Date :2020/10/19 15:19
# @Desc :
import pandas as pd
import matplotlib.pyplot as plt
from docx import Document
imgname = 'data.jpg'
students = pd.read_excel('F:pythonProject2staticpeople.xlsx')
students.sort_values(by='Score', inplace=True, ascending=False)
# 绘制柱状图
plt.bar(students.Name, students.Score, color='orange')
plt.title('Student Score', fontsize=10)
plt.xlabel('Name')
plt.ylabel('Score')
# 旋转x轴标签
plt.xticks(students.Name, rotation='90')
# 紧凑显示
plt.tight_layout()
# 保存图片
plt.savefig(imgname)
document = Document()
document.add_heading('数据分析报告', level=0)
# 调整排序后的索引
first_student = students.iloc[0,:]['Name']
first_score = students.iloc[0,:]['Score']
p = document.add_paragraph(f'总共有{len(students.Name)}名学生参加了考试,学生考试的总体情况!')
table = document.add_table(rows=len(students.Name)+1, cols=2)
# 设置表格样式
table.style = 'LightShading-Accent1'
table.cell(0, 0).text = '学生姓名'
table.cell(0, 1).text = '学生分数'
for i, (index, row) in enumerate(students.iterrows()):
table.cell(i+1, 0).text = str(row['Name'])
table.cell(i+1, 1).text = str(row['Score'])
document.add_picture(imgname)
document.save('Student.docx')
print('Done!')
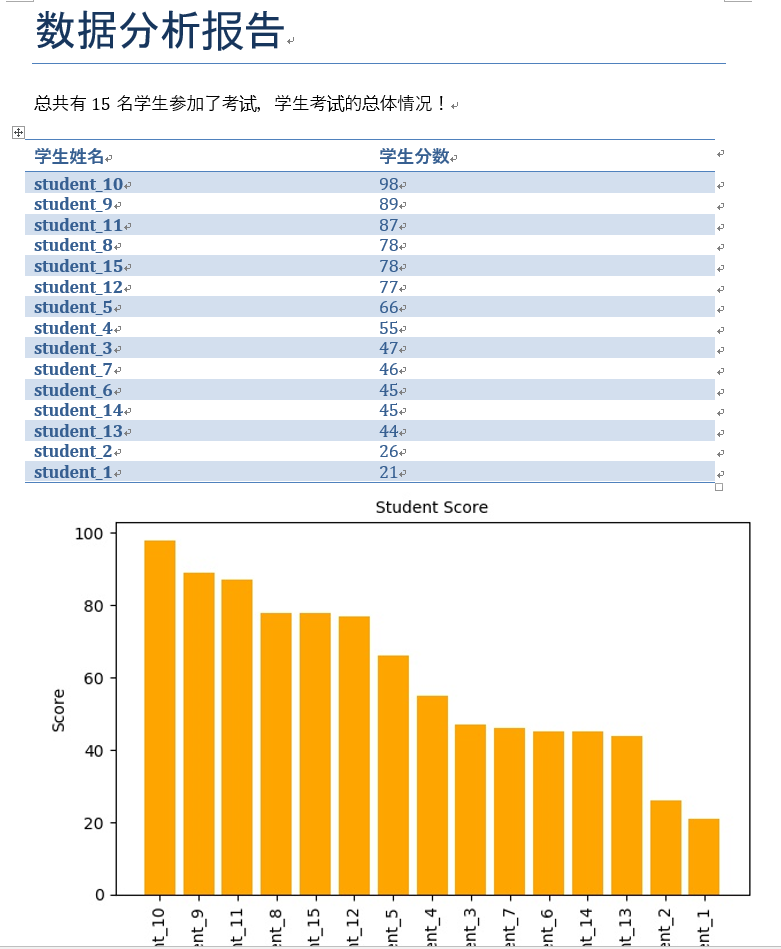