题面:
B. Hoofball
Input file: standard input
Output file: standard output
Time limit: 5 second
Memory limit: 512 megabytes
In preparation for the upcoming hoofball tournament, Farmer John is drilling his N cows (conveniently numbered 1 . . . N, where 1 ≤ N ≤ 100) in passing the ball. The cows are all standing along a very long line on one side of the barn, with cow i standing xi units away from the barn (1 ≤ xi ≤ 1000). Each cow is standing at a distinct location.
At the beginning of the drill, Farmer John will pass several balls to different cows. When cow i receives a ball, either from Farmer John or from another cow, she will pass the ball to the cow nearest her (and if multiple cows are the same distance from her, she will pass the ball to the cow farthest to the left among these). So that all cows get at least a little bit of practice passing, Farmer John wants to make sure that every cow will hold a ball at least once. Help him figure out the minimum number of balls he needs to distribute initially to ensure this can happen, assuming he hands the balls to an appropriate initial set of cows.
Input
The first line of input contains N. The second line contains N space-separated integers, where the ith integer is xi .
Output
Please output the minimum number of balls Farmer John must initially pass to the cows, so that every cow can hold a ball at least once.
Example
Input
5
7 1 3 11 4
Output
2
Note
In the above example, Farmer John should pass a ball to the cow at x = 1 and pass a ball to the cow at x = 11. The cow at x = 1 will pass her ball to the cow at x = 3, after which this ball will oscillate between the cow at x = 3 and the cow at x = 4. The cow at x = 11 will pass her ball to the cow at x = 7, who will pass the ball to the cow at x = 4, after which this ball will also cycle between the cow at x = 3 and the cow at x = 4. In this way, all cows will be passed a ball at least once (possibly by Farmer John, possibly by another cow).
It can be seen that there is no single cow to whom Farmer John could initially pass a ball so that every cow would eventually be passed a ball.
题目描述:
有n头奶牛,农夫会让不同的奶牛拿到一个球,然后凡是拿到球的奶牛会都会把球传给离它最近的奶牛,传来传去。农夫想找到最少的球,使每个牛都能拿到一次球。
题目分析:
这道题我们可以通过对题目的数据进行排序,然后从1到N头牛遍历一遍就能得到每头牛的“方向”(往左或往右走),第一头奶牛肯定是向右走的,第N头奶牛肯定是向左走的。我们用一个数组记录每头牛的方向后,怎样花费最少的球?我们可以对这些箭头的情况进行分类讨论:
第一种:这时只需要放一个球在左端或右端就行了
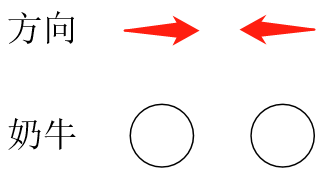
第二种:最左端放一个球
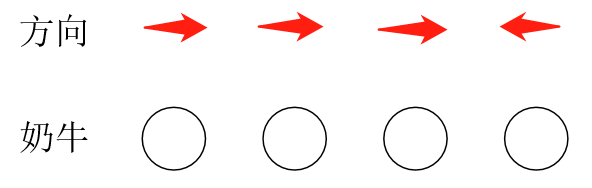
第三种:最右端放一个球
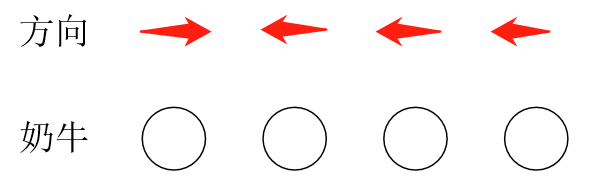
第四种:左右各放一个
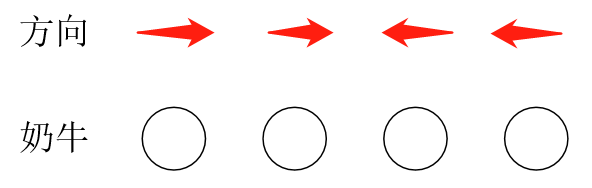
为什么会分成这四类情况呢?其实我们可以发现,分成这四种情况后,每种情况通过箭头把这些点连起来就变成了一个图,比如第一种情况通过箭头连接点就变成了这样:
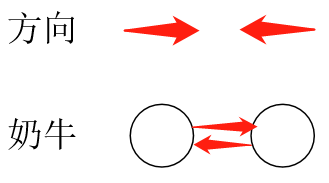
变成了一个图。我们对不同的图进行讨论。如果两个相邻的球不是同一个图,那么就分开讨论左图和有图,也就是“断开了”,比如像这样:
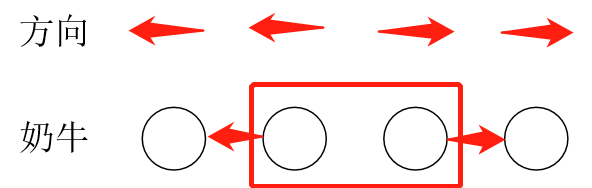
从1-N遍历一次数组记录的方向就可以解决了。
AC代码:
1 #include <cstdio> 2 #include <cstring> 3 #include <iostream> 4 #include <cmath> 5 #include <set> 6 #include <algorithm> 7 using namespace std; 8 int x[105]; 9 char s[105]; 10 int mmap[105]; 11 12 int main(){ 13 int n; 14 cin >> n; 15 for(int i = 0; i < n; i++) cin >> x[i]; 16 17 sort(x, x+n); 18 for(int i = 0; i < n; i++){ 19 if(i == 0) s[i] = '>'; //开始方向为右 20 else if(i == n-1) s[i] = '<'; //结尾方向为左 21 else{ 22 int a, b; 23 a = abs(x[i]-x[i-1]); 24 b = abs(x[i]-x[i+1]); 25 if(a > b){ 26 s[i] = '>'; 27 } 28 else if(a <= b){ 29 s[i] = '<'; 30 } 31 } 32 } 33 34 s[n] = '>'; //方便判断用,建议先看下面再上来理解这个 35 int cnt = 0; //球的数量 36 for(int i = 0; i < n; ){ 37 int flag = 0; //箭头向右的数量 38 while(s[i] == '>' && i < n) {i++; flag++;} 39 //循环说明s[i] == '<'或者遍历完了 40 if(s[i+1] == '>') {cnt++; i++;} //如果下一个点是另一个图,进行计数,然后到下一个点(这个包含第一种和第二种情况) 41 else{ 42 while(s[i] == '<' && i < n) i++; 43 if(flag > 1) cnt += 2; //两个以上向右的箭头(此时这个图向左的箭头超过了两个才会到这一步),就是第四种情况 44 else cnt++; //第三种情况 45 } 46 } 47 48 cout << cnt << endl; 49 return 0; 50 }