import axios from "axios";
import { getOssTicket } from "@/pages/mb/constants/API";
// 拿到ticket
const getTicket = function (): any {
return new Promise((resolve, rejcet) => {
getOssTicket({}).then(res => {
resolve(res);
}).catch(error => {
rejcet(error);
})
})
}
// 上传
export const uploadPhoto = async function (neededObj: any, photos: any,cb:Function) {
const { file } = neededObj;
const data = await getTicket();
if (file) {
const uuid = getUuid();
// 取最后一个(最新添加的)
const fileItem = neededObj.fileList[neededObj.fileList.length - 1];
const first = fileItem.file.name.lastIndexOf(".");
const second = fileItem.file.name.length;
// 取后缀名
const postf = fileItem.file.name.substring(first, second);
const filePathName = data.dir + uuid + postf;
// 拿到上传完阿里云的返回信息
let uploadRes = await handleMessage(data, filePathName, neededObj.fileList)
// 这里返回一个promise,让外面调用的时候通过回调知道上传状态,做上传时候的显示交互(遮罩层上传中和关闭时机)
return new Promise((resolve,reject)=> {
// 如果状态码是200,说明上传阿里云成功了,然后把上传阿里云的这个路径通过回调函数传出去
if(uploadRes.status===200){
cb(filePathName);
resolve(true)
}
})
}
};
// 组织上传阿里云的数据
const handleMessage = function (needMessage: any, filePathName: any, listArr: any): any {
let formData = new FormData();
formData.append("key", filePathName); // OSS的保存路径
formData.append("policy", needMessage.policy); // 策略
formData.append("OSSAccessKeyId", needMessage.accessid); // OSS对象的标识
formData.append("success_action_status", "200"); // 成功返回码
formData.append("signature", needMessage.signature); // 签名
formData.append("file", listArr[listArr.length - 1].file); // 图片文件,$('input[name="pic"]').files[0]
return new Promise((reslove, rejcet) => {
axios.post(needMessage.host, formData, {
headers: {
'x-oss-object-acl': 'private'
},
}).then(res => {
reslove(res);
}).catch(err=>{
rejcet(err)
})
})
}
// 拼出不重复的数,为了确保图片不重复,也可以用时间戳
const S4 = function () {
return (((1 + Math.random()) * 0x10000) | 0).toString(16).substring(1);
}
const getUuid = function () {
return (S4() + S4() + "-" + S4() + "-" + S4() + "-" + S4() + "-" + S4() + S4() + S4());
}
接口:
// oss上传 获取ticket
export const getOssTicket = (data:Object)=>sendPost('/api/oss/assets/ticket',{})
使用:
使用:
import {uploadPhoto} from '~mb/constants/oss';
组件中使用:
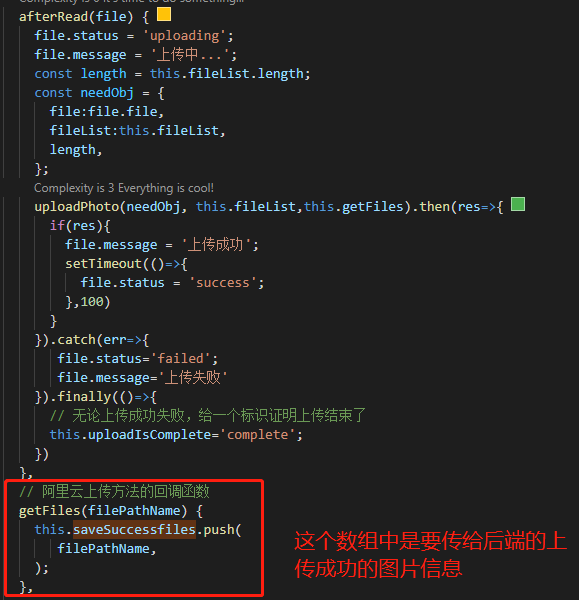