需求功能:
冲顶大会与百万英雄类的在线直播答题辅助,通过截图,识别中文(题目与选项),百度搜索分析搜索结果。
代码块:
#!/usr/bin/python # coding=utf-8 import subprocess from PIL import Image import time import requests from threading import Thread # # 颜色兼容Win 10 from colorama import init, Fore init() flag = False def get_screenshot(): """ 获取界面截图,返回Image.image :return: """ process = subprocess.Popen('D:\adb\adb.exe shell screencap -p', shell=True, stdout=subprocess.PIPE) binary_screenshot = process.stdout.read() binary_screenshot = binary_screenshot.replace(b' ', b' ') with open('screenshot.png', 'wb') as fp: fp.write(binary_screenshot) img = Image.open('screenshot.png') return img def crop_image(img): """ 圖片裁剪,裁剪出冲顶大会问题框区域box :param img: :return: """ region = (50, 350, 990, 1200) img_block = img.crop(region) img_block.save('image_croped.png') return img_block def baidu_ocr(image_path): """ 百度AIP,图片文字识别,返回问题标题与选项列表 :param image_path: :return: """ from aip import AipOcr APP_ID = '10680685' API_KEY = '5RhLj43kWTcQP26FNpKan3Lz' SECRET_KEY = 'C1YSYESz9Z8Zt9Ms0A7PTuIdGq8oOzUL' client = AipOcr(APP_ID, API_KEY, SECRET_KEY) with open(image_path, 'rb') as fp: image = fp.read() result = client.basicGeneral(image) result_list = result.get('words_result') quetion_lenth = len(result_list) - 3 count = 0 question = '' choices = [] for item in result.get('words_result'): if count < quetion_lenth: question = question + item.get('words') count += 1 else: choices.append(item.get('words')) return question, choices def search_baidu(type, question, choices): """ 百度搜索,返回搜索结果 :param type: :param question: :param choices: :return: """ global flag if '不是' in question or '没有' in question: print('**请注意此题为否定题,选计数最少的**') flag = True else: flag = False if type == 1: counts = [] # print(' -- 方法1: 题目搜索,搜索返回页源码中包含选项总量统计 -- ') content = '' for i in range(3): req = requests.get(url='http://www.baidu.com/s', params={'wd': question, 'pn': i * 10}) content = content + req.text for i in range(len(choices)): counts.append(content.count(choices[i])) if type == 2: counts = [] # print(' -- 方法2: 题目+选项 搜索结果匹配总量统计 -- ') for i in range(len(choices)): req = requests.get(url='http://www.baidu.com/s', params={'wd': question + ' +' + choices[i]}) content = req.text # print(req.url) index = content.find('百度为您找到相关结果约') + 11 content = content[index:] index = content.find('个') count = content[:index].replace(',', '') counts.append(count) if counts: output(question, choices, counts, type) def output(question, choices, counts, type): global flag counts = list(map(int, counts)) # 计数最高 index_max = counts.index(max(counts)) max_count = int(counts[index_max]) # 计数最少 index_min = counts.index(min(counts)) min_count = int(counts[index_min]) if max_count - min_count < 8: # print(Fore.RED + "计数差距过小,此方法失效!" + Fore.RESET) search_baidu(2, question, choices) return else: print(question + " --->查找方式type=" + str(type)) for i in range(len(choices)): if i == index_min: # 红色为计数最低的答案 print(Fore.MAGENTA + "{0} : {1}".format(choices[i], counts[i]) + Fore.RESET) elif i == index_max: # 绿色为计数最高的答案 print(Fore.GREEN + "{0} : {1} ".format(choices[i], counts[i]) + Fore.RESET) else: print("{0} : {1} ".format(choices[i], counts[i])) print("*" * 100) if __name__ == '__main__': while True: # step1:截图,获取图片 screenshot_time = time.time() img = get_screenshot() print("screenshot_time:", time.time() - screenshot_time) # step2:图片裁剪 crop_time = time.time() img = crop_image(img) print("crop_time:", time.time() - crop_time) # img.show() # step3:图片识别,获取问题与选项列表 baidu_time = time.time() question, choices = baidu_ocr("image_croped.png") print("baidu_time:", time.time() - baidu_time) # step4:问题搜索,返回搜索结果集,输出结果提示 search_time = time.time() search_baidu(1, question, choices) # m1 = Thread(search_baidu(1, question, choices)) # m2 = Thread(search_baidu(2, question, choices)) # m1.start() # m2.start() print("search_time:", time.time() - search_time) go = input('输入回车继续运行,输入 n 回车结束运行: ') if go == 'n': break
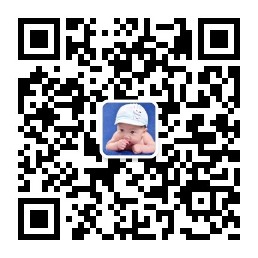