动手动脑
一.JAVA的类的对象实例化
1)定义:在面向对象的编程中,通常把用类创建对象的过程称为实例化,其格式为:类名 对象名 = new 类名(参数1,参数2...参数n);
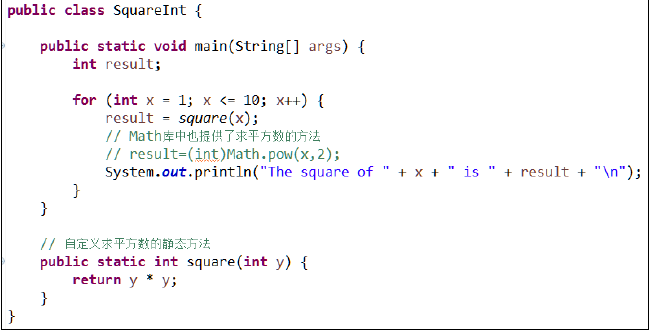
图2:进行了对象实例化:
二.利用线性同余法生成随机数
1)定义:
2)例子:
课后作业
一.组合数问题
1)程序设计思想:1)利用阶乘方法来实现2)利用杨辉三角的递归来实现3)利用递归来实现
2)源代码:
//2016/10/15 XuetongGao
//组合数的三种实现方式
import java.io.*;
public class CombinatorialNumber {
public static void main(String[] args) throws IOException{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String num1 = reader.readLine(); String num2 = reader.readLine();
int n = Integer.parseInt(num1);int k = Integer.parseInt(num2);
if(n>=2&&k>1&&(n>=k))
{
int sum1,sum2,sum3;
sum1 = nStratum(n,k);
System.out.println("The combinatorial number of (n,k)is " + sum1);
sum2 = triangleYH(n,k);
System.out.println("The combinatorial number of (n+1,k)is " + sum2);
sum3 = digui(n,k);
System.out.println("The combinatorial number of (n,k)is " + sum3);
}
else if(n>=2&&k==1)
{
System.out.println("The combinatorial number of (n,k)is " + n);
}
else if(n==1&&k==1)
{
System.out.println("The combinatorial number of (n,k)is 1.");
}
else
{
System.out.println("Error!please input again!");
}
}
public static int nStratum(int n,int k)//用阶乘来实现组合数问题
{
int n_stratum=1,k_stratum=1,n_k_stratum=1,sum=1;
int i;
for(i=1;i<=n;i++) { n_stratum=n_stratum*i; }
for(i=1;i<=k;i++) { k_stratum=k_stratum*i; }
for(i=1;i<=(n-k);i++) { n_k_stratum=n_k_stratum*i; }
sum = n_stratum/(k_stratum*n_k_stratum);
return sum;
}
public static int digui(int n,int k)//用递归来实现组合数问题
{
if(k==1) return n;
else
{
int sum = (digui(n,k-1))*(n-1)/k;
return sum;
}
}
public static int triangleYH(int n,int k)//用杨辉三角来实现组合数问题
{
int sum,sum1,sum2;
sum1 = digui(n,k);
sum2 = digui(n,k-1);
sum = sum1+sum2;
return sum;
}
}
3)实验截图
:
二.汉诺塔问题
1)程序设计思想:
(1)在主方法中设置输入流,输入盘子的个数,引入solveTowers方法;
(2)solveTowers方法:若盘子数为1,则不继续递归,结束;若不为1,则将若剩余盘子数不为1 则继续递归 :先将a上上边n-1个盘子移动到b上,将最大的圆盘移动到C上,将B上的圆盘移动到C上;
(3)在主方法中输出。
2)源代码:
//2010/10/14 XuetongGao
//用递归方式解决汉诺塔问题
import java.io.*;//引入包
public class TowersOfHanoi
{
public static void main( String[] args ) throws IOException//扔出流
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));//设定输入流
String num = reader.readLine();//输入
int n = Integer.parseInt(num);//强制类型转化
solveTowers(n,'A','B','C');//引用方法
}
public static void solveTowers(int n,char a,char b,char c)//方法:解决汉诺塔问题
{
if (n == 1)//若剩余盘子数为1 则不继续递归 结束
System.out.println("盘 " + n + " 由 " + a + " 移至 " + c);
else {//若剩余盘子数不为1 则继续递归 :先将a上上边n-1个盘子移动到b上
solveTowers(n - 1, a, c, b);//算法:将上n-1个圆盘移动到B上
System.out.println("盘 " + n + " 由 " + a + " 移至 " + c);//算法:将最大的圆盘移动到C上
solveTowers(n - 1, b, a, c);//算法:将B上的圆盘移动到C上
}
}
}
3)实验截图:
三.运用递归判断某个字符串是否回文
1)程序设计思想:在主方法中调用判断是否为函数的方法,该函数的参数为(字符串,前对应字符,后对应字符),若两字符的位置不相等,则递归判断一直到两字符的位置相等,同时若两字符相等返回真,若不相等,则返回假。
2)源代码:
//2016/10/15 XuetongGao
//判断字符串是否回文
public class Palindrome {
public static boolean isPalindrome(String s,int i,int j)
{ if(i>j) throw new IllegalArgumentException();
if(i == j) return true;
else{ return (s.charAt(i)==s.charAt(j))&& isPalindrome(s,i+1,j-1); }
}
public static void main(String[] args) {
String test = "ABCBA";
int i=0; int j=test.length()-1;
System.out.println(test + " is Palindrome?" + Palindrome.isPalindrome(test, i, j));
}
}
3)实验截图: