Power Network
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 31086 | Accepted: 15986 |
题目链接:http://poj.org/problem?id=1459
Description:
A power network consists of nodes (power stations, consumers and dispatchers) connected by power transport lines. A node u may be supplied with an amount s(u) >= 0 of power, may produce an amount 0 <= p(u) <= pmax(u) of power, may consume an amount 0 <= c(u) <= min(s(u),cmax(u)) of power, and may deliver an amount d(u)=s(u)+p(u)-c(u) of power. The following restrictions apply: c(u)=0 for any power station, p(u)=0 for any consumer, and p(u)=c(u)=0 for any dispatcher. There is at most one power transport line (u,v) from a node u to a node v in the net; it transports an amount 0 <= l(u,v) <= lmax(u,v) of power delivered by u to v. Let Con=Σuc(u) be the power consumed in the net. The problem is to compute the maximum value of Con.
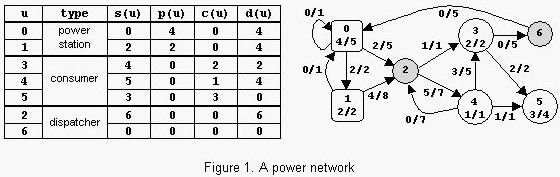
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input:
There are several data sets in the input. Each data set encodes a power network. It starts with four integers: 0 <= n <= 100 (nodes), 0 <= np <= n (power stations), 0 <= nc <= n (consumers), and 0 <= m <= n^2 (power transport lines). Follow m data triplets (u,v)z, where u and v are node identifiers (starting from 0) and 0 <= z <= 1000 is the value of lmax(u,v). Follow np doublets (u)z, where u is the identifier of a power station and 0 <= z <= 10000 is the value of pmax(u). The data set ends with nc doublets (u)z, where u is the identifier of a consumer and 0 <= z <= 10000 is the value of cmax(u). All input numbers are integers. Except the (u,v)z triplets and the (u)z doublets, which do not contain white spaces, white spaces can occur freely in input. Input data terminate with an end of file and are correct.
Output:
For each data set from the input, the program prints on the standard output the maximum amount of power that can be consumed in the corresponding network. Each result has an integral value and is printed from the beginning of a separate line.
Sample Input:
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20 7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7 (3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5 (0)5 (1)2 (3)2 (4)1 (5)4
Sample Output:
15 6
Hint:
The sample input contains two data sets. The first data set encodes a network with 2 nodes, power station 0 with pmax(0)=15 and consumer 1 with cmax(1)=20, and 2 power transport lines with lmax(0,1)=20 and lmax(1,0)=10. The maximum value of Con is 15. The second data set encodes the network from figure 1.
题意:
有三种点,一种是生产者,一种是消费者,一种是中转站,经过生产者的流会增加,经过消费者的流会减少,中转站就不会增加也不会减小。
问被消费者减少的流最大为多少。
题解:
我们知道,在一个网络流中,除开源点和汇点,其它点的进出流值都是相等的。但在这里,经过生产者流会增加,经过消费者流会减少。
所以我们考虑给每个生产者连一条边,权值就为其生产的权值;而给每个消费者连出一条边,权值为其消耗的权值。这样就可以满足流网络的性质。
最后就会多出两个点,分别为超级源点和超级汇点,我们要求连出的边上流量的最大值,所以不妨让消费者与超级汇点相连。
最后直接跑个最大流就好了。
注意下输入。
代码如下:
#include <cstdio> #include <cstring> #include <algorithm> #include <iostream> #include <queue> #define INF 99999999 #define t 200 using namespace std; typedef long long ll; const int N = 205; int head[N],d[N]; int tot,n,np,nc,m; struct Edge{ int v,c,next; }e[(N*N)<<1]; void adde(int u,int v,int w){ e[tot].v=v;e[tot].c=w;e[tot].next=head[u];head[u]=tot++; e[tot].v=u;e[tot].c=0;e[tot].next=head[v];head[v]=tot++; } int bfs(){ memset(d,0,sizeof(d));d[0]=1; queue <int > q;q.push(0); while(!q.empty()){ int u=q.front();q.pop(); for(int i=head[u];i!=-1;i=e[i].next){ int v=e[i].v; if(e[i].c>0 && !d[v]){ d[v]=d[u]+1; q.push(v); } } } return d[t]!=0; } int dfs(int s,int a){ if(s==t || a==0) return a; int flow=0,f; for(int i=head[s];i!=-1;i=e[i].next){ int v=e[i].v; if(d[v]!=d[s]+1) continue ; f=dfs(v,min(a,e[i].c)); if(f>0){ e[i].c-=f; e[i^1].c+=f; flow+=f; a-=f; if(a==0) break; } } if(!flow) d[s]=-1; return flow; } int Dinic(){ int flow=0; while(bfs()) flow+=dfs(0,INF); return flow; } int main(){ while(scanf("%d%d%d%d",&n,&np,&nc,&m)!=EOF){ tot=0;memset(head,-1,sizeof(head)); for(int i=1,u,v,w;i<=m;i++){ while(getchar()!='('); scanf("%d,%d)%d",&u,&v,&w); u++;v++; adde(u,v,w); } for(int i=1,u,w;i<=np;i++){ while(getchar()!='('); scanf("%d)%d",&u,&w); u++; adde(0,u,w); } for(int i=1,v,w;i<=nc;i++){ while(getchar()!='('); scanf("%d)%d",&v,&w); v++; adde(v,t,w); } printf("%d ",Dinic()); } return 0; }