一、昨日内容回顾
数据类型补充:
str <--> list split join
str <--> list split join
同一个代码块的缓存机制:
适用的数据类型:str int bool
适用的数据类型:str int bool
小数据池(也是一种缓存机制):
适用的数据类型:int(-5~256)str(符合一定规则的)bool
小数据池缓存机制和同一个代码块的缓存机制的意义:节省内存,提高性能
编码进阶——转换
二、文件操作
初识:
如果想要通过python代码操作这个文件:必要的三要素:
1、path:即文件路径
2、mode:即模式 r w r+ w+ a ......
3、encoding:编码方式
以上就是open()方法所需要的参数其中mode参数默认mode='r'
读(r rb r+ r+b):
r模式下的物种读取方式:
read()
|
全部读取
|
read(n)
|
按照字符读取(rb模式下按照字节读取)
|
readline()
|
按行读取
|
readlines() |
返回一个列表,每个元素是原文件的每行数据
|
for循环
|
# file_handler = open('test.txt', mode='r+', encoding='utf-8')
# for line in file_handler:
# print(line.strip())
# file_handler.close()
|
rb模式下的物种读取方式:
read()
|
全部读取
|
read(n)
|
按照字节读取(r模式下按照字符读取)
|
readline()
|
按行读取
|
readlines()
|
返回一个列表,每个元素是原文件的每行数据
|
for循环
|
# file_handler = open('test.txt', mode='rb')
# for line in file_handler:
# print(line.strip())
# file_handler.close()
|
b模式操作的文件是非文字类的文件:图片,视频,音频等的
r+模式,应该先读后写
那如果先写后读呢?
这涉及一个光标的问题,需要调整光标。 用seek方法调整光标位置seek有三种移动方式0,1,2,其中1和2必须在b模式下进行,但无论哪种模式,都是以bytes为单位移动的
写(w wb w+ w+b)
w模式必须以字符串的内容写入
有文件先清空写入,没有文件创造文件写入
w+模式,先写后读,做完写的操作后,光标已经移动到文件的最后位置,所以如果后面再读的话,读不出内容,要通过调整光标解决这个问题
追加(a ab a+ a+b)
分析一下这串代码:
f1 = open('d:诶呀学习好有趣.ext', endoding = 'utf-8', mode= 'r') countent = f1.read() print(countent) f.close()
f1 f file file_handler f_h......这些叫文件句柄
open()内置函数 这个函数实际上是调用的操作系统的对文件操作的功能
Windows:默认的编码是gbk
linux:默认的编码方式是utf-8
IOS:默认的编码方式是utf-8
接下来你对文件进行的任何操作,都需要借助文件句柄操作
f.close()
Python打开一个文件总共分三步:
第一步:打开文件产生文件句柄
第二步:对文件句柄进行操作
第三步:关闭文件句柄
python文件方法中有一种傻瓜式的骚操作,不用手动关闭文件句柄(会自动关闭),并且可以同一语句操作创建多个文件句柄:
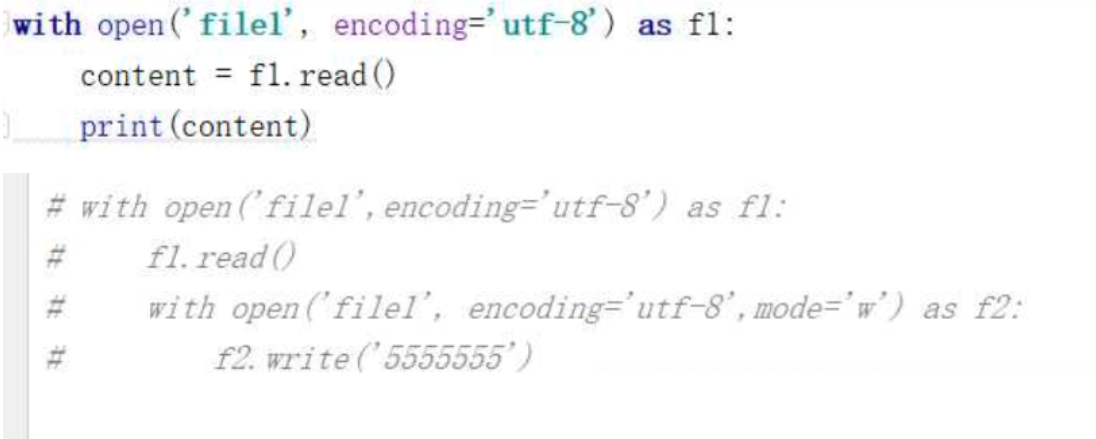
文件的改(当前几乎所有的文件编辑软件都是这种套路):
1.以读的模式打开原文件产生文件句柄f1
1.以读的模式打开原文件产生文件句柄f1
2.以写的模式打开一个新文件产生文件句柄f2
3.读取原文件,将原文件的内容改写成新内容写入新文件
4.删除原文件
5.将新文件重命名成原文件
python的实现方式:
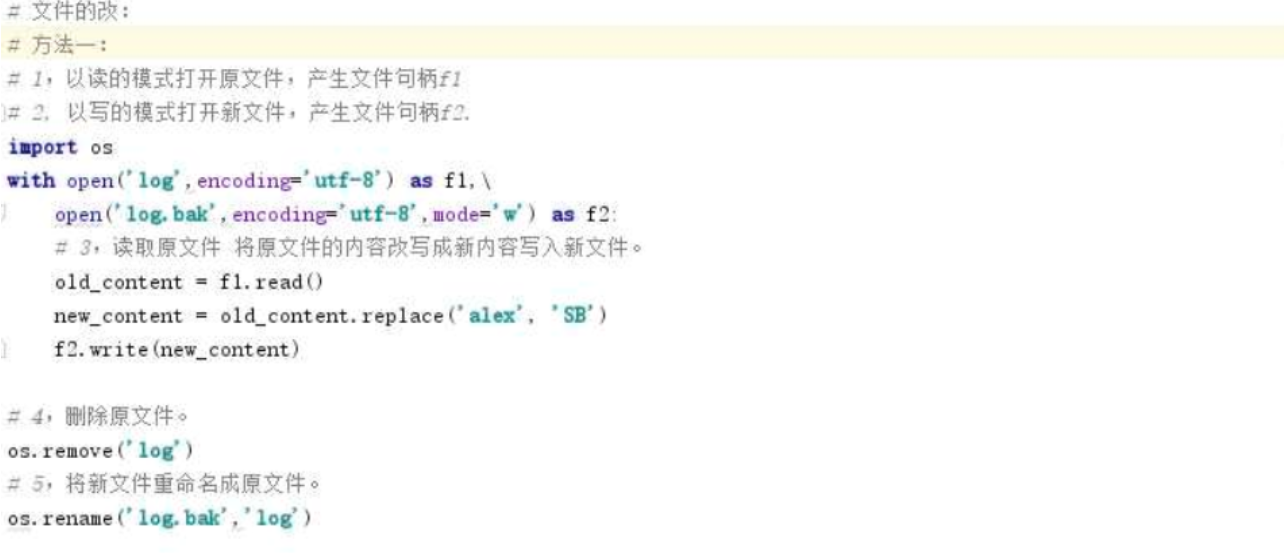

1 import os # 调用系统模块 2 3 with open('a.txt') as read_f,open('.a.txt.swap','w') as write_f: 4 data=read_f.read() #全部读入内存,如果文件很大,会很卡 5 data=data.replace('alex','SB') #在内存中完成修改 6 7 write_f.write(data) #一次性写入新文件 8 9 os.remove('a.txt') #删除原文件 10 os.rename('.a.txt.swap','a.txt') #将新建的文件重命名为原文件
升级版
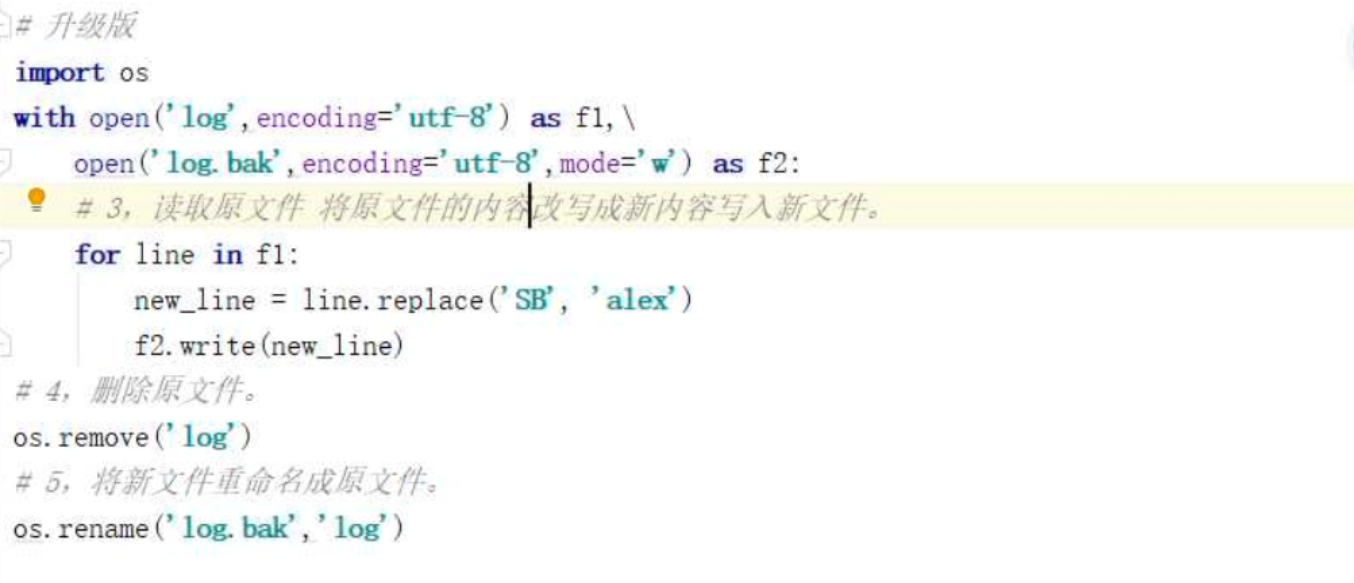

1 import os 2 3 with open('a.txt') as read_f,open('.a.txt.swap','w') as write_f: 4 for line in read_f: 5 line=line.replace('alex','SB') 6 write_f.write(line) 7 8 os.remove('a.txt') 9 os.rename('.a.txt.swap','a.txt')
萌新的常见报错原因:
1、路径报错。与后面的字符产生了特殊意义。
解决方式:

解决方式:用什么编码存储的文件就要用什么编码进行操作
3、encodding 只是声明了此文件的需要用什么编码本解码而已
补充知识点:
绝对路径:从磁盘(根目录)开始,直到找到文件。
相对路径:当前路径(当前文件夹)找到的文件
文件的其他操作:

1 class file(object) 2 def close(self): # real signature unknown; restored from __doc__ 3 关闭文件 4 """ 5 close() -> None or (perhaps) an integer. Close the file. 6 7 Sets data attribute .closed to True. A closed file cannot be used for 8 further I/O operations. close() may be called more than once without 9 error. Some kinds of file objects (for example, opened by popen()) 10 may return an exit status upon closing. 11 """ 12 13 def fileno(self): # real signature unknown; restored from __doc__ 14 文件描述符 15 """ 16 fileno() -> integer "file descriptor". 17 18 This is needed for lower-level file interfaces, such os.read(). 19 """ 20 return 0 21 22 def flush(self): # real signature unknown; restored from __doc__ 23 刷新文件内部缓冲区 24 """ flush() -> None. Flush the internal I/O buffer. """ 25 pass 26 27 28 def isatty(self): # real signature unknown; restored from __doc__ 29 判断文件是否是同意tty设备 30 """ isatty() -> true or false. True if the file is connected to a tty device. """ 31 return False 32 33 34 def next(self): # real signature unknown; restored from __doc__ 35 获取下一行数据,不存在,则报错 36 """ x.next() -> the next value, or raise StopIteration """ 37 pass 38 39 def read(self, size=None): # real signature unknown; restored from __doc__ 40 读取指定字节数据 41 """ 42 read([size]) -> read at most size bytes, returned as a string. 43 44 If the size argument is negative or omitted, read until EOF is reached. 45 Notice that when in non-blocking mode, less data than what was requested 46 may be returned, even if no size parameter was given. 47 """ 48 pass 49 50 def readinto(self): # real signature unknown; restored from __doc__ 51 读取到缓冲区,不要用,将被遗弃 52 """ readinto() -> Undocumented. Don't use this; it may go away. """ 53 pass 54 55 def readline(self, size=None): # real signature unknown; restored from __doc__ 56 仅读取一行数据 57 """ 58 readline([size]) -> next line from the file, as a string. 59 60 Retain newline. A non-negative size argument limits the maximum 61 number of bytes to return (an incomplete line may be returned then). 62 Return an empty string at EOF. 63 """ 64 pass 65 66 def readlines(self, size=None): # real signature unknown; restored from __doc__ 67 读取所有数据,并根据换行保存值列表 68 """ 69 readlines([size]) -> list of strings, each a line from the file. 70 71 Call readline() repeatedly and return a list of the lines so read. 72 The optional size argument, if given, is an approximate bound on the 73 total number of bytes in the lines returned. 74 """ 75 return [] 76 77 def seek(self, offset, whence=None): # real signature unknown; restored from __doc__ 78 指定文件中指针位置 79 """ 80 seek(offset[, whence]) -> None. Move to new file position. 81 82 Argument offset is a byte count. Optional argument whence defaults to 83 (offset from start of file, offset should be >= 0); other values are 1 84 (move relative to current position, positive or negative), and 2 (move 85 relative to end of file, usually negative, although many platforms allow 86 seeking beyond the end of a file). If the file is opened in text mode, 87 only offsets returned by tell() are legal. Use of other offsets causes 88 undefined behavior. 89 Note that not all file objects are seekable. 90 """ 91 pass 92 93 def tell(self): # real signature unknown; restored from __doc__ 94 获取当前指针位置 95 """ tell() -> current file position, an integer (may be a long integer). """ 96 pass 97 98 def truncate(self, size=None): # real signature unknown; restored from __doc__ 99 截断数据,仅保留指定之前数据 100 """ 101 truncate([size]) -> None. Truncate the file to at most size bytes. 102 103 Size defaults to the current file position, as returned by tell(). 104 """ 105 pass 106 107 def write(self, p_str): # real signature unknown; restored from __doc__ 108 写内容 109 """ 110 write(str) -> None. Write string str to file. 111 112 Note that due to buffering, flush() or close() may be needed before 113 the file on disk reflects the data written. 114 """ 115 pass 116 117 def writelines(self, sequence_of_strings): # real signature unknown; restored from __doc__ 118 将一个字符串列表写入文件 119 """ 120 writelines(sequence_of_strings) -> None. Write the strings to the file. 121 122 Note that newlines are not added. The sequence can be any iterable object 123 producing strings. This is equivalent to calling write() for each string. 124 """ 125 pass 126 127 def xreadlines(self): # real signature unknown; restored from __doc__ 128 可用于逐行读取文件,非全部 129 """ 130 xreadlines() -> returns self. 131 132 For backward compatibility. File objects now include the performance 133 optimizations previously implemented in the xreadlines module. 134 """ 135 pass 136 137 2.x

1 class TextIOWrapper(_TextIOBase): 2 """ 3 Character and line based layer over a BufferedIOBase object, buffer. 4 5 encoding gives the name of the encoding that the stream will be 6 decoded or encoded with. It defaults to locale.getpreferredencoding(False). 7 8 errors determines the strictness of encoding and decoding (see 9 help(codecs.Codec) or the documentation for codecs.register) and 10 defaults to "strict". 11 12 newline controls how line endings are handled. It can be None, '', 13 ' ', ' ', and ' '. It works as follows: 14 15 * On input, if newline is None, universal newlines mode is 16 enabled. Lines in the input can end in ' ', ' ', or ' ', and 17 these are translated into ' ' before being returned to the 18 caller. If it is '', universal newline mode is enabled, but line 19 endings are returned to the caller untranslated. If it has any of 20 the other legal values, input lines are only terminated by the given 21 string, and the line ending is returned to the caller untranslated. 22 23 * On output, if newline is None, any ' ' characters written are 24 translated to the system default line separator, os.linesep. If 25 newline is '' or ' ', no translation takes place. If newline is any 26 of the other legal values, any ' ' characters written are translated 27 to the given string. 28 29 If line_buffering is True, a call to flush is implied when a call to 30 write contains a newline character. 31 """ 32 def close(self, *args, **kwargs): # real signature unknown 33 关闭文件 34 pass 35 36 def fileno(self, *args, **kwargs): # real signature unknown 37 文件描述符 38 pass 39 40 def flush(self, *args, **kwargs): # real signature unknown 41 刷新文件内部缓冲区 42 pass 43 44 def isatty(self, *args, **kwargs): # real signature unknown 45 判断文件是否是同意tty设备 46 pass 47 48 def read(self, *args, **kwargs): # real signature unknown 49 读取指定字节数据 50 pass 51 52 def readable(self, *args, **kwargs): # real signature unknown 53 是否可读 54 pass 55 56 def readline(self, *args, **kwargs): # real signature unknown 57 仅读取一行数据 58 pass 59 60 def seek(self, *args, **kwargs): # real signature unknown 61 指定文件中指针位置 62 pass 63 64 def seekable(self, *args, **kwargs): # real signature unknown 65 指针是否可操作 66 pass 67 68 def tell(self, *args, **kwargs): # real signature unknown 69 获取指针位置 70 pass 71 72 def truncate(self, *args, **kwargs): # real signature unknown 73 截断数据,仅保留指定之前数据 74 pass 75 76 def writable(self, *args, **kwargs): # real signature unknown 77 是否可写 78 pass 79 80 def write(self, *args, **kwargs): # real signature unknown 81 写内容 82 pass 83 84 def __getstate__(self, *args, **kwargs): # real signature unknown 85 pass 86 87 def __init__(self, *args, **kwargs): # real signature unknown 88 pass 89 90 @staticmethod # known case of __new__ 91 def __new__(*args, **kwargs): # real signature unknown 92 """ Create and return a new object. See help(type) for accurate signature. """ 93 pass 94 95 def __next__(self, *args, **kwargs): # real signature unknown 96 """ Implement next(self). """ 97 pass 98 99 def __repr__(self, *args, **kwargs): # real signature unknown 100 """ Return repr(self). """ 101 pass 102 103 buffer = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 104 105 closed = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 106 107 encoding = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 108 109 errors = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 110 111 line_buffering = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 112 113 name = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 114 115 newlines = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 116 117 _CHUNK_SIZE = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 118 119 _finalizing = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 120 121 3.x