随机验证码需要借助pillow模块
pip install pillow
创建图片
from PIL import Image image =Image.new(mode='RGB',size=(240,350),color='red')
#这是在内存中看到的 image.show()
#保存到本地
#with open ('red_picture','wb')as f:
#img.save(f,format='png')
这是本地图片用图片选择器加载出来的
创建画笔
img
=
Image.new(mode
=
'RGB'
, size
=
(
120
,
30
), color
=
(
255
,
255
,
255
))
draw
=
ImageDraw.Draw(img, mode
=
'RGB'
)
画点
img
=
Image.new(mode
=
'RGB'
, size
=
(
120
,
30
), color
=
(
255
,
255
,
255
))
draw
=
ImageDraw.Draw(img, mode
=
'RGB'
)
画线
img
=
Image.new(mode
=
'RGB'
, size
=
(
120
,
30
), color
=
(
255
,
255
,
255
))
draw
=
ImageDraw.Draw(img, mode
=
'RGB'
)
# 第一个参数:表示坐标
# 第二个参数:表示颜色
draw.point([
100
,
100
], fill
=
"red"
)
draw.point([
300
,
300
], fill
=
(
255
,
255
,
255
))
画圆
img
=
Image.new(mode
=
'RGB'
, size
=
(
120
,
30
), color
=
(
255
,
255
,
255
))
draw
=
ImageDraw.Draw(img, mode
=
'RGB'
)
# 第一个参数:表示起始坐标和结束坐标(圆要画在其中间)
# 第二个参数:表示开始角度
# 第三个参数:表示结束角度
# 第四个参数:表示颜色
draw.arc((
100
,
100
,
300
,
300
),
0
,
90
,fill
=
"red"
)
写文本
img
=
Image.new(mode
=
'RGB'
, size
=
(
120
,
30
), color
=
(
255
,
255
,
255
))
draw
=
ImageDraw.Draw(img, mode
=
'RGB'
)
# 第一个参数:表示起始坐标
# 第二个参数:表示写入内容
# 第三个参数:表示颜色
draw.text([
0
,
0
],
'python'
,
"red"
)
特殊体文字
img
=
Image.new(mode
=
'RGB'
, size
=
(
120
,
30
), color
=
(
255
,
255
,
255
))
draw
=
ImageDraw.Draw(img, mode
=
'RGB'
)
# 第一个参数:表示字体文件路径
# 第二个参数:表示字体大小
font
=
ImageFont.truetype(
"mo.ttf"
,
28
)
# 第一个参数:表示起始坐标
# 第二个参数:表示写入内容
# 第三个参数:表示颜色
# 第四个参数:表示颜色
draw.text([
0
,
0
],
'python'
,
"red"
, font
=
font)
这个文件的font是要去下载的
打开Windows里面的font是可以看到本机支持的字体
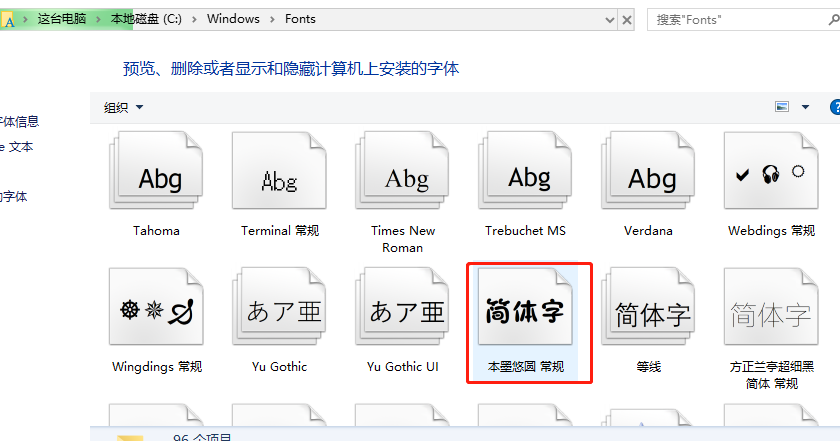
特殊验证码
import random def check_code(width=120, height=30, char_length=5, font_file='kumo.ttf', font_size=28): code = [] img = Image.new(mode='RGB', size=(width, height), color=(255, 255, 255)) draw = ImageDraw.Draw(img, mode='RGB') def rndChar(): """ 生成随机字母 :return: """ return chr(random.randint(65, 90)) def rndColor(): """ 生成随机颜色 :return: """ return (random.randint(0, 255), random.randint(10, 255), random.randint(64, 255)) # 写文字 font = ImageFont.truetype(font_file, font_size) for i in range(char_length): char = rndChar() code.append(char) h = random.randint(0, 4) draw.text([i * width / char_length, h], char, font=font, fill=rndColor()) # 写干扰点 for i in range(40): draw.point([random.randint(0, width), random.randint(0, height)], fill=rndColor()) # 写干扰圆圈 for i in range(40): draw.point([random.randint(0, width), random.randint(0, height)], fill=rndColor()) x = random.randint(0, width) y = random.randint(0, height) draw.arc((x, y, x + 4, y + 4), 0, 90, fill=rndColor()) # 画干扰线 for i in range(5): x1 = random.randint(0, width) y1 = random.randint(0, height) x2 = random.randint(0, width) y2 = random.randint(0, height) draw.line((x1, y1, x2, y2), fill=rndColor()) img = img.filter(ImageFilter.EDGE_ENHANCE_MORE) return img,''.join(code) if __name__ == '__main__': # 1. 直接打开 # img,code = check_code() # img.show() # 2. 写入文件 # img,code = check_code() # with open('code.png','wb') as f: # img.save(f,format='png') # 3. 写入内存(Python3) # from io import BytesIO # stream = BytesIO() # img.save(stream, 'png') # stream.getvalue() # 4. 写入内存(Python2) # import StringIO # stream = StringIO.StringIO() # img.save(stream, 'png') # stream.getvalue() pass
验证码
from io import BytesIO import random from PIL import Image,ImageDraw,ImageFont img=Image.new(mode="RGB",size=(120,40),color=(random.randint(0,255),random.randint(0,255),random.randint(0,255))) draw=ImageDraw.Draw(img,"RGB") font=ImageFont.truetype("app01/static/font/kumo.ttf",30) vilid_list=[] for i in range(5): random_num=str(random.randint(0,9)) random_lower_word=chr(random.randint(65,90)) random_upper_word=chr(random.randint(97,122)) random_char=random.choice([random_num,random_lower_word,random_upper_word]) draw.text([5+i*24,10],random_char,(random.randint(0,255),random.randint(0,255),random.randint(0,255)),font=font) vilid_list.append(random_char) for i in range(500): draw.point([random.randint(0,133), random.randint(0,40)], fill=(random.randint(0,255),random.randint(0,255),random.randint(0,255))) f=BytesIO() img.save(f,"png") data=f.getvalue() vilid_str="".join(vilid_list) request.session["ValidCode"]=vilid_str return HttpResponse(data)