题目:
Description
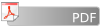
A friend of you is doing research on the Traveling Knight Problem (TKP) where you are to find the shortest closed tour of knight moves that visits each square of a given set of n squares on a chessboard exactly once. He thinks that the most difficult part of the problem is determining the smallest number of knight moves between two given squares and that, once you have accomplished this, finding the tour would be easy.
Of course you know that it is vice versa. So you offer him to write a program that solves the "difficult" part.
Your job is to write a program that takes two squares a and b as input and then determines the number of knight moves on a shortest route from a to b.
Input Specification
The input file will contain one or more test cases. Each test case consists of one line containing two squares separated by one space. A square is a string consisting of a letter (a-h) representing the column and a digit (1-8) representing the row on the chessboard.
Output Specification
For each test case, print one line saying "To get from xx to yy takes n knight moves.".
题目大意:给你个8*8的方格,给你开始的坐标和目标,最短的步数。
解题思路:直接用宽域搜索法。
代码:
1 #include<iostream>
2 #include<queue>
3 #include<string>
4 #include<cstring>
5 using namespace std;
6 int bad[10][10];
7 int t;
8 int check(int x,int y)
9 {
10 if(x<0 || y<0 || x>=8 || y>=8 || bad[x][y])
11 return 1;
12 return 0;
13 }
14 struct node
15 {
16 int x;
17 int y;
18 int t;
19 }temp,temp1,temp2;
20 int main()
21 {
22 string a,c;
23 int dx,dy,i;
24 while(cin>>a>>c)
25 {
26 memset(bad,0,sizeof(bad));
27 dx=c[0]-'a';
28 dy=c[1]-'1';
29 struct node temp={a[0]-'a',a[1]-'1',0};
30 bad[temp.x][temp.y]=1;
31 queue<node>q;
32 q.push(temp);
33 while(!q.empty())
34 {
35 temp1=q.front();
36 q.pop();
37 if(temp1.x==dx&&temp1.y==dy)
38 {
39 cout<<"To get from "<<a<<" to "<<c<<" takes "<<temp1.t<<" knight moves."<<endl;
40 break;
41 }
42 for( i=0;i<8;i++)
43 {
44 int go[8][2]={1,2 ,1,-2 ,-1,2, -1,-2, 2,1, 2,-1, -2,-1, -2,1};
45 temp2.x=temp1.x+go[i][0];
46 temp2.y=temp1.y+go[i][1];
47 temp2.t=temp1.t+1;
48 if(check(temp2.x,temp2.y))
49 continue;
50 bad[temp2.x][temp2.y]=1;
51 q.push(temp2);
52 }
53 }
54
55
56 }
57 return 0;
58 }