So Easy!
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 3459 Accepted Submission(s): 1113
Problem Description
A sequence Sn is defined as:
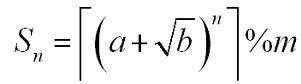
Where a, b, n, m are positive integers.┌x┐is the ceil of x. For example, ┌3.14┐=4. You are to calculate Sn.
You, a top coder, say: So easy!
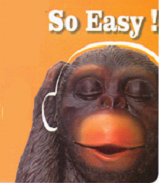
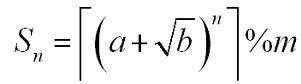
Where a, b, n, m are positive integers.┌x┐is the ceil of x. For example, ┌3.14┐=4. You are to calculate Sn.
You, a top coder, say: So easy!
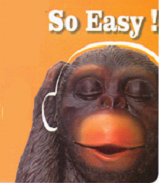
Input
There are several test cases, each test case in one line contains four positive integers: a, b, n, m. Where 0< a, m < 215, (a-1)2< b < a2, 0 < b, n < 231.The input will finish with the end of file.
Output
For each the case, output an integer Sn.
Sample Input
2 3 1 2013
2 3 2 2013
2 2 1 2013
Sample Output
4
14
4
Source
解析:矩阵快速幂。
根据共轭式的原理,(a+√b)n+(a-√b)n必为整数。
而(a-1)2< b < a2
=> a-1< √b < a
=> 0< a-√b <1
=> 0< (a-√b)n <1
所以(a+√b)n+(a-√b)n = ceil( (a+√b)n )。
接下来就可以运用矩阵快速幂求解。
1 #include <cstdio> 2 #include <cstring> 3 4 long long a,b,n,m; 5 6 struct Mat1{ 7 long long mat[2][2]; 8 }; 9 10 struct Mat2{ 11 long long mat[2][1]; 12 }; 13 14 Mat1 operator * (Mat1 A,Mat1 B) 15 { 16 Mat1 ret; 17 memset(ret.mat,0,sizeof(ret)); 18 for(int i = 0; i<2; ++i) 19 for(int j = 0; j<2; ++j) 20 for(int k = 0; k<2; ++k) 21 ret.mat[i][j] = (ret.mat[i][j]+A.mat[i][k]*B.mat[k][j])%m; 22 return ret; 23 } 24 25 Mat2 operator * (Mat1 A,Mat2 B) 26 { 27 Mat2 ret; 28 memset(ret.mat,0,sizeof(ret)); 29 for(int i = 0; i<2; ++i) 30 for(int j = 0; j<1; ++j) 31 for(int k = 0; k<2; ++k) 32 ret.mat[i][j] = (ret.mat[i][j]+A.mat[i][k]*B.mat[k][j])%m; 33 return ret; 34 } 35 36 void matquickpowmod() 37 { 38 Mat1 x; 39 x.mat[0][0] = 2*a; 40 x.mat[0][1] = b-a*a; 41 x.mat[1][0] = 1; 42 x.mat[1][1] = 0; 43 Mat2 y; 44 y.mat[0][0] = 2*a; 45 y.mat[1][0] = 2; 46 while(n){ 47 if(n&1) 48 y = x*y; 49 x = x*x; 50 n >>= 1; 51 } 52 printf("%I64d ",(y.mat[1][0]%m+m)%m); 53 } 54 55 int main() 56 { 57 while(~scanf("%I64d%I64d%I64d%I64d",&a,&b,&n,&m)){ 58 matquickpowmod(); 59 } 60 return 0; 61 }